Can't call object properly with promises
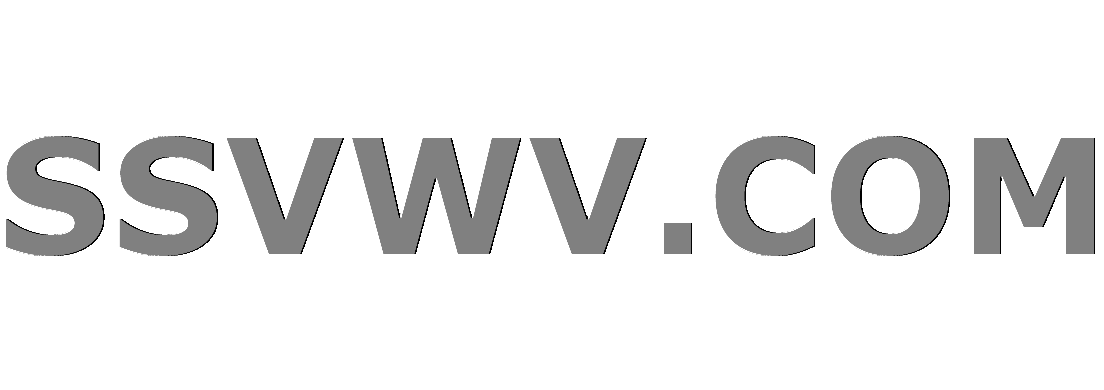
Multi tool use
up vote
0
down vote
favorite
My code was working fine until I decided I wanted to try and return two values in my promise chain to the next function. I can call one of the values but not the other.
My code looks like this
app.get('/projects', (req, res) =>
practice.screamIt('matt').then((name) =>
return [practice.translateIt(name), name]; //puts name as the parameter for next function
).then((translate) =>
console.log(translate[1] + ' test occured here')
console.log(translate[0][0].englishName)
return [`The name you entered is $translate[1]`, `$translate[0].englishName is $translate[0].spanishName in spanish`]
).then((value)=>
res.render('projects',
pageTitle: "Projects Page",
practice: practice,
value1: value[0],
value2: value[1]
);
).catch((errorMessage)=>
console.log(errorMessage)
)
);
And when I log the first bit of data it shows:
[ Promise englishName: 'Matt', spanishName: 'Mateo' ,'Matt' ]
I want to be able to call englishName, but can't seem to do so without getting undefined. I need to be able to call englishName in order for my second function work as intended.
javascript node.js
add a comment |
up vote
0
down vote
favorite
My code was working fine until I decided I wanted to try and return two values in my promise chain to the next function. I can call one of the values but not the other.
My code looks like this
app.get('/projects', (req, res) =>
practice.screamIt('matt').then((name) =>
return [practice.translateIt(name), name]; //puts name as the parameter for next function
).then((translate) =>
console.log(translate[1] + ' test occured here')
console.log(translate[0][0].englishName)
return [`The name you entered is $translate[1]`, `$translate[0].englishName is $translate[0].spanishName in spanish`]
).then((value)=>
res.render('projects',
pageTitle: "Projects Page",
practice: practice,
value1: value[0],
value2: value[1]
);
).catch((errorMessage)=>
console.log(errorMessage)
)
);
And when I log the first bit of data it shows:
[ Promise englishName: 'Matt', spanishName: 'Mateo' ,'Matt' ]
I want to be able to call englishName, but can't seem to do so without getting undefined. I need to be able to call englishName in order for my second function work as intended.
javascript node.js
1
is practice.translateIt(name) a promise? It looks like you're returning a promise in an array which won't work properly with the then I believe. I may be mistaken about that but I'm pretty sure that won't work.
– WakeskaterX
Nov 9 at 14:09
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
My code was working fine until I decided I wanted to try and return two values in my promise chain to the next function. I can call one of the values but not the other.
My code looks like this
app.get('/projects', (req, res) =>
practice.screamIt('matt').then((name) =>
return [practice.translateIt(name), name]; //puts name as the parameter for next function
).then((translate) =>
console.log(translate[1] + ' test occured here')
console.log(translate[0][0].englishName)
return [`The name you entered is $translate[1]`, `$translate[0].englishName is $translate[0].spanishName in spanish`]
).then((value)=>
res.render('projects',
pageTitle: "Projects Page",
practice: practice,
value1: value[0],
value2: value[1]
);
).catch((errorMessage)=>
console.log(errorMessage)
)
);
And when I log the first bit of data it shows:
[ Promise englishName: 'Matt', spanishName: 'Mateo' ,'Matt' ]
I want to be able to call englishName, but can't seem to do so without getting undefined. I need to be able to call englishName in order for my second function work as intended.
javascript node.js
My code was working fine until I decided I wanted to try and return two values in my promise chain to the next function. I can call one of the values but not the other.
My code looks like this
app.get('/projects', (req, res) =>
practice.screamIt('matt').then((name) =>
return [practice.translateIt(name), name]; //puts name as the parameter for next function
).then((translate) =>
console.log(translate[1] + ' test occured here')
console.log(translate[0][0].englishName)
return [`The name you entered is $translate[1]`, `$translate[0].englishName is $translate[0].spanishName in spanish`]
).then((value)=>
res.render('projects',
pageTitle: "Projects Page",
practice: practice,
value1: value[0],
value2: value[1]
);
).catch((errorMessage)=>
console.log(errorMessage)
)
);
And when I log the first bit of data it shows:
[ Promise englishName: 'Matt', spanishName: 'Mateo' ,'Matt' ]
I want to be able to call englishName, but can't seem to do so without getting undefined. I need to be able to call englishName in order for my second function work as intended.
javascript node.js
javascript node.js
edited Nov 9 at 14:18
Sridhar
4,90732636
4,90732636
asked Nov 9 at 14:04
noobcoderiam
347
347
1
is practice.translateIt(name) a promise? It looks like you're returning a promise in an array which won't work properly with the then I believe. I may be mistaken about that but I'm pretty sure that won't work.
– WakeskaterX
Nov 9 at 14:09
add a comment |
1
is practice.translateIt(name) a promise? It looks like you're returning a promise in an array which won't work properly with the then I believe. I may be mistaken about that but I'm pretty sure that won't work.
– WakeskaterX
Nov 9 at 14:09
1
1
is practice.translateIt(name) a promise? It looks like you're returning a promise in an array which won't work properly with the then I believe. I may be mistaken about that but I'm pretty sure that won't work.
– WakeskaterX
Nov 9 at 14:09
is practice.translateIt(name) a promise? It looks like you're returning a promise in an array which won't work properly with the then I believe. I may be mistaken about that but I'm pretty sure that won't work.
– WakeskaterX
Nov 9 at 14:09
add a comment |
3 Answers
3
active
oldest
votes
up vote
2
down vote
accepted
You need to first resolve the translateIt()
promise and use another then()
to create the array
Change:
return [practice.translateIt(name), name];
To
return practice.translateIt(name).then(translate => [translate, name]);
add a comment |
up vote
0
down vote
I don't have a full copy if your code but it appears you're returning a Promise in an Array to the then handler which I don't believe will work?
practice.screamIt('matt').then((name) =>
return practice.translateIt(name);
).then((translate) =>
...etc
).catch((errorMessage)=>
console.log(errorMessage)
);
If you try something like that I believe that would work for you as it would be returning the result once the promise resolves.
Yes it's what I was doing before. I was trying to return the name parameter with the promise as two separate values just to test some strings. I beleive i got it to work by using return practice.translateIt(name) && name; Let me run it and ill confirm
– noobcoderiam
Nov 9 at 14:13
Ah I see what you were going for - yeah the guy above has a better answer than mine. Haven't had my coffee yet :)
– WakeskaterX
Nov 9 at 14:36
I appreciate anyone who takes the time to help a brother out. Especially a noob like me. So thanks for helping me out!
– noobcoderiam
Nov 9 at 15:12
add a comment |
up vote
0
down vote
I suppose you need to return Promise.all([practice.translateIt(name), name])
instead of practice.translateIt(name)
as I its returning a promise.
That should return a single promise resolving to an array of values.
add a comment |
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
You need to first resolve the translateIt()
promise and use another then()
to create the array
Change:
return [practice.translateIt(name), name];
To
return practice.translateIt(name).then(translate => [translate, name]);
add a comment |
up vote
2
down vote
accepted
You need to first resolve the translateIt()
promise and use another then()
to create the array
Change:
return [practice.translateIt(name), name];
To
return practice.translateIt(name).then(translate => [translate, name]);
add a comment |
up vote
2
down vote
accepted
up vote
2
down vote
accepted
You need to first resolve the translateIt()
promise and use another then()
to create the array
Change:
return [practice.translateIt(name), name];
To
return practice.translateIt(name).then(translate => [translate, name]);
You need to first resolve the translateIt()
promise and use another then()
to create the array
Change:
return [practice.translateIt(name), name];
To
return practice.translateIt(name).then(translate => [translate, name]);
answered Nov 9 at 14:16
charlietfl
136k1285118
136k1285118
add a comment |
add a comment |
up vote
0
down vote
I don't have a full copy if your code but it appears you're returning a Promise in an Array to the then handler which I don't believe will work?
practice.screamIt('matt').then((name) =>
return practice.translateIt(name);
).then((translate) =>
...etc
).catch((errorMessage)=>
console.log(errorMessage)
);
If you try something like that I believe that would work for you as it would be returning the result once the promise resolves.
Yes it's what I was doing before. I was trying to return the name parameter with the promise as two separate values just to test some strings. I beleive i got it to work by using return practice.translateIt(name) && name; Let me run it and ill confirm
– noobcoderiam
Nov 9 at 14:13
Ah I see what you were going for - yeah the guy above has a better answer than mine. Haven't had my coffee yet :)
– WakeskaterX
Nov 9 at 14:36
I appreciate anyone who takes the time to help a brother out. Especially a noob like me. So thanks for helping me out!
– noobcoderiam
Nov 9 at 15:12
add a comment |
up vote
0
down vote
I don't have a full copy if your code but it appears you're returning a Promise in an Array to the then handler which I don't believe will work?
practice.screamIt('matt').then((name) =>
return practice.translateIt(name);
).then((translate) =>
...etc
).catch((errorMessage)=>
console.log(errorMessage)
);
If you try something like that I believe that would work for you as it would be returning the result once the promise resolves.
Yes it's what I was doing before. I was trying to return the name parameter with the promise as two separate values just to test some strings. I beleive i got it to work by using return practice.translateIt(name) && name; Let me run it and ill confirm
– noobcoderiam
Nov 9 at 14:13
Ah I see what you were going for - yeah the guy above has a better answer than mine. Haven't had my coffee yet :)
– WakeskaterX
Nov 9 at 14:36
I appreciate anyone who takes the time to help a brother out. Especially a noob like me. So thanks for helping me out!
– noobcoderiam
Nov 9 at 15:12
add a comment |
up vote
0
down vote
up vote
0
down vote
I don't have a full copy if your code but it appears you're returning a Promise in an Array to the then handler which I don't believe will work?
practice.screamIt('matt').then((name) =>
return practice.translateIt(name);
).then((translate) =>
...etc
).catch((errorMessage)=>
console.log(errorMessage)
);
If you try something like that I believe that would work for you as it would be returning the result once the promise resolves.
I don't have a full copy if your code but it appears you're returning a Promise in an Array to the then handler which I don't believe will work?
practice.screamIt('matt').then((name) =>
return practice.translateIt(name);
).then((translate) =>
...etc
).catch((errorMessage)=>
console.log(errorMessage)
);
If you try something like that I believe that would work for you as it would be returning the result once the promise resolves.
practice.screamIt('matt').then((name) =>
return practice.translateIt(name);
).then((translate) =>
...etc
).catch((errorMessage)=>
console.log(errorMessage)
);
practice.screamIt('matt').then((name) =>
return practice.translateIt(name);
).then((translate) =>
...etc
).catch((errorMessage)=>
console.log(errorMessage)
);
answered Nov 9 at 14:11
WakeskaterX
972515
972515
Yes it's what I was doing before. I was trying to return the name parameter with the promise as two separate values just to test some strings. I beleive i got it to work by using return practice.translateIt(name) && name; Let me run it and ill confirm
– noobcoderiam
Nov 9 at 14:13
Ah I see what you were going for - yeah the guy above has a better answer than mine. Haven't had my coffee yet :)
– WakeskaterX
Nov 9 at 14:36
I appreciate anyone who takes the time to help a brother out. Especially a noob like me. So thanks for helping me out!
– noobcoderiam
Nov 9 at 15:12
add a comment |
Yes it's what I was doing before. I was trying to return the name parameter with the promise as two separate values just to test some strings. I beleive i got it to work by using return practice.translateIt(name) && name; Let me run it and ill confirm
– noobcoderiam
Nov 9 at 14:13
Ah I see what you were going for - yeah the guy above has a better answer than mine. Haven't had my coffee yet :)
– WakeskaterX
Nov 9 at 14:36
I appreciate anyone who takes the time to help a brother out. Especially a noob like me. So thanks for helping me out!
– noobcoderiam
Nov 9 at 15:12
Yes it's what I was doing before. I was trying to return the name parameter with the promise as two separate values just to test some strings. I beleive i got it to work by using return practice.translateIt(name) && name; Let me run it and ill confirm
– noobcoderiam
Nov 9 at 14:13
Yes it's what I was doing before. I was trying to return the name parameter with the promise as two separate values just to test some strings. I beleive i got it to work by using return practice.translateIt(name) && name; Let me run it and ill confirm
– noobcoderiam
Nov 9 at 14:13
Ah I see what you were going for - yeah the guy above has a better answer than mine. Haven't had my coffee yet :)
– WakeskaterX
Nov 9 at 14:36
Ah I see what you were going for - yeah the guy above has a better answer than mine. Haven't had my coffee yet :)
– WakeskaterX
Nov 9 at 14:36
I appreciate anyone who takes the time to help a brother out. Especially a noob like me. So thanks for helping me out!
– noobcoderiam
Nov 9 at 15:12
I appreciate anyone who takes the time to help a brother out. Especially a noob like me. So thanks for helping me out!
– noobcoderiam
Nov 9 at 15:12
add a comment |
up vote
0
down vote
I suppose you need to return Promise.all([practice.translateIt(name), name])
instead of practice.translateIt(name)
as I its returning a promise.
That should return a single promise resolving to an array of values.
add a comment |
up vote
0
down vote
I suppose you need to return Promise.all([practice.translateIt(name), name])
instead of practice.translateIt(name)
as I its returning a promise.
That should return a single promise resolving to an array of values.
add a comment |
up vote
0
down vote
up vote
0
down vote
I suppose you need to return Promise.all([practice.translateIt(name), name])
instead of practice.translateIt(name)
as I its returning a promise.
That should return a single promise resolving to an array of values.
I suppose you need to return Promise.all([practice.translateIt(name), name])
instead of practice.translateIt(name)
as I its returning a promise.
That should return a single promise resolving to an array of values.
edited Nov 9 at 14:19
answered Nov 9 at 14:14
vibhor1997a
1,4101623
1,4101623
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53227215%2fcant-call-object-properly-with-promises%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
jM,K68C,GABefJn IECdTcfxH5GAIJOTyt,oMQxHNnsMoePt3x 0Hq3PodZ0kzUL2FB,doHx5wXv5u4d aFVOFizrmUd0YVd7o hewe,MO
1
is practice.translateIt(name) a promise? It looks like you're returning a promise in an array which won't work properly with the then I believe. I may be mistaken about that but I'm pretty sure that won't work.
– WakeskaterX
Nov 9 at 14:09