Taking multiple substrings from a single line in a .txt file in C#
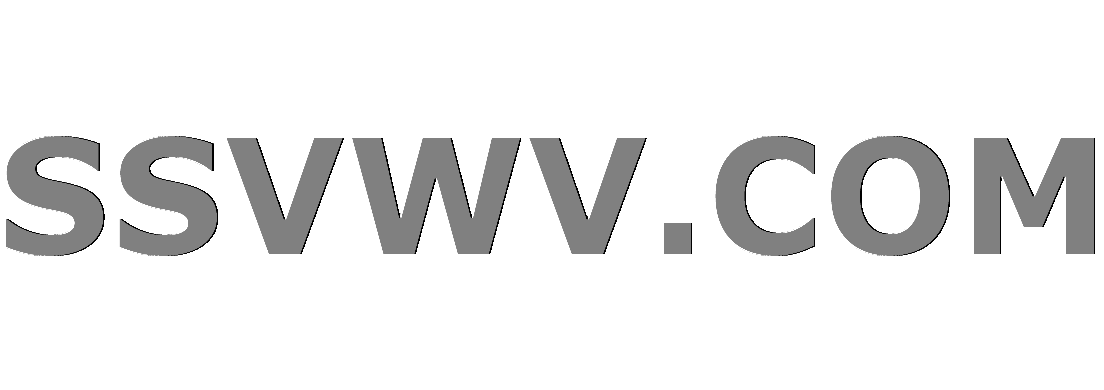
Multi tool use
up vote
1
down vote
favorite
Part of a class assignment I'm working on requires me to take isolate certain parts of a "students" info from a .txt file such as last name, first name, and gpa. While I can get the last name to appear properly, going beyond that is iffy, first names will be cut off or go to the middle initial seemingly randomly. I need help with having consistent cut off points based on markers (apostrophes) in the lines.
this is a sample of the .txt file Students2 which is located in the program's Bin Debug
(LIST (LIST 'Abbott 'Ashley 'J ) '8697387888 'ajabbott@mail.usi.edu 2.3073320999676614 )
(LIST (LIST 'Abbott 'Bradley 'M ) 'NONE 'bmabbott@mail.usi.edu 3.1915725161177115 )
(LIST (LIST 'Abbott 'Ryan 'T ) '8698689793 'rtabbott@mail.usi.edu 3.448215586562192 )
(LIST (LIST 'Abel 'Heather 'M ) '8698689386 'hmabel@mail.usi.edu 3.2517764202656974 )
Hopefully what I meant by markers makes sense, and the info is fake if anyone wondered.
The following is my current code, some of which was provided in an example.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
namespace ConsoleApplication61
class Program
static void Main(string args)
StreamReader inFile;
string inLine;
if (File.Exists("Students2.txt"))
try
using (StreamWriter Malachi = File.AppendText("Students2.txt"))
Malachi.WriteLine("(LIST (LIST 'Constant 'Malachi 'J ) '1832878847 'mconstant@mail.usi.edu 4.0000000000000000 )");
inFile = new StreamReader("Students2.txt");
while ((inLine = inFile.ReadLine()) != null)
int start = inLine.IndexOf("'");
if (start >= 0)
inLine = inLine.Substring(start);
int end = inLine.IndexOf(" ");
string lastname = inLine.Substring(0, end);
int endTwo = inLine.IndexOf(" ");
string firstname = inLine.Substring(end, endTwo);
int endThree = inLine.IndexOf(" ");
string email = inLine.Substring(endTwo, endThree);
Console.WriteLine( lastname + firstname );
catch (System.IO.IOException exc)
Console.WriteLine("Error");
Again I'd like to know what I'm doing wrong in regards to cutting off at specific points. Any and all help is greatly appreciated.
c# substring
add a comment |
up vote
1
down vote
favorite
Part of a class assignment I'm working on requires me to take isolate certain parts of a "students" info from a .txt file such as last name, first name, and gpa. While I can get the last name to appear properly, going beyond that is iffy, first names will be cut off or go to the middle initial seemingly randomly. I need help with having consistent cut off points based on markers (apostrophes) in the lines.
this is a sample of the .txt file Students2 which is located in the program's Bin Debug
(LIST (LIST 'Abbott 'Ashley 'J ) '8697387888 'ajabbott@mail.usi.edu 2.3073320999676614 )
(LIST (LIST 'Abbott 'Bradley 'M ) 'NONE 'bmabbott@mail.usi.edu 3.1915725161177115 )
(LIST (LIST 'Abbott 'Ryan 'T ) '8698689793 'rtabbott@mail.usi.edu 3.448215586562192 )
(LIST (LIST 'Abel 'Heather 'M ) '8698689386 'hmabel@mail.usi.edu 3.2517764202656974 )
Hopefully what I meant by markers makes sense, and the info is fake if anyone wondered.
The following is my current code, some of which was provided in an example.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
namespace ConsoleApplication61
class Program
static void Main(string args)
StreamReader inFile;
string inLine;
if (File.Exists("Students2.txt"))
try
using (StreamWriter Malachi = File.AppendText("Students2.txt"))
Malachi.WriteLine("(LIST (LIST 'Constant 'Malachi 'J ) '1832878847 'mconstant@mail.usi.edu 4.0000000000000000 )");
inFile = new StreamReader("Students2.txt");
while ((inLine = inFile.ReadLine()) != null)
int start = inLine.IndexOf("'");
if (start >= 0)
inLine = inLine.Substring(start);
int end = inLine.IndexOf(" ");
string lastname = inLine.Substring(0, end);
int endTwo = inLine.IndexOf(" ");
string firstname = inLine.Substring(end, endTwo);
int endThree = inLine.IndexOf(" ");
string email = inLine.Substring(endTwo, endThree);
Console.WriteLine( lastname + firstname );
catch (System.IO.IOException exc)
Console.WriteLine("Error");
Again I'd like to know what I'm doing wrong in regards to cutting off at specific points. Any and all help is greatly appreciated.
c# substring
2
why not just split the string based on ' and use array index to get values?
– Karthik Ganesan
Nov 9 at 19:27
It seems you think thatinLine.IndexOf(" ");
gives different results when you call it repeatedly on the same string/line. Why would you think so? Please verify your assumptions by reading the documentation ofstring.IndexOf()
(docs.microsoft.com/en-gb/dotnet/api/…) and spend some time observing what your code does in the debugger (Learn to debug using Visual Studio)
– elgonzo
Nov 9 at 19:31
It's an interesting problem (particularly since I worked in Lisp for two years way back in the late 90s). I believe the nested lists precludes using regular expressions, and it will also make a simple "look for markers" strategy very complicated. I think you will need a simple recursive parser (that recognizes three types, Lists, strings (that start with apostrophes) and numbers (that don't).
– Flydog57
Nov 9 at 19:36
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
Part of a class assignment I'm working on requires me to take isolate certain parts of a "students" info from a .txt file such as last name, first name, and gpa. While I can get the last name to appear properly, going beyond that is iffy, first names will be cut off or go to the middle initial seemingly randomly. I need help with having consistent cut off points based on markers (apostrophes) in the lines.
this is a sample of the .txt file Students2 which is located in the program's Bin Debug
(LIST (LIST 'Abbott 'Ashley 'J ) '8697387888 'ajabbott@mail.usi.edu 2.3073320999676614 )
(LIST (LIST 'Abbott 'Bradley 'M ) 'NONE 'bmabbott@mail.usi.edu 3.1915725161177115 )
(LIST (LIST 'Abbott 'Ryan 'T ) '8698689793 'rtabbott@mail.usi.edu 3.448215586562192 )
(LIST (LIST 'Abel 'Heather 'M ) '8698689386 'hmabel@mail.usi.edu 3.2517764202656974 )
Hopefully what I meant by markers makes sense, and the info is fake if anyone wondered.
The following is my current code, some of which was provided in an example.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
namespace ConsoleApplication61
class Program
static void Main(string args)
StreamReader inFile;
string inLine;
if (File.Exists("Students2.txt"))
try
using (StreamWriter Malachi = File.AppendText("Students2.txt"))
Malachi.WriteLine("(LIST (LIST 'Constant 'Malachi 'J ) '1832878847 'mconstant@mail.usi.edu 4.0000000000000000 )");
inFile = new StreamReader("Students2.txt");
while ((inLine = inFile.ReadLine()) != null)
int start = inLine.IndexOf("'");
if (start >= 0)
inLine = inLine.Substring(start);
int end = inLine.IndexOf(" ");
string lastname = inLine.Substring(0, end);
int endTwo = inLine.IndexOf(" ");
string firstname = inLine.Substring(end, endTwo);
int endThree = inLine.IndexOf(" ");
string email = inLine.Substring(endTwo, endThree);
Console.WriteLine( lastname + firstname );
catch (System.IO.IOException exc)
Console.WriteLine("Error");
Again I'd like to know what I'm doing wrong in regards to cutting off at specific points. Any and all help is greatly appreciated.
c# substring
Part of a class assignment I'm working on requires me to take isolate certain parts of a "students" info from a .txt file such as last name, first name, and gpa. While I can get the last name to appear properly, going beyond that is iffy, first names will be cut off or go to the middle initial seemingly randomly. I need help with having consistent cut off points based on markers (apostrophes) in the lines.
this is a sample of the .txt file Students2 which is located in the program's Bin Debug
(LIST (LIST 'Abbott 'Ashley 'J ) '8697387888 'ajabbott@mail.usi.edu 2.3073320999676614 )
(LIST (LIST 'Abbott 'Bradley 'M ) 'NONE 'bmabbott@mail.usi.edu 3.1915725161177115 )
(LIST (LIST 'Abbott 'Ryan 'T ) '8698689793 'rtabbott@mail.usi.edu 3.448215586562192 )
(LIST (LIST 'Abel 'Heather 'M ) '8698689386 'hmabel@mail.usi.edu 3.2517764202656974 )
Hopefully what I meant by markers makes sense, and the info is fake if anyone wondered.
The following is my current code, some of which was provided in an example.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
namespace ConsoleApplication61
class Program
static void Main(string args)
StreamReader inFile;
string inLine;
if (File.Exists("Students2.txt"))
try
using (StreamWriter Malachi = File.AppendText("Students2.txt"))
Malachi.WriteLine("(LIST (LIST 'Constant 'Malachi 'J ) '1832878847 'mconstant@mail.usi.edu 4.0000000000000000 )");
inFile = new StreamReader("Students2.txt");
while ((inLine = inFile.ReadLine()) != null)
int start = inLine.IndexOf("'");
if (start >= 0)
inLine = inLine.Substring(start);
int end = inLine.IndexOf(" ");
string lastname = inLine.Substring(0, end);
int endTwo = inLine.IndexOf(" ");
string firstname = inLine.Substring(end, endTwo);
int endThree = inLine.IndexOf(" ");
string email = inLine.Substring(endTwo, endThree);
Console.WriteLine( lastname + firstname );
catch (System.IO.IOException exc)
Console.WriteLine("Error");
Again I'd like to know what I'm doing wrong in regards to cutting off at specific points. Any and all help is greatly appreciated.
c# substring
c# substring
asked Nov 9 at 19:23
W. Schmitt
113
113
2
why not just split the string based on ' and use array index to get values?
– Karthik Ganesan
Nov 9 at 19:27
It seems you think thatinLine.IndexOf(" ");
gives different results when you call it repeatedly on the same string/line. Why would you think so? Please verify your assumptions by reading the documentation ofstring.IndexOf()
(docs.microsoft.com/en-gb/dotnet/api/…) and spend some time observing what your code does in the debugger (Learn to debug using Visual Studio)
– elgonzo
Nov 9 at 19:31
It's an interesting problem (particularly since I worked in Lisp for two years way back in the late 90s). I believe the nested lists precludes using regular expressions, and it will also make a simple "look for markers" strategy very complicated. I think you will need a simple recursive parser (that recognizes three types, Lists, strings (that start with apostrophes) and numbers (that don't).
– Flydog57
Nov 9 at 19:36
add a comment |
2
why not just split the string based on ' and use array index to get values?
– Karthik Ganesan
Nov 9 at 19:27
It seems you think thatinLine.IndexOf(" ");
gives different results when you call it repeatedly on the same string/line. Why would you think so? Please verify your assumptions by reading the documentation ofstring.IndexOf()
(docs.microsoft.com/en-gb/dotnet/api/…) and spend some time observing what your code does in the debugger (Learn to debug using Visual Studio)
– elgonzo
Nov 9 at 19:31
It's an interesting problem (particularly since I worked in Lisp for two years way back in the late 90s). I believe the nested lists precludes using regular expressions, and it will also make a simple "look for markers" strategy very complicated. I think you will need a simple recursive parser (that recognizes three types, Lists, strings (that start with apostrophes) and numbers (that don't).
– Flydog57
Nov 9 at 19:36
2
2
why not just split the string based on ' and use array index to get values?
– Karthik Ganesan
Nov 9 at 19:27
why not just split the string based on ' and use array index to get values?
– Karthik Ganesan
Nov 9 at 19:27
It seems you think that
inLine.IndexOf(" ");
gives different results when you call it repeatedly on the same string/line. Why would you think so? Please verify your assumptions by reading the documentation of string.IndexOf()
(docs.microsoft.com/en-gb/dotnet/api/…) and spend some time observing what your code does in the debugger (Learn to debug using Visual Studio)– elgonzo
Nov 9 at 19:31
It seems you think that
inLine.IndexOf(" ");
gives different results when you call it repeatedly on the same string/line. Why would you think so? Please verify your assumptions by reading the documentation of string.IndexOf()
(docs.microsoft.com/en-gb/dotnet/api/…) and spend some time observing what your code does in the debugger (Learn to debug using Visual Studio)– elgonzo
Nov 9 at 19:31
It's an interesting problem (particularly since I worked in Lisp for two years way back in the late 90s). I believe the nested lists precludes using regular expressions, and it will also make a simple "look for markers" strategy very complicated. I think you will need a simple recursive parser (that recognizes three types, Lists, strings (that start with apostrophes) and numbers (that don't).
– Flydog57
Nov 9 at 19:36
It's an interesting problem (particularly since I worked in Lisp for two years way back in the late 90s). I believe the nested lists precludes using regular expressions, and it will also make a simple "look for markers" strategy very complicated. I think you will need a simple recursive parser (that recognizes three types, Lists, strings (that start with apostrophes) and numbers (that don't).
– Flydog57
Nov 9 at 19:36
add a comment |
3 Answers
3
active
oldest
votes
up vote
2
down vote
you can use the split function and replace function to get what you are looking for something like this
string test = "(LIST (LIST 'Constant 'Malachi 'J ) '1832878847 'mconstant@mail.usi.edu 4.0000000000000000 )";
test = test.Replace(")","");
string abc = test.Split(new string "'" , StringSplitOptions.None);
Console.WriteLine("Last Name =" + abc[1]);
Console.WriteLine("First Name =" + abc[2]);
Console.WriteLine("Middle Initial =" + abc[3]);
Console.WriteLine("Email gpa =" + abc[abc.Length-1]);
UPDATE
In case there is no apostrophe for gpa you can get those values as below, just replace the last line with this
Console.WriteLine("Email =" + (abc[abc.Length-1]).Split(' ')[0]);
Console.WriteLine("gpa =" + (abc[abc.Length-1]).Split(' ')[1]);
Here is a fiddle for it https://dotnetfiddle.net/vJYLXW
2
Well, that's a lot simpler than what I was thinking about - but not as general. +1 to you!
– Flydog57
Nov 9 at 19:48
Unfortunatelly your code gives incorrect email address.
– Pati
Nov 9 at 20:17
@Pati Your string doesn't have a apostrophe to differentiate between email and gpa if that's the case I have updated the fiddle accordingly
– Karthik Ganesan
Nov 9 at 20:22
add a comment |
up vote
1
down vote
you need to update inLine every time you take off substring like -
int start = inLine.IndexOf("'");
if (start >= 0)
inLine = inLine.Substring(start);
int end = inLine.IndexOf(" ");
string lastname = inLine.Substring(0, end);
inLine = inLine.Substring(end + 1);
int endTwo = inLine.IndexOf(" ");
string firstname = inLine.Substring(end, endTwo);
inLine = inLine.Substring(endTwo + 1);
int endThree = inLine.IndexOf(" ");
string email = inLine.Substring(endTwo, endThree);
.
.
and so on.....
OR you take find next space after end point of first string like this -
int start = inLine.IndexOf("'");
if (start >= 0)
inLine = inLine.Substring(start);
int end = inLine.IndexOf(" ");
string lastname = inLine.Substring(0, end);
int endTwo = inLine.IndexOf(' ', end + 1);
string firstname = inLine.Substring(end, endTwo - end);
int endThree = inLine.IndexOf(' ', endTwo + 1);
string middleinitial = inLine.Substring(endTwo, endThree - endTwo);
endThree += 2; // to escape ')' after middle initial
int endFour = inLine.IndexOf(' ', endThree + 1);
string phone = inLine.Substring(endThree, endFour - endThree);
int endFive = inLine.IndexOf(' ', endFour + 1);
string email = inLine.Substring(endFour, endFive - endFour);
int endSix = inLine.IndexOf(' ', endFive + 1);
string gpa = inLine.Substring(endFive, endSix - endFive);
Console.WriteLine("Last Name - " + lastname);
Console.WriteLine("First Name - " + firstname);
Console.WriteLine("Middle Initial - " + middleinitial);
Console.WriteLine("Phone - " + phone);
Console.WriteLine("Email - " + email);
Console.WriteLine("GPA - " + gpa);
But I would recommend string split option. Read about String.Split Method
while ((inLine = inFile.ReadLine()) != null)
var splits = inLine.Split(new ' ', ''' , StringSplitOptions.RemoveEmptyEntries);
if (splits.Length > 0)
Console.WriteLine("Last Name - " + splits[2]);
Console.WriteLine("First Name - " + splits[3]);
Console.WriteLine("Middle Initial - " + splits[4]);
Console.WriteLine("Phone - " + splits[6]);
Console.WriteLine("Email - " + splits[7]);
Console.WriteLine("GPA - " + splits[8]);
Console.WriteLine("------------------------------------------------------");
Using the second suggestion it does this: 'Abbott 'Ashley 'J ) ' 'J ) '8697387888 ) '8697387888 'ajab '8697387888 'ajabbott@mail.usi. At this point, I've tried to print the GPA but as you can see the middle initial repeats along with the phone number
– W. Schmitt
Nov 9 at 20:30
In order to separate every element you need few adjustments. Check here.
– Poonam Shingrut
Nov 12 at 14:38
Also if you need to remove apostrophes from each element, you'll need further adjustments. String.split method is pretty straight forward and you can take out all the sub parts easily.
– Poonam Shingrut
Nov 12 at 14:43
add a comment |
up vote
0
down vote
It is much easier to get the desired fields with regexp:
Regex rPattern= new Regex(@"'([^'s]+)['s]+([^'s]+)['s]+([^'s]+)['s]+)['s]+([^'s]+)['s]+([^'s]+)['s]+([^'s]+)");
....
Match m = rPattern.Match(inLine );
if(m.Success)
string lastname = m.Groups[1].Value;
string firstname = m.Groups[2].Value;
string middlename = m.Groups[3].Value;
string phone = m.Groups[4].Value;
string email = m.Groups[5].Value;
string somenumber = m.Groups[6].Value;
Your code can be something like this:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
//PTK: don't forget include following line. IT'S IMPORTANT !!!
using System.Text.RegularExpressions;
namespace ConsoleApplication61
class Program
static void Main(string args)
StreamReader inFile;
string inLine;
//PTK: patern for your fields
Regex rPattern= new Regex(@"'([^'s]+)['s]+([^'s]+)['s]+([^'s]+)['s]+)['s]+([^'s]+)['s]+([^'s]+)['s]+([^'s]+)");
if (File.Exists("Students2.txt"))
try
using (StreamWriter Malachi = File.AppendText("Students2.txt"))
Malachi.WriteLine("(LIST (LIST 'Constant 'Malachi 'J ) '1832878847 'mconstant@mail.usi.edu 4.0000000000000000 )");
inFile = new StreamReader("Students2.txt");
while ((inLine = inFile.ReadLine()) != null)
//PTK: match the current line with the pattern
Match m = rPattern.Match(inLine );
if(m.Success)
string lastname = m.Groups[1].Value;
string firstname = m.Groups[2].Value;
string middlename = m.Groups[3].Value;
string phone = m.Groups[4].Value;
string email = m.Groups[5].Value;
string somenumber = m.Groups[6].Value;
Console.WriteLine( lastname + firstname );
catch (System.IO.IOException exc)
Console.WriteLine("Error");
You can see working demo here: https://dotnetfiddle.net/FrU4l5
add a comment |
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
you can use the split function and replace function to get what you are looking for something like this
string test = "(LIST (LIST 'Constant 'Malachi 'J ) '1832878847 'mconstant@mail.usi.edu 4.0000000000000000 )";
test = test.Replace(")","");
string abc = test.Split(new string "'" , StringSplitOptions.None);
Console.WriteLine("Last Name =" + abc[1]);
Console.WriteLine("First Name =" + abc[2]);
Console.WriteLine("Middle Initial =" + abc[3]);
Console.WriteLine("Email gpa =" + abc[abc.Length-1]);
UPDATE
In case there is no apostrophe for gpa you can get those values as below, just replace the last line with this
Console.WriteLine("Email =" + (abc[abc.Length-1]).Split(' ')[0]);
Console.WriteLine("gpa =" + (abc[abc.Length-1]).Split(' ')[1]);
Here is a fiddle for it https://dotnetfiddle.net/vJYLXW
2
Well, that's a lot simpler than what I was thinking about - but not as general. +1 to you!
– Flydog57
Nov 9 at 19:48
Unfortunatelly your code gives incorrect email address.
– Pati
Nov 9 at 20:17
@Pati Your string doesn't have a apostrophe to differentiate between email and gpa if that's the case I have updated the fiddle accordingly
– Karthik Ganesan
Nov 9 at 20:22
add a comment |
up vote
2
down vote
you can use the split function and replace function to get what you are looking for something like this
string test = "(LIST (LIST 'Constant 'Malachi 'J ) '1832878847 'mconstant@mail.usi.edu 4.0000000000000000 )";
test = test.Replace(")","");
string abc = test.Split(new string "'" , StringSplitOptions.None);
Console.WriteLine("Last Name =" + abc[1]);
Console.WriteLine("First Name =" + abc[2]);
Console.WriteLine("Middle Initial =" + abc[3]);
Console.WriteLine("Email gpa =" + abc[abc.Length-1]);
UPDATE
In case there is no apostrophe for gpa you can get those values as below, just replace the last line with this
Console.WriteLine("Email =" + (abc[abc.Length-1]).Split(' ')[0]);
Console.WriteLine("gpa =" + (abc[abc.Length-1]).Split(' ')[1]);
Here is a fiddle for it https://dotnetfiddle.net/vJYLXW
2
Well, that's a lot simpler than what I was thinking about - but not as general. +1 to you!
– Flydog57
Nov 9 at 19:48
Unfortunatelly your code gives incorrect email address.
– Pati
Nov 9 at 20:17
@Pati Your string doesn't have a apostrophe to differentiate between email and gpa if that's the case I have updated the fiddle accordingly
– Karthik Ganesan
Nov 9 at 20:22
add a comment |
up vote
2
down vote
up vote
2
down vote
you can use the split function and replace function to get what you are looking for something like this
string test = "(LIST (LIST 'Constant 'Malachi 'J ) '1832878847 'mconstant@mail.usi.edu 4.0000000000000000 )";
test = test.Replace(")","");
string abc = test.Split(new string "'" , StringSplitOptions.None);
Console.WriteLine("Last Name =" + abc[1]);
Console.WriteLine("First Name =" + abc[2]);
Console.WriteLine("Middle Initial =" + abc[3]);
Console.WriteLine("Email gpa =" + abc[abc.Length-1]);
UPDATE
In case there is no apostrophe for gpa you can get those values as below, just replace the last line with this
Console.WriteLine("Email =" + (abc[abc.Length-1]).Split(' ')[0]);
Console.WriteLine("gpa =" + (abc[abc.Length-1]).Split(' ')[1]);
Here is a fiddle for it https://dotnetfiddle.net/vJYLXW
you can use the split function and replace function to get what you are looking for something like this
string test = "(LIST (LIST 'Constant 'Malachi 'J ) '1832878847 'mconstant@mail.usi.edu 4.0000000000000000 )";
test = test.Replace(")","");
string abc = test.Split(new string "'" , StringSplitOptions.None);
Console.WriteLine("Last Name =" + abc[1]);
Console.WriteLine("First Name =" + abc[2]);
Console.WriteLine("Middle Initial =" + abc[3]);
Console.WriteLine("Email gpa =" + abc[abc.Length-1]);
UPDATE
In case there is no apostrophe for gpa you can get those values as below, just replace the last line with this
Console.WriteLine("Email =" + (abc[abc.Length-1]).Split(' ')[0]);
Console.WriteLine("gpa =" + (abc[abc.Length-1]).Split(' ')[1]);
Here is a fiddle for it https://dotnetfiddle.net/vJYLXW
edited Nov 9 at 20:22
answered Nov 9 at 19:35


Karthik Ganesan
3,10611736
3,10611736
2
Well, that's a lot simpler than what I was thinking about - but not as general. +1 to you!
– Flydog57
Nov 9 at 19:48
Unfortunatelly your code gives incorrect email address.
– Pati
Nov 9 at 20:17
@Pati Your string doesn't have a apostrophe to differentiate between email and gpa if that's the case I have updated the fiddle accordingly
– Karthik Ganesan
Nov 9 at 20:22
add a comment |
2
Well, that's a lot simpler than what I was thinking about - but not as general. +1 to you!
– Flydog57
Nov 9 at 19:48
Unfortunatelly your code gives incorrect email address.
– Pati
Nov 9 at 20:17
@Pati Your string doesn't have a apostrophe to differentiate between email and gpa if that's the case I have updated the fiddle accordingly
– Karthik Ganesan
Nov 9 at 20:22
2
2
Well, that's a lot simpler than what I was thinking about - but not as general. +1 to you!
– Flydog57
Nov 9 at 19:48
Well, that's a lot simpler than what I was thinking about - but not as general. +1 to you!
– Flydog57
Nov 9 at 19:48
Unfortunatelly your code gives incorrect email address.
– Pati
Nov 9 at 20:17
Unfortunatelly your code gives incorrect email address.
– Pati
Nov 9 at 20:17
@Pati Your string doesn't have a apostrophe to differentiate between email and gpa if that's the case I have updated the fiddle accordingly
– Karthik Ganesan
Nov 9 at 20:22
@Pati Your string doesn't have a apostrophe to differentiate between email and gpa if that's the case I have updated the fiddle accordingly
– Karthik Ganesan
Nov 9 at 20:22
add a comment |
up vote
1
down vote
you need to update inLine every time you take off substring like -
int start = inLine.IndexOf("'");
if (start >= 0)
inLine = inLine.Substring(start);
int end = inLine.IndexOf(" ");
string lastname = inLine.Substring(0, end);
inLine = inLine.Substring(end + 1);
int endTwo = inLine.IndexOf(" ");
string firstname = inLine.Substring(end, endTwo);
inLine = inLine.Substring(endTwo + 1);
int endThree = inLine.IndexOf(" ");
string email = inLine.Substring(endTwo, endThree);
.
.
and so on.....
OR you take find next space after end point of first string like this -
int start = inLine.IndexOf("'");
if (start >= 0)
inLine = inLine.Substring(start);
int end = inLine.IndexOf(" ");
string lastname = inLine.Substring(0, end);
int endTwo = inLine.IndexOf(' ', end + 1);
string firstname = inLine.Substring(end, endTwo - end);
int endThree = inLine.IndexOf(' ', endTwo + 1);
string middleinitial = inLine.Substring(endTwo, endThree - endTwo);
endThree += 2; // to escape ')' after middle initial
int endFour = inLine.IndexOf(' ', endThree + 1);
string phone = inLine.Substring(endThree, endFour - endThree);
int endFive = inLine.IndexOf(' ', endFour + 1);
string email = inLine.Substring(endFour, endFive - endFour);
int endSix = inLine.IndexOf(' ', endFive + 1);
string gpa = inLine.Substring(endFive, endSix - endFive);
Console.WriteLine("Last Name - " + lastname);
Console.WriteLine("First Name - " + firstname);
Console.WriteLine("Middle Initial - " + middleinitial);
Console.WriteLine("Phone - " + phone);
Console.WriteLine("Email - " + email);
Console.WriteLine("GPA - " + gpa);
But I would recommend string split option. Read about String.Split Method
while ((inLine = inFile.ReadLine()) != null)
var splits = inLine.Split(new ' ', ''' , StringSplitOptions.RemoveEmptyEntries);
if (splits.Length > 0)
Console.WriteLine("Last Name - " + splits[2]);
Console.WriteLine("First Name - " + splits[3]);
Console.WriteLine("Middle Initial - " + splits[4]);
Console.WriteLine("Phone - " + splits[6]);
Console.WriteLine("Email - " + splits[7]);
Console.WriteLine("GPA - " + splits[8]);
Console.WriteLine("------------------------------------------------------");
Using the second suggestion it does this: 'Abbott 'Ashley 'J ) ' 'J ) '8697387888 ) '8697387888 'ajab '8697387888 'ajabbott@mail.usi. At this point, I've tried to print the GPA but as you can see the middle initial repeats along with the phone number
– W. Schmitt
Nov 9 at 20:30
In order to separate every element you need few adjustments. Check here.
– Poonam Shingrut
Nov 12 at 14:38
Also if you need to remove apostrophes from each element, you'll need further adjustments. String.split method is pretty straight forward and you can take out all the sub parts easily.
– Poonam Shingrut
Nov 12 at 14:43
add a comment |
up vote
1
down vote
you need to update inLine every time you take off substring like -
int start = inLine.IndexOf("'");
if (start >= 0)
inLine = inLine.Substring(start);
int end = inLine.IndexOf(" ");
string lastname = inLine.Substring(0, end);
inLine = inLine.Substring(end + 1);
int endTwo = inLine.IndexOf(" ");
string firstname = inLine.Substring(end, endTwo);
inLine = inLine.Substring(endTwo + 1);
int endThree = inLine.IndexOf(" ");
string email = inLine.Substring(endTwo, endThree);
.
.
and so on.....
OR you take find next space after end point of first string like this -
int start = inLine.IndexOf("'");
if (start >= 0)
inLine = inLine.Substring(start);
int end = inLine.IndexOf(" ");
string lastname = inLine.Substring(0, end);
int endTwo = inLine.IndexOf(' ', end + 1);
string firstname = inLine.Substring(end, endTwo - end);
int endThree = inLine.IndexOf(' ', endTwo + 1);
string middleinitial = inLine.Substring(endTwo, endThree - endTwo);
endThree += 2; // to escape ')' after middle initial
int endFour = inLine.IndexOf(' ', endThree + 1);
string phone = inLine.Substring(endThree, endFour - endThree);
int endFive = inLine.IndexOf(' ', endFour + 1);
string email = inLine.Substring(endFour, endFive - endFour);
int endSix = inLine.IndexOf(' ', endFive + 1);
string gpa = inLine.Substring(endFive, endSix - endFive);
Console.WriteLine("Last Name - " + lastname);
Console.WriteLine("First Name - " + firstname);
Console.WriteLine("Middle Initial - " + middleinitial);
Console.WriteLine("Phone - " + phone);
Console.WriteLine("Email - " + email);
Console.WriteLine("GPA - " + gpa);
But I would recommend string split option. Read about String.Split Method
while ((inLine = inFile.ReadLine()) != null)
var splits = inLine.Split(new ' ', ''' , StringSplitOptions.RemoveEmptyEntries);
if (splits.Length > 0)
Console.WriteLine("Last Name - " + splits[2]);
Console.WriteLine("First Name - " + splits[3]);
Console.WriteLine("Middle Initial - " + splits[4]);
Console.WriteLine("Phone - " + splits[6]);
Console.WriteLine("Email - " + splits[7]);
Console.WriteLine("GPA - " + splits[8]);
Console.WriteLine("------------------------------------------------------");
Using the second suggestion it does this: 'Abbott 'Ashley 'J ) ' 'J ) '8697387888 ) '8697387888 'ajab '8697387888 'ajabbott@mail.usi. At this point, I've tried to print the GPA but as you can see the middle initial repeats along with the phone number
– W. Schmitt
Nov 9 at 20:30
In order to separate every element you need few adjustments. Check here.
– Poonam Shingrut
Nov 12 at 14:38
Also if you need to remove apostrophes from each element, you'll need further adjustments. String.split method is pretty straight forward and you can take out all the sub parts easily.
– Poonam Shingrut
Nov 12 at 14:43
add a comment |
up vote
1
down vote
up vote
1
down vote
you need to update inLine every time you take off substring like -
int start = inLine.IndexOf("'");
if (start >= 0)
inLine = inLine.Substring(start);
int end = inLine.IndexOf(" ");
string lastname = inLine.Substring(0, end);
inLine = inLine.Substring(end + 1);
int endTwo = inLine.IndexOf(" ");
string firstname = inLine.Substring(end, endTwo);
inLine = inLine.Substring(endTwo + 1);
int endThree = inLine.IndexOf(" ");
string email = inLine.Substring(endTwo, endThree);
.
.
and so on.....
OR you take find next space after end point of first string like this -
int start = inLine.IndexOf("'");
if (start >= 0)
inLine = inLine.Substring(start);
int end = inLine.IndexOf(" ");
string lastname = inLine.Substring(0, end);
int endTwo = inLine.IndexOf(' ', end + 1);
string firstname = inLine.Substring(end, endTwo - end);
int endThree = inLine.IndexOf(' ', endTwo + 1);
string middleinitial = inLine.Substring(endTwo, endThree - endTwo);
endThree += 2; // to escape ')' after middle initial
int endFour = inLine.IndexOf(' ', endThree + 1);
string phone = inLine.Substring(endThree, endFour - endThree);
int endFive = inLine.IndexOf(' ', endFour + 1);
string email = inLine.Substring(endFour, endFive - endFour);
int endSix = inLine.IndexOf(' ', endFive + 1);
string gpa = inLine.Substring(endFive, endSix - endFive);
Console.WriteLine("Last Name - " + lastname);
Console.WriteLine("First Name - " + firstname);
Console.WriteLine("Middle Initial - " + middleinitial);
Console.WriteLine("Phone - " + phone);
Console.WriteLine("Email - " + email);
Console.WriteLine("GPA - " + gpa);
But I would recommend string split option. Read about String.Split Method
while ((inLine = inFile.ReadLine()) != null)
var splits = inLine.Split(new ' ', ''' , StringSplitOptions.RemoveEmptyEntries);
if (splits.Length > 0)
Console.WriteLine("Last Name - " + splits[2]);
Console.WriteLine("First Name - " + splits[3]);
Console.WriteLine("Middle Initial - " + splits[4]);
Console.WriteLine("Phone - " + splits[6]);
Console.WriteLine("Email - " + splits[7]);
Console.WriteLine("GPA - " + splits[8]);
Console.WriteLine("------------------------------------------------------");
you need to update inLine every time you take off substring like -
int start = inLine.IndexOf("'");
if (start >= 0)
inLine = inLine.Substring(start);
int end = inLine.IndexOf(" ");
string lastname = inLine.Substring(0, end);
inLine = inLine.Substring(end + 1);
int endTwo = inLine.IndexOf(" ");
string firstname = inLine.Substring(end, endTwo);
inLine = inLine.Substring(endTwo + 1);
int endThree = inLine.IndexOf(" ");
string email = inLine.Substring(endTwo, endThree);
.
.
and so on.....
OR you take find next space after end point of first string like this -
int start = inLine.IndexOf("'");
if (start >= 0)
inLine = inLine.Substring(start);
int end = inLine.IndexOf(" ");
string lastname = inLine.Substring(0, end);
int endTwo = inLine.IndexOf(' ', end + 1);
string firstname = inLine.Substring(end, endTwo - end);
int endThree = inLine.IndexOf(' ', endTwo + 1);
string middleinitial = inLine.Substring(endTwo, endThree - endTwo);
endThree += 2; // to escape ')' after middle initial
int endFour = inLine.IndexOf(' ', endThree + 1);
string phone = inLine.Substring(endThree, endFour - endThree);
int endFive = inLine.IndexOf(' ', endFour + 1);
string email = inLine.Substring(endFour, endFive - endFour);
int endSix = inLine.IndexOf(' ', endFive + 1);
string gpa = inLine.Substring(endFive, endSix - endFive);
Console.WriteLine("Last Name - " + lastname);
Console.WriteLine("First Name - " + firstname);
Console.WriteLine("Middle Initial - " + middleinitial);
Console.WriteLine("Phone - " + phone);
Console.WriteLine("Email - " + email);
Console.WriteLine("GPA - " + gpa);
But I would recommend string split option. Read about String.Split Method
while ((inLine = inFile.ReadLine()) != null)
var splits = inLine.Split(new ' ', ''' , StringSplitOptions.RemoveEmptyEntries);
if (splits.Length > 0)
Console.WriteLine("Last Name - " + splits[2]);
Console.WriteLine("First Name - " + splits[3]);
Console.WriteLine("Middle Initial - " + splits[4]);
Console.WriteLine("Phone - " + splits[6]);
Console.WriteLine("Email - " + splits[7]);
Console.WriteLine("GPA - " + splits[8]);
Console.WriteLine("------------------------------------------------------");
edited Nov 12 at 14:58
answered Nov 9 at 19:50


Poonam Shingrut
615
615
Using the second suggestion it does this: 'Abbott 'Ashley 'J ) ' 'J ) '8697387888 ) '8697387888 'ajab '8697387888 'ajabbott@mail.usi. At this point, I've tried to print the GPA but as you can see the middle initial repeats along with the phone number
– W. Schmitt
Nov 9 at 20:30
In order to separate every element you need few adjustments. Check here.
– Poonam Shingrut
Nov 12 at 14:38
Also if you need to remove apostrophes from each element, you'll need further adjustments. String.split method is pretty straight forward and you can take out all the sub parts easily.
– Poonam Shingrut
Nov 12 at 14:43
add a comment |
Using the second suggestion it does this: 'Abbott 'Ashley 'J ) ' 'J ) '8697387888 ) '8697387888 'ajab '8697387888 'ajabbott@mail.usi. At this point, I've tried to print the GPA but as you can see the middle initial repeats along with the phone number
– W. Schmitt
Nov 9 at 20:30
In order to separate every element you need few adjustments. Check here.
– Poonam Shingrut
Nov 12 at 14:38
Also if you need to remove apostrophes from each element, you'll need further adjustments. String.split method is pretty straight forward and you can take out all the sub parts easily.
– Poonam Shingrut
Nov 12 at 14:43
Using the second suggestion it does this: 'Abbott 'Ashley 'J ) ' 'J ) '8697387888 ) '8697387888 'ajab '8697387888 'ajabbott@mail.usi. At this point, I've tried to print the GPA but as you can see the middle initial repeats along with the phone number
– W. Schmitt
Nov 9 at 20:30
Using the second suggestion it does this: 'Abbott 'Ashley 'J ) ' 'J ) '8697387888 ) '8697387888 'ajab '8697387888 'ajabbott@mail.usi. At this point, I've tried to print the GPA but as you can see the middle initial repeats along with the phone number
– W. Schmitt
Nov 9 at 20:30
In order to separate every element you need few adjustments. Check here.
– Poonam Shingrut
Nov 12 at 14:38
In order to separate every element you need few adjustments. Check here.
– Poonam Shingrut
Nov 12 at 14:38
Also if you need to remove apostrophes from each element, you'll need further adjustments. String.split method is pretty straight forward and you can take out all the sub parts easily.
– Poonam Shingrut
Nov 12 at 14:43
Also if you need to remove apostrophes from each element, you'll need further adjustments. String.split method is pretty straight forward and you can take out all the sub parts easily.
– Poonam Shingrut
Nov 12 at 14:43
add a comment |
up vote
0
down vote
It is much easier to get the desired fields with regexp:
Regex rPattern= new Regex(@"'([^'s]+)['s]+([^'s]+)['s]+([^'s]+)['s]+)['s]+([^'s]+)['s]+([^'s]+)['s]+([^'s]+)");
....
Match m = rPattern.Match(inLine );
if(m.Success)
string lastname = m.Groups[1].Value;
string firstname = m.Groups[2].Value;
string middlename = m.Groups[3].Value;
string phone = m.Groups[4].Value;
string email = m.Groups[5].Value;
string somenumber = m.Groups[6].Value;
Your code can be something like this:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
//PTK: don't forget include following line. IT'S IMPORTANT !!!
using System.Text.RegularExpressions;
namespace ConsoleApplication61
class Program
static void Main(string args)
StreamReader inFile;
string inLine;
//PTK: patern for your fields
Regex rPattern= new Regex(@"'([^'s]+)['s]+([^'s]+)['s]+([^'s]+)['s]+)['s]+([^'s]+)['s]+([^'s]+)['s]+([^'s]+)");
if (File.Exists("Students2.txt"))
try
using (StreamWriter Malachi = File.AppendText("Students2.txt"))
Malachi.WriteLine("(LIST (LIST 'Constant 'Malachi 'J ) '1832878847 'mconstant@mail.usi.edu 4.0000000000000000 )");
inFile = new StreamReader("Students2.txt");
while ((inLine = inFile.ReadLine()) != null)
//PTK: match the current line with the pattern
Match m = rPattern.Match(inLine );
if(m.Success)
string lastname = m.Groups[1].Value;
string firstname = m.Groups[2].Value;
string middlename = m.Groups[3].Value;
string phone = m.Groups[4].Value;
string email = m.Groups[5].Value;
string somenumber = m.Groups[6].Value;
Console.WriteLine( lastname + firstname );
catch (System.IO.IOException exc)
Console.WriteLine("Error");
You can see working demo here: https://dotnetfiddle.net/FrU4l5
add a comment |
up vote
0
down vote
It is much easier to get the desired fields with regexp:
Regex rPattern= new Regex(@"'([^'s]+)['s]+([^'s]+)['s]+([^'s]+)['s]+)['s]+([^'s]+)['s]+([^'s]+)['s]+([^'s]+)");
....
Match m = rPattern.Match(inLine );
if(m.Success)
string lastname = m.Groups[1].Value;
string firstname = m.Groups[2].Value;
string middlename = m.Groups[3].Value;
string phone = m.Groups[4].Value;
string email = m.Groups[5].Value;
string somenumber = m.Groups[6].Value;
Your code can be something like this:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
//PTK: don't forget include following line. IT'S IMPORTANT !!!
using System.Text.RegularExpressions;
namespace ConsoleApplication61
class Program
static void Main(string args)
StreamReader inFile;
string inLine;
//PTK: patern for your fields
Regex rPattern= new Regex(@"'([^'s]+)['s]+([^'s]+)['s]+([^'s]+)['s]+)['s]+([^'s]+)['s]+([^'s]+)['s]+([^'s]+)");
if (File.Exists("Students2.txt"))
try
using (StreamWriter Malachi = File.AppendText("Students2.txt"))
Malachi.WriteLine("(LIST (LIST 'Constant 'Malachi 'J ) '1832878847 'mconstant@mail.usi.edu 4.0000000000000000 )");
inFile = new StreamReader("Students2.txt");
while ((inLine = inFile.ReadLine()) != null)
//PTK: match the current line with the pattern
Match m = rPattern.Match(inLine );
if(m.Success)
string lastname = m.Groups[1].Value;
string firstname = m.Groups[2].Value;
string middlename = m.Groups[3].Value;
string phone = m.Groups[4].Value;
string email = m.Groups[5].Value;
string somenumber = m.Groups[6].Value;
Console.WriteLine( lastname + firstname );
catch (System.IO.IOException exc)
Console.WriteLine("Error");
You can see working demo here: https://dotnetfiddle.net/FrU4l5
add a comment |
up vote
0
down vote
up vote
0
down vote
It is much easier to get the desired fields with regexp:
Regex rPattern= new Regex(@"'([^'s]+)['s]+([^'s]+)['s]+([^'s]+)['s]+)['s]+([^'s]+)['s]+([^'s]+)['s]+([^'s]+)");
....
Match m = rPattern.Match(inLine );
if(m.Success)
string lastname = m.Groups[1].Value;
string firstname = m.Groups[2].Value;
string middlename = m.Groups[3].Value;
string phone = m.Groups[4].Value;
string email = m.Groups[5].Value;
string somenumber = m.Groups[6].Value;
Your code can be something like this:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
//PTK: don't forget include following line. IT'S IMPORTANT !!!
using System.Text.RegularExpressions;
namespace ConsoleApplication61
class Program
static void Main(string args)
StreamReader inFile;
string inLine;
//PTK: patern for your fields
Regex rPattern= new Regex(@"'([^'s]+)['s]+([^'s]+)['s]+([^'s]+)['s]+)['s]+([^'s]+)['s]+([^'s]+)['s]+([^'s]+)");
if (File.Exists("Students2.txt"))
try
using (StreamWriter Malachi = File.AppendText("Students2.txt"))
Malachi.WriteLine("(LIST (LIST 'Constant 'Malachi 'J ) '1832878847 'mconstant@mail.usi.edu 4.0000000000000000 )");
inFile = new StreamReader("Students2.txt");
while ((inLine = inFile.ReadLine()) != null)
//PTK: match the current line with the pattern
Match m = rPattern.Match(inLine );
if(m.Success)
string lastname = m.Groups[1].Value;
string firstname = m.Groups[2].Value;
string middlename = m.Groups[3].Value;
string phone = m.Groups[4].Value;
string email = m.Groups[5].Value;
string somenumber = m.Groups[6].Value;
Console.WriteLine( lastname + firstname );
catch (System.IO.IOException exc)
Console.WriteLine("Error");
You can see working demo here: https://dotnetfiddle.net/FrU4l5
It is much easier to get the desired fields with regexp:
Regex rPattern= new Regex(@"'([^'s]+)['s]+([^'s]+)['s]+([^'s]+)['s]+)['s]+([^'s]+)['s]+([^'s]+)['s]+([^'s]+)");
....
Match m = rPattern.Match(inLine );
if(m.Success)
string lastname = m.Groups[1].Value;
string firstname = m.Groups[2].Value;
string middlename = m.Groups[3].Value;
string phone = m.Groups[4].Value;
string email = m.Groups[5].Value;
string somenumber = m.Groups[6].Value;
Your code can be something like this:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
//PTK: don't forget include following line. IT'S IMPORTANT !!!
using System.Text.RegularExpressions;
namespace ConsoleApplication61
class Program
static void Main(string args)
StreamReader inFile;
string inLine;
//PTK: patern for your fields
Regex rPattern= new Regex(@"'([^'s]+)['s]+([^'s]+)['s]+([^'s]+)['s]+)['s]+([^'s]+)['s]+([^'s]+)['s]+([^'s]+)");
if (File.Exists("Students2.txt"))
try
using (StreamWriter Malachi = File.AppendText("Students2.txt"))
Malachi.WriteLine("(LIST (LIST 'Constant 'Malachi 'J ) '1832878847 'mconstant@mail.usi.edu 4.0000000000000000 )");
inFile = new StreamReader("Students2.txt");
while ((inLine = inFile.ReadLine()) != null)
//PTK: match the current line with the pattern
Match m = rPattern.Match(inLine );
if(m.Success)
string lastname = m.Groups[1].Value;
string firstname = m.Groups[2].Value;
string middlename = m.Groups[3].Value;
string phone = m.Groups[4].Value;
string email = m.Groups[5].Value;
string somenumber = m.Groups[6].Value;
Console.WriteLine( lastname + firstname );
catch (System.IO.IOException exc)
Console.WriteLine("Error");
You can see working demo here: https://dotnetfiddle.net/FrU4l5
edited Nov 9 at 20:26
answered Nov 9 at 20:08


Pati
42748
42748
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53232093%2ftaking-multiple-substrings-from-a-single-line-in-a-txt-file-in-c-sharp%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
121yeIpRPrJp f9rFz9iL5BTqdV7X6xfMsQVEDt
2
why not just split the string based on ' and use array index to get values?
– Karthik Ganesan
Nov 9 at 19:27
It seems you think that
inLine.IndexOf(" ");
gives different results when you call it repeatedly on the same string/line. Why would you think so? Please verify your assumptions by reading the documentation ofstring.IndexOf()
(docs.microsoft.com/en-gb/dotnet/api/…) and spend some time observing what your code does in the debugger (Learn to debug using Visual Studio)– elgonzo
Nov 9 at 19:31
It's an interesting problem (particularly since I worked in Lisp for two years way back in the late 90s). I believe the nested lists precludes using regular expressions, and it will also make a simple "look for markers" strategy very complicated. I think you will need a simple recursive parser (that recognizes three types, Lists, strings (that start with apostrophes) and numbers (that don't).
– Flydog57
Nov 9 at 19:36