Have to onPress twice to increase this.state.count - React Native
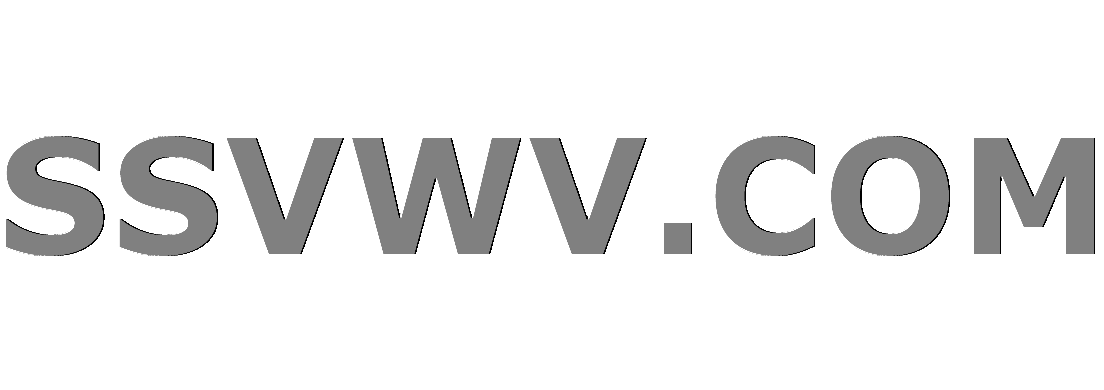
Multi tool use
up vote
1
down vote
favorite
first question ever!
I have the file on Expo - https://snack.expo.io/@pssoor/Y3JhYm
The problem I have is that if you answer a multiplication question correctly, I have to press it again before the accumulator works. Everything else is functioning OK.
The idea behind the code is to select a number from a modal, which makes an array of numbers up to that particular number. The array is then shuffled. A question is made ( ? * accumulator = total) which the user answers.
Hope someone can help!
Thanks
Here's the whole snack (apologies for the mess!)
const arr =
export default class NumberBonds extends React.Component
constructor()
super()
this.state =
accumulator: '',
count: 0,
number: '',
total: '',
// Modal Functions
setModalVisible(visible)
this.setState( modalVisible: !visible )
renderArray(val)
for (let i = 1; i <= val; i++) arr.push(i) let i = 0; let j = 0; let temp = null; for (i = arr.length - 1; i > 0; i -= 1) j = Math.floor(Math.random() * (i + 1)); temp = arr[i]; arr[i] = arr[j]; arr[j] = temp;
this.setModalVisible(!this.state.modalVisible)
this.setState(
accumulator: arr[this.state.count],
)
return arr
modalPassValue(value)
this.setState(
total: this.state.total + value
)
// Main Functions
customAlertCorrect()
Alert.alert(
'Correct',
'Well Done!',
[
text: 'Next',
onPress: () => this.setState(prevState => (count: prevState.count + 1))
]
)
customAlertDone()
Alert.alert('Fantastic', 'All Done!')
customAlertWrong()
Alert.alert('Not Quite', 'Try Again!')
check()
// If numbers matching, say correct. Move on. If not matching, say incorrect. Move on. If matching and then no more, STOP
if (parseInt(this.state.number) + this.state.accumulator === parseInt(this.state.total))
this.setState(
number: '',
accumulator: arr[this.state.count]
)
this.customAlertCorrect()
else
this.customAlertWrong()
this.setState(
number: '',
)
if (parseInt(this.state.count) === parseInt(this.state.total))
this.customAlertDone()
render()
return (
<View style=styles.container>
<View style= marginTop: 100, marginLeft: 100 >
<Modal animationType="slide" transparent=false visible=this.state.modalVisible>
<View>
<View style= marginTop: 50, >
<Text style= fontSize: 50, fontFamily: 'hobostd', >Enter your Number Bond</Text>
<Text style= fontSize: 50, backgroundColor: 'pink', height: 200, color: 'white' >this.state.total</Text>
</View>
</View>
<View style= flex: 1, backgroundColor: 'white', flexDirection: 'row', flexWrap: 'wrap', justifyContent: 'center', alignItems: 'center' >
<View style= backgroundColor: 'white', flexDirection: 'row', flexWrap: 'wrap', justifyContent: 'flex-start' >
<TouchableOpacity onPress=() => this.modalPassValue(1) style=styles.buttons><Text style=styles.buttonsText>1</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.modalPassValue(2) style=styles.buttons><Text style=styles.buttonsText>2</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.modalPassValue(3) style=styles.buttons><Text style=styles.buttonsText>3</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.modalPassValue(4) style=styles.buttons><Text style=styles.buttonsText>4</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.modalPassValue(5) style=styles.buttons><Text style=styles.buttonsText>5</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.modalPassValue(6) style=styles.buttons><Text style=styles.buttonsText>6</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.modalPassValue(7) style=styles.buttons><Text style=styles.buttonsText>7</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.modalPassValue(8) style=styles.buttons><Text style=styles.buttonsText>8</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.modalPassValue(9) style=styles.buttons><Text style=styles.buttonsText>9</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.setState( total: '' ) style=styles.buttons><Text style=styles.buttonsText>Del</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.modalPassValue(0) style=styles.buttons><Text style=styles.buttonsText>0</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.renderArray(this.state.total) style=styles.buttons><Text style=styles.buttonsText>Done</Text></TouchableOpacity>
</View>
</View>
</Modal>
</View>
<View style= marginBottom: 20 ><Text style= fontSize: 50, textAlign: 'center' >Accumulator: this.state.accumulator</Text></View>
<View style= marginBottom: 20, flexDirection: 'row', justifyContent: 'space-around' >
<View style= backgroundColor: 'red', height: 70, width: 100, borderRadius: 20, ><Text style= fontSize: 50, textAlign: 'center', >this.state.accumulator</Text></View>
<Text style= fontSize: 50, textAlign: 'center', height: 50, width: 40, >+</Text>
<View style= backgroundColor: 'yellow', height: 70, width: 100, borderRadius: 20, ><Text style= fontSize: 50, textAlign: 'center', >this.state.number</Text></View>
<Text style= fontSize: 50, textAlign: 'center', height: 50, width: 40, >=</Text>
<View style= backgroundColor: 'orange', height: 70, width: 100, borderRadius: 20, ><Text style= fontSize: 50, textAlign: 'center', >this.state.total</Text></View>
</View>
<View style= flex: 1, backgroundColor: 'white', flexDirection: 'row', flexWrap: 'wrap', justifyContent: 'center', alignItems: 'center' >
<View style= backgroundColor: 'white', flexDirection: 'row', flexWrap: 'wrap', justifyContent: 'center' >
<TouchableOpacity onPress=() => this.setState( number: this.state.number + 1 ) style=styles.buttons><Text style=styles.buttonsText>1</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.setState( number: this.state.number + 2 ) style=styles.buttons><Text style=styles.buttonsText>2</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.setState( number: this.state.number + 3 ) style=styles.buttons><Text style=styles.buttonsText>3</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.setState( number: this.state.number + 4 ) style=styles.buttons><Text style=styles.buttonsText>4</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.setState( number: this.state.number + 5 ) style=styles.buttons><Text style=styles.buttonsText>5</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.setState( number: this.state.number + 6 ) style=styles.buttons><Text style=styles.buttonsText>6</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.setState( number: this.state.number + 7 ) style=styles.buttons><Text style=styles.buttonsText>7</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.setState( number: this.state.number + 8 ) style=styles.buttons><Text style=styles.buttonsText>8</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.setState( number: this.state.number + 9 ) style=styles.buttons><Text style=styles.buttonsText>9</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.setState( number: '' ) style=styles.buttons><Text style=styles.buttonsText>Del</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.setState( number: this.state.number + 0 ) style=styles.buttons><Text style=styles.buttonsText>0</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.check() style=styles.buttons><Text style=styles.buttonsText>Check</Text></TouchableOpacity>
</View>
</View>
</View>
);
reactjs react-native
add a comment |
up vote
1
down vote
favorite
first question ever!
I have the file on Expo - https://snack.expo.io/@pssoor/Y3JhYm
The problem I have is that if you answer a multiplication question correctly, I have to press it again before the accumulator works. Everything else is functioning OK.
The idea behind the code is to select a number from a modal, which makes an array of numbers up to that particular number. The array is then shuffled. A question is made ( ? * accumulator = total) which the user answers.
Hope someone can help!
Thanks
Here's the whole snack (apologies for the mess!)
const arr =
export default class NumberBonds extends React.Component
constructor()
super()
this.state =
accumulator: '',
count: 0,
number: '',
total: '',
// Modal Functions
setModalVisible(visible)
this.setState( modalVisible: !visible )
renderArray(val)
for (let i = 1; i <= val; i++) arr.push(i) let i = 0; let j = 0; let temp = null; for (i = arr.length - 1; i > 0; i -= 1) j = Math.floor(Math.random() * (i + 1)); temp = arr[i]; arr[i] = arr[j]; arr[j] = temp;
this.setModalVisible(!this.state.modalVisible)
this.setState(
accumulator: arr[this.state.count],
)
return arr
modalPassValue(value)
this.setState(
total: this.state.total + value
)
// Main Functions
customAlertCorrect()
Alert.alert(
'Correct',
'Well Done!',
[
text: 'Next',
onPress: () => this.setState(prevState => (count: prevState.count + 1))
]
)
customAlertDone()
Alert.alert('Fantastic', 'All Done!')
customAlertWrong()
Alert.alert('Not Quite', 'Try Again!')
check()
// If numbers matching, say correct. Move on. If not matching, say incorrect. Move on. If matching and then no more, STOP
if (parseInt(this.state.number) + this.state.accumulator === parseInt(this.state.total))
this.setState(
number: '',
accumulator: arr[this.state.count]
)
this.customAlertCorrect()
else
this.customAlertWrong()
this.setState(
number: '',
)
if (parseInt(this.state.count) === parseInt(this.state.total))
this.customAlertDone()
render()
return (
<View style=styles.container>
<View style= marginTop: 100, marginLeft: 100 >
<Modal animationType="slide" transparent=false visible=this.state.modalVisible>
<View>
<View style= marginTop: 50, >
<Text style= fontSize: 50, fontFamily: 'hobostd', >Enter your Number Bond</Text>
<Text style= fontSize: 50, backgroundColor: 'pink', height: 200, color: 'white' >this.state.total</Text>
</View>
</View>
<View style= flex: 1, backgroundColor: 'white', flexDirection: 'row', flexWrap: 'wrap', justifyContent: 'center', alignItems: 'center' >
<View style= backgroundColor: 'white', flexDirection: 'row', flexWrap: 'wrap', justifyContent: 'flex-start' >
<TouchableOpacity onPress=() => this.modalPassValue(1) style=styles.buttons><Text style=styles.buttonsText>1</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.modalPassValue(2) style=styles.buttons><Text style=styles.buttonsText>2</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.modalPassValue(3) style=styles.buttons><Text style=styles.buttonsText>3</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.modalPassValue(4) style=styles.buttons><Text style=styles.buttonsText>4</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.modalPassValue(5) style=styles.buttons><Text style=styles.buttonsText>5</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.modalPassValue(6) style=styles.buttons><Text style=styles.buttonsText>6</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.modalPassValue(7) style=styles.buttons><Text style=styles.buttonsText>7</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.modalPassValue(8) style=styles.buttons><Text style=styles.buttonsText>8</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.modalPassValue(9) style=styles.buttons><Text style=styles.buttonsText>9</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.setState( total: '' ) style=styles.buttons><Text style=styles.buttonsText>Del</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.modalPassValue(0) style=styles.buttons><Text style=styles.buttonsText>0</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.renderArray(this.state.total) style=styles.buttons><Text style=styles.buttonsText>Done</Text></TouchableOpacity>
</View>
</View>
</Modal>
</View>
<View style= marginBottom: 20 ><Text style= fontSize: 50, textAlign: 'center' >Accumulator: this.state.accumulator</Text></View>
<View style= marginBottom: 20, flexDirection: 'row', justifyContent: 'space-around' >
<View style= backgroundColor: 'red', height: 70, width: 100, borderRadius: 20, ><Text style= fontSize: 50, textAlign: 'center', >this.state.accumulator</Text></View>
<Text style= fontSize: 50, textAlign: 'center', height: 50, width: 40, >+</Text>
<View style= backgroundColor: 'yellow', height: 70, width: 100, borderRadius: 20, ><Text style= fontSize: 50, textAlign: 'center', >this.state.number</Text></View>
<Text style= fontSize: 50, textAlign: 'center', height: 50, width: 40, >=</Text>
<View style= backgroundColor: 'orange', height: 70, width: 100, borderRadius: 20, ><Text style= fontSize: 50, textAlign: 'center', >this.state.total</Text></View>
</View>
<View style= flex: 1, backgroundColor: 'white', flexDirection: 'row', flexWrap: 'wrap', justifyContent: 'center', alignItems: 'center' >
<View style= backgroundColor: 'white', flexDirection: 'row', flexWrap: 'wrap', justifyContent: 'center' >
<TouchableOpacity onPress=() => this.setState( number: this.state.number + 1 ) style=styles.buttons><Text style=styles.buttonsText>1</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.setState( number: this.state.number + 2 ) style=styles.buttons><Text style=styles.buttonsText>2</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.setState( number: this.state.number + 3 ) style=styles.buttons><Text style=styles.buttonsText>3</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.setState( number: this.state.number + 4 ) style=styles.buttons><Text style=styles.buttonsText>4</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.setState( number: this.state.number + 5 ) style=styles.buttons><Text style=styles.buttonsText>5</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.setState( number: this.state.number + 6 ) style=styles.buttons><Text style=styles.buttonsText>6</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.setState( number: this.state.number + 7 ) style=styles.buttons><Text style=styles.buttonsText>7</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.setState( number: this.state.number + 8 ) style=styles.buttons><Text style=styles.buttonsText>8</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.setState( number: this.state.number + 9 ) style=styles.buttons><Text style=styles.buttonsText>9</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.setState( number: '' ) style=styles.buttons><Text style=styles.buttonsText>Del</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.setState( number: this.state.number + 0 ) style=styles.buttons><Text style=styles.buttonsText>0</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.check() style=styles.buttons><Text style=styles.buttonsText>Check</Text></TouchableOpacity>
</View>
</View>
</View>
);
reactjs react-native
Just to mention, I couldn't fix it using the setState (or updater) methods. I concluded that the issue is the asynchronous nature of state. Installed mobx and it worked right away! Problem solved!
– Pav Soor
Nov 12 at 10:37
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
first question ever!
I have the file on Expo - https://snack.expo.io/@pssoor/Y3JhYm
The problem I have is that if you answer a multiplication question correctly, I have to press it again before the accumulator works. Everything else is functioning OK.
The idea behind the code is to select a number from a modal, which makes an array of numbers up to that particular number. The array is then shuffled. A question is made ( ? * accumulator = total) which the user answers.
Hope someone can help!
Thanks
Here's the whole snack (apologies for the mess!)
const arr =
export default class NumberBonds extends React.Component
constructor()
super()
this.state =
accumulator: '',
count: 0,
number: '',
total: '',
// Modal Functions
setModalVisible(visible)
this.setState( modalVisible: !visible )
renderArray(val)
for (let i = 1; i <= val; i++) arr.push(i) let i = 0; let j = 0; let temp = null; for (i = arr.length - 1; i > 0; i -= 1) j = Math.floor(Math.random() * (i + 1)); temp = arr[i]; arr[i] = arr[j]; arr[j] = temp;
this.setModalVisible(!this.state.modalVisible)
this.setState(
accumulator: arr[this.state.count],
)
return arr
modalPassValue(value)
this.setState(
total: this.state.total + value
)
// Main Functions
customAlertCorrect()
Alert.alert(
'Correct',
'Well Done!',
[
text: 'Next',
onPress: () => this.setState(prevState => (count: prevState.count + 1))
]
)
customAlertDone()
Alert.alert('Fantastic', 'All Done!')
customAlertWrong()
Alert.alert('Not Quite', 'Try Again!')
check()
// If numbers matching, say correct. Move on. If not matching, say incorrect. Move on. If matching and then no more, STOP
if (parseInt(this.state.number) + this.state.accumulator === parseInt(this.state.total))
this.setState(
number: '',
accumulator: arr[this.state.count]
)
this.customAlertCorrect()
else
this.customAlertWrong()
this.setState(
number: '',
)
if (parseInt(this.state.count) === parseInt(this.state.total))
this.customAlertDone()
render()
return (
<View style=styles.container>
<View style= marginTop: 100, marginLeft: 100 >
<Modal animationType="slide" transparent=false visible=this.state.modalVisible>
<View>
<View style= marginTop: 50, >
<Text style= fontSize: 50, fontFamily: 'hobostd', >Enter your Number Bond</Text>
<Text style= fontSize: 50, backgroundColor: 'pink', height: 200, color: 'white' >this.state.total</Text>
</View>
</View>
<View style= flex: 1, backgroundColor: 'white', flexDirection: 'row', flexWrap: 'wrap', justifyContent: 'center', alignItems: 'center' >
<View style= backgroundColor: 'white', flexDirection: 'row', flexWrap: 'wrap', justifyContent: 'flex-start' >
<TouchableOpacity onPress=() => this.modalPassValue(1) style=styles.buttons><Text style=styles.buttonsText>1</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.modalPassValue(2) style=styles.buttons><Text style=styles.buttonsText>2</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.modalPassValue(3) style=styles.buttons><Text style=styles.buttonsText>3</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.modalPassValue(4) style=styles.buttons><Text style=styles.buttonsText>4</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.modalPassValue(5) style=styles.buttons><Text style=styles.buttonsText>5</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.modalPassValue(6) style=styles.buttons><Text style=styles.buttonsText>6</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.modalPassValue(7) style=styles.buttons><Text style=styles.buttonsText>7</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.modalPassValue(8) style=styles.buttons><Text style=styles.buttonsText>8</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.modalPassValue(9) style=styles.buttons><Text style=styles.buttonsText>9</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.setState( total: '' ) style=styles.buttons><Text style=styles.buttonsText>Del</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.modalPassValue(0) style=styles.buttons><Text style=styles.buttonsText>0</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.renderArray(this.state.total) style=styles.buttons><Text style=styles.buttonsText>Done</Text></TouchableOpacity>
</View>
</View>
</Modal>
</View>
<View style= marginBottom: 20 ><Text style= fontSize: 50, textAlign: 'center' >Accumulator: this.state.accumulator</Text></View>
<View style= marginBottom: 20, flexDirection: 'row', justifyContent: 'space-around' >
<View style= backgroundColor: 'red', height: 70, width: 100, borderRadius: 20, ><Text style= fontSize: 50, textAlign: 'center', >this.state.accumulator</Text></View>
<Text style= fontSize: 50, textAlign: 'center', height: 50, width: 40, >+</Text>
<View style= backgroundColor: 'yellow', height: 70, width: 100, borderRadius: 20, ><Text style= fontSize: 50, textAlign: 'center', >this.state.number</Text></View>
<Text style= fontSize: 50, textAlign: 'center', height: 50, width: 40, >=</Text>
<View style= backgroundColor: 'orange', height: 70, width: 100, borderRadius: 20, ><Text style= fontSize: 50, textAlign: 'center', >this.state.total</Text></View>
</View>
<View style= flex: 1, backgroundColor: 'white', flexDirection: 'row', flexWrap: 'wrap', justifyContent: 'center', alignItems: 'center' >
<View style= backgroundColor: 'white', flexDirection: 'row', flexWrap: 'wrap', justifyContent: 'center' >
<TouchableOpacity onPress=() => this.setState( number: this.state.number + 1 ) style=styles.buttons><Text style=styles.buttonsText>1</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.setState( number: this.state.number + 2 ) style=styles.buttons><Text style=styles.buttonsText>2</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.setState( number: this.state.number + 3 ) style=styles.buttons><Text style=styles.buttonsText>3</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.setState( number: this.state.number + 4 ) style=styles.buttons><Text style=styles.buttonsText>4</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.setState( number: this.state.number + 5 ) style=styles.buttons><Text style=styles.buttonsText>5</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.setState( number: this.state.number + 6 ) style=styles.buttons><Text style=styles.buttonsText>6</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.setState( number: this.state.number + 7 ) style=styles.buttons><Text style=styles.buttonsText>7</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.setState( number: this.state.number + 8 ) style=styles.buttons><Text style=styles.buttonsText>8</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.setState( number: this.state.number + 9 ) style=styles.buttons><Text style=styles.buttonsText>9</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.setState( number: '' ) style=styles.buttons><Text style=styles.buttonsText>Del</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.setState( number: this.state.number + 0 ) style=styles.buttons><Text style=styles.buttonsText>0</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.check() style=styles.buttons><Text style=styles.buttonsText>Check</Text></TouchableOpacity>
</View>
</View>
</View>
);
reactjs react-native
first question ever!
I have the file on Expo - https://snack.expo.io/@pssoor/Y3JhYm
The problem I have is that if you answer a multiplication question correctly, I have to press it again before the accumulator works. Everything else is functioning OK.
The idea behind the code is to select a number from a modal, which makes an array of numbers up to that particular number. The array is then shuffled. A question is made ( ? * accumulator = total) which the user answers.
Hope someone can help!
Thanks
Here's the whole snack (apologies for the mess!)
const arr =
export default class NumberBonds extends React.Component
constructor()
super()
this.state =
accumulator: '',
count: 0,
number: '',
total: '',
// Modal Functions
setModalVisible(visible)
this.setState( modalVisible: !visible )
renderArray(val)
for (let i = 1; i <= val; i++) arr.push(i) let i = 0; let j = 0; let temp = null; for (i = arr.length - 1; i > 0; i -= 1) j = Math.floor(Math.random() * (i + 1)); temp = arr[i]; arr[i] = arr[j]; arr[j] = temp;
this.setModalVisible(!this.state.modalVisible)
this.setState(
accumulator: arr[this.state.count],
)
return arr
modalPassValue(value)
this.setState(
total: this.state.total + value
)
// Main Functions
customAlertCorrect()
Alert.alert(
'Correct',
'Well Done!',
[
text: 'Next',
onPress: () => this.setState(prevState => (count: prevState.count + 1))
]
)
customAlertDone()
Alert.alert('Fantastic', 'All Done!')
customAlertWrong()
Alert.alert('Not Quite', 'Try Again!')
check()
// If numbers matching, say correct. Move on. If not matching, say incorrect. Move on. If matching and then no more, STOP
if (parseInt(this.state.number) + this.state.accumulator === parseInt(this.state.total))
this.setState(
number: '',
accumulator: arr[this.state.count]
)
this.customAlertCorrect()
else
this.customAlertWrong()
this.setState(
number: '',
)
if (parseInt(this.state.count) === parseInt(this.state.total))
this.customAlertDone()
render()
return (
<View style=styles.container>
<View style= marginTop: 100, marginLeft: 100 >
<Modal animationType="slide" transparent=false visible=this.state.modalVisible>
<View>
<View style= marginTop: 50, >
<Text style= fontSize: 50, fontFamily: 'hobostd', >Enter your Number Bond</Text>
<Text style= fontSize: 50, backgroundColor: 'pink', height: 200, color: 'white' >this.state.total</Text>
</View>
</View>
<View style= flex: 1, backgroundColor: 'white', flexDirection: 'row', flexWrap: 'wrap', justifyContent: 'center', alignItems: 'center' >
<View style= backgroundColor: 'white', flexDirection: 'row', flexWrap: 'wrap', justifyContent: 'flex-start' >
<TouchableOpacity onPress=() => this.modalPassValue(1) style=styles.buttons><Text style=styles.buttonsText>1</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.modalPassValue(2) style=styles.buttons><Text style=styles.buttonsText>2</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.modalPassValue(3) style=styles.buttons><Text style=styles.buttonsText>3</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.modalPassValue(4) style=styles.buttons><Text style=styles.buttonsText>4</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.modalPassValue(5) style=styles.buttons><Text style=styles.buttonsText>5</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.modalPassValue(6) style=styles.buttons><Text style=styles.buttonsText>6</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.modalPassValue(7) style=styles.buttons><Text style=styles.buttonsText>7</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.modalPassValue(8) style=styles.buttons><Text style=styles.buttonsText>8</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.modalPassValue(9) style=styles.buttons><Text style=styles.buttonsText>9</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.setState( total: '' ) style=styles.buttons><Text style=styles.buttonsText>Del</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.modalPassValue(0) style=styles.buttons><Text style=styles.buttonsText>0</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.renderArray(this.state.total) style=styles.buttons><Text style=styles.buttonsText>Done</Text></TouchableOpacity>
</View>
</View>
</Modal>
</View>
<View style= marginBottom: 20 ><Text style= fontSize: 50, textAlign: 'center' >Accumulator: this.state.accumulator</Text></View>
<View style= marginBottom: 20, flexDirection: 'row', justifyContent: 'space-around' >
<View style= backgroundColor: 'red', height: 70, width: 100, borderRadius: 20, ><Text style= fontSize: 50, textAlign: 'center', >this.state.accumulator</Text></View>
<Text style= fontSize: 50, textAlign: 'center', height: 50, width: 40, >+</Text>
<View style= backgroundColor: 'yellow', height: 70, width: 100, borderRadius: 20, ><Text style= fontSize: 50, textAlign: 'center', >this.state.number</Text></View>
<Text style= fontSize: 50, textAlign: 'center', height: 50, width: 40, >=</Text>
<View style= backgroundColor: 'orange', height: 70, width: 100, borderRadius: 20, ><Text style= fontSize: 50, textAlign: 'center', >this.state.total</Text></View>
</View>
<View style= flex: 1, backgroundColor: 'white', flexDirection: 'row', flexWrap: 'wrap', justifyContent: 'center', alignItems: 'center' >
<View style= backgroundColor: 'white', flexDirection: 'row', flexWrap: 'wrap', justifyContent: 'center' >
<TouchableOpacity onPress=() => this.setState( number: this.state.number + 1 ) style=styles.buttons><Text style=styles.buttonsText>1</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.setState( number: this.state.number + 2 ) style=styles.buttons><Text style=styles.buttonsText>2</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.setState( number: this.state.number + 3 ) style=styles.buttons><Text style=styles.buttonsText>3</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.setState( number: this.state.number + 4 ) style=styles.buttons><Text style=styles.buttonsText>4</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.setState( number: this.state.number + 5 ) style=styles.buttons><Text style=styles.buttonsText>5</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.setState( number: this.state.number + 6 ) style=styles.buttons><Text style=styles.buttonsText>6</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.setState( number: this.state.number + 7 ) style=styles.buttons><Text style=styles.buttonsText>7</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.setState( number: this.state.number + 8 ) style=styles.buttons><Text style=styles.buttonsText>8</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.setState( number: this.state.number + 9 ) style=styles.buttons><Text style=styles.buttonsText>9</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.setState( number: '' ) style=styles.buttons><Text style=styles.buttonsText>Del</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.setState( number: this.state.number + 0 ) style=styles.buttons><Text style=styles.buttonsText>0</Text></TouchableOpacity>
<TouchableOpacity onPress=() => this.check() style=styles.buttons><Text style=styles.buttonsText>Check</Text></TouchableOpacity>
</View>
</View>
</View>
);
reactjs react-native
reactjs react-native
edited Nov 9 at 18:02


Prasun
2,2642813
2,2642813
asked Nov 9 at 17:48


Pav Soor
61
61
Just to mention, I couldn't fix it using the setState (or updater) methods. I concluded that the issue is the asynchronous nature of state. Installed mobx and it worked right away! Problem solved!
– Pav Soor
Nov 12 at 10:37
add a comment |
Just to mention, I couldn't fix it using the setState (or updater) methods. I concluded that the issue is the asynchronous nature of state. Installed mobx and it worked right away! Problem solved!
– Pav Soor
Nov 12 at 10:37
Just to mention, I couldn't fix it using the setState (or updater) methods. I concluded that the issue is the asynchronous nature of state. Installed mobx and it worked right away! Problem solved!
– Pav Soor
Nov 12 at 10:37
Just to mention, I couldn't fix it using the setState (or updater) methods. I concluded that the issue is the asynchronous nature of state. Installed mobx and it worked right away! Problem solved!
– Pav Soor
Nov 12 at 10:37
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53230901%2fhave-to-onpress-twice-to-increase-this-state-count-react-native%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
RQ3sZ4n18oZv77RffpEMjK9OhEOBzOIRVpxfA zA,KgoORqpvV2TnZnhdpi7zo CQ91iC8QnoSqN5GWe
Just to mention, I couldn't fix it using the setState (or updater) methods. I concluded that the issue is the asynchronous nature of state. Installed mobx and it worked right away! Problem solved!
– Pav Soor
Nov 12 at 10:37