Set Border/Stroke to Vector Drawable Programmatically
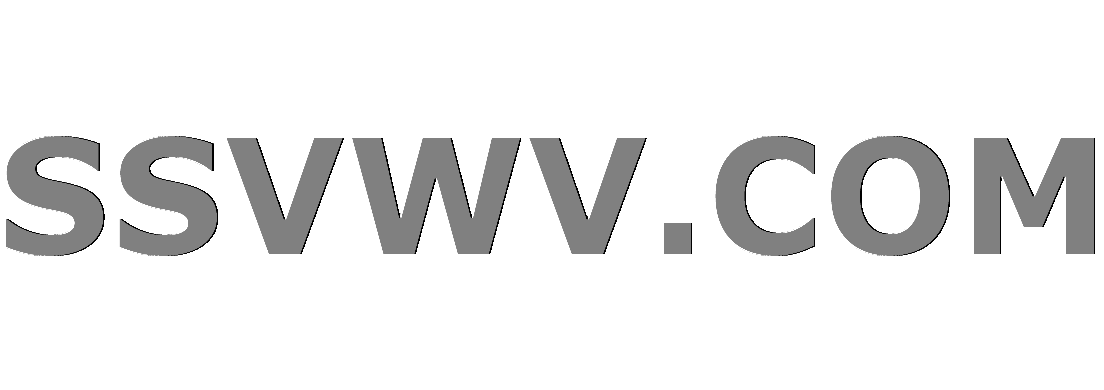
Multi tool use
I can change the color of vector drawables programmatically, but I want to apply the stroke to vector drawable. I need a method that will change the vector drawable stroke at runtime:
previously i used this method but failed in my case.
i converted the Vector drawable into bitmap and then apply border with this function but it fills all with black, the stroke is not applied.
private static Bitmap getBitmap(VectorDrawable vectorDrawable)
Bitmap bitmap = Bitmap.createBitmap(vectorDrawable.getIntrinsicWidth(),
vectorDrawable.getIntrinsicHeight(), Bitmap.Config.ARGB_8888);
Canvas canvas = new Canvas(bitmap);
vectorDrawable.setBounds(0, 0, canvas.getWidth(), canvas.getHeight());
vectorDrawable.draw(canvas);
return bitmap;
private static Bitmap getBitmap(Context context, int drawableId)
Drawable drawable = ContextCompat.getDrawable(context, drawableId);
if (drawable instanceof BitmapDrawable)
return ((BitmapDrawable) drawable).getBitmap();
else if (drawable instanceof VectorDrawable)
return getBitmap((VectorDrawable) drawable);
else
throw new IllegalArgumentException("unsupported drawable type");
private Bitmap addWhiteBorder(Bitmap bmp, int borderSize)
Bitmap bmpWithBorder = Bitmap.createBitmap(bmp.getWidth() + borderSize*2 , bmp.getHeight() + borderSize*2 , bmp.getConfig());
Canvas canvas = new Canvas(bmpWithBorder);
canvas.drawColor(Color.BLACK);
canvas.drawBitmap(bmp, borderSize, borderSize, null);
return bmpWithBorder;

add a comment |
I can change the color of vector drawables programmatically, but I want to apply the stroke to vector drawable. I need a method that will change the vector drawable stroke at runtime:
previously i used this method but failed in my case.
i converted the Vector drawable into bitmap and then apply border with this function but it fills all with black, the stroke is not applied.
private static Bitmap getBitmap(VectorDrawable vectorDrawable)
Bitmap bitmap = Bitmap.createBitmap(vectorDrawable.getIntrinsicWidth(),
vectorDrawable.getIntrinsicHeight(), Bitmap.Config.ARGB_8888);
Canvas canvas = new Canvas(bitmap);
vectorDrawable.setBounds(0, 0, canvas.getWidth(), canvas.getHeight());
vectorDrawable.draw(canvas);
return bitmap;
private static Bitmap getBitmap(Context context, int drawableId)
Drawable drawable = ContextCompat.getDrawable(context, drawableId);
if (drawable instanceof BitmapDrawable)
return ((BitmapDrawable) drawable).getBitmap();
else if (drawable instanceof VectorDrawable)
return getBitmap((VectorDrawable) drawable);
else
throw new IllegalArgumentException("unsupported drawable type");
private Bitmap addWhiteBorder(Bitmap bmp, int borderSize)
Bitmap bmpWithBorder = Bitmap.createBitmap(bmp.getWidth() + borderSize*2 , bmp.getHeight() + borderSize*2 , bmp.getConfig());
Canvas canvas = new Canvas(bmpWithBorder);
canvas.drawColor(Color.BLACK);
canvas.drawBitmap(bmp, borderSize, borderSize, null);
return bmpWithBorder;

add a comment |
I can change the color of vector drawables programmatically, but I want to apply the stroke to vector drawable. I need a method that will change the vector drawable stroke at runtime:
previously i used this method but failed in my case.
i converted the Vector drawable into bitmap and then apply border with this function but it fills all with black, the stroke is not applied.
private static Bitmap getBitmap(VectorDrawable vectorDrawable)
Bitmap bitmap = Bitmap.createBitmap(vectorDrawable.getIntrinsicWidth(),
vectorDrawable.getIntrinsicHeight(), Bitmap.Config.ARGB_8888);
Canvas canvas = new Canvas(bitmap);
vectorDrawable.setBounds(0, 0, canvas.getWidth(), canvas.getHeight());
vectorDrawable.draw(canvas);
return bitmap;
private static Bitmap getBitmap(Context context, int drawableId)
Drawable drawable = ContextCompat.getDrawable(context, drawableId);
if (drawable instanceof BitmapDrawable)
return ((BitmapDrawable) drawable).getBitmap();
else if (drawable instanceof VectorDrawable)
return getBitmap((VectorDrawable) drawable);
else
throw new IllegalArgumentException("unsupported drawable type");
private Bitmap addWhiteBorder(Bitmap bmp, int borderSize)
Bitmap bmpWithBorder = Bitmap.createBitmap(bmp.getWidth() + borderSize*2 , bmp.getHeight() + borderSize*2 , bmp.getConfig());
Canvas canvas = new Canvas(bmpWithBorder);
canvas.drawColor(Color.BLACK);
canvas.drawBitmap(bmp, borderSize, borderSize, null);
return bmpWithBorder;

I can change the color of vector drawables programmatically, but I want to apply the stroke to vector drawable. I need a method that will change the vector drawable stroke at runtime:
previously i used this method but failed in my case.
i converted the Vector drawable into bitmap and then apply border with this function but it fills all with black, the stroke is not applied.
private static Bitmap getBitmap(VectorDrawable vectorDrawable)
Bitmap bitmap = Bitmap.createBitmap(vectorDrawable.getIntrinsicWidth(),
vectorDrawable.getIntrinsicHeight(), Bitmap.Config.ARGB_8888);
Canvas canvas = new Canvas(bitmap);
vectorDrawable.setBounds(0, 0, canvas.getWidth(), canvas.getHeight());
vectorDrawable.draw(canvas);
return bitmap;
private static Bitmap getBitmap(Context context, int drawableId)
Drawable drawable = ContextCompat.getDrawable(context, drawableId);
if (drawable instanceof BitmapDrawable)
return ((BitmapDrawable) drawable).getBitmap();
else if (drawable instanceof VectorDrawable)
return getBitmap((VectorDrawable) drawable);
else
throw new IllegalArgumentException("unsupported drawable type");
private Bitmap addWhiteBorder(Bitmap bmp, int borderSize)
Bitmap bmpWithBorder = Bitmap.createBitmap(bmp.getWidth() + borderSize*2 , bmp.getHeight() + borderSize*2 , bmp.getConfig());
Canvas canvas = new Canvas(bmpWithBorder);
canvas.drawColor(Color.BLACK);
canvas.drawBitmap(bmp, borderSize, borderSize, null);
return bmpWithBorder;


edited Nov 14 '17 at 9:38
Vadim Kotov
4,82863549
4,82863549
asked Nov 18 '16 at 10:17
waqaswaqas
63
63
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
Look at this answer
https://stackoverflow.com/a/52960168/10592895
You can use vector drawable with different viewport width and height as background.
To do it programmatically, you can set background to transparent or a drawable with different dimension based on your needs.
Hope this helps.
add a comment |
Assume you have the VectorDrawable defined in vectordrawable.xml
<vector
android:width="100dp"
android:height="100dp"
android:viewportWidth="100"
android:viewportHeight="100">
<path
android:name="headset"
android:strokeColor="#FF000000"
android:strokeWidth="0"
...
android:pathData="..." />
</vector>
Then you can define an AnimatedVectorDrawable to change the strokeWidth
<?xml version="1.0" encoding="utf-8"?>
<animated-vector
xmlns:android="http://schemas.android.com/apk/res/android"
android:drawable="@drawable/vectordrawable">
<target
android:animation="@animator/change_stroke_width"
android:name="headset" />
</animated-vector>
Finally, define the animation that changes the strokeWidth in change_stroke_width.xml:
<set xmlns:android="http://schemas.android.com/apk/res/android" >
<objectAnimator
android:duration="100"
android:propertyName="strokeWidth"
android:valueFrom="0"
android:valueTo="10" />
</set>
i assume this will draw a rectangle border around the bitmap, whereas i want to add the stroke/border to the path of vector drawable (or the bitmap created from it) @Doris
– waqas
Nov 22 '16 at 7:09
i added the image in question please see @Doris
– waqas
Nov 22 '16 at 7:13
Yes, the answer above would produce a rectangular border. After seeing the image you added, I assume this VectorDrawable can be represented with one path, in which case you would need to define strokeWidth and strokeColor to achieve that black outline/border.
– Doris Liu
Nov 22 '16 at 7:25
Is the strokeWidth determined programmatically? @waqas
– Doris Liu
Nov 22 '16 at 7:27
no the stroke and width on the image is not added programatically, i added in the vector drawables xml , but i want same kind of result programatically ,could u please help. @Doris
– waqas
Nov 22 '16 at 7:44
|
show 3 more comments
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f40674459%2fset-border-stroke-to-vector-drawable-programmatically%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Look at this answer
https://stackoverflow.com/a/52960168/10592895
You can use vector drawable with different viewport width and height as background.
To do it programmatically, you can set background to transparent or a drawable with different dimension based on your needs.
Hope this helps.
add a comment |
Look at this answer
https://stackoverflow.com/a/52960168/10592895
You can use vector drawable with different viewport width and height as background.
To do it programmatically, you can set background to transparent or a drawable with different dimension based on your needs.
Hope this helps.
add a comment |
Look at this answer
https://stackoverflow.com/a/52960168/10592895
You can use vector drawable with different viewport width and height as background.
To do it programmatically, you can set background to transparent or a drawable with different dimension based on your needs.
Hope this helps.
Look at this answer
https://stackoverflow.com/a/52960168/10592895
You can use vector drawable with different viewport width and height as background.
To do it programmatically, you can set background to transparent or a drawable with different dimension based on your needs.
Hope this helps.
answered Nov 15 '18 at 5:15
user10592895
add a comment |
add a comment |
Assume you have the VectorDrawable defined in vectordrawable.xml
<vector
android:width="100dp"
android:height="100dp"
android:viewportWidth="100"
android:viewportHeight="100">
<path
android:name="headset"
android:strokeColor="#FF000000"
android:strokeWidth="0"
...
android:pathData="..." />
</vector>
Then you can define an AnimatedVectorDrawable to change the strokeWidth
<?xml version="1.0" encoding="utf-8"?>
<animated-vector
xmlns:android="http://schemas.android.com/apk/res/android"
android:drawable="@drawable/vectordrawable">
<target
android:animation="@animator/change_stroke_width"
android:name="headset" />
</animated-vector>
Finally, define the animation that changes the strokeWidth in change_stroke_width.xml:
<set xmlns:android="http://schemas.android.com/apk/res/android" >
<objectAnimator
android:duration="100"
android:propertyName="strokeWidth"
android:valueFrom="0"
android:valueTo="10" />
</set>
i assume this will draw a rectangle border around the bitmap, whereas i want to add the stroke/border to the path of vector drawable (or the bitmap created from it) @Doris
– waqas
Nov 22 '16 at 7:09
i added the image in question please see @Doris
– waqas
Nov 22 '16 at 7:13
Yes, the answer above would produce a rectangular border. After seeing the image you added, I assume this VectorDrawable can be represented with one path, in which case you would need to define strokeWidth and strokeColor to achieve that black outline/border.
– Doris Liu
Nov 22 '16 at 7:25
Is the strokeWidth determined programmatically? @waqas
– Doris Liu
Nov 22 '16 at 7:27
no the stroke and width on the image is not added programatically, i added in the vector drawables xml , but i want same kind of result programatically ,could u please help. @Doris
– waqas
Nov 22 '16 at 7:44
|
show 3 more comments
Assume you have the VectorDrawable defined in vectordrawable.xml
<vector
android:width="100dp"
android:height="100dp"
android:viewportWidth="100"
android:viewportHeight="100">
<path
android:name="headset"
android:strokeColor="#FF000000"
android:strokeWidth="0"
...
android:pathData="..." />
</vector>
Then you can define an AnimatedVectorDrawable to change the strokeWidth
<?xml version="1.0" encoding="utf-8"?>
<animated-vector
xmlns:android="http://schemas.android.com/apk/res/android"
android:drawable="@drawable/vectordrawable">
<target
android:animation="@animator/change_stroke_width"
android:name="headset" />
</animated-vector>
Finally, define the animation that changes the strokeWidth in change_stroke_width.xml:
<set xmlns:android="http://schemas.android.com/apk/res/android" >
<objectAnimator
android:duration="100"
android:propertyName="strokeWidth"
android:valueFrom="0"
android:valueTo="10" />
</set>
i assume this will draw a rectangle border around the bitmap, whereas i want to add the stroke/border to the path of vector drawable (or the bitmap created from it) @Doris
– waqas
Nov 22 '16 at 7:09
i added the image in question please see @Doris
– waqas
Nov 22 '16 at 7:13
Yes, the answer above would produce a rectangular border. After seeing the image you added, I assume this VectorDrawable can be represented with one path, in which case you would need to define strokeWidth and strokeColor to achieve that black outline/border.
– Doris Liu
Nov 22 '16 at 7:25
Is the strokeWidth determined programmatically? @waqas
– Doris Liu
Nov 22 '16 at 7:27
no the stroke and width on the image is not added programatically, i added in the vector drawables xml , but i want same kind of result programatically ,could u please help. @Doris
– waqas
Nov 22 '16 at 7:44
|
show 3 more comments
Assume you have the VectorDrawable defined in vectordrawable.xml
<vector
android:width="100dp"
android:height="100dp"
android:viewportWidth="100"
android:viewportHeight="100">
<path
android:name="headset"
android:strokeColor="#FF000000"
android:strokeWidth="0"
...
android:pathData="..." />
</vector>
Then you can define an AnimatedVectorDrawable to change the strokeWidth
<?xml version="1.0" encoding="utf-8"?>
<animated-vector
xmlns:android="http://schemas.android.com/apk/res/android"
android:drawable="@drawable/vectordrawable">
<target
android:animation="@animator/change_stroke_width"
android:name="headset" />
</animated-vector>
Finally, define the animation that changes the strokeWidth in change_stroke_width.xml:
<set xmlns:android="http://schemas.android.com/apk/res/android" >
<objectAnimator
android:duration="100"
android:propertyName="strokeWidth"
android:valueFrom="0"
android:valueTo="10" />
</set>
Assume you have the VectorDrawable defined in vectordrawable.xml
<vector
android:width="100dp"
android:height="100dp"
android:viewportWidth="100"
android:viewportHeight="100">
<path
android:name="headset"
android:strokeColor="#FF000000"
android:strokeWidth="0"
...
android:pathData="..." />
</vector>
Then you can define an AnimatedVectorDrawable to change the strokeWidth
<?xml version="1.0" encoding="utf-8"?>
<animated-vector
xmlns:android="http://schemas.android.com/apk/res/android"
android:drawable="@drawable/vectordrawable">
<target
android:animation="@animator/change_stroke_width"
android:name="headset" />
</animated-vector>
Finally, define the animation that changes the strokeWidth in change_stroke_width.xml:
<set xmlns:android="http://schemas.android.com/apk/res/android" >
<objectAnimator
android:duration="100"
android:propertyName="strokeWidth"
android:valueFrom="0"
android:valueTo="10" />
</set>
edited Nov 22 '16 at 8:29
answered Nov 22 '16 at 3:27


Doris LiuDoris Liu
965
965
i assume this will draw a rectangle border around the bitmap, whereas i want to add the stroke/border to the path of vector drawable (or the bitmap created from it) @Doris
– waqas
Nov 22 '16 at 7:09
i added the image in question please see @Doris
– waqas
Nov 22 '16 at 7:13
Yes, the answer above would produce a rectangular border. After seeing the image you added, I assume this VectorDrawable can be represented with one path, in which case you would need to define strokeWidth and strokeColor to achieve that black outline/border.
– Doris Liu
Nov 22 '16 at 7:25
Is the strokeWidth determined programmatically? @waqas
– Doris Liu
Nov 22 '16 at 7:27
no the stroke and width on the image is not added programatically, i added in the vector drawables xml , but i want same kind of result programatically ,could u please help. @Doris
– waqas
Nov 22 '16 at 7:44
|
show 3 more comments
i assume this will draw a rectangle border around the bitmap, whereas i want to add the stroke/border to the path of vector drawable (or the bitmap created from it) @Doris
– waqas
Nov 22 '16 at 7:09
i added the image in question please see @Doris
– waqas
Nov 22 '16 at 7:13
Yes, the answer above would produce a rectangular border. After seeing the image you added, I assume this VectorDrawable can be represented with one path, in which case you would need to define strokeWidth and strokeColor to achieve that black outline/border.
– Doris Liu
Nov 22 '16 at 7:25
Is the strokeWidth determined programmatically? @waqas
– Doris Liu
Nov 22 '16 at 7:27
no the stroke and width on the image is not added programatically, i added in the vector drawables xml , but i want same kind of result programatically ,could u please help. @Doris
– waqas
Nov 22 '16 at 7:44
i assume this will draw a rectangle border around the bitmap, whereas i want to add the stroke/border to the path of vector drawable (or the bitmap created from it) @Doris
– waqas
Nov 22 '16 at 7:09
i assume this will draw a rectangle border around the bitmap, whereas i want to add the stroke/border to the path of vector drawable (or the bitmap created from it) @Doris
– waqas
Nov 22 '16 at 7:09
i added the image in question please see @Doris
– waqas
Nov 22 '16 at 7:13
i added the image in question please see @Doris
– waqas
Nov 22 '16 at 7:13
Yes, the answer above would produce a rectangular border. After seeing the image you added, I assume this VectorDrawable can be represented with one path, in which case you would need to define strokeWidth and strokeColor to achieve that black outline/border.
– Doris Liu
Nov 22 '16 at 7:25
Yes, the answer above would produce a rectangular border. After seeing the image you added, I assume this VectorDrawable can be represented with one path, in which case you would need to define strokeWidth and strokeColor to achieve that black outline/border.
– Doris Liu
Nov 22 '16 at 7:25
Is the strokeWidth determined programmatically? @waqas
– Doris Liu
Nov 22 '16 at 7:27
Is the strokeWidth determined programmatically? @waqas
– Doris Liu
Nov 22 '16 at 7:27
no the stroke and width on the image is not added programatically, i added in the vector drawables xml , but i want same kind of result programatically ,could u please help. @Doris
– waqas
Nov 22 '16 at 7:44
no the stroke and width on the image is not added programatically, i added in the vector drawables xml , but i want same kind of result programatically ,could u please help. @Doris
– waqas
Nov 22 '16 at 7:44
|
show 3 more comments
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f40674459%2fset-border-stroke-to-vector-drawable-programmatically%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
nPlfSl2dlr38hj wadj 6IX,Y0sb