Using external abstract class in Typescript without extending it
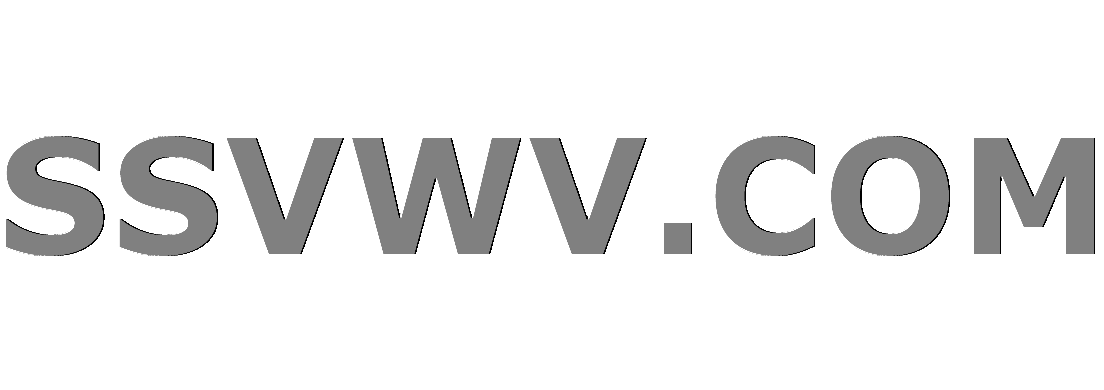
Multi tool use
up vote
1
down vote
favorite
I've got one module called utils.tsx
so that:
interface IUtils
toUri: (route: string) => string
export default abstract class Utils implements IUtils
public toUri = (route: string) =>
return route
and another file where I wish to use this utils module:
import Utils from '../helpers/utils'
class Router
private getBuilder = (search: string) =>
const path = Utils.toUri(search)
When I am trying to use Utils.toUri
I am getting TS error:
[ts] Property 'toUri' does not exist on type 'typeof Utils'.
My intention is to call the external abstract class function without extending the Router
class nor inheriting from it (as I will have more than one external module in the main file).
Could someone help me to bypass it and understand?
PS: I tried with public abstract toUri()
too. Perhaps I mixed up the routines from other programming languages and confusing usage of static with abstract here...
typescript oop typescript-typings
add a comment |
up vote
1
down vote
favorite
I've got one module called utils.tsx
so that:
interface IUtils
toUri: (route: string) => string
export default abstract class Utils implements IUtils
public toUri = (route: string) =>
return route
and another file where I wish to use this utils module:
import Utils from '../helpers/utils'
class Router
private getBuilder = (search: string) =>
const path = Utils.toUri(search)
When I am trying to use Utils.toUri
I am getting TS error:
[ts] Property 'toUri' does not exist on type 'typeof Utils'.
My intention is to call the external abstract class function without extending the Router
class nor inheriting from it (as I will have more than one external module in the main file).
Could someone help me to bypass it and understand?
PS: I tried with public abstract toUri()
too. Perhaps I mixed up the routines from other programming languages and confusing usage of static with abstract here...
typescript oop typescript-typings
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I've got one module called utils.tsx
so that:
interface IUtils
toUri: (route: string) => string
export default abstract class Utils implements IUtils
public toUri = (route: string) =>
return route
and another file where I wish to use this utils module:
import Utils from '../helpers/utils'
class Router
private getBuilder = (search: string) =>
const path = Utils.toUri(search)
When I am trying to use Utils.toUri
I am getting TS error:
[ts] Property 'toUri' does not exist on type 'typeof Utils'.
My intention is to call the external abstract class function without extending the Router
class nor inheriting from it (as I will have more than one external module in the main file).
Could someone help me to bypass it and understand?
PS: I tried with public abstract toUri()
too. Perhaps I mixed up the routines from other programming languages and confusing usage of static with abstract here...
typescript oop typescript-typings
I've got one module called utils.tsx
so that:
interface IUtils
toUri: (route: string) => string
export default abstract class Utils implements IUtils
public toUri = (route: string) =>
return route
and another file where I wish to use this utils module:
import Utils from '../helpers/utils'
class Router
private getBuilder = (search: string) =>
const path = Utils.toUri(search)
When I am trying to use Utils.toUri
I am getting TS error:
[ts] Property 'toUri' does not exist on type 'typeof Utils'.
My intention is to call the external abstract class function without extending the Router
class nor inheriting from it (as I will have more than one external module in the main file).
Could someone help me to bypass it and understand?
PS: I tried with public abstract toUri()
too. Perhaps I mixed up the routines from other programming languages and confusing usage of static with abstract here...
typescript oop typescript-typings
typescript oop typescript-typings
asked Nov 9 at 17:15


Greg Woz
144111
144111
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
accepted
You don't want Utils
to implement IUtils
, since Utils
is the instance type of the class. It looks like you want the Utils
constructor (whose type is typeof Utils
) to implement IUtils
. That is, you want toUri
to be a static method of the Utils
class. Like this:
abstract class Utils
public static toUri = (route: string) =>
return route
There is no way to annotate the class Utils
declaration to say that the static side of the class implements IUtils
. Luckily, the Utils
constructor will automatically be seen as something that implements IUtils
without needing to write implements IUtils
anywhere. Thanks, structural typing:
declare function takeIUtils(iUtils: IUtils): void;
takeIUtils(Utils); // works anyway
This should allow your Router
class to behave as desired.
Side note, I wonder if you really want Utils
to be a class at all. If you never want to see an instance of Utils
like class X extends Utils ...; new X()
, then you're probably making life unnecessarily hard for yourself. Maybe it should just be a value:
export const Utils : IUtils =
toUri(route: string)
return route
Or a namespace
:
export namespace Utils
export function toUri(route: string)
return route
Or a module, or something.
Anyway, hope that helps. Good luck!
Yes, definitelynamespace
is a solution to my problems. Thank you. :)
– Greg Woz
Nov 12 at 21:09
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
You don't want Utils
to implement IUtils
, since Utils
is the instance type of the class. It looks like you want the Utils
constructor (whose type is typeof Utils
) to implement IUtils
. That is, you want toUri
to be a static method of the Utils
class. Like this:
abstract class Utils
public static toUri = (route: string) =>
return route
There is no way to annotate the class Utils
declaration to say that the static side of the class implements IUtils
. Luckily, the Utils
constructor will automatically be seen as something that implements IUtils
without needing to write implements IUtils
anywhere. Thanks, structural typing:
declare function takeIUtils(iUtils: IUtils): void;
takeIUtils(Utils); // works anyway
This should allow your Router
class to behave as desired.
Side note, I wonder if you really want Utils
to be a class at all. If you never want to see an instance of Utils
like class X extends Utils ...; new X()
, then you're probably making life unnecessarily hard for yourself. Maybe it should just be a value:
export const Utils : IUtils =
toUri(route: string)
return route
Or a namespace
:
export namespace Utils
export function toUri(route: string)
return route
Or a module, or something.
Anyway, hope that helps. Good luck!
Yes, definitelynamespace
is a solution to my problems. Thank you. :)
– Greg Woz
Nov 12 at 21:09
add a comment |
up vote
1
down vote
accepted
You don't want Utils
to implement IUtils
, since Utils
is the instance type of the class. It looks like you want the Utils
constructor (whose type is typeof Utils
) to implement IUtils
. That is, you want toUri
to be a static method of the Utils
class. Like this:
abstract class Utils
public static toUri = (route: string) =>
return route
There is no way to annotate the class Utils
declaration to say that the static side of the class implements IUtils
. Luckily, the Utils
constructor will automatically be seen as something that implements IUtils
without needing to write implements IUtils
anywhere. Thanks, structural typing:
declare function takeIUtils(iUtils: IUtils): void;
takeIUtils(Utils); // works anyway
This should allow your Router
class to behave as desired.
Side note, I wonder if you really want Utils
to be a class at all. If you never want to see an instance of Utils
like class X extends Utils ...; new X()
, then you're probably making life unnecessarily hard for yourself. Maybe it should just be a value:
export const Utils : IUtils =
toUri(route: string)
return route
Or a namespace
:
export namespace Utils
export function toUri(route: string)
return route
Or a module, or something.
Anyway, hope that helps. Good luck!
Yes, definitelynamespace
is a solution to my problems. Thank you. :)
– Greg Woz
Nov 12 at 21:09
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
You don't want Utils
to implement IUtils
, since Utils
is the instance type of the class. It looks like you want the Utils
constructor (whose type is typeof Utils
) to implement IUtils
. That is, you want toUri
to be a static method of the Utils
class. Like this:
abstract class Utils
public static toUri = (route: string) =>
return route
There is no way to annotate the class Utils
declaration to say that the static side of the class implements IUtils
. Luckily, the Utils
constructor will automatically be seen as something that implements IUtils
without needing to write implements IUtils
anywhere. Thanks, structural typing:
declare function takeIUtils(iUtils: IUtils): void;
takeIUtils(Utils); // works anyway
This should allow your Router
class to behave as desired.
Side note, I wonder if you really want Utils
to be a class at all. If you never want to see an instance of Utils
like class X extends Utils ...; new X()
, then you're probably making life unnecessarily hard for yourself. Maybe it should just be a value:
export const Utils : IUtils =
toUri(route: string)
return route
Or a namespace
:
export namespace Utils
export function toUri(route: string)
return route
Or a module, or something.
Anyway, hope that helps. Good luck!
You don't want Utils
to implement IUtils
, since Utils
is the instance type of the class. It looks like you want the Utils
constructor (whose type is typeof Utils
) to implement IUtils
. That is, you want toUri
to be a static method of the Utils
class. Like this:
abstract class Utils
public static toUri = (route: string) =>
return route
There is no way to annotate the class Utils
declaration to say that the static side of the class implements IUtils
. Luckily, the Utils
constructor will automatically be seen as something that implements IUtils
without needing to write implements IUtils
anywhere. Thanks, structural typing:
declare function takeIUtils(iUtils: IUtils): void;
takeIUtils(Utils); // works anyway
This should allow your Router
class to behave as desired.
Side note, I wonder if you really want Utils
to be a class at all. If you never want to see an instance of Utils
like class X extends Utils ...; new X()
, then you're probably making life unnecessarily hard for yourself. Maybe it should just be a value:
export const Utils : IUtils =
toUri(route: string)
return route
Or a namespace
:
export namespace Utils
export function toUri(route: string)
return route
Or a module, or something.
Anyway, hope that helps. Good luck!
answered Nov 9 at 17:39


jcalz
19.9k21535
19.9k21535
Yes, definitelynamespace
is a solution to my problems. Thank you. :)
– Greg Woz
Nov 12 at 21:09
add a comment |
Yes, definitelynamespace
is a solution to my problems. Thank you. :)
– Greg Woz
Nov 12 at 21:09
Yes, definitely
namespace
is a solution to my problems. Thank you. :)– Greg Woz
Nov 12 at 21:09
Yes, definitely
namespace
is a solution to my problems. Thank you. :)– Greg Woz
Nov 12 at 21:09
add a comment |
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53230459%2fusing-external-abstract-class-in-typescript-without-extending-it%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
nK D,oMH,k2J,idN8MQv,7,QNNO