How to check if a number in a list is greater than the number before it - python
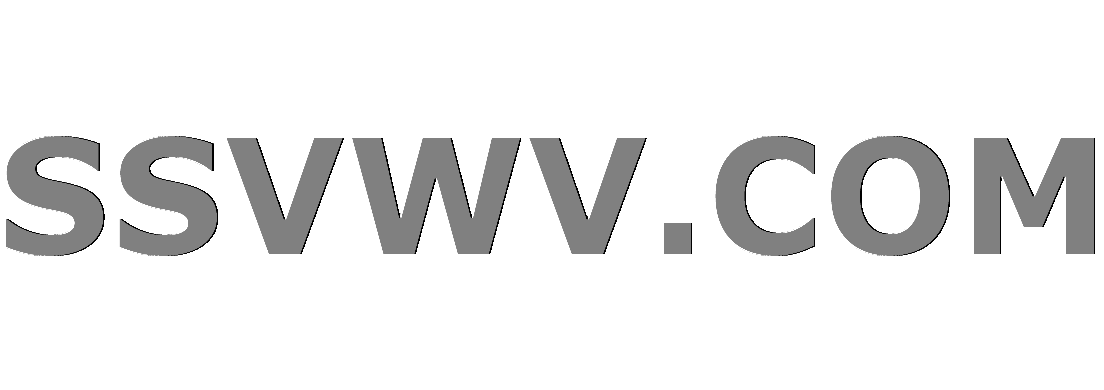
Multi tool use
I have 3 sets of data zipped together (date,somenumber,price). I want to iterated through the table and whenever somenumber is less than somenumber before it in the list I want to pull the date,somenumber,price for when that happens. Currently I just have:
for a,b,c in zip(date,somenumber,price):
print(a,b,c)
and it prints something like:
2018-01-30 18:42:00 859235 6.95
2018-02-01 09:08:00 323405 7.43
2018-02-02 15:16:00 528963 6.4
2018-02-05 18:48:00 808739 7.91
2018-02-07 14:42:00 462541 7.33
2018-02-10 18:48:00 598001 6.21
2018-02-11 03:32:00 650558 7.31
2018-02-11 11:28:00 670392 6.21
When somenumber went from 808739 to 462541 I want to return the data for that lower number:
2018-02-07 14:42:00 462541 7.33
Thanks!
python
add a comment |
I have 3 sets of data zipped together (date,somenumber,price). I want to iterated through the table and whenever somenumber is less than somenumber before it in the list I want to pull the date,somenumber,price for when that happens. Currently I just have:
for a,b,c in zip(date,somenumber,price):
print(a,b,c)
and it prints something like:
2018-01-30 18:42:00 859235 6.95
2018-02-01 09:08:00 323405 7.43
2018-02-02 15:16:00 528963 6.4
2018-02-05 18:48:00 808739 7.91
2018-02-07 14:42:00 462541 7.33
2018-02-10 18:48:00 598001 6.21
2018-02-11 03:32:00 650558 7.31
2018-02-11 11:28:00 670392 6.21
When somenumber went from 808739 to 462541 I want to return the data for that lower number:
2018-02-07 14:42:00 462541 7.33
Thanks!
python
323405 is less than 859235. Do you want to report it, too? If not, why?
– DYZ
Nov 15 '18 at 4:38
add a comment |
I have 3 sets of data zipped together (date,somenumber,price). I want to iterated through the table and whenever somenumber is less than somenumber before it in the list I want to pull the date,somenumber,price for when that happens. Currently I just have:
for a,b,c in zip(date,somenumber,price):
print(a,b,c)
and it prints something like:
2018-01-30 18:42:00 859235 6.95
2018-02-01 09:08:00 323405 7.43
2018-02-02 15:16:00 528963 6.4
2018-02-05 18:48:00 808739 7.91
2018-02-07 14:42:00 462541 7.33
2018-02-10 18:48:00 598001 6.21
2018-02-11 03:32:00 650558 7.31
2018-02-11 11:28:00 670392 6.21
When somenumber went from 808739 to 462541 I want to return the data for that lower number:
2018-02-07 14:42:00 462541 7.33
Thanks!
python
I have 3 sets of data zipped together (date,somenumber,price). I want to iterated through the table and whenever somenumber is less than somenumber before it in the list I want to pull the date,somenumber,price for when that happens. Currently I just have:
for a,b,c in zip(date,somenumber,price):
print(a,b,c)
and it prints something like:
2018-01-30 18:42:00 859235 6.95
2018-02-01 09:08:00 323405 7.43
2018-02-02 15:16:00 528963 6.4
2018-02-05 18:48:00 808739 7.91
2018-02-07 14:42:00 462541 7.33
2018-02-10 18:48:00 598001 6.21
2018-02-11 03:32:00 650558 7.31
2018-02-11 11:28:00 670392 6.21
When somenumber went from 808739 to 462541 I want to return the data for that lower number:
2018-02-07 14:42:00 462541 7.33
Thanks!
python
python
asked Nov 15 '18 at 4:35


ZakZak
413
413
323405 is less than 859235. Do you want to report it, too? If not, why?
– DYZ
Nov 15 '18 at 4:38
add a comment |
323405 is less than 859235. Do you want to report it, too? If not, why?
– DYZ
Nov 15 '18 at 4:38
323405 is less than 859235. Do you want to report it, too? If not, why?
– DYZ
Nov 15 '18 at 4:38
323405 is less than 859235. Do you want to report it, too? If not, why?
– DYZ
Nov 15 '18 at 4:38
add a comment |
2 Answers
2
active
oldest
votes
This will compare somenumber+1 to somenumber, and if somenumber + 1 (the next number in the sequence is less than the current) it will print all data for that row. If it is not less it will not print anything.
for a,b,c,d in zip(date, somenumber, somenumber[1:], price):
if c < b:
print(a, b, c, d)
add a comment |
What I understood from the question is every time, the value of somenumber
decreases in the next row, it should print the row. It's very easy to implement. Just store the previous value in another variable (prev_number
). For the first row, though, we need to initialize prev_number
to the minimum number possible. Following is the solution:
import sys
prev_number = -sys.maxint - 1 #initialize prev_number to lowest possible number in python
for a,b,c in zip(date,somenumber,price):
if b < prev_number:
print(a,b,c)
prev_number = d
Let me know if it works for you.
there is no lowest possible integer number in python:n = -sys.maxint**10
is smaller then what you used. It is also not needed:prev_number = min(somenumber)-1
would be enough and you can get rid of the import
– Patrick Artner
Feb 22 at 11:13
@PatrickArtner That's right. but here, we don't know the min range (some number) right. That's why we can use sys.maxint values.
– amulya349
Feb 25 at 9:10
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53312502%2fhow-to-check-if-a-number-in-a-list-is-greater-than-the-number-before-it-python%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
This will compare somenumber+1 to somenumber, and if somenumber + 1 (the next number in the sequence is less than the current) it will print all data for that row. If it is not less it will not print anything.
for a,b,c,d in zip(date, somenumber, somenumber[1:], price):
if c < b:
print(a, b, c, d)
add a comment |
This will compare somenumber+1 to somenumber, and if somenumber + 1 (the next number in the sequence is less than the current) it will print all data for that row. If it is not less it will not print anything.
for a,b,c,d in zip(date, somenumber, somenumber[1:], price):
if c < b:
print(a, b, c, d)
add a comment |
This will compare somenumber+1 to somenumber, and if somenumber + 1 (the next number in the sequence is less than the current) it will print all data for that row. If it is not less it will not print anything.
for a,b,c,d in zip(date, somenumber, somenumber[1:], price):
if c < b:
print(a, b, c, d)
This will compare somenumber+1 to somenumber, and if somenumber + 1 (the next number in the sequence is less than the current) it will print all data for that row. If it is not less it will not print anything.
for a,b,c,d in zip(date, somenumber, somenumber[1:], price):
if c < b:
print(a, b, c, d)
edited Nov 15 '18 at 4:47
answered Nov 15 '18 at 4:41
d_kennetzd_kennetz
2,2483927
2,2483927
add a comment |
add a comment |
What I understood from the question is every time, the value of somenumber
decreases in the next row, it should print the row. It's very easy to implement. Just store the previous value in another variable (prev_number
). For the first row, though, we need to initialize prev_number
to the minimum number possible. Following is the solution:
import sys
prev_number = -sys.maxint - 1 #initialize prev_number to lowest possible number in python
for a,b,c in zip(date,somenumber,price):
if b < prev_number:
print(a,b,c)
prev_number = d
Let me know if it works for you.
there is no lowest possible integer number in python:n = -sys.maxint**10
is smaller then what you used. It is also not needed:prev_number = min(somenumber)-1
would be enough and you can get rid of the import
– Patrick Artner
Feb 22 at 11:13
@PatrickArtner That's right. but here, we don't know the min range (some number) right. That's why we can use sys.maxint values.
– amulya349
Feb 25 at 9:10
add a comment |
What I understood from the question is every time, the value of somenumber
decreases in the next row, it should print the row. It's very easy to implement. Just store the previous value in another variable (prev_number
). For the first row, though, we need to initialize prev_number
to the minimum number possible. Following is the solution:
import sys
prev_number = -sys.maxint - 1 #initialize prev_number to lowest possible number in python
for a,b,c in zip(date,somenumber,price):
if b < prev_number:
print(a,b,c)
prev_number = d
Let me know if it works for you.
there is no lowest possible integer number in python:n = -sys.maxint**10
is smaller then what you used. It is also not needed:prev_number = min(somenumber)-1
would be enough and you can get rid of the import
– Patrick Artner
Feb 22 at 11:13
@PatrickArtner That's right. but here, we don't know the min range (some number) right. That's why we can use sys.maxint values.
– amulya349
Feb 25 at 9:10
add a comment |
What I understood from the question is every time, the value of somenumber
decreases in the next row, it should print the row. It's very easy to implement. Just store the previous value in another variable (prev_number
). For the first row, though, we need to initialize prev_number
to the minimum number possible. Following is the solution:
import sys
prev_number = -sys.maxint - 1 #initialize prev_number to lowest possible number in python
for a,b,c in zip(date,somenumber,price):
if b < prev_number:
print(a,b,c)
prev_number = d
Let me know if it works for you.
What I understood from the question is every time, the value of somenumber
decreases in the next row, it should print the row. It's very easy to implement. Just store the previous value in another variable (prev_number
). For the first row, though, we need to initialize prev_number
to the minimum number possible. Following is the solution:
import sys
prev_number = -sys.maxint - 1 #initialize prev_number to lowest possible number in python
for a,b,c in zip(date,somenumber,price):
if b < prev_number:
print(a,b,c)
prev_number = d
Let me know if it works for you.
answered Nov 15 '18 at 4:48
amulya349amulya349
698920
698920
there is no lowest possible integer number in python:n = -sys.maxint**10
is smaller then what you used. It is also not needed:prev_number = min(somenumber)-1
would be enough and you can get rid of the import
– Patrick Artner
Feb 22 at 11:13
@PatrickArtner That's right. but here, we don't know the min range (some number) right. That's why we can use sys.maxint values.
– amulya349
Feb 25 at 9:10
add a comment |
there is no lowest possible integer number in python:n = -sys.maxint**10
is smaller then what you used. It is also not needed:prev_number = min(somenumber)-1
would be enough and you can get rid of the import
– Patrick Artner
Feb 22 at 11:13
@PatrickArtner That's right. but here, we don't know the min range (some number) right. That's why we can use sys.maxint values.
– amulya349
Feb 25 at 9:10
there is no lowest possible integer number in python:
n = -sys.maxint**10
is smaller then what you used. It is also not needed: prev_number = min(somenumber)-1
would be enough and you can get rid of the import– Patrick Artner
Feb 22 at 11:13
there is no lowest possible integer number in python:
n = -sys.maxint**10
is smaller then what you used. It is also not needed: prev_number = min(somenumber)-1
would be enough and you can get rid of the import– Patrick Artner
Feb 22 at 11:13
@PatrickArtner That's right. but here, we don't know the min range (some number) right. That's why we can use sys.maxint values.
– amulya349
Feb 25 at 9:10
@PatrickArtner That's right. but here, we don't know the min range (some number) right. That's why we can use sys.maxint values.
– amulya349
Feb 25 at 9:10
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53312502%2fhow-to-check-if-a-number-in-a-list-is-greater-than-the-number-before-it-python%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
B8,0U GYeuG vPazrVor HFgJpz43foajIcsFiUEO,Usq8OjHlSdLIPN,pQH2ppepScP0DZO
323405 is less than 859235. Do you want to report it, too? If not, why?
– DYZ
Nov 15 '18 at 4:38