Java httpServer basic authentication for different request methods
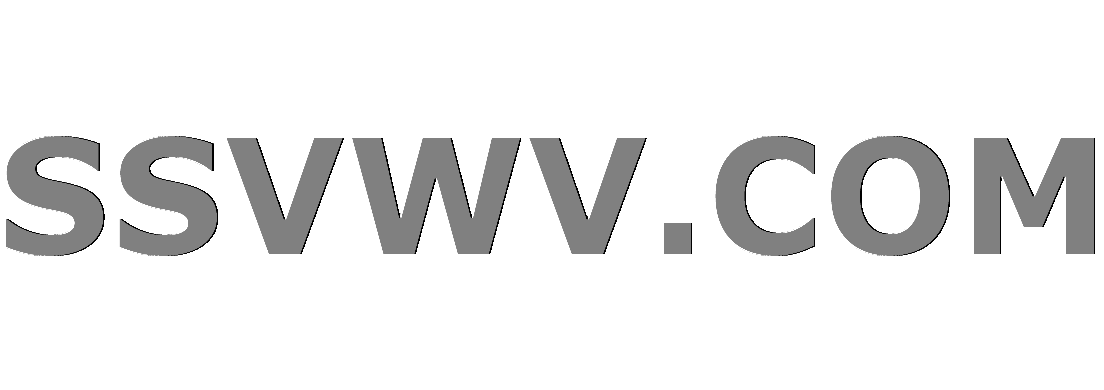
Multi tool use
up vote
5
down vote
favorite
I'm using a very simple httpServer in Java for an api rest with GET, POST, PUT and DELETE. I'm using Basic Authentication and I have a couple classes Authentication.java and Authorisation.java which I use to authenticate and check permissions for the users.
So, the thing is that I want all users (authenticated) to be able to GET information from my api rest, but only users with certain privileges to be able to POST, PUT and DELETE. So how can I do that?
This is what I got
public class Server
private static HttpServer server;
public static void start() throws IOException
server = HttpServer.create(new InetSocketAddress(8000), 0);
HttpContext ctx = server.createContext("/users", new UserHandler());
ctx.setAuthenticator(new ApiRestBasicAuthentication("users"));
server.start();
And this is my ApiRestBasicAuthentication
public class ApiRestBasicAuthentication extends BasicAuthenticator
private UserAuthentication authentication = new UserAuthentication();
public ApiRestBasicAuthentication(String realm)
super(realm);
@Override
public boolean checkCredentials(String user, String pwd)
int authCode = authentication.authenticate(user, pwd);
return authCode == UserAuthentication.USER_AUTHENTICATED;
As this is now, check credentials is only checking if the user is authenticated.
But I'd like to check, if the method is POST, DELETE or PUT I should also check the specific credentials. But how can I get the method in my ApiRestBasicAuthentication? I'm doing that in my handler class
public void handle(HttpExchange httpExchange) throws IOException
String method = httpExchange.getRequestMethod();
if ("post".equalsIgnoreCase(method))
createUser(httpExchange);
else if ("get".equalsIgnoreCase(method))
readUsers(httpExchange);
else if ("put".equalsIgnoreCase(method))
updateUser(httpExchange);
else if ("delete".equalsIgnoreCase(method))
deleteUser(httpExchange);
Maybe this is supposed to be done some other way.
Any ideas?
Many thanks.
java rest basic-authentication httpserver
This question has an open bounty worth +100
reputation from user2690527 ending ending at 2018-11-15 11:38:59Z">tomorrow.
This question has not received enough attention.
add a comment |
up vote
5
down vote
favorite
I'm using a very simple httpServer in Java for an api rest with GET, POST, PUT and DELETE. I'm using Basic Authentication and I have a couple classes Authentication.java and Authorisation.java which I use to authenticate and check permissions for the users.
So, the thing is that I want all users (authenticated) to be able to GET information from my api rest, but only users with certain privileges to be able to POST, PUT and DELETE. So how can I do that?
This is what I got
public class Server
private static HttpServer server;
public static void start() throws IOException
server = HttpServer.create(new InetSocketAddress(8000), 0);
HttpContext ctx = server.createContext("/users", new UserHandler());
ctx.setAuthenticator(new ApiRestBasicAuthentication("users"));
server.start();
And this is my ApiRestBasicAuthentication
public class ApiRestBasicAuthentication extends BasicAuthenticator
private UserAuthentication authentication = new UserAuthentication();
public ApiRestBasicAuthentication(String realm)
super(realm);
@Override
public boolean checkCredentials(String user, String pwd)
int authCode = authentication.authenticate(user, pwd);
return authCode == UserAuthentication.USER_AUTHENTICATED;
As this is now, check credentials is only checking if the user is authenticated.
But I'd like to check, if the method is POST, DELETE or PUT I should also check the specific credentials. But how can I get the method in my ApiRestBasicAuthentication? I'm doing that in my handler class
public void handle(HttpExchange httpExchange) throws IOException
String method = httpExchange.getRequestMethod();
if ("post".equalsIgnoreCase(method))
createUser(httpExchange);
else if ("get".equalsIgnoreCase(method))
readUsers(httpExchange);
else if ("put".equalsIgnoreCase(method))
updateUser(httpExchange);
else if ("delete".equalsIgnoreCase(method))
deleteUser(httpExchange);
Maybe this is supposed to be done some other way.
Any ideas?
Many thanks.
java rest basic-authentication httpserver
This question has an open bounty worth +100
reputation from user2690527 ending ending at 2018-11-15 11:38:59Z">tomorrow.
This question has not received enough attention.
I suppose you'll need some authorisation aspect (or similiar functionality given by filter or interceptor) that will only respond to non-GETs if user is authorised, though I cannot help you right now with full answer. Figure out which of those (aspect, filter, interceptor) you have available and read about it.
– Filip Malczak
Dec 31 '16 at 11:16
That could work because I can check the method (POST, GET, PUT,...) in the filter, but then how can I get the username sent in the request inside the filter? My ApiRestBasicAuthentication has a checkCredentials() function which receives the user and password, but in the Filter I just have the httpExchange object, and the username/password is encripted.
– David
Dec 31 '16 at 17:31
I suggest you to usespring-security
for that. Take a lookt at the following answer: stackoverflow.com/a/45965232/540286
– Ortwin Angermeier
Nov 9 at 15:30
add a comment |
up vote
5
down vote
favorite
up vote
5
down vote
favorite
I'm using a very simple httpServer in Java for an api rest with GET, POST, PUT and DELETE. I'm using Basic Authentication and I have a couple classes Authentication.java and Authorisation.java which I use to authenticate and check permissions for the users.
So, the thing is that I want all users (authenticated) to be able to GET information from my api rest, but only users with certain privileges to be able to POST, PUT and DELETE. So how can I do that?
This is what I got
public class Server
private static HttpServer server;
public static void start() throws IOException
server = HttpServer.create(new InetSocketAddress(8000), 0);
HttpContext ctx = server.createContext("/users", new UserHandler());
ctx.setAuthenticator(new ApiRestBasicAuthentication("users"));
server.start();
And this is my ApiRestBasicAuthentication
public class ApiRestBasicAuthentication extends BasicAuthenticator
private UserAuthentication authentication = new UserAuthentication();
public ApiRestBasicAuthentication(String realm)
super(realm);
@Override
public boolean checkCredentials(String user, String pwd)
int authCode = authentication.authenticate(user, pwd);
return authCode == UserAuthentication.USER_AUTHENTICATED;
As this is now, check credentials is only checking if the user is authenticated.
But I'd like to check, if the method is POST, DELETE or PUT I should also check the specific credentials. But how can I get the method in my ApiRestBasicAuthentication? I'm doing that in my handler class
public void handle(HttpExchange httpExchange) throws IOException
String method = httpExchange.getRequestMethod();
if ("post".equalsIgnoreCase(method))
createUser(httpExchange);
else if ("get".equalsIgnoreCase(method))
readUsers(httpExchange);
else if ("put".equalsIgnoreCase(method))
updateUser(httpExchange);
else if ("delete".equalsIgnoreCase(method))
deleteUser(httpExchange);
Maybe this is supposed to be done some other way.
Any ideas?
Many thanks.
java rest basic-authentication httpserver
I'm using a very simple httpServer in Java for an api rest with GET, POST, PUT and DELETE. I'm using Basic Authentication and I have a couple classes Authentication.java and Authorisation.java which I use to authenticate and check permissions for the users.
So, the thing is that I want all users (authenticated) to be able to GET information from my api rest, but only users with certain privileges to be able to POST, PUT and DELETE. So how can I do that?
This is what I got
public class Server
private static HttpServer server;
public static void start() throws IOException
server = HttpServer.create(new InetSocketAddress(8000), 0);
HttpContext ctx = server.createContext("/users", new UserHandler());
ctx.setAuthenticator(new ApiRestBasicAuthentication("users"));
server.start();
And this is my ApiRestBasicAuthentication
public class ApiRestBasicAuthentication extends BasicAuthenticator
private UserAuthentication authentication = new UserAuthentication();
public ApiRestBasicAuthentication(String realm)
super(realm);
@Override
public boolean checkCredentials(String user, String pwd)
int authCode = authentication.authenticate(user, pwd);
return authCode == UserAuthentication.USER_AUTHENTICATED;
As this is now, check credentials is only checking if the user is authenticated.
But I'd like to check, if the method is POST, DELETE or PUT I should also check the specific credentials. But how can I get the method in my ApiRestBasicAuthentication? I'm doing that in my handler class
public void handle(HttpExchange httpExchange) throws IOException
String method = httpExchange.getRequestMethod();
if ("post".equalsIgnoreCase(method))
createUser(httpExchange);
else if ("get".equalsIgnoreCase(method))
readUsers(httpExchange);
else if ("put".equalsIgnoreCase(method))
updateUser(httpExchange);
else if ("delete".equalsIgnoreCase(method))
deleteUser(httpExchange);
Maybe this is supposed to be done some other way.
Any ideas?
Many thanks.
java rest basic-authentication httpserver
java rest basic-authentication httpserver
asked Dec 31 '16 at 10:31
David
88021548
88021548
This question has an open bounty worth +100
reputation from user2690527 ending ending at 2018-11-15 11:38:59Z">tomorrow.
This question has not received enough attention.
This question has an open bounty worth +100
reputation from user2690527 ending ending at 2018-11-15 11:38:59Z">tomorrow.
This question has not received enough attention.
I suppose you'll need some authorisation aspect (or similiar functionality given by filter or interceptor) that will only respond to non-GETs if user is authorised, though I cannot help you right now with full answer. Figure out which of those (aspect, filter, interceptor) you have available and read about it.
– Filip Malczak
Dec 31 '16 at 11:16
That could work because I can check the method (POST, GET, PUT,...) in the filter, but then how can I get the username sent in the request inside the filter? My ApiRestBasicAuthentication has a checkCredentials() function which receives the user and password, but in the Filter I just have the httpExchange object, and the username/password is encripted.
– David
Dec 31 '16 at 17:31
I suggest you to usespring-security
for that. Take a lookt at the following answer: stackoverflow.com/a/45965232/540286
– Ortwin Angermeier
Nov 9 at 15:30
add a comment |
I suppose you'll need some authorisation aspect (or similiar functionality given by filter or interceptor) that will only respond to non-GETs if user is authorised, though I cannot help you right now with full answer. Figure out which of those (aspect, filter, interceptor) you have available and read about it.
– Filip Malczak
Dec 31 '16 at 11:16
That could work because I can check the method (POST, GET, PUT,...) in the filter, but then how can I get the username sent in the request inside the filter? My ApiRestBasicAuthentication has a checkCredentials() function which receives the user and password, but in the Filter I just have the httpExchange object, and the username/password is encripted.
– David
Dec 31 '16 at 17:31
I suggest you to usespring-security
for that. Take a lookt at the following answer: stackoverflow.com/a/45965232/540286
– Ortwin Angermeier
Nov 9 at 15:30
I suppose you'll need some authorisation aspect (or similiar functionality given by filter or interceptor) that will only respond to non-GETs if user is authorised, though I cannot help you right now with full answer. Figure out which of those (aspect, filter, interceptor) you have available and read about it.
– Filip Malczak
Dec 31 '16 at 11:16
I suppose you'll need some authorisation aspect (or similiar functionality given by filter or interceptor) that will only respond to non-GETs if user is authorised, though I cannot help you right now with full answer. Figure out which of those (aspect, filter, interceptor) you have available and read about it.
– Filip Malczak
Dec 31 '16 at 11:16
That could work because I can check the method (POST, GET, PUT,...) in the filter, but then how can I get the username sent in the request inside the filter? My ApiRestBasicAuthentication has a checkCredentials() function which receives the user and password, but in the Filter I just have the httpExchange object, and the username/password is encripted.
– David
Dec 31 '16 at 17:31
That could work because I can check the method (POST, GET, PUT,...) in the filter, but then how can I get the username sent in the request inside the filter? My ApiRestBasicAuthentication has a checkCredentials() function which receives the user and password, but in the Filter I just have the httpExchange object, and the username/password is encripted.
– David
Dec 31 '16 at 17:31
I suggest you to use
spring-security
for that. Take a lookt at the following answer: stackoverflow.com/a/45965232/540286– Ortwin Angermeier
Nov 9 at 15:30
I suggest you to use
spring-security
for that. Take a lookt at the following answer: stackoverflow.com/a/45965232/540286– Ortwin Angermeier
Nov 9 at 15:30
add a comment |
3 Answers
3
active
oldest
votes
up vote
3
down vote
accepted
A simple way to do it would be to change your
ApiRestBasicAuthentication like:
public class ApiRestBasicAuthentication extends BasicAuthenticator
private UserAuthentication authentication = new UserAuthentication();
public ApiRestBasicAuthentication(String realm)
super(realm);
@Override
public Authenticator.Result authenticate(HttpExchange exch)
Authenticator.Result result=super.authenticate(exch);
if(result instanceof Authenticator.Success)
HttpPrincipal principal=((Authenticator.Success)result).getPrincipal();
String requestMethod=exch.getRequestMethod();
if( ADD SOME LOGIC HERE FOR PRINCIPAL AND REQUEST METHOD)
return new return new Authenticator.Failure(401);
return result;
@Override
public boolean checkCredentials(String user, String pwd)
int authCode = authentication.authenticate(user, pwd);
return authCode == UserAuthentication.USER_AUTHENTICATED;
And add some logic there for requests/users that you want to fail the authenticator. I have shown you here how to get the method in the authenticate method but you need to specify the types of credentials.
Another solution would be if you check the source code of BasicAuthenticator you can see how it implements authenticate
method and you can create your own implementation in a similar way instead of extending BasicAuthenticator and use the get method instead of just the username and password. You can see the source code here and I am sure you will be able to find your way around ;)
Usually in enterprise application you can use some external security management system - for example if you use Spring (the de facto standard in the current java web apps) you can use spring security and do such security patterns and filters in a more declarative way
add a comment |
up vote
0
down vote
There might be many ways to solve this issue. Here is one of my proposal:
Create a User Object with fields that you want and one field called something like "role". Lets say only "admins" are allowed to do make Http requests other than "GET" while "regular" users can only do "GET". Many ways to do this but one way is to make the "role" field String and assign values to it using an ENUM, so that it's easy to change later and only specific terms are used. But you don't have to do that. Write get and set method for the fields you create and that you might need later, and definitely for role.
You need to make sure that class containing the handle(HttpExchange httpExchange) is able to see the currently logged in user, and refer to the User object associated with them. Then you need to modify the method so that
if(loggedInUser.getRole().equals("admin"))
//allow whatever method
else
// allow "GET" or give some denied permission error
Since other implementations have not been provided, I can't give a more detailed answer or be sure that this will work for you.
add a comment |
up vote
0
down vote
I think what you should create an AuthenticationInterceptor
and by-pass GET
the requests there and correspondingly apply authentication mechanism for rest non-GET
requests.
public class AuthenticationInterceptor extends HandlerInterceptorAdapter
@Autowired
private ApiRestBasicAuthentication apiRestBasicAuthentication;
public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) throws Exception
switch (request.getMethod())
case "GET" :
// by-passing all GET requests
return true;
default :
return apiRestBasicAuthentication.checkCredentials(username, password);
add a comment |
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
3
down vote
accepted
A simple way to do it would be to change your
ApiRestBasicAuthentication like:
public class ApiRestBasicAuthentication extends BasicAuthenticator
private UserAuthentication authentication = new UserAuthentication();
public ApiRestBasicAuthentication(String realm)
super(realm);
@Override
public Authenticator.Result authenticate(HttpExchange exch)
Authenticator.Result result=super.authenticate(exch);
if(result instanceof Authenticator.Success)
HttpPrincipal principal=((Authenticator.Success)result).getPrincipal();
String requestMethod=exch.getRequestMethod();
if( ADD SOME LOGIC HERE FOR PRINCIPAL AND REQUEST METHOD)
return new return new Authenticator.Failure(401);
return result;
@Override
public boolean checkCredentials(String user, String pwd)
int authCode = authentication.authenticate(user, pwd);
return authCode == UserAuthentication.USER_AUTHENTICATED;
And add some logic there for requests/users that you want to fail the authenticator. I have shown you here how to get the method in the authenticate method but you need to specify the types of credentials.
Another solution would be if you check the source code of BasicAuthenticator you can see how it implements authenticate
method and you can create your own implementation in a similar way instead of extending BasicAuthenticator and use the get method instead of just the username and password. You can see the source code here and I am sure you will be able to find your way around ;)
Usually in enterprise application you can use some external security management system - for example if you use Spring (the de facto standard in the current java web apps) you can use spring security and do such security patterns and filters in a more declarative way
add a comment |
up vote
3
down vote
accepted
A simple way to do it would be to change your
ApiRestBasicAuthentication like:
public class ApiRestBasicAuthentication extends BasicAuthenticator
private UserAuthentication authentication = new UserAuthentication();
public ApiRestBasicAuthentication(String realm)
super(realm);
@Override
public Authenticator.Result authenticate(HttpExchange exch)
Authenticator.Result result=super.authenticate(exch);
if(result instanceof Authenticator.Success)
HttpPrincipal principal=((Authenticator.Success)result).getPrincipal();
String requestMethod=exch.getRequestMethod();
if( ADD SOME LOGIC HERE FOR PRINCIPAL AND REQUEST METHOD)
return new return new Authenticator.Failure(401);
return result;
@Override
public boolean checkCredentials(String user, String pwd)
int authCode = authentication.authenticate(user, pwd);
return authCode == UserAuthentication.USER_AUTHENTICATED;
And add some logic there for requests/users that you want to fail the authenticator. I have shown you here how to get the method in the authenticate method but you need to specify the types of credentials.
Another solution would be if you check the source code of BasicAuthenticator you can see how it implements authenticate
method and you can create your own implementation in a similar way instead of extending BasicAuthenticator and use the get method instead of just the username and password. You can see the source code here and I am sure you will be able to find your way around ;)
Usually in enterprise application you can use some external security management system - for example if you use Spring (the de facto standard in the current java web apps) you can use spring security and do such security patterns and filters in a more declarative way
add a comment |
up vote
3
down vote
accepted
up vote
3
down vote
accepted
A simple way to do it would be to change your
ApiRestBasicAuthentication like:
public class ApiRestBasicAuthentication extends BasicAuthenticator
private UserAuthentication authentication = new UserAuthentication();
public ApiRestBasicAuthentication(String realm)
super(realm);
@Override
public Authenticator.Result authenticate(HttpExchange exch)
Authenticator.Result result=super.authenticate(exch);
if(result instanceof Authenticator.Success)
HttpPrincipal principal=((Authenticator.Success)result).getPrincipal();
String requestMethod=exch.getRequestMethod();
if( ADD SOME LOGIC HERE FOR PRINCIPAL AND REQUEST METHOD)
return new return new Authenticator.Failure(401);
return result;
@Override
public boolean checkCredentials(String user, String pwd)
int authCode = authentication.authenticate(user, pwd);
return authCode == UserAuthentication.USER_AUTHENTICATED;
And add some logic there for requests/users that you want to fail the authenticator. I have shown you here how to get the method in the authenticate method but you need to specify the types of credentials.
Another solution would be if you check the source code of BasicAuthenticator you can see how it implements authenticate
method and you can create your own implementation in a similar way instead of extending BasicAuthenticator and use the get method instead of just the username and password. You can see the source code here and I am sure you will be able to find your way around ;)
Usually in enterprise application you can use some external security management system - for example if you use Spring (the de facto standard in the current java web apps) you can use spring security and do such security patterns and filters in a more declarative way
A simple way to do it would be to change your
ApiRestBasicAuthentication like:
public class ApiRestBasicAuthentication extends BasicAuthenticator
private UserAuthentication authentication = new UserAuthentication();
public ApiRestBasicAuthentication(String realm)
super(realm);
@Override
public Authenticator.Result authenticate(HttpExchange exch)
Authenticator.Result result=super.authenticate(exch);
if(result instanceof Authenticator.Success)
HttpPrincipal principal=((Authenticator.Success)result).getPrincipal();
String requestMethod=exch.getRequestMethod();
if( ADD SOME LOGIC HERE FOR PRINCIPAL AND REQUEST METHOD)
return new return new Authenticator.Failure(401);
return result;
@Override
public boolean checkCredentials(String user, String pwd)
int authCode = authentication.authenticate(user, pwd);
return authCode == UserAuthentication.USER_AUTHENTICATED;
And add some logic there for requests/users that you want to fail the authenticator. I have shown you here how to get the method in the authenticate method but you need to specify the types of credentials.
Another solution would be if you check the source code of BasicAuthenticator you can see how it implements authenticate
method and you can create your own implementation in a similar way instead of extending BasicAuthenticator and use the get method instead of just the username and password. You can see the source code here and I am sure you will be able to find your way around ;)
Usually in enterprise application you can use some external security management system - for example if you use Spring (the de facto standard in the current java web apps) you can use spring security and do such security patterns and filters in a more declarative way
edited yesterday
answered Nov 9 at 15:05
Veselin Davidov
5,2361414
5,2361414
add a comment |
add a comment |
up vote
0
down vote
There might be many ways to solve this issue. Here is one of my proposal:
Create a User Object with fields that you want and one field called something like "role". Lets say only "admins" are allowed to do make Http requests other than "GET" while "regular" users can only do "GET". Many ways to do this but one way is to make the "role" field String and assign values to it using an ENUM, so that it's easy to change later and only specific terms are used. But you don't have to do that. Write get and set method for the fields you create and that you might need later, and definitely for role.
You need to make sure that class containing the handle(HttpExchange httpExchange) is able to see the currently logged in user, and refer to the User object associated with them. Then you need to modify the method so that
if(loggedInUser.getRole().equals("admin"))
//allow whatever method
else
// allow "GET" or give some denied permission error
Since other implementations have not been provided, I can't give a more detailed answer or be sure that this will work for you.
add a comment |
up vote
0
down vote
There might be many ways to solve this issue. Here is one of my proposal:
Create a User Object with fields that you want and one field called something like "role". Lets say only "admins" are allowed to do make Http requests other than "GET" while "regular" users can only do "GET". Many ways to do this but one way is to make the "role" field String and assign values to it using an ENUM, so that it's easy to change later and only specific terms are used. But you don't have to do that. Write get and set method for the fields you create and that you might need later, and definitely for role.
You need to make sure that class containing the handle(HttpExchange httpExchange) is able to see the currently logged in user, and refer to the User object associated with them. Then you need to modify the method so that
if(loggedInUser.getRole().equals("admin"))
//allow whatever method
else
// allow "GET" or give some denied permission error
Since other implementations have not been provided, I can't give a more detailed answer or be sure that this will work for you.
add a comment |
up vote
0
down vote
up vote
0
down vote
There might be many ways to solve this issue. Here is one of my proposal:
Create a User Object with fields that you want and one field called something like "role". Lets say only "admins" are allowed to do make Http requests other than "GET" while "regular" users can only do "GET". Many ways to do this but one way is to make the "role" field String and assign values to it using an ENUM, so that it's easy to change later and only specific terms are used. But you don't have to do that. Write get and set method for the fields you create and that you might need later, and definitely for role.
You need to make sure that class containing the handle(HttpExchange httpExchange) is able to see the currently logged in user, and refer to the User object associated with them. Then you need to modify the method so that
if(loggedInUser.getRole().equals("admin"))
//allow whatever method
else
// allow "GET" or give some denied permission error
Since other implementations have not been provided, I can't give a more detailed answer or be sure that this will work for you.
There might be many ways to solve this issue. Here is one of my proposal:
Create a User Object with fields that you want and one field called something like "role". Lets say only "admins" are allowed to do make Http requests other than "GET" while "regular" users can only do "GET". Many ways to do this but one way is to make the "role" field String and assign values to it using an ENUM, so that it's easy to change later and only specific terms are used. But you don't have to do that. Write get and set method for the fields you create and that you might need later, and definitely for role.
You need to make sure that class containing the handle(HttpExchange httpExchange) is able to see the currently logged in user, and refer to the User object associated with them. Then you need to modify the method so that
if(loggedInUser.getRole().equals("admin"))
//allow whatever method
else
// allow "GET" or give some denied permission error
Since other implementations have not been provided, I can't give a more detailed answer or be sure that this will work for you.
edited Nov 9 at 14:49
Tezra
4,65021038
4,65021038
answered Nov 9 at 14:07
Chief Nish
758
758
add a comment |
add a comment |
up vote
0
down vote
I think what you should create an AuthenticationInterceptor
and by-pass GET
the requests there and correspondingly apply authentication mechanism for rest non-GET
requests.
public class AuthenticationInterceptor extends HandlerInterceptorAdapter
@Autowired
private ApiRestBasicAuthentication apiRestBasicAuthentication;
public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) throws Exception
switch (request.getMethod())
case "GET" :
// by-passing all GET requests
return true;
default :
return apiRestBasicAuthentication.checkCredentials(username, password);
add a comment |
up vote
0
down vote
I think what you should create an AuthenticationInterceptor
and by-pass GET
the requests there and correspondingly apply authentication mechanism for rest non-GET
requests.
public class AuthenticationInterceptor extends HandlerInterceptorAdapter
@Autowired
private ApiRestBasicAuthentication apiRestBasicAuthentication;
public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) throws Exception
switch (request.getMethod())
case "GET" :
// by-passing all GET requests
return true;
default :
return apiRestBasicAuthentication.checkCredentials(username, password);
add a comment |
up vote
0
down vote
up vote
0
down vote
I think what you should create an AuthenticationInterceptor
and by-pass GET
the requests there and correspondingly apply authentication mechanism for rest non-GET
requests.
public class AuthenticationInterceptor extends HandlerInterceptorAdapter
@Autowired
private ApiRestBasicAuthentication apiRestBasicAuthentication;
public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) throws Exception
switch (request.getMethod())
case "GET" :
// by-passing all GET requests
return true;
default :
return apiRestBasicAuthentication.checkCredentials(username, password);
I think what you should create an AuthenticationInterceptor
and by-pass GET
the requests there and correspondingly apply authentication mechanism for rest non-GET
requests.
public class AuthenticationInterceptor extends HandlerInterceptorAdapter
@Autowired
private ApiRestBasicAuthentication apiRestBasicAuthentication;
public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) throws Exception
switch (request.getMethod())
case "GET" :
// by-passing all GET requests
return true;
default :
return apiRestBasicAuthentication.checkCredentials(username, password);
answered Nov 9 at 15:22


swayamraina
448410
448410
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f41407510%2fjava-httpserver-basic-authentication-for-different-request-methods%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
BUCeOgd5YX NNX,yPw,XXYXprDFituCzfo K4utm3O
I suppose you'll need some authorisation aspect (or similiar functionality given by filter or interceptor) that will only respond to non-GETs if user is authorised, though I cannot help you right now with full answer. Figure out which of those (aspect, filter, interceptor) you have available and read about it.
– Filip Malczak
Dec 31 '16 at 11:16
That could work because I can check the method (POST, GET, PUT,...) in the filter, but then how can I get the username sent in the request inside the filter? My ApiRestBasicAuthentication has a checkCredentials() function which receives the user and password, but in the Filter I just have the httpExchange object, and the username/password is encripted.
– David
Dec 31 '16 at 17:31
I suggest you to use
spring-security
for that. Take a lookt at the following answer: stackoverflow.com/a/45965232/540286– Ortwin Angermeier
Nov 9 at 15:30