C# Getting Nearby Points on a Grid within radius
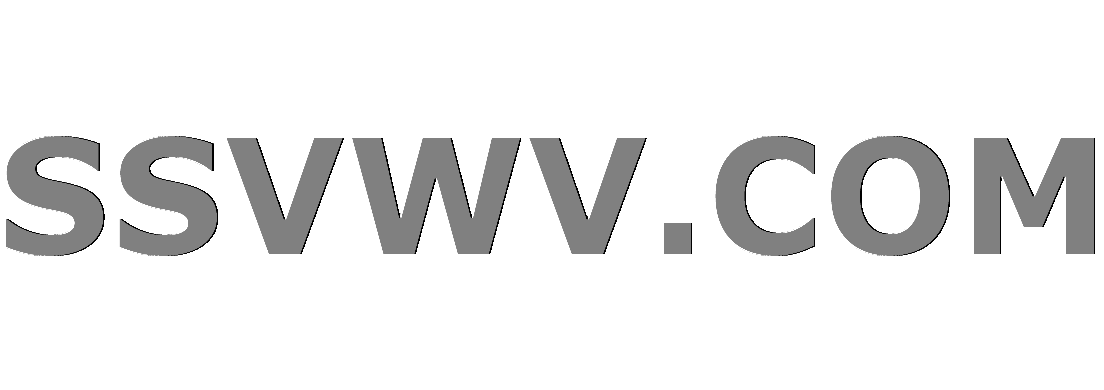
Multi tool use
up vote
0
down vote
favorite
I have a grid that has X and Y coordinates. On this grid I have a starting point and I want to get all nearby cells within a certain radius.
I have made made the following function that goes +1 one in each direction and returns the spots.
public List<Point> GetLocationsStraightLine(Point fromLocation, int Distance)
var nearbySpots = new List<Point>();
int StartDistance = 1;
while (StartDistance < Distance)
nearbySpots.Add(new Point(fromLocation.X, (short)(fromLocation.Y - StartDistance)));
nearbySpots.Add(new Point((short)(fromLocation.X + StartDistance), fromLocation.Y));
nearbySpots.Add(new Point(fromLocation.X, (short)(fromLocation.Y + StartDistance)));
nearbySpots.Add(new Point((short)(fromLocation.X - StartDistance), fromLocation.Y));
StartDistance++;
return nearbySpots;
This returns all points in a straight line from my starting point. However I want to to also grab the inbetween spots.
This is what I currently get (Sorry for the bad image)
image of points i return
However I want to be able to enter a distance of 2 and get the full square around the start location.
what i want full square with diagnonal
So I am asking is there any easy way I can get the diagonals and not just a straight line from my starting point?
c# grid points
add a comment |
up vote
0
down vote
favorite
I have a grid that has X and Y coordinates. On this grid I have a starting point and I want to get all nearby cells within a certain radius.
I have made made the following function that goes +1 one in each direction and returns the spots.
public List<Point> GetLocationsStraightLine(Point fromLocation, int Distance)
var nearbySpots = new List<Point>();
int StartDistance = 1;
while (StartDistance < Distance)
nearbySpots.Add(new Point(fromLocation.X, (short)(fromLocation.Y - StartDistance)));
nearbySpots.Add(new Point((short)(fromLocation.X + StartDistance), fromLocation.Y));
nearbySpots.Add(new Point(fromLocation.X, (short)(fromLocation.Y + StartDistance)));
nearbySpots.Add(new Point((short)(fromLocation.X - StartDistance), fromLocation.Y));
StartDistance++;
return nearbySpots;
This returns all points in a straight line from my starting point. However I want to to also grab the inbetween spots.
This is what I currently get (Sorry for the bad image)
image of points i return
However I want to be able to enter a distance of 2 and get the full square around the start location.
what i want full square with diagnonal
So I am asking is there any easy way I can get the diagonals and not just a straight line from my starting point?
c# grid points
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have a grid that has X and Y coordinates. On this grid I have a starting point and I want to get all nearby cells within a certain radius.
I have made made the following function that goes +1 one in each direction and returns the spots.
public List<Point> GetLocationsStraightLine(Point fromLocation, int Distance)
var nearbySpots = new List<Point>();
int StartDistance = 1;
while (StartDistance < Distance)
nearbySpots.Add(new Point(fromLocation.X, (short)(fromLocation.Y - StartDistance)));
nearbySpots.Add(new Point((short)(fromLocation.X + StartDistance), fromLocation.Y));
nearbySpots.Add(new Point(fromLocation.X, (short)(fromLocation.Y + StartDistance)));
nearbySpots.Add(new Point((short)(fromLocation.X - StartDistance), fromLocation.Y));
StartDistance++;
return nearbySpots;
This returns all points in a straight line from my starting point. However I want to to also grab the inbetween spots.
This is what I currently get (Sorry for the bad image)
image of points i return
However I want to be able to enter a distance of 2 and get the full square around the start location.
what i want full square with diagnonal
So I am asking is there any easy way I can get the diagonals and not just a straight line from my starting point?
c# grid points
I have a grid that has X and Y coordinates. On this grid I have a starting point and I want to get all nearby cells within a certain radius.
I have made made the following function that goes +1 one in each direction and returns the spots.
public List<Point> GetLocationsStraightLine(Point fromLocation, int Distance)
var nearbySpots = new List<Point>();
int StartDistance = 1;
while (StartDistance < Distance)
nearbySpots.Add(new Point(fromLocation.X, (short)(fromLocation.Y - StartDistance)));
nearbySpots.Add(new Point((short)(fromLocation.X + StartDistance), fromLocation.Y));
nearbySpots.Add(new Point(fromLocation.X, (short)(fromLocation.Y + StartDistance)));
nearbySpots.Add(new Point((short)(fromLocation.X - StartDistance), fromLocation.Y));
StartDistance++;
return nearbySpots;
This returns all points in a straight line from my starting point. However I want to to also grab the inbetween spots.
This is what I currently get (Sorry for the bad image)
image of points i return
However I want to be able to enter a distance of 2 and get the full square around the start location.
what i want full square with diagnonal
So I am asking is there any easy way I can get the diagonals and not just a straight line from my starting point?
c# grid points
c# grid points
asked Nov 10 at 3:53
Incognito
32
32
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
accepted
Since it's a rectangle, you can use a very simple approach. Assuming that Distance
is always positive:
public List<Point> GetLocationsStraightLine(Point fromLocation, int Distance)
var nearbySpots = new List<Point>();
for (int i = -Distance; i <= Distance; ++i)
for (int j = -Distance; j <= Distance; ++j)
nearbySpots.Add(new Point(fromLocation.X + j, fromLocation.Y + i));
return nearbySpots;
The idea is to start at the upper left point, and add each point in the rectangle row by row, ending at the bottom right point.
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
Since it's a rectangle, you can use a very simple approach. Assuming that Distance
is always positive:
public List<Point> GetLocationsStraightLine(Point fromLocation, int Distance)
var nearbySpots = new List<Point>();
for (int i = -Distance; i <= Distance; ++i)
for (int j = -Distance; j <= Distance; ++j)
nearbySpots.Add(new Point(fromLocation.X + j, fromLocation.Y + i));
return nearbySpots;
The idea is to start at the upper left point, and add each point in the rectangle row by row, ending at the bottom right point.
add a comment |
up vote
0
down vote
accepted
Since it's a rectangle, you can use a very simple approach. Assuming that Distance
is always positive:
public List<Point> GetLocationsStraightLine(Point fromLocation, int Distance)
var nearbySpots = new List<Point>();
for (int i = -Distance; i <= Distance; ++i)
for (int j = -Distance; j <= Distance; ++j)
nearbySpots.Add(new Point(fromLocation.X + j, fromLocation.Y + i));
return nearbySpots;
The idea is to start at the upper left point, and add each point in the rectangle row by row, ending at the bottom right point.
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
Since it's a rectangle, you can use a very simple approach. Assuming that Distance
is always positive:
public List<Point> GetLocationsStraightLine(Point fromLocation, int Distance)
var nearbySpots = new List<Point>();
for (int i = -Distance; i <= Distance; ++i)
for (int j = -Distance; j <= Distance; ++j)
nearbySpots.Add(new Point(fromLocation.X + j, fromLocation.Y + i));
return nearbySpots;
The idea is to start at the upper left point, and add each point in the rectangle row by row, ending at the bottom right point.
Since it's a rectangle, you can use a very simple approach. Assuming that Distance
is always positive:
public List<Point> GetLocationsStraightLine(Point fromLocation, int Distance)
var nearbySpots = new List<Point>();
for (int i = -Distance; i <= Distance; ++i)
for (int j = -Distance; j <= Distance; ++j)
nearbySpots.Add(new Point(fromLocation.X + j, fromLocation.Y + i));
return nearbySpots;
The idea is to start at the upper left point, and add each point in the rectangle row by row, ending at the bottom right point.
answered Nov 10 at 4:23


Squidy
533514
533514
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53235863%2fc-sharp-getting-nearby-points-on-a-grid-within-radius%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
I,Ie,QTHFxX2n PmBZLHg7LQoYU3PTUqlqoWVHMq