Pythonic way of executing a loop on a dictionary of lists
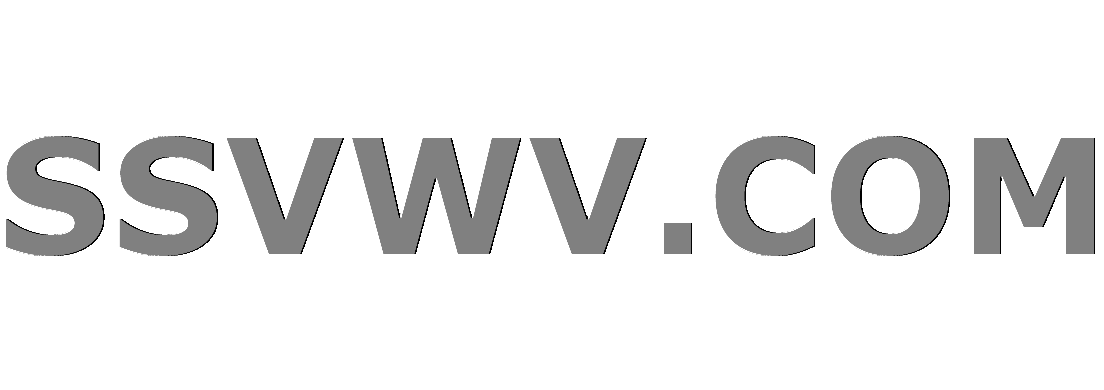
Multi tool use
up vote
4
down vote
favorite
This code works but I was wondering if there is a more pythonic way for writing it.
word_frequency
is a dictionary of lists, e.g.:
word_frequency = 'dogs': [1234, 4321], 'are': [9999, 0000], 'fun': [4389, 3234]
vocab_frequency = [0, 0] # stores the total times all the words used in each class
for word in word_frequency: # that is not the most elegant solution, but it works!
vocab_frequency[0] += word_frequency[word][0] #negative class
vocab_frequency[1] += word_frequency[word][1] #positive class
Is there a more elegant way of writing this loop?
python loops
add a comment |
up vote
4
down vote
favorite
This code works but I was wondering if there is a more pythonic way for writing it.
word_frequency
is a dictionary of lists, e.g.:
word_frequency = 'dogs': [1234, 4321], 'are': [9999, 0000], 'fun': [4389, 3234]
vocab_frequency = [0, 0] # stores the total times all the words used in each class
for word in word_frequency: # that is not the most elegant solution, but it works!
vocab_frequency[0] += word_frequency[word][0] #negative class
vocab_frequency[1] += word_frequency[word][1] #positive class
Is there a more elegant way of writing this loop?
python loops
2
Since you're not really usingword
except to look back intoword_frequency
you can iterate overword_frequency.values()
.
– pdexter
Nov 9 at 13:41
Lot's of simple and elegant answers here. So... I guess we might say "pythonic" means that the same thing can be done in a myriad of neat ways?
– Karl
Nov 9 at 21:52
@Karl python allows us to write very neat and concise code. However if, like me, you learned to program in C, many times you will catch yourself creating resource consuming loops and functions that could be handled in a much more elegant and often more efficient way. blog.startifact.com/posts/older/what-is-pythonic.html
– Victor Zuanazzi
Nov 10 at 14:20
Yee, my post was nothing more than a recognition of that. I was trying to point out if you ask a community of python programmers to come up the "the most pythonic way" (a question which I see all the time), what is revealed as pythonic is in fact that python allows for such a compact idiom in multiple ways
– Karl
Nov 10 at 17:33
add a comment |
up vote
4
down vote
favorite
up vote
4
down vote
favorite
This code works but I was wondering if there is a more pythonic way for writing it.
word_frequency
is a dictionary of lists, e.g.:
word_frequency = 'dogs': [1234, 4321], 'are': [9999, 0000], 'fun': [4389, 3234]
vocab_frequency = [0, 0] # stores the total times all the words used in each class
for word in word_frequency: # that is not the most elegant solution, but it works!
vocab_frequency[0] += word_frequency[word][0] #negative class
vocab_frequency[1] += word_frequency[word][1] #positive class
Is there a more elegant way of writing this loop?
python loops
This code works but I was wondering if there is a more pythonic way for writing it.
word_frequency
is a dictionary of lists, e.g.:
word_frequency = 'dogs': [1234, 4321], 'are': [9999, 0000], 'fun': [4389, 3234]
vocab_frequency = [0, 0] # stores the total times all the words used in each class
for word in word_frequency: # that is not the most elegant solution, but it works!
vocab_frequency[0] += word_frequency[word][0] #negative class
vocab_frequency[1] += word_frequency[word][1] #positive class
Is there a more elegant way of writing this loop?
python loops
python loops
edited Nov 9 at 13:40


Vlad
15.2k43162
15.2k43162
asked Nov 9 at 13:35
Victor Zuanazzi
6015
6015
2
Since you're not really usingword
except to look back intoword_frequency
you can iterate overword_frequency.values()
.
– pdexter
Nov 9 at 13:41
Lot's of simple and elegant answers here. So... I guess we might say "pythonic" means that the same thing can be done in a myriad of neat ways?
– Karl
Nov 9 at 21:52
@Karl python allows us to write very neat and concise code. However if, like me, you learned to program in C, many times you will catch yourself creating resource consuming loops and functions that could be handled in a much more elegant and often more efficient way. blog.startifact.com/posts/older/what-is-pythonic.html
– Victor Zuanazzi
Nov 10 at 14:20
Yee, my post was nothing more than a recognition of that. I was trying to point out if you ask a community of python programmers to come up the "the most pythonic way" (a question which I see all the time), what is revealed as pythonic is in fact that python allows for such a compact idiom in multiple ways
– Karl
Nov 10 at 17:33
add a comment |
2
Since you're not really usingword
except to look back intoword_frequency
you can iterate overword_frequency.values()
.
– pdexter
Nov 9 at 13:41
Lot's of simple and elegant answers here. So... I guess we might say "pythonic" means that the same thing can be done in a myriad of neat ways?
– Karl
Nov 9 at 21:52
@Karl python allows us to write very neat and concise code. However if, like me, you learned to program in C, many times you will catch yourself creating resource consuming loops and functions that could be handled in a much more elegant and often more efficient way. blog.startifact.com/posts/older/what-is-pythonic.html
– Victor Zuanazzi
Nov 10 at 14:20
Yee, my post was nothing more than a recognition of that. I was trying to point out if you ask a community of python programmers to come up the "the most pythonic way" (a question which I see all the time), what is revealed as pythonic is in fact that python allows for such a compact idiom in multiple ways
– Karl
Nov 10 at 17:33
2
2
Since you're not really using
word
except to look back into word_frequency
you can iterate over word_frequency.values()
.– pdexter
Nov 9 at 13:41
Since you're not really using
word
except to look back into word_frequency
you can iterate over word_frequency.values()
.– pdexter
Nov 9 at 13:41
Lot's of simple and elegant answers here. So... I guess we might say "pythonic" means that the same thing can be done in a myriad of neat ways?
– Karl
Nov 9 at 21:52
Lot's of simple and elegant answers here. So... I guess we might say "pythonic" means that the same thing can be done in a myriad of neat ways?
– Karl
Nov 9 at 21:52
@Karl python allows us to write very neat and concise code. However if, like me, you learned to program in C, many times you will catch yourself creating resource consuming loops and functions that could be handled in a much more elegant and often more efficient way. blog.startifact.com/posts/older/what-is-pythonic.html
– Victor Zuanazzi
Nov 10 at 14:20
@Karl python allows us to write very neat and concise code. However if, like me, you learned to program in C, many times you will catch yourself creating resource consuming loops and functions that could be handled in a much more elegant and often more efficient way. blog.startifact.com/posts/older/what-is-pythonic.html
– Victor Zuanazzi
Nov 10 at 14:20
Yee, my post was nothing more than a recognition of that. I was trying to point out if you ask a community of python programmers to come up the "the most pythonic way" (a question which I see all the time), what is revealed as pythonic is in fact that python allows for such a compact idiom in multiple ways
– Karl
Nov 10 at 17:33
Yee, my post was nothing more than a recognition of that. I was trying to point out if you ask a community of python programmers to come up the "the most pythonic way" (a question which I see all the time), what is revealed as pythonic is in fact that python allows for such a compact idiom in multiple ways
– Karl
Nov 10 at 17:33
add a comment |
9 Answers
9
active
oldest
votes
up vote
4
down vote
accepted
You could use numpy for that:
import numpy as np
word_frequency = 'dogs': [1234, 4321], 'are': [9999, 0000], 'fun': [4389, 3234]
vocab_frequency = np.sum(list(word_frequency.values()), axis=0)
1
Using numpy is like total over kill :P
– SirtusKottus
Nov 9 at 13:43
Why numpy...its -like Sirtuskottus said- overkill
– NaruS
Nov 9 at 13:44
1
True, it's overkill. But depending on the context you might have it imported already.
– David Speck
Nov 9 at 13:46
add a comment |
up vote
8
down vote
I'm not sure if this is more Pythonic:
>>> word_frequency = 'dogs': [1234, 4321], 'are': [9999, 0000], 'fun': [4389, 3234]
>>> vocab_frequency = [sum(x[0] for x in word_frequency.values()),
sum(x[1] for x in word_frequency.values())]
>>> print(vocab_frequency)
[15622, 7555]
Alternate solution with reduce
:
>>> reduce(lambda x, y: [x[0] + y[0], x[1] + y[1]], word_frequency.values())
[15622, 7555]
A list comprehension over a for loop is more Pythonic in my eyes.
– doctorlove
Nov 9 at 13:45
add a comment |
up vote
2
down vote
list(map(sum, zip(*word_frequency.values())))
I think this is the shortest possible solution to the problem.
– hochl
Nov 9 at 13:51
add a comment |
up vote
2
down vote
Probably not the shortest way to solve this, but hopefully the most comprehensible...
word_frequency = 'dogs': [1234, 4321], 'are': [9999, 0000], 'fun': [4389, 3234]
negative = (v[0] for v in word_frequency.values())
positive = (v[1] for v in word_frequency.values())
vocab_frequency = sum(negative), sum(positive)
print (vocab_frequency) # (15622, 7555)
Though more experienced Pythonistas might rather use zip to unpack the values:
negative, positive = zip(*word_frequency.values())
vocab_frequency = sum(negative), sum(positive)
add a comment |
up vote
1
down vote
for frequencies in word_frequency.values():
vocab_frequency = [sum(x) for x in zip(vocab_frequency, frequencies)]
add a comment |
up vote
1
down vote
Another approach would be this:
vocab_frequency[0], vocab_frequency[1] = list(sum([word_frequency[elem][i] for elem in word_frequency]) for i in range(2))
print(vocab_frequency[0])
print(vocab_frequency[1])
Output:
15622
7555
Still, one more way to do it, kind of far fetched is this:
*vocab_frequency, = list(map(sum,zip(*word_frequency.values())))
print(vocab_frequency)
Output:
[15622, 7555]
add a comment |
up vote
1
down vote
You can convert that dictionary to a pandas DataFrame and it would be a lot easier to handle.
import pandas as pd
word_frequency = 'dogs': [1234, 4321], 'are': [9999, 0000], 'fun': [4389, 3234]
#Syntax to create DataFrame
df = pd.DataFrame(word_frequency)
#Result
dogs are fun
0 1234 9999 4389
1 4321 0 3234
Now just take the sum of each row and either convert back to list or keep as a dataframe object.
#Take sum of each row and convert to list
df = df.sum(axis=1)
df = df.values.tolist()
print(df)
#Output
[15622, 7555]
New contributor
Drew Nicolette is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
Try This Single Line Solution:
[sum([word_frequency[i][0] for i in word_frequency]),sum([word_frequency[i][1] for i in word_frequency])]
add a comment |
up vote
0
down vote
for n, p in your_dict.vales():
res[0] += n
res[1] += p
This will be fast and elegant enough.
Sent from phone. Sorry for the format.
add a comment |
9 Answers
9
active
oldest
votes
9 Answers
9
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
4
down vote
accepted
You could use numpy for that:
import numpy as np
word_frequency = 'dogs': [1234, 4321], 'are': [9999, 0000], 'fun': [4389, 3234]
vocab_frequency = np.sum(list(word_frequency.values()), axis=0)
1
Using numpy is like total over kill :P
– SirtusKottus
Nov 9 at 13:43
Why numpy...its -like Sirtuskottus said- overkill
– NaruS
Nov 9 at 13:44
1
True, it's overkill. But depending on the context you might have it imported already.
– David Speck
Nov 9 at 13:46
add a comment |
up vote
4
down vote
accepted
You could use numpy for that:
import numpy as np
word_frequency = 'dogs': [1234, 4321], 'are': [9999, 0000], 'fun': [4389, 3234]
vocab_frequency = np.sum(list(word_frequency.values()), axis=0)
1
Using numpy is like total over kill :P
– SirtusKottus
Nov 9 at 13:43
Why numpy...its -like Sirtuskottus said- overkill
– NaruS
Nov 9 at 13:44
1
True, it's overkill. But depending on the context you might have it imported already.
– David Speck
Nov 9 at 13:46
add a comment |
up vote
4
down vote
accepted
up vote
4
down vote
accepted
You could use numpy for that:
import numpy as np
word_frequency = 'dogs': [1234, 4321], 'are': [9999, 0000], 'fun': [4389, 3234]
vocab_frequency = np.sum(list(word_frequency.values()), axis=0)
You could use numpy for that:
import numpy as np
word_frequency = 'dogs': [1234, 4321], 'are': [9999, 0000], 'fun': [4389, 3234]
vocab_frequency = np.sum(list(word_frequency.values()), axis=0)
answered Nov 9 at 13:42


David Speck
1586
1586
1
Using numpy is like total over kill :P
– SirtusKottus
Nov 9 at 13:43
Why numpy...its -like Sirtuskottus said- overkill
– NaruS
Nov 9 at 13:44
1
True, it's overkill. But depending on the context you might have it imported already.
– David Speck
Nov 9 at 13:46
add a comment |
1
Using numpy is like total over kill :P
– SirtusKottus
Nov 9 at 13:43
Why numpy...its -like Sirtuskottus said- overkill
– NaruS
Nov 9 at 13:44
1
True, it's overkill. But depending on the context you might have it imported already.
– David Speck
Nov 9 at 13:46
1
1
Using numpy is like total over kill :P
– SirtusKottus
Nov 9 at 13:43
Using numpy is like total over kill :P
– SirtusKottus
Nov 9 at 13:43
Why numpy...its -like Sirtuskottus said- overkill
– NaruS
Nov 9 at 13:44
Why numpy...its -like Sirtuskottus said- overkill
– NaruS
Nov 9 at 13:44
1
1
True, it's overkill. But depending on the context you might have it imported already.
– David Speck
Nov 9 at 13:46
True, it's overkill. But depending on the context you might have it imported already.
– David Speck
Nov 9 at 13:46
add a comment |
up vote
8
down vote
I'm not sure if this is more Pythonic:
>>> word_frequency = 'dogs': [1234, 4321], 'are': [9999, 0000], 'fun': [4389, 3234]
>>> vocab_frequency = [sum(x[0] for x in word_frequency.values()),
sum(x[1] for x in word_frequency.values())]
>>> print(vocab_frequency)
[15622, 7555]
Alternate solution with reduce
:
>>> reduce(lambda x, y: [x[0] + y[0], x[1] + y[1]], word_frequency.values())
[15622, 7555]
A list comprehension over a for loop is more Pythonic in my eyes.
– doctorlove
Nov 9 at 13:45
add a comment |
up vote
8
down vote
I'm not sure if this is more Pythonic:
>>> word_frequency = 'dogs': [1234, 4321], 'are': [9999, 0000], 'fun': [4389, 3234]
>>> vocab_frequency = [sum(x[0] for x in word_frequency.values()),
sum(x[1] for x in word_frequency.values())]
>>> print(vocab_frequency)
[15622, 7555]
Alternate solution with reduce
:
>>> reduce(lambda x, y: [x[0] + y[0], x[1] + y[1]], word_frequency.values())
[15622, 7555]
A list comprehension over a for loop is more Pythonic in my eyes.
– doctorlove
Nov 9 at 13:45
add a comment |
up vote
8
down vote
up vote
8
down vote
I'm not sure if this is more Pythonic:
>>> word_frequency = 'dogs': [1234, 4321], 'are': [9999, 0000], 'fun': [4389, 3234]
>>> vocab_frequency = [sum(x[0] for x in word_frequency.values()),
sum(x[1] for x in word_frequency.values())]
>>> print(vocab_frequency)
[15622, 7555]
Alternate solution with reduce
:
>>> reduce(lambda x, y: [x[0] + y[0], x[1] + y[1]], word_frequency.values())
[15622, 7555]
I'm not sure if this is more Pythonic:
>>> word_frequency = 'dogs': [1234, 4321], 'are': [9999, 0000], 'fun': [4389, 3234]
>>> vocab_frequency = [sum(x[0] for x in word_frequency.values()),
sum(x[1] for x in word_frequency.values())]
>>> print(vocab_frequency)
[15622, 7555]
Alternate solution with reduce
:
>>> reduce(lambda x, y: [x[0] + y[0], x[1] + y[1]], word_frequency.values())
[15622, 7555]
answered Nov 9 at 13:41
hochl
8,78273367
8,78273367
A list comprehension over a for loop is more Pythonic in my eyes.
– doctorlove
Nov 9 at 13:45
add a comment |
A list comprehension over a for loop is more Pythonic in my eyes.
– doctorlove
Nov 9 at 13:45
A list comprehension over a for loop is more Pythonic in my eyes.
– doctorlove
Nov 9 at 13:45
A list comprehension over a for loop is more Pythonic in my eyes.
– doctorlove
Nov 9 at 13:45
add a comment |
up vote
2
down vote
list(map(sum, zip(*word_frequency.values())))
I think this is the shortest possible solution to the problem.
– hochl
Nov 9 at 13:51
add a comment |
up vote
2
down vote
list(map(sum, zip(*word_frequency.values())))
I think this is the shortest possible solution to the problem.
– hochl
Nov 9 at 13:51
add a comment |
up vote
2
down vote
up vote
2
down vote
list(map(sum, zip(*word_frequency.values())))
list(map(sum, zip(*word_frequency.values())))
answered Nov 9 at 13:49
drhodes
635814
635814
I think this is the shortest possible solution to the problem.
– hochl
Nov 9 at 13:51
add a comment |
I think this is the shortest possible solution to the problem.
– hochl
Nov 9 at 13:51
I think this is the shortest possible solution to the problem.
– hochl
Nov 9 at 13:51
I think this is the shortest possible solution to the problem.
– hochl
Nov 9 at 13:51
add a comment |
up vote
2
down vote
Probably not the shortest way to solve this, but hopefully the most comprehensible...
word_frequency = 'dogs': [1234, 4321], 'are': [9999, 0000], 'fun': [4389, 3234]
negative = (v[0] for v in word_frequency.values())
positive = (v[1] for v in word_frequency.values())
vocab_frequency = sum(negative), sum(positive)
print (vocab_frequency) # (15622, 7555)
Though more experienced Pythonistas might rather use zip to unpack the values:
negative, positive = zip(*word_frequency.values())
vocab_frequency = sum(negative), sum(positive)
add a comment |
up vote
2
down vote
Probably not the shortest way to solve this, but hopefully the most comprehensible...
word_frequency = 'dogs': [1234, 4321], 'are': [9999, 0000], 'fun': [4389, 3234]
negative = (v[0] for v in word_frequency.values())
positive = (v[1] for v in word_frequency.values())
vocab_frequency = sum(negative), sum(positive)
print (vocab_frequency) # (15622, 7555)
Though more experienced Pythonistas might rather use zip to unpack the values:
negative, positive = zip(*word_frequency.values())
vocab_frequency = sum(negative), sum(positive)
add a comment |
up vote
2
down vote
up vote
2
down vote
Probably not the shortest way to solve this, but hopefully the most comprehensible...
word_frequency = 'dogs': [1234, 4321], 'are': [9999, 0000], 'fun': [4389, 3234]
negative = (v[0] for v in word_frequency.values())
positive = (v[1] for v in word_frequency.values())
vocab_frequency = sum(negative), sum(positive)
print (vocab_frequency) # (15622, 7555)
Though more experienced Pythonistas might rather use zip to unpack the values:
negative, positive = zip(*word_frequency.values())
vocab_frequency = sum(negative), sum(positive)
Probably not the shortest way to solve this, but hopefully the most comprehensible...
word_frequency = 'dogs': [1234, 4321], 'are': [9999, 0000], 'fun': [4389, 3234]
negative = (v[0] for v in word_frequency.values())
positive = (v[1] for v in word_frequency.values())
vocab_frequency = sum(negative), sum(positive)
print (vocab_frequency) # (15622, 7555)
Though more experienced Pythonistas might rather use zip to unpack the values:
negative, positive = zip(*word_frequency.values())
vocab_frequency = sum(negative), sum(positive)
edited Nov 9 at 14:00
answered Nov 9 at 13:50


Sebastian Loehner
59735
59735
add a comment |
add a comment |
up vote
1
down vote
for frequencies in word_frequency.values():
vocab_frequency = [sum(x) for x in zip(vocab_frequency, frequencies)]
add a comment |
up vote
1
down vote
for frequencies in word_frequency.values():
vocab_frequency = [sum(x) for x in zip(vocab_frequency, frequencies)]
add a comment |
up vote
1
down vote
up vote
1
down vote
for frequencies in word_frequency.values():
vocab_frequency = [sum(x) for x in zip(vocab_frequency, frequencies)]
for frequencies in word_frequency.values():
vocab_frequency = [sum(x) for x in zip(vocab_frequency, frequencies)]
answered Nov 9 at 13:46
Karl
1,28842649
1,28842649
add a comment |
add a comment |
up vote
1
down vote
Another approach would be this:
vocab_frequency[0], vocab_frequency[1] = list(sum([word_frequency[elem][i] for elem in word_frequency]) for i in range(2))
print(vocab_frequency[0])
print(vocab_frequency[1])
Output:
15622
7555
Still, one more way to do it, kind of far fetched is this:
*vocab_frequency, = list(map(sum,zip(*word_frequency.values())))
print(vocab_frequency)
Output:
[15622, 7555]
add a comment |
up vote
1
down vote
Another approach would be this:
vocab_frequency[0], vocab_frequency[1] = list(sum([word_frequency[elem][i] for elem in word_frequency]) for i in range(2))
print(vocab_frequency[0])
print(vocab_frequency[1])
Output:
15622
7555
Still, one more way to do it, kind of far fetched is this:
*vocab_frequency, = list(map(sum,zip(*word_frequency.values())))
print(vocab_frequency)
Output:
[15622, 7555]
add a comment |
up vote
1
down vote
up vote
1
down vote
Another approach would be this:
vocab_frequency[0], vocab_frequency[1] = list(sum([word_frequency[elem][i] for elem in word_frequency]) for i in range(2))
print(vocab_frequency[0])
print(vocab_frequency[1])
Output:
15622
7555
Still, one more way to do it, kind of far fetched is this:
*vocab_frequency, = list(map(sum,zip(*word_frequency.values())))
print(vocab_frequency)
Output:
[15622, 7555]
Another approach would be this:
vocab_frequency[0], vocab_frequency[1] = list(sum([word_frequency[elem][i] for elem in word_frequency]) for i in range(2))
print(vocab_frequency[0])
print(vocab_frequency[1])
Output:
15622
7555
Still, one more way to do it, kind of far fetched is this:
*vocab_frequency, = list(map(sum,zip(*word_frequency.values())))
print(vocab_frequency)
Output:
[15622, 7555]
edited Nov 9 at 13:52
answered Nov 9 at 13:44


Vasilis G.
2,5892621
2,5892621
add a comment |
add a comment |
up vote
1
down vote
You can convert that dictionary to a pandas DataFrame and it would be a lot easier to handle.
import pandas as pd
word_frequency = 'dogs': [1234, 4321], 'are': [9999, 0000], 'fun': [4389, 3234]
#Syntax to create DataFrame
df = pd.DataFrame(word_frequency)
#Result
dogs are fun
0 1234 9999 4389
1 4321 0 3234
Now just take the sum of each row and either convert back to list or keep as a dataframe object.
#Take sum of each row and convert to list
df = df.sum(axis=1)
df = df.values.tolist()
print(df)
#Output
[15622, 7555]
New contributor
Drew Nicolette is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
1
down vote
You can convert that dictionary to a pandas DataFrame and it would be a lot easier to handle.
import pandas as pd
word_frequency = 'dogs': [1234, 4321], 'are': [9999, 0000], 'fun': [4389, 3234]
#Syntax to create DataFrame
df = pd.DataFrame(word_frequency)
#Result
dogs are fun
0 1234 9999 4389
1 4321 0 3234
Now just take the sum of each row and either convert back to list or keep as a dataframe object.
#Take sum of each row and convert to list
df = df.sum(axis=1)
df = df.values.tolist()
print(df)
#Output
[15622, 7555]
New contributor
Drew Nicolette is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
1
down vote
up vote
1
down vote
You can convert that dictionary to a pandas DataFrame and it would be a lot easier to handle.
import pandas as pd
word_frequency = 'dogs': [1234, 4321], 'are': [9999, 0000], 'fun': [4389, 3234]
#Syntax to create DataFrame
df = pd.DataFrame(word_frequency)
#Result
dogs are fun
0 1234 9999 4389
1 4321 0 3234
Now just take the sum of each row and either convert back to list or keep as a dataframe object.
#Take sum of each row and convert to list
df = df.sum(axis=1)
df = df.values.tolist()
print(df)
#Output
[15622, 7555]
New contributor
Drew Nicolette is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
You can convert that dictionary to a pandas DataFrame and it would be a lot easier to handle.
import pandas as pd
word_frequency = 'dogs': [1234, 4321], 'are': [9999, 0000], 'fun': [4389, 3234]
#Syntax to create DataFrame
df = pd.DataFrame(word_frequency)
#Result
dogs are fun
0 1234 9999 4389
1 4321 0 3234
Now just take the sum of each row and either convert back to list or keep as a dataframe object.
#Take sum of each row and convert to list
df = df.sum(axis=1)
df = df.values.tolist()
print(df)
#Output
[15622, 7555]
New contributor
Drew Nicolette is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Drew Nicolette is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
answered Nov 9 at 13:53


Drew Nicolette
963
963
New contributor
Drew Nicolette is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Drew Nicolette is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Drew Nicolette is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
up vote
0
down vote
Try This Single Line Solution:
[sum([word_frequency[i][0] for i in word_frequency]),sum([word_frequency[i][1] for i in word_frequency])]
add a comment |
up vote
0
down vote
Try This Single Line Solution:
[sum([word_frequency[i][0] for i in word_frequency]),sum([word_frequency[i][1] for i in word_frequency])]
add a comment |
up vote
0
down vote
up vote
0
down vote
Try This Single Line Solution:
[sum([word_frequency[i][0] for i in word_frequency]),sum([word_frequency[i][1] for i in word_frequency])]
Try This Single Line Solution:
[sum([word_frequency[i][0] for i in word_frequency]),sum([word_frequency[i][1] for i in word_frequency])]
answered Nov 9 at 14:06


Rohit-Pandey
888613
888613
add a comment |
add a comment |
up vote
0
down vote
for n, p in your_dict.vales():
res[0] += n
res[1] += p
This will be fast and elegant enough.
Sent from phone. Sorry for the format.
add a comment |
up vote
0
down vote
for n, p in your_dict.vales():
res[0] += n
res[1] += p
This will be fast and elegant enough.
Sent from phone. Sorry for the format.
add a comment |
up vote
0
down vote
up vote
0
down vote
for n, p in your_dict.vales():
res[0] += n
res[1] += p
This will be fast and elegant enough.
Sent from phone. Sorry for the format.
for n, p in your_dict.vales():
res[0] += n
res[1] += p
This will be fast and elegant enough.
Sent from phone. Sorry for the format.
answered Nov 9 at 14:11


fakeKmz
146
146
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53226715%2fpythonic-way-of-executing-a-loop-on-a-dictionary-of-lists%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
1HNK0LF3,9ZUviq0fk6L,kk,l3,RL ZadKzNb H,Ogjr f9lZRa,cl
2
Since you're not really using
word
except to look back intoword_frequency
you can iterate overword_frequency.values()
.– pdexter
Nov 9 at 13:41
Lot's of simple and elegant answers here. So... I guess we might say "pythonic" means that the same thing can be done in a myriad of neat ways?
– Karl
Nov 9 at 21:52
@Karl python allows us to write very neat and concise code. However if, like me, you learned to program in C, many times you will catch yourself creating resource consuming loops and functions that could be handled in a much more elegant and often more efficient way. blog.startifact.com/posts/older/what-is-pythonic.html
– Victor Zuanazzi
Nov 10 at 14:20
Yee, my post was nothing more than a recognition of that. I was trying to point out if you ask a community of python programmers to come up the "the most pythonic way" (a question which I see all the time), what is revealed as pythonic is in fact that python allows for such a compact idiom in multiple ways
– Karl
Nov 10 at 17:33