d3 line chart - object digging
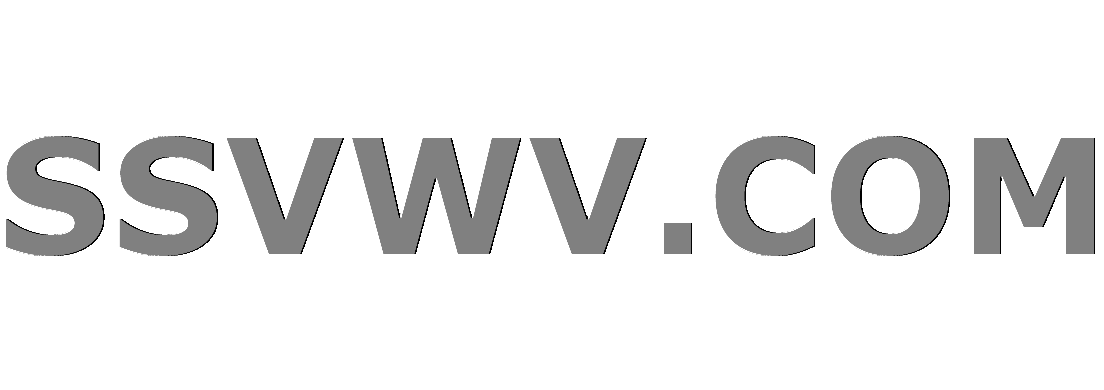
Multi tool use
up vote
0
down vote
favorite
Trying to build a linechart (multiple lines). Initial data has been an array of object such as:
[
2010: 8236.082,
countryName: "Afghanistan"
]
Each line required an array of x/y pairs [[x,y],[x,y]]. My x and y are year and amount of emissions. This means I had to restructure my data it to make it look like this:
[
country: "Afganistan",
emissions: [
year: 2019, amount: 8236.082
]
]
However this doesn't work for me. Is the problem in the domain?
Please help.
Codepen
//Define full width, full height and margins
let fullWidth = 600;
let fullHeight = 700;
let margin =
top: 20,
left: 70,
bottom: 100,
right: 10
//Define line chart with and height
let width = fullWidth - margin.left - margin.right;
let height = fullHeight - margin.top - margin.bottom;
//Define x and y scale range
let xScale = d3.scaleLinear()
.range([0, width])
let yScale = d3.scaleLinear()
.range([0, height])
//Draw svg
let svg = d3.select("body")
.attr("width", fullWidth)
.attr("height", fullHeight)
.append("svg")
.append("g")
d3.json("https://api.myjson.com/bins/izmg6").then(data =>
console.log(data);
//Structure data so should be an array of arrays etc [[x,y], [x,y], [x,y]]
let years = d3.keys(data[0]).slice(0, 50);
console.log(years);
let dataset = ;
data.forEach((d, i) =>
let myEmissions = ;
years.forEach(y =>
if (d[y])
myEmissions.push(
year: y,
amount: d[y]
)
)
dataset.push(
country: d.countryName,
emissions: myEmissions
);
)
console.log(dataset);
//Define x and y domain
xScale
.domain(d3.extent(years, d =>d))
yScale
.domain([d3.max(dataset, d =>
d3.max(d.emissions, d =>
+d.amount)), 0])
//Generate line
let line = d3.line()
.x(d =>
xScale(d.year))
.y(d =>
yScale(d.amount));
let groups = svg.selectAll("g")
.data(dataset)
.enter()
.append("g")
groups.append("title")
.text(d => d.country)
groups.selectAll("path")
.data(d => [d.emissions])
.enter()
.append("path")
.attr("d", line)
.attr("class", line)
).catch(error => console.log(error))
javascript arrays object d3.js
add a comment |
up vote
0
down vote
favorite
Trying to build a linechart (multiple lines). Initial data has been an array of object such as:
[
2010: 8236.082,
countryName: "Afghanistan"
]
Each line required an array of x/y pairs [[x,y],[x,y]]. My x and y are year and amount of emissions. This means I had to restructure my data it to make it look like this:
[
country: "Afganistan",
emissions: [
year: 2019, amount: 8236.082
]
]
However this doesn't work for me. Is the problem in the domain?
Please help.
Codepen
//Define full width, full height and margins
let fullWidth = 600;
let fullHeight = 700;
let margin =
top: 20,
left: 70,
bottom: 100,
right: 10
//Define line chart with and height
let width = fullWidth - margin.left - margin.right;
let height = fullHeight - margin.top - margin.bottom;
//Define x and y scale range
let xScale = d3.scaleLinear()
.range([0, width])
let yScale = d3.scaleLinear()
.range([0, height])
//Draw svg
let svg = d3.select("body")
.attr("width", fullWidth)
.attr("height", fullHeight)
.append("svg")
.append("g")
d3.json("https://api.myjson.com/bins/izmg6").then(data =>
console.log(data);
//Structure data so should be an array of arrays etc [[x,y], [x,y], [x,y]]
let years = d3.keys(data[0]).slice(0, 50);
console.log(years);
let dataset = ;
data.forEach((d, i) =>
let myEmissions = ;
years.forEach(y =>
if (d[y])
myEmissions.push(
year: y,
amount: d[y]
)
)
dataset.push(
country: d.countryName,
emissions: myEmissions
);
)
console.log(dataset);
//Define x and y domain
xScale
.domain(d3.extent(years, d =>d))
yScale
.domain([d3.max(dataset, d =>
d3.max(d.emissions, d =>
+d.amount)), 0])
//Generate line
let line = d3.line()
.x(d =>
xScale(d.year))
.y(d =>
yScale(d.amount));
let groups = svg.selectAll("g")
.data(dataset)
.enter()
.append("g")
groups.append("title")
.text(d => d.country)
groups.selectAll("path")
.data(d => [d.emissions])
.enter()
.append("path")
.attr("d", line)
.attr("class", line)
).catch(error => console.log(error))
javascript arrays object d3.js
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
Trying to build a linechart (multiple lines). Initial data has been an array of object such as:
[
2010: 8236.082,
countryName: "Afghanistan"
]
Each line required an array of x/y pairs [[x,y],[x,y]]. My x and y are year and amount of emissions. This means I had to restructure my data it to make it look like this:
[
country: "Afganistan",
emissions: [
year: 2019, amount: 8236.082
]
]
However this doesn't work for me. Is the problem in the domain?
Please help.
Codepen
//Define full width, full height and margins
let fullWidth = 600;
let fullHeight = 700;
let margin =
top: 20,
left: 70,
bottom: 100,
right: 10
//Define line chart with and height
let width = fullWidth - margin.left - margin.right;
let height = fullHeight - margin.top - margin.bottom;
//Define x and y scale range
let xScale = d3.scaleLinear()
.range([0, width])
let yScale = d3.scaleLinear()
.range([0, height])
//Draw svg
let svg = d3.select("body")
.attr("width", fullWidth)
.attr("height", fullHeight)
.append("svg")
.append("g")
d3.json("https://api.myjson.com/bins/izmg6").then(data =>
console.log(data);
//Structure data so should be an array of arrays etc [[x,y], [x,y], [x,y]]
let years = d3.keys(data[0]).slice(0, 50);
console.log(years);
let dataset = ;
data.forEach((d, i) =>
let myEmissions = ;
years.forEach(y =>
if (d[y])
myEmissions.push(
year: y,
amount: d[y]
)
)
dataset.push(
country: d.countryName,
emissions: myEmissions
);
)
console.log(dataset);
//Define x and y domain
xScale
.domain(d3.extent(years, d =>d))
yScale
.domain([d3.max(dataset, d =>
d3.max(d.emissions, d =>
+d.amount)), 0])
//Generate line
let line = d3.line()
.x(d =>
xScale(d.year))
.y(d =>
yScale(d.amount));
let groups = svg.selectAll("g")
.data(dataset)
.enter()
.append("g")
groups.append("title")
.text(d => d.country)
groups.selectAll("path")
.data(d => [d.emissions])
.enter()
.append("path")
.attr("d", line)
.attr("class", line)
).catch(error => console.log(error))
javascript arrays object d3.js
Trying to build a linechart (multiple lines). Initial data has been an array of object such as:
[
2010: 8236.082,
countryName: "Afghanistan"
]
Each line required an array of x/y pairs [[x,y],[x,y]]. My x and y are year and amount of emissions. This means I had to restructure my data it to make it look like this:
[
country: "Afganistan",
emissions: [
year: 2019, amount: 8236.082
]
]
However this doesn't work for me. Is the problem in the domain?
Please help.
Codepen
//Define full width, full height and margins
let fullWidth = 600;
let fullHeight = 700;
let margin =
top: 20,
left: 70,
bottom: 100,
right: 10
//Define line chart with and height
let width = fullWidth - margin.left - margin.right;
let height = fullHeight - margin.top - margin.bottom;
//Define x and y scale range
let xScale = d3.scaleLinear()
.range([0, width])
let yScale = d3.scaleLinear()
.range([0, height])
//Draw svg
let svg = d3.select("body")
.attr("width", fullWidth)
.attr("height", fullHeight)
.append("svg")
.append("g")
d3.json("https://api.myjson.com/bins/izmg6").then(data =>
console.log(data);
//Structure data so should be an array of arrays etc [[x,y], [x,y], [x,y]]
let years = d3.keys(data[0]).slice(0, 50);
console.log(years);
let dataset = ;
data.forEach((d, i) =>
let myEmissions = ;
years.forEach(y =>
if (d[y])
myEmissions.push(
year: y,
amount: d[y]
)
)
dataset.push(
country: d.countryName,
emissions: myEmissions
);
)
console.log(dataset);
//Define x and y domain
xScale
.domain(d3.extent(years, d =>d))
yScale
.domain([d3.max(dataset, d =>
d3.max(d.emissions, d =>
+d.amount)), 0])
//Generate line
let line = d3.line()
.x(d =>
xScale(d.year))
.y(d =>
yScale(d.amount));
let groups = svg.selectAll("g")
.data(dataset)
.enter()
.append("g")
groups.append("title")
.text(d => d.country)
groups.selectAll("path")
.data(d => [d.emissions])
.enter()
.append("path")
.attr("d", line)
.attr("class", line)
).catch(error => console.log(error))
javascript arrays object d3.js
javascript arrays object d3.js
asked Nov 9 at 14:06


Edgar Kiljak
19312
19312
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
accepted
Minor change/typo:
You're assigning height
and width
to the body
and not the svg
. Interchanging those 2 lines:
let svg = d3.select("body")
.append("svg")
.attr("width", fullWidth)
.attr("height", fullHeight)
And adding some CSS to the paths:
path.line
fill: none;
stroke: #000;
Here's a fork: https://codepen.io/shashank2104/pen/GwqjVK
Thank you! Works perfectly.
– Edgar Kiljak
Nov 10 at 14:00
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
Minor change/typo:
You're assigning height
and width
to the body
and not the svg
. Interchanging those 2 lines:
let svg = d3.select("body")
.append("svg")
.attr("width", fullWidth)
.attr("height", fullHeight)
And adding some CSS to the paths:
path.line
fill: none;
stroke: #000;
Here's a fork: https://codepen.io/shashank2104/pen/GwqjVK
Thank you! Works perfectly.
– Edgar Kiljak
Nov 10 at 14:00
add a comment |
up vote
1
down vote
accepted
Minor change/typo:
You're assigning height
and width
to the body
and not the svg
. Interchanging those 2 lines:
let svg = d3.select("body")
.append("svg")
.attr("width", fullWidth)
.attr("height", fullHeight)
And adding some CSS to the paths:
path.line
fill: none;
stroke: #000;
Here's a fork: https://codepen.io/shashank2104/pen/GwqjVK
Thank you! Works perfectly.
– Edgar Kiljak
Nov 10 at 14:00
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
Minor change/typo:
You're assigning height
and width
to the body
and not the svg
. Interchanging those 2 lines:
let svg = d3.select("body")
.append("svg")
.attr("width", fullWidth)
.attr("height", fullHeight)
And adding some CSS to the paths:
path.line
fill: none;
stroke: #000;
Here's a fork: https://codepen.io/shashank2104/pen/GwqjVK
Minor change/typo:
You're assigning height
and width
to the body
and not the svg
. Interchanging those 2 lines:
let svg = d3.select("body")
.append("svg")
.attr("width", fullWidth)
.attr("height", fullHeight)
And adding some CSS to the paths:
path.line
fill: none;
stroke: #000;
Here's a fork: https://codepen.io/shashank2104/pen/GwqjVK
answered Nov 9 at 14:42
Shashank
4,1351412
4,1351412
Thank you! Works perfectly.
– Edgar Kiljak
Nov 10 at 14:00
add a comment |
Thank you! Works perfectly.
– Edgar Kiljak
Nov 10 at 14:00
Thank you! Works perfectly.
– Edgar Kiljak
Nov 10 at 14:00
Thank you! Works perfectly.
– Edgar Kiljak
Nov 10 at 14:00
add a comment |
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53227253%2fd3-line-chart-object-digging%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
YpjFNZqeE Nt W lv9e3Fp8WoO ff6ig23,Mhth CCIA8jl7