iterate SpatialPoints and SpatialPolygonsDataFrame merge in R
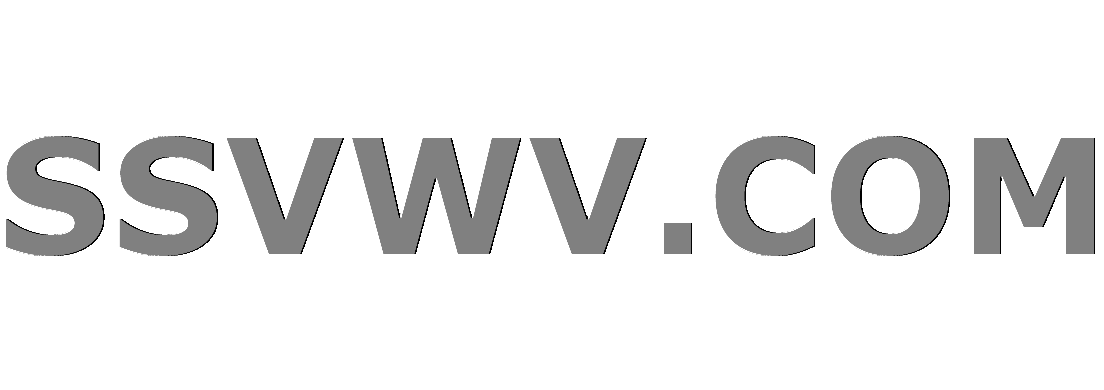
Multi tool use
I am trying to iterate thru the process of creating centroids of a list of SpatialPolygonsDataFrames
(each with several polygon features within), with the resulting SpatialPoints
preserving the attributes (data) of the parent polygons. I have tried the sp::over
function, but it seems to be problematic because centroids do not necessarily overlap with parent polygons. Furthermore, I am new to coding/R and do not know how to achieve this in a for
loop and/or using an apply
function.
So specifically, I need to (1) find a different function that will link the SpatialPolygonsDataFrames
with the associated SpatialPoints
(centroids); and (2) iterate thru the entire process and merge the SpatialPolygonsDataFrames
data with the appropriate SpatialPoints
- I do not know how to match the index value of one list to the index value in another while running a loop.
Here is a reproducible example for a single SpatialPolygonsDataFrames
object showing that using sp::over
doesn't work because some centroids do not end up overlapping parent polygons:
library(maptools) ## For wrld_simpl
library(sp)
## Example SpatialPolygonsDataFrames (SPDF)
data(wrld_simpl) #polygon of world countries
spdf1 <- wrld_simpl[1:25,] #country subset 1
spdf2 <- wrld_simpl[26:36,] #subset 2
spdf3 <- wrld_simpl[36:50,] #subset 3
spdfl[[1]]@data
#plot, so you can see it
plot(spdf1)
plot(spdf2, add=TRUE, col=4)
plot(spdf3, add=TRUE, col=3)
#make list of SPDF objects
spdfl<-list()
spdfl[[1]]<-spdf1
spdfl[[2]]<-spdf2
spdfl[[2]]<-spdf3
#create polygon centroids for each polygon feature (country) within spdfl[[1]]
spdf1c <- gCentroid(spdfl[[1]], byid=TRUE)
plot(spdfl[[1]])
plot(spdf1c, add=TRUE)
#add attributes 'NAME' and 'AREA' to SpatialPoints (centroid) object from SPDF data
spdf.NAME <- over(spdf1c, spdfl[[1]][,"NAME"])
spdf.AREA <- over(spdf1c, spdfl[[1]][,"AREA"])
spdf1c$NAME <- spdf.NAME
spdf1c$AREA <- spdf.AREA
spdf1c@data
#`sp::over` output error = name and area for ATG, ASM, BHS, and SLB are missing
r for-loop polygon spatial
add a comment |
I am trying to iterate thru the process of creating centroids of a list of SpatialPolygonsDataFrames
(each with several polygon features within), with the resulting SpatialPoints
preserving the attributes (data) of the parent polygons. I have tried the sp::over
function, but it seems to be problematic because centroids do not necessarily overlap with parent polygons. Furthermore, I am new to coding/R and do not know how to achieve this in a for
loop and/or using an apply
function.
So specifically, I need to (1) find a different function that will link the SpatialPolygonsDataFrames
with the associated SpatialPoints
(centroids); and (2) iterate thru the entire process and merge the SpatialPolygonsDataFrames
data with the appropriate SpatialPoints
- I do not know how to match the index value of one list to the index value in another while running a loop.
Here is a reproducible example for a single SpatialPolygonsDataFrames
object showing that using sp::over
doesn't work because some centroids do not end up overlapping parent polygons:
library(maptools) ## For wrld_simpl
library(sp)
## Example SpatialPolygonsDataFrames (SPDF)
data(wrld_simpl) #polygon of world countries
spdf1 <- wrld_simpl[1:25,] #country subset 1
spdf2 <- wrld_simpl[26:36,] #subset 2
spdf3 <- wrld_simpl[36:50,] #subset 3
spdfl[[1]]@data
#plot, so you can see it
plot(spdf1)
plot(spdf2, add=TRUE, col=4)
plot(spdf3, add=TRUE, col=3)
#make list of SPDF objects
spdfl<-list()
spdfl[[1]]<-spdf1
spdfl[[2]]<-spdf2
spdfl[[2]]<-spdf3
#create polygon centroids for each polygon feature (country) within spdfl[[1]]
spdf1c <- gCentroid(spdfl[[1]], byid=TRUE)
plot(spdfl[[1]])
plot(spdf1c, add=TRUE)
#add attributes 'NAME' and 'AREA' to SpatialPoints (centroid) object from SPDF data
spdf.NAME <- over(spdf1c, spdfl[[1]][,"NAME"])
spdf.AREA <- over(spdf1c, spdfl[[1]][,"AREA"])
spdf1c$NAME <- spdf.NAME
spdf1c$AREA <- spdf.AREA
spdf1c@data
#`sp::over` output error = name and area for ATG, ASM, BHS, and SLB are missing
r for-loop polygon spatial
add a comment |
I am trying to iterate thru the process of creating centroids of a list of SpatialPolygonsDataFrames
(each with several polygon features within), with the resulting SpatialPoints
preserving the attributes (data) of the parent polygons. I have tried the sp::over
function, but it seems to be problematic because centroids do not necessarily overlap with parent polygons. Furthermore, I am new to coding/R and do not know how to achieve this in a for
loop and/or using an apply
function.
So specifically, I need to (1) find a different function that will link the SpatialPolygonsDataFrames
with the associated SpatialPoints
(centroids); and (2) iterate thru the entire process and merge the SpatialPolygonsDataFrames
data with the appropriate SpatialPoints
- I do not know how to match the index value of one list to the index value in another while running a loop.
Here is a reproducible example for a single SpatialPolygonsDataFrames
object showing that using sp::over
doesn't work because some centroids do not end up overlapping parent polygons:
library(maptools) ## For wrld_simpl
library(sp)
## Example SpatialPolygonsDataFrames (SPDF)
data(wrld_simpl) #polygon of world countries
spdf1 <- wrld_simpl[1:25,] #country subset 1
spdf2 <- wrld_simpl[26:36,] #subset 2
spdf3 <- wrld_simpl[36:50,] #subset 3
spdfl[[1]]@data
#plot, so you can see it
plot(spdf1)
plot(spdf2, add=TRUE, col=4)
plot(spdf3, add=TRUE, col=3)
#make list of SPDF objects
spdfl<-list()
spdfl[[1]]<-spdf1
spdfl[[2]]<-spdf2
spdfl[[2]]<-spdf3
#create polygon centroids for each polygon feature (country) within spdfl[[1]]
spdf1c <- gCentroid(spdfl[[1]], byid=TRUE)
plot(spdfl[[1]])
plot(spdf1c, add=TRUE)
#add attributes 'NAME' and 'AREA' to SpatialPoints (centroid) object from SPDF data
spdf.NAME <- over(spdf1c, spdfl[[1]][,"NAME"])
spdf.AREA <- over(spdf1c, spdfl[[1]][,"AREA"])
spdf1c$NAME <- spdf.NAME
spdf1c$AREA <- spdf.AREA
spdf1c@data
#`sp::over` output error = name and area for ATG, ASM, BHS, and SLB are missing
r for-loop polygon spatial
I am trying to iterate thru the process of creating centroids of a list of SpatialPolygonsDataFrames
(each with several polygon features within), with the resulting SpatialPoints
preserving the attributes (data) of the parent polygons. I have tried the sp::over
function, but it seems to be problematic because centroids do not necessarily overlap with parent polygons. Furthermore, I am new to coding/R and do not know how to achieve this in a for
loop and/or using an apply
function.
So specifically, I need to (1) find a different function that will link the SpatialPolygonsDataFrames
with the associated SpatialPoints
(centroids); and (2) iterate thru the entire process and merge the SpatialPolygonsDataFrames
data with the appropriate SpatialPoints
- I do not know how to match the index value of one list to the index value in another while running a loop.
Here is a reproducible example for a single SpatialPolygonsDataFrames
object showing that using sp::over
doesn't work because some centroids do not end up overlapping parent polygons:
library(maptools) ## For wrld_simpl
library(sp)
## Example SpatialPolygonsDataFrames (SPDF)
data(wrld_simpl) #polygon of world countries
spdf1 <- wrld_simpl[1:25,] #country subset 1
spdf2 <- wrld_simpl[26:36,] #subset 2
spdf3 <- wrld_simpl[36:50,] #subset 3
spdfl[[1]]@data
#plot, so you can see it
plot(spdf1)
plot(spdf2, add=TRUE, col=4)
plot(spdf3, add=TRUE, col=3)
#make list of SPDF objects
spdfl<-list()
spdfl[[1]]<-spdf1
spdfl[[2]]<-spdf2
spdfl[[2]]<-spdf3
#create polygon centroids for each polygon feature (country) within spdfl[[1]]
spdf1c <- gCentroid(spdfl[[1]], byid=TRUE)
plot(spdfl[[1]])
plot(spdf1c, add=TRUE)
#add attributes 'NAME' and 'AREA' to SpatialPoints (centroid) object from SPDF data
spdf.NAME <- over(spdf1c, spdfl[[1]][,"NAME"])
spdf.AREA <- over(spdf1c, spdfl[[1]][,"AREA"])
spdf1c$NAME <- spdf.NAME
spdf1c$AREA <- spdf.AREA
spdf1c@data
#`sp::over` output error = name and area for ATG, ASM, BHS, and SLB are missing
r for-loop polygon spatial
r for-loop polygon spatial
asked Nov 11 '18 at 23:41
Dorothy
828
828
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
I find SF
is a great package for working with spatial data in R. I have fixed a few typos and added the right for loop below.
library(maptools) ## For wrld_simpl
library(sp)
library(sf)
## Example SpatialPolygonsDataFrames (SPDF)
data(wrld_simpl) #polygon of world countries
spdf1 <- wrld_simpl[1:25,] #country subset 1
spdf2 <- wrld_simpl[26:36,] #subset 2
spdf3 <- wrld_simpl[36:50,] #subset 3
spdfl[[1]]@data
#plot, so you can see it
plot(spdf1)
plot(spdf2, add=TRUE, col=4)
plot(spdf3, add=TRUE, col=3)
#make list of SPDF objects
spdfl<-list()
spdfl[[1]]<-spdf1
spdfl[[2]]<-spdf2
spdfl[[3]]<-spdf3
# Empty List for Centroids
centres <-list()
# For Loop
for (i in 1:length(spdfl))
centres[[i]] <- spdfl[[i]] %>%
sf::st_as_sf() %>% # translate to SF object
sf::st_centroid() %>% # get centroids
as(.,'Spatial') # If you want to keep as SF objects remove this line
#Plot your Spatial Objects
plot(spdfl[[1]])
plot(centres[[1]], add=TRUE)
Here is a solution which uses SF and sp. SF is great as it keeps things as dataframes which can easy to deal with. More information here: https://r-spatial.github.io/sf/
Thank you SO much @Michael.Brian.Gordon! Works like a charm.
– Dorothy
Nov 13 '18 at 0:34
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53254367%2fiterate-spatialpoints-and-spatialpolygonsdataframe-merge-in-r%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I find SF
is a great package for working with spatial data in R. I have fixed a few typos and added the right for loop below.
library(maptools) ## For wrld_simpl
library(sp)
library(sf)
## Example SpatialPolygonsDataFrames (SPDF)
data(wrld_simpl) #polygon of world countries
spdf1 <- wrld_simpl[1:25,] #country subset 1
spdf2 <- wrld_simpl[26:36,] #subset 2
spdf3 <- wrld_simpl[36:50,] #subset 3
spdfl[[1]]@data
#plot, so you can see it
plot(spdf1)
plot(spdf2, add=TRUE, col=4)
plot(spdf3, add=TRUE, col=3)
#make list of SPDF objects
spdfl<-list()
spdfl[[1]]<-spdf1
spdfl[[2]]<-spdf2
spdfl[[3]]<-spdf3
# Empty List for Centroids
centres <-list()
# For Loop
for (i in 1:length(spdfl))
centres[[i]] <- spdfl[[i]] %>%
sf::st_as_sf() %>% # translate to SF object
sf::st_centroid() %>% # get centroids
as(.,'Spatial') # If you want to keep as SF objects remove this line
#Plot your Spatial Objects
plot(spdfl[[1]])
plot(centres[[1]], add=TRUE)
Here is a solution which uses SF and sp. SF is great as it keeps things as dataframes which can easy to deal with. More information here: https://r-spatial.github.io/sf/
Thank you SO much @Michael.Brian.Gordon! Works like a charm.
– Dorothy
Nov 13 '18 at 0:34
add a comment |
I find SF
is a great package for working with spatial data in R. I have fixed a few typos and added the right for loop below.
library(maptools) ## For wrld_simpl
library(sp)
library(sf)
## Example SpatialPolygonsDataFrames (SPDF)
data(wrld_simpl) #polygon of world countries
spdf1 <- wrld_simpl[1:25,] #country subset 1
spdf2 <- wrld_simpl[26:36,] #subset 2
spdf3 <- wrld_simpl[36:50,] #subset 3
spdfl[[1]]@data
#plot, so you can see it
plot(spdf1)
plot(spdf2, add=TRUE, col=4)
plot(spdf3, add=TRUE, col=3)
#make list of SPDF objects
spdfl<-list()
spdfl[[1]]<-spdf1
spdfl[[2]]<-spdf2
spdfl[[3]]<-spdf3
# Empty List for Centroids
centres <-list()
# For Loop
for (i in 1:length(spdfl))
centres[[i]] <- spdfl[[i]] %>%
sf::st_as_sf() %>% # translate to SF object
sf::st_centroid() %>% # get centroids
as(.,'Spatial') # If you want to keep as SF objects remove this line
#Plot your Spatial Objects
plot(spdfl[[1]])
plot(centres[[1]], add=TRUE)
Here is a solution which uses SF and sp. SF is great as it keeps things as dataframes which can easy to deal with. More information here: https://r-spatial.github.io/sf/
Thank you SO much @Michael.Brian.Gordon! Works like a charm.
– Dorothy
Nov 13 '18 at 0:34
add a comment |
I find SF
is a great package for working with spatial data in R. I have fixed a few typos and added the right for loop below.
library(maptools) ## For wrld_simpl
library(sp)
library(sf)
## Example SpatialPolygonsDataFrames (SPDF)
data(wrld_simpl) #polygon of world countries
spdf1 <- wrld_simpl[1:25,] #country subset 1
spdf2 <- wrld_simpl[26:36,] #subset 2
spdf3 <- wrld_simpl[36:50,] #subset 3
spdfl[[1]]@data
#plot, so you can see it
plot(spdf1)
plot(spdf2, add=TRUE, col=4)
plot(spdf3, add=TRUE, col=3)
#make list of SPDF objects
spdfl<-list()
spdfl[[1]]<-spdf1
spdfl[[2]]<-spdf2
spdfl[[3]]<-spdf3
# Empty List for Centroids
centres <-list()
# For Loop
for (i in 1:length(spdfl))
centres[[i]] <- spdfl[[i]] %>%
sf::st_as_sf() %>% # translate to SF object
sf::st_centroid() %>% # get centroids
as(.,'Spatial') # If you want to keep as SF objects remove this line
#Plot your Spatial Objects
plot(spdfl[[1]])
plot(centres[[1]], add=TRUE)
Here is a solution which uses SF and sp. SF is great as it keeps things as dataframes which can easy to deal with. More information here: https://r-spatial.github.io/sf/
I find SF
is a great package for working with spatial data in R. I have fixed a few typos and added the right for loop below.
library(maptools) ## For wrld_simpl
library(sp)
library(sf)
## Example SpatialPolygonsDataFrames (SPDF)
data(wrld_simpl) #polygon of world countries
spdf1 <- wrld_simpl[1:25,] #country subset 1
spdf2 <- wrld_simpl[26:36,] #subset 2
spdf3 <- wrld_simpl[36:50,] #subset 3
spdfl[[1]]@data
#plot, so you can see it
plot(spdf1)
plot(spdf2, add=TRUE, col=4)
plot(spdf3, add=TRUE, col=3)
#make list of SPDF objects
spdfl<-list()
spdfl[[1]]<-spdf1
spdfl[[2]]<-spdf2
spdfl[[3]]<-spdf3
# Empty List for Centroids
centres <-list()
# For Loop
for (i in 1:length(spdfl))
centres[[i]] <- spdfl[[i]] %>%
sf::st_as_sf() %>% # translate to SF object
sf::st_centroid() %>% # get centroids
as(.,'Spatial') # If you want to keep as SF objects remove this line
#Plot your Spatial Objects
plot(spdfl[[1]])
plot(centres[[1]], add=TRUE)
Here is a solution which uses SF and sp. SF is great as it keeps things as dataframes which can easy to deal with. More information here: https://r-spatial.github.io/sf/
answered Nov 12 '18 at 0:43
Mwatch
363
363
Thank you SO much @Michael.Brian.Gordon! Works like a charm.
– Dorothy
Nov 13 '18 at 0:34
add a comment |
Thank you SO much @Michael.Brian.Gordon! Works like a charm.
– Dorothy
Nov 13 '18 at 0:34
Thank you SO much @Michael.Brian.Gordon! Works like a charm.
– Dorothy
Nov 13 '18 at 0:34
Thank you SO much @Michael.Brian.Gordon! Works like a charm.
– Dorothy
Nov 13 '18 at 0:34
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53254367%2fiterate-spatialpoints-and-spatialpolygonsdataframe-merge-in-r%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
lUTL,PxAlhHB97 0RKlfmg9EGkdRCQ,eQ,f M4IQ mo eby5e