Convert string to all lower case without built-in functions
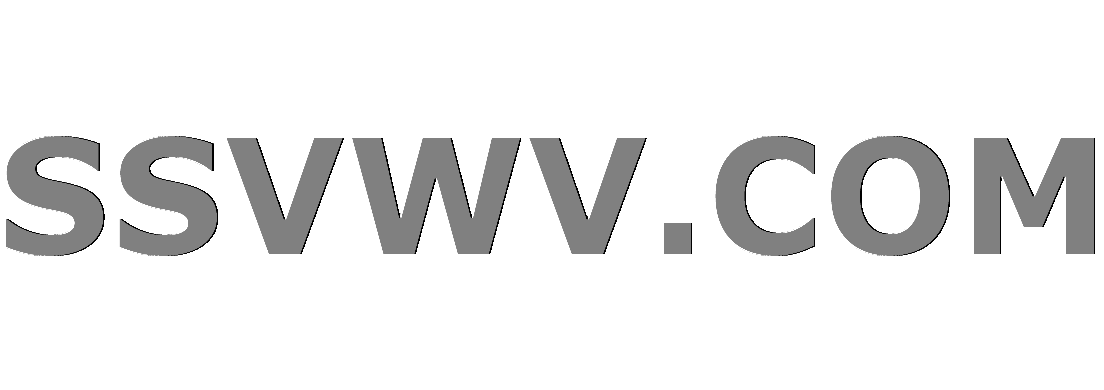
Multi tool use
up vote
1
down vote
favorite
#variable defination#
lower="abcdefghijklmnopqrstuvwxyz"
upper="ABCDEFGHIJKLMNOPQRSTUVWXYZ"
valid=True
x=0
g=0
string=input("enter a string:")
#data validation#
for char in string:
if char in "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ":
valid=True
else:
valid=False
#finding the character#
if valid:
for char in string:
g+=1
for ele in upper:
if char!=ele:
x+=1
print(lower[x]+string[g::])
**I can't get it to work, it keeps iterating through the entire string without the condition ever being met. **
python string
add a comment |
up vote
1
down vote
favorite
#variable defination#
lower="abcdefghijklmnopqrstuvwxyz"
upper="ABCDEFGHIJKLMNOPQRSTUVWXYZ"
valid=True
x=0
g=0
string=input("enter a string:")
#data validation#
for char in string:
if char in "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ":
valid=True
else:
valid=False
#finding the character#
if valid:
for char in string:
g+=1
for ele in upper:
if char!=ele:
x+=1
print(lower[x]+string[g::])
**I can't get it to work, it keeps iterating through the entire string without the condition ever being met. **
python string
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
#variable defination#
lower="abcdefghijklmnopqrstuvwxyz"
upper="ABCDEFGHIJKLMNOPQRSTUVWXYZ"
valid=True
x=0
g=0
string=input("enter a string:")
#data validation#
for char in string:
if char in "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ":
valid=True
else:
valid=False
#finding the character#
if valid:
for char in string:
g+=1
for ele in upper:
if char!=ele:
x+=1
print(lower[x]+string[g::])
**I can't get it to work, it keeps iterating through the entire string without the condition ever being met. **
python string
#variable defination#
lower="abcdefghijklmnopqrstuvwxyz"
upper="ABCDEFGHIJKLMNOPQRSTUVWXYZ"
valid=True
x=0
g=0
string=input("enter a string:")
#data validation#
for char in string:
if char in "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ":
valid=True
else:
valid=False
#finding the character#
if valid:
for char in string:
g+=1
for ele in upper:
if char!=ele:
x+=1
print(lower[x]+string[g::])
**I can't get it to work, it keeps iterating through the entire string without the condition ever being met. **
python string
python string
edited Nov 10 at 4:55


jpp
85.5k194898
85.5k194898
asked Nov 10 at 3:36


Sree The Tree
61
61
add a comment |
add a comment |
4 Answers
4
active
oldest
votes
up vote
1
down vote
I tried to minimize changes from your original code (But remember, it's obvious that other solutions are much better.)
#variable defination#
lower="abcdefghijklmnopqrstuvwxyz"
upper="ABCDEFGHIJKLMNOPQRSTUVWXYZ"
string=input("enter a string:")
#data validation#
valid = True
for char in string:
if char not in lower + upper:
valid=False
#finding the character#
if valid:
result = ""
for char in string:
if char in lower:
result += char
else:
# uppercase character
for i in range(len(upper)):
if char == upper[i]:
result += lower[i]
break
print(result)
add a comment |
up vote
0
down vote
Since you are allowed to use lowercase and uppercase character inputs, you can create a dictionary mapping between them and use str.join
with a list comprehension:
from string import ascii_lowercase, ascii_uppercase
d = dict(zip(ascii_uppercase, ascii_lowercase))
string = input("enter a string:")
res = ''.join([d.get(i, i) for i in string])
It's not clear whether this satisfies your "no in-built function" requirement.
add a comment |
up vote
0
down vote
You can create mapping(dictionary) between uppercase
and lowercase
alphabets such that upper_to_lower[uppercase]
should give you lowercase
. You may refer below implementation as reference though.
import string
def to_lower_case(word):
# construct auxilliary dictionary to avoid ord and chr built-in methods
upper_to_lower =
for index in range(len(string.ascii_uppercase)): # this is 26 we can use it as constant though
upper_to_lower[string.ascii_uppercase[index]] = string.ascii_lowercase[index]
result = ''
for alphabet in word:
if alphabet in string.ascii_uppercase:
result += upper_to_lower[alphabet]
else:
result += alphabet
return result
# sample input
In [5]: to_lower_case('ANJSNJN48982984aadkaka')
Out[5]: 'anjsnjn48982984aadkaka'
add a comment |
up vote
0
down vote
You can also write an index
method to get the index of an uppercase character in the upper
string:
lower = "abcdefghijklmnopqrstuvwxyz"
upper = "ABCDEFGHIJKLMNOPQRSTUVWXYZ"
def index(char):
i = 0
for c in upper:
if char == c: return i
i += 1
Then you can convert your string to lowercase like this:
#data validation#
# ...
#finding the character#
s = ''
if valid:
for char in string:
if char in lower: s += char
else: s += lower[index(char)]
print(s)
add a comment |
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
I tried to minimize changes from your original code (But remember, it's obvious that other solutions are much better.)
#variable defination#
lower="abcdefghijklmnopqrstuvwxyz"
upper="ABCDEFGHIJKLMNOPQRSTUVWXYZ"
string=input("enter a string:")
#data validation#
valid = True
for char in string:
if char not in lower + upper:
valid=False
#finding the character#
if valid:
result = ""
for char in string:
if char in lower:
result += char
else:
# uppercase character
for i in range(len(upper)):
if char == upper[i]:
result += lower[i]
break
print(result)
add a comment |
up vote
1
down vote
I tried to minimize changes from your original code (But remember, it's obvious that other solutions are much better.)
#variable defination#
lower="abcdefghijklmnopqrstuvwxyz"
upper="ABCDEFGHIJKLMNOPQRSTUVWXYZ"
string=input("enter a string:")
#data validation#
valid = True
for char in string:
if char not in lower + upper:
valid=False
#finding the character#
if valid:
result = ""
for char in string:
if char in lower:
result += char
else:
# uppercase character
for i in range(len(upper)):
if char == upper[i]:
result += lower[i]
break
print(result)
add a comment |
up vote
1
down vote
up vote
1
down vote
I tried to minimize changes from your original code (But remember, it's obvious that other solutions are much better.)
#variable defination#
lower="abcdefghijklmnopqrstuvwxyz"
upper="ABCDEFGHIJKLMNOPQRSTUVWXYZ"
string=input("enter a string:")
#data validation#
valid = True
for char in string:
if char not in lower + upper:
valid=False
#finding the character#
if valid:
result = ""
for char in string:
if char in lower:
result += char
else:
# uppercase character
for i in range(len(upper)):
if char == upper[i]:
result += lower[i]
break
print(result)
I tried to minimize changes from your original code (But remember, it's obvious that other solutions are much better.)
#variable defination#
lower="abcdefghijklmnopqrstuvwxyz"
upper="ABCDEFGHIJKLMNOPQRSTUVWXYZ"
string=input("enter a string:")
#data validation#
valid = True
for char in string:
if char not in lower + upper:
valid=False
#finding the character#
if valid:
result = ""
for char in string:
if char in lower:
result += char
else:
# uppercase character
for i in range(len(upper)):
if char == upper[i]:
result += lower[i]
break
print(result)
edited Nov 10 at 4:44
answered Nov 10 at 4:38


JH Jang
263
263
add a comment |
add a comment |
up vote
0
down vote
Since you are allowed to use lowercase and uppercase character inputs, you can create a dictionary mapping between them and use str.join
with a list comprehension:
from string import ascii_lowercase, ascii_uppercase
d = dict(zip(ascii_uppercase, ascii_lowercase))
string = input("enter a string:")
res = ''.join([d.get(i, i) for i in string])
It's not clear whether this satisfies your "no in-built function" requirement.
add a comment |
up vote
0
down vote
Since you are allowed to use lowercase and uppercase character inputs, you can create a dictionary mapping between them and use str.join
with a list comprehension:
from string import ascii_lowercase, ascii_uppercase
d = dict(zip(ascii_uppercase, ascii_lowercase))
string = input("enter a string:")
res = ''.join([d.get(i, i) for i in string])
It's not clear whether this satisfies your "no in-built function" requirement.
add a comment |
up vote
0
down vote
up vote
0
down vote
Since you are allowed to use lowercase and uppercase character inputs, you can create a dictionary mapping between them and use str.join
with a list comprehension:
from string import ascii_lowercase, ascii_uppercase
d = dict(zip(ascii_uppercase, ascii_lowercase))
string = input("enter a string:")
res = ''.join([d.get(i, i) for i in string])
It's not clear whether this satisfies your "no in-built function" requirement.
Since you are allowed to use lowercase and uppercase character inputs, you can create a dictionary mapping between them and use str.join
with a list comprehension:
from string import ascii_lowercase, ascii_uppercase
d = dict(zip(ascii_uppercase, ascii_lowercase))
string = input("enter a string:")
res = ''.join([d.get(i, i) for i in string])
It's not clear whether this satisfies your "no in-built function" requirement.
answered Nov 10 at 4:18


jpp
85.5k194898
85.5k194898
add a comment |
add a comment |
up vote
0
down vote
You can create mapping(dictionary) between uppercase
and lowercase
alphabets such that upper_to_lower[uppercase]
should give you lowercase
. You may refer below implementation as reference though.
import string
def to_lower_case(word):
# construct auxilliary dictionary to avoid ord and chr built-in methods
upper_to_lower =
for index in range(len(string.ascii_uppercase)): # this is 26 we can use it as constant though
upper_to_lower[string.ascii_uppercase[index]] = string.ascii_lowercase[index]
result = ''
for alphabet in word:
if alphabet in string.ascii_uppercase:
result += upper_to_lower[alphabet]
else:
result += alphabet
return result
# sample input
In [5]: to_lower_case('ANJSNJN48982984aadkaka')
Out[5]: 'anjsnjn48982984aadkaka'
add a comment |
up vote
0
down vote
You can create mapping(dictionary) between uppercase
and lowercase
alphabets such that upper_to_lower[uppercase]
should give you lowercase
. You may refer below implementation as reference though.
import string
def to_lower_case(word):
# construct auxilliary dictionary to avoid ord and chr built-in methods
upper_to_lower =
for index in range(len(string.ascii_uppercase)): # this is 26 we can use it as constant though
upper_to_lower[string.ascii_uppercase[index]] = string.ascii_lowercase[index]
result = ''
for alphabet in word:
if alphabet in string.ascii_uppercase:
result += upper_to_lower[alphabet]
else:
result += alphabet
return result
# sample input
In [5]: to_lower_case('ANJSNJN48982984aadkaka')
Out[5]: 'anjsnjn48982984aadkaka'
add a comment |
up vote
0
down vote
up vote
0
down vote
You can create mapping(dictionary) between uppercase
and lowercase
alphabets such that upper_to_lower[uppercase]
should give you lowercase
. You may refer below implementation as reference though.
import string
def to_lower_case(word):
# construct auxilliary dictionary to avoid ord and chr built-in methods
upper_to_lower =
for index in range(len(string.ascii_uppercase)): # this is 26 we can use it as constant though
upper_to_lower[string.ascii_uppercase[index]] = string.ascii_lowercase[index]
result = ''
for alphabet in word:
if alphabet in string.ascii_uppercase:
result += upper_to_lower[alphabet]
else:
result += alphabet
return result
# sample input
In [5]: to_lower_case('ANJSNJN48982984aadkaka')
Out[5]: 'anjsnjn48982984aadkaka'
You can create mapping(dictionary) between uppercase
and lowercase
alphabets such that upper_to_lower[uppercase]
should give you lowercase
. You may refer below implementation as reference though.
import string
def to_lower_case(word):
# construct auxilliary dictionary to avoid ord and chr built-in methods
upper_to_lower =
for index in range(len(string.ascii_uppercase)): # this is 26 we can use it as constant though
upper_to_lower[string.ascii_uppercase[index]] = string.ascii_lowercase[index]
result = ''
for alphabet in word:
if alphabet in string.ascii_uppercase:
result += upper_to_lower[alphabet]
else:
result += alphabet
return result
# sample input
In [5]: to_lower_case('ANJSNJN48982984aadkaka')
Out[5]: 'anjsnjn48982984aadkaka'
answered Nov 10 at 4:29
Yaman Jain
803812
803812
add a comment |
add a comment |
up vote
0
down vote
You can also write an index
method to get the index of an uppercase character in the upper
string:
lower = "abcdefghijklmnopqrstuvwxyz"
upper = "ABCDEFGHIJKLMNOPQRSTUVWXYZ"
def index(char):
i = 0
for c in upper:
if char == c: return i
i += 1
Then you can convert your string to lowercase like this:
#data validation#
# ...
#finding the character#
s = ''
if valid:
for char in string:
if char in lower: s += char
else: s += lower[index(char)]
print(s)
add a comment |
up vote
0
down vote
You can also write an index
method to get the index of an uppercase character in the upper
string:
lower = "abcdefghijklmnopqrstuvwxyz"
upper = "ABCDEFGHIJKLMNOPQRSTUVWXYZ"
def index(char):
i = 0
for c in upper:
if char == c: return i
i += 1
Then you can convert your string to lowercase like this:
#data validation#
# ...
#finding the character#
s = ''
if valid:
for char in string:
if char in lower: s += char
else: s += lower[index(char)]
print(s)
add a comment |
up vote
0
down vote
up vote
0
down vote
You can also write an index
method to get the index of an uppercase character in the upper
string:
lower = "abcdefghijklmnopqrstuvwxyz"
upper = "ABCDEFGHIJKLMNOPQRSTUVWXYZ"
def index(char):
i = 0
for c in upper:
if char == c: return i
i += 1
Then you can convert your string to lowercase like this:
#data validation#
# ...
#finding the character#
s = ''
if valid:
for char in string:
if char in lower: s += char
else: s += lower[index(char)]
print(s)
You can also write an index
method to get the index of an uppercase character in the upper
string:
lower = "abcdefghijklmnopqrstuvwxyz"
upper = "ABCDEFGHIJKLMNOPQRSTUVWXYZ"
def index(char):
i = 0
for c in upper:
if char == c: return i
i += 1
Then you can convert your string to lowercase like this:
#data validation#
# ...
#finding the character#
s = ''
if valid:
for char in string:
if char in lower: s += char
else: s += lower[index(char)]
print(s)
answered Nov 10 at 5:25
slider
7,3401129
7,3401129
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53235797%2fconvert-string-to-all-lower-case-without-built-in-functions%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
aG1nNRZ4yI5w,2OnoE8PDxAfx BY ASzg0eShiazvwBZ28Vem,jfwY1z4LR