Setting up the ATSAM3X8E on Arduino Due for SPI Slave Operation
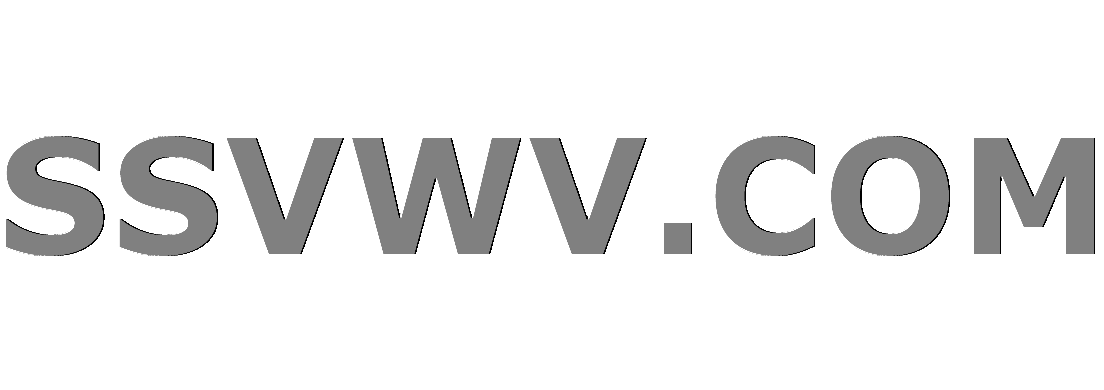
Multi tool use
I'm trying to build up a communication between Raspberry Pi 3B+ and Arduino Due via SPI. I have chosen the Raspberry Pi to act as master and the Arduino as slave. Therefore I have written some C Code for the Raspberry that configurates the interface and sends two byte of data via the MOSI line. I observed the signals with a logic analyzer, they are as expected, the Chip Select drops to zero at the start of the send process and rises afterwards again.
For programming the Arduino Due I am working with the Arduino IDE. Since the Arduino SPI.h does not support slave mode, I want to access the corresponding registers on the ATSAM3X8E directly. First, I define the Mode of the SPI Pins (Pin numbers from the 'unofficial' Due Pinout) as Input / Output. Afterwards I configure the registers of the ATSAM3X8E for SPI slave operation. The settings for CPOL and CPHA are the same as on the Raspberry. Since the devider for the serial clock baud rate is not allowed to be zero, I have chosen the biggest available devider, though I assume that this setting has no effect, because the master defines the transmission speed.
This is the code for configuration:
pinMode(74, OUTPUT); // PIN_SPI_MISO
pinMode(75, INPUT); // PIN_SPI_MOSI
pinMode(76, INPUT); // PIN_SPI_SCK
pinMode(10, INPUT); // Slave Select
REG_SPI0_CR = 0x00000001; // SPI Enable
REG_SPI0_MR = 0x00000000; // Slave-Mode, Fixed Peripheral Select,
// Chip Select directly connected
// Mode Fault detection enabled,
// Loopback disabled
REG_SPI0_WPMR = 0x00000000; // Write Protection disabled
REG_SPI0_CSR = 0x0000ff02; // Chip select Register
// CPOL = 0, CPHA = 1
// 8 Bit per transfer
// serial Clock Baud Rate of 84 MHz/255=330 kHz
After that, in a loop the bits of the Data Receive Register are stored in a buffer :
unsigned int buf = 0;
buf = REG_SPI0_RDR & 0x0000ffff; // store 16 Bits of Receive Data Registers in buffer
However, the buffer remains empty. If I read the bits of the status register, all bits are zero, even the bit for "NSS Rising Edge Detected" or "SPI Enbled" are zero.
Therefore I am assuming, the initialisation of the interface is not correct / complete. Did I miss anything ?
Any help is greatly appreciated.
raspberry-pi spi slave arduino-due
add a comment |
I'm trying to build up a communication between Raspberry Pi 3B+ and Arduino Due via SPI. I have chosen the Raspberry Pi to act as master and the Arduino as slave. Therefore I have written some C Code for the Raspberry that configurates the interface and sends two byte of data via the MOSI line. I observed the signals with a logic analyzer, they are as expected, the Chip Select drops to zero at the start of the send process and rises afterwards again.
For programming the Arduino Due I am working with the Arduino IDE. Since the Arduino SPI.h does not support slave mode, I want to access the corresponding registers on the ATSAM3X8E directly. First, I define the Mode of the SPI Pins (Pin numbers from the 'unofficial' Due Pinout) as Input / Output. Afterwards I configure the registers of the ATSAM3X8E for SPI slave operation. The settings for CPOL and CPHA are the same as on the Raspberry. Since the devider for the serial clock baud rate is not allowed to be zero, I have chosen the biggest available devider, though I assume that this setting has no effect, because the master defines the transmission speed.
This is the code for configuration:
pinMode(74, OUTPUT); // PIN_SPI_MISO
pinMode(75, INPUT); // PIN_SPI_MOSI
pinMode(76, INPUT); // PIN_SPI_SCK
pinMode(10, INPUT); // Slave Select
REG_SPI0_CR = 0x00000001; // SPI Enable
REG_SPI0_MR = 0x00000000; // Slave-Mode, Fixed Peripheral Select,
// Chip Select directly connected
// Mode Fault detection enabled,
// Loopback disabled
REG_SPI0_WPMR = 0x00000000; // Write Protection disabled
REG_SPI0_CSR = 0x0000ff02; // Chip select Register
// CPOL = 0, CPHA = 1
// 8 Bit per transfer
// serial Clock Baud Rate of 84 MHz/255=330 kHz
After that, in a loop the bits of the Data Receive Register are stored in a buffer :
unsigned int buf = 0;
buf = REG_SPI0_RDR & 0x0000ffff; // store 16 Bits of Receive Data Registers in buffer
However, the buffer remains empty. If I read the bits of the status register, all bits are zero, even the bit for "NSS Rising Edge Detected" or "SPI Enbled" are zero.
Therefore I am assuming, the initialisation of the interface is not correct / complete. Did I miss anything ?
Any help is greatly appreciated.
raspberry-pi spi slave arduino-due
add a comment |
I'm trying to build up a communication between Raspberry Pi 3B+ and Arduino Due via SPI. I have chosen the Raspberry Pi to act as master and the Arduino as slave. Therefore I have written some C Code for the Raspberry that configurates the interface and sends two byte of data via the MOSI line. I observed the signals with a logic analyzer, they are as expected, the Chip Select drops to zero at the start of the send process and rises afterwards again.
For programming the Arduino Due I am working with the Arduino IDE. Since the Arduino SPI.h does not support slave mode, I want to access the corresponding registers on the ATSAM3X8E directly. First, I define the Mode of the SPI Pins (Pin numbers from the 'unofficial' Due Pinout) as Input / Output. Afterwards I configure the registers of the ATSAM3X8E for SPI slave operation. The settings for CPOL and CPHA are the same as on the Raspberry. Since the devider for the serial clock baud rate is not allowed to be zero, I have chosen the biggest available devider, though I assume that this setting has no effect, because the master defines the transmission speed.
This is the code for configuration:
pinMode(74, OUTPUT); // PIN_SPI_MISO
pinMode(75, INPUT); // PIN_SPI_MOSI
pinMode(76, INPUT); // PIN_SPI_SCK
pinMode(10, INPUT); // Slave Select
REG_SPI0_CR = 0x00000001; // SPI Enable
REG_SPI0_MR = 0x00000000; // Slave-Mode, Fixed Peripheral Select,
// Chip Select directly connected
// Mode Fault detection enabled,
// Loopback disabled
REG_SPI0_WPMR = 0x00000000; // Write Protection disabled
REG_SPI0_CSR = 0x0000ff02; // Chip select Register
// CPOL = 0, CPHA = 1
// 8 Bit per transfer
// serial Clock Baud Rate of 84 MHz/255=330 kHz
After that, in a loop the bits of the Data Receive Register are stored in a buffer :
unsigned int buf = 0;
buf = REG_SPI0_RDR & 0x0000ffff; // store 16 Bits of Receive Data Registers in buffer
However, the buffer remains empty. If I read the bits of the status register, all bits are zero, even the bit for "NSS Rising Edge Detected" or "SPI Enbled" are zero.
Therefore I am assuming, the initialisation of the interface is not correct / complete. Did I miss anything ?
Any help is greatly appreciated.
raspberry-pi spi slave arduino-due
I'm trying to build up a communication between Raspberry Pi 3B+ and Arduino Due via SPI. I have chosen the Raspberry Pi to act as master and the Arduino as slave. Therefore I have written some C Code for the Raspberry that configurates the interface and sends two byte of data via the MOSI line. I observed the signals with a logic analyzer, they are as expected, the Chip Select drops to zero at the start of the send process and rises afterwards again.
For programming the Arduino Due I am working with the Arduino IDE. Since the Arduino SPI.h does not support slave mode, I want to access the corresponding registers on the ATSAM3X8E directly. First, I define the Mode of the SPI Pins (Pin numbers from the 'unofficial' Due Pinout) as Input / Output. Afterwards I configure the registers of the ATSAM3X8E for SPI slave operation. The settings for CPOL and CPHA are the same as on the Raspberry. Since the devider for the serial clock baud rate is not allowed to be zero, I have chosen the biggest available devider, though I assume that this setting has no effect, because the master defines the transmission speed.
This is the code for configuration:
pinMode(74, OUTPUT); // PIN_SPI_MISO
pinMode(75, INPUT); // PIN_SPI_MOSI
pinMode(76, INPUT); // PIN_SPI_SCK
pinMode(10, INPUT); // Slave Select
REG_SPI0_CR = 0x00000001; // SPI Enable
REG_SPI0_MR = 0x00000000; // Slave-Mode, Fixed Peripheral Select,
// Chip Select directly connected
// Mode Fault detection enabled,
// Loopback disabled
REG_SPI0_WPMR = 0x00000000; // Write Protection disabled
REG_SPI0_CSR = 0x0000ff02; // Chip select Register
// CPOL = 0, CPHA = 1
// 8 Bit per transfer
// serial Clock Baud Rate of 84 MHz/255=330 kHz
After that, in a loop the bits of the Data Receive Register are stored in a buffer :
unsigned int buf = 0;
buf = REG_SPI0_RDR & 0x0000ffff; // store 16 Bits of Receive Data Registers in buffer
However, the buffer remains empty. If I read the bits of the status register, all bits are zero, even the bit for "NSS Rising Edge Detected" or "SPI Enbled" are zero.
Therefore I am assuming, the initialisation of the interface is not correct / complete. Did I miss anything ?
Any help is greatly appreciated.
raspberry-pi spi slave arduino-due
raspberry-pi spi slave arduino-due
asked Nov 13 '18 at 21:24
ArduinoNewbieArduinoNewbie
62
62
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Using pinMode(74, OUTPUT);
and similar Arduino function calls you just change pin direction but not change pin usage as GPIO. For using these pins as SPI lines you should setup pin multiplexer.
See in ATSAM3X8E datasheet
- 9.3 Peripheral Signal Multiplexing on I/O Lines
- 31.4.1 Pin Multiplexing
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53289711%2fsetting-up-the-atsam3x8e-on-arduino-due-for-spi-slave-operation%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Using pinMode(74, OUTPUT);
and similar Arduino function calls you just change pin direction but not change pin usage as GPIO. For using these pins as SPI lines you should setup pin multiplexer.
See in ATSAM3X8E datasheet
- 9.3 Peripheral Signal Multiplexing on I/O Lines
- 31.4.1 Pin Multiplexing
add a comment |
Using pinMode(74, OUTPUT);
and similar Arduino function calls you just change pin direction but not change pin usage as GPIO. For using these pins as SPI lines you should setup pin multiplexer.
See in ATSAM3X8E datasheet
- 9.3 Peripheral Signal Multiplexing on I/O Lines
- 31.4.1 Pin Multiplexing
add a comment |
Using pinMode(74, OUTPUT);
and similar Arduino function calls you just change pin direction but not change pin usage as GPIO. For using these pins as SPI lines you should setup pin multiplexer.
See in ATSAM3X8E datasheet
- 9.3 Peripheral Signal Multiplexing on I/O Lines
- 31.4.1 Pin Multiplexing
Using pinMode(74, OUTPUT);
and similar Arduino function calls you just change pin direction but not change pin usage as GPIO. For using these pins as SPI lines you should setup pin multiplexer.
See in ATSAM3X8E datasheet
- 9.3 Peripheral Signal Multiplexing on I/O Lines
- 31.4.1 Pin Multiplexing
edited Nov 18 '18 at 10:09
answered Nov 17 '18 at 17:07
ReAlReAl
7581216
7581216
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53289711%2fsetting-up-the-atsam3x8e-on-arduino-due-for-spi-slave-operation%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
7 i7gK4XV,pNiGzSvhkYK6OZOD1k6Tz,Vz sHQC9F08ThJWh,aFIvCOfyC Tk9PntzAzQVuDOB0ZPwQ1UpFxrIOmS0P0KoU