Two dimensional function not returning array of values?
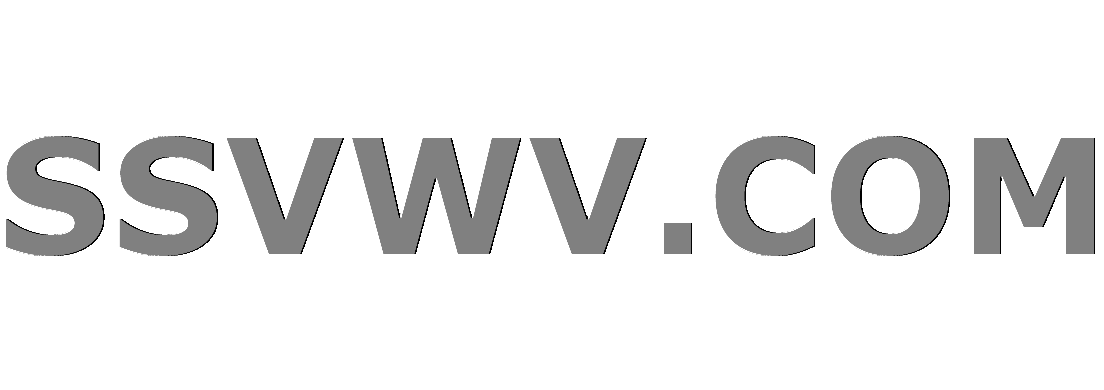
Multi tool use
up vote
0
down vote
favorite
I'm trying to plot a 2-dimensional function (specifically, a 2-d Laplace solution). I defined my function and it returns the right value when I put in specific numbers, but when I try running through an array of values (x,y below), it still returns only one number. I tried with a random function of x and y (e.g., f(x,y) = x^2 + y^2) and it gives me an array of values.
def V_func(x,y):
a = 5
b = 4
Vo = 4
n = np.arange(1,100,2)
sum_list =
for indx in range(len(n)):
sum_term = (1/n[indx])*(np.cosh(n[indx]*np.pi*x/a))/(np.cosh(n[indx]*np.pi*b/a))*np.sin(n[indx]*np.pi*y/a)
sum_list = np.append(sum_list,sum_term)
summation = np.sum(sum_list)
V = 4*Vo/np.pi * summation
return V
x = np.linspace(-4,4,50)
y = np.linspace(0,5,50)
V_func(x,y)
Out: 53.633709914177224
python arrays numpy
add a comment |
up vote
0
down vote
favorite
I'm trying to plot a 2-dimensional function (specifically, a 2-d Laplace solution). I defined my function and it returns the right value when I put in specific numbers, but when I try running through an array of values (x,y below), it still returns only one number. I tried with a random function of x and y (e.g., f(x,y) = x^2 + y^2) and it gives me an array of values.
def V_func(x,y):
a = 5
b = 4
Vo = 4
n = np.arange(1,100,2)
sum_list =
for indx in range(len(n)):
sum_term = (1/n[indx])*(np.cosh(n[indx]*np.pi*x/a))/(np.cosh(n[indx]*np.pi*b/a))*np.sin(n[indx]*np.pi*y/a)
sum_list = np.append(sum_list,sum_term)
summation = np.sum(sum_list)
V = 4*Vo/np.pi * summation
return V
x = np.linspace(-4,4,50)
y = np.linspace(0,5,50)
V_func(x,y)
Out: 53.633709914177224
python arrays numpy
sum_list
starts as a list.
sum_term
looks like it would produce an array the same size asx
andy
. Then you append this tosum_list
usingnp.append
(why notsum_list.append
?). Sosum_list
ends up a 1d array (read thenp.append
docs). Then younp.sum
that reducing it to one number (read its docs). It isn't clear where the 2d is supposed to come from? Fromx
,y
,n
or some outer product?
– hpaulj
Nov 9 at 5:04
From x and y, the summation is just a part of the function. Basically, I want to get a single number as an outcome but when I input an array, I'd like for it to return an array. For example, if the function z = x2 + y2 was given the same values for x and y as above, it returns an array.
– user10476896
Nov 9 at 9:28
does that simpler function produce a 1d or 2d array?
– hpaulj
Nov 9 at 15:11
1d, then I used the meshgrid function to get a 2d array.
– user10476896
Nov 9 at 17:42
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I'm trying to plot a 2-dimensional function (specifically, a 2-d Laplace solution). I defined my function and it returns the right value when I put in specific numbers, but when I try running through an array of values (x,y below), it still returns only one number. I tried with a random function of x and y (e.g., f(x,y) = x^2 + y^2) and it gives me an array of values.
def V_func(x,y):
a = 5
b = 4
Vo = 4
n = np.arange(1,100,2)
sum_list =
for indx in range(len(n)):
sum_term = (1/n[indx])*(np.cosh(n[indx]*np.pi*x/a))/(np.cosh(n[indx]*np.pi*b/a))*np.sin(n[indx]*np.pi*y/a)
sum_list = np.append(sum_list,sum_term)
summation = np.sum(sum_list)
V = 4*Vo/np.pi * summation
return V
x = np.linspace(-4,4,50)
y = np.linspace(0,5,50)
V_func(x,y)
Out: 53.633709914177224
python arrays numpy
I'm trying to plot a 2-dimensional function (specifically, a 2-d Laplace solution). I defined my function and it returns the right value when I put in specific numbers, but when I try running through an array of values (x,y below), it still returns only one number. I tried with a random function of x and y (e.g., f(x,y) = x^2 + y^2) and it gives me an array of values.
def V_func(x,y):
a = 5
b = 4
Vo = 4
n = np.arange(1,100,2)
sum_list =
for indx in range(len(n)):
sum_term = (1/n[indx])*(np.cosh(n[indx]*np.pi*x/a))/(np.cosh(n[indx]*np.pi*b/a))*np.sin(n[indx]*np.pi*y/a)
sum_list = np.append(sum_list,sum_term)
summation = np.sum(sum_list)
V = 4*Vo/np.pi * summation
return V
x = np.linspace(-4,4,50)
y = np.linspace(0,5,50)
V_func(x,y)
Out: 53.633709914177224
python arrays numpy
python arrays numpy
edited Nov 9 at 2:45
mjhm
13.6k93555
13.6k93555
asked Nov 9 at 2:19
user10476896
11
11
sum_list
starts as a list.
sum_term
looks like it would produce an array the same size asx
andy
. Then you append this tosum_list
usingnp.append
(why notsum_list.append
?). Sosum_list
ends up a 1d array (read thenp.append
docs). Then younp.sum
that reducing it to one number (read its docs). It isn't clear where the 2d is supposed to come from? Fromx
,y
,n
or some outer product?
– hpaulj
Nov 9 at 5:04
From x and y, the summation is just a part of the function. Basically, I want to get a single number as an outcome but when I input an array, I'd like for it to return an array. For example, if the function z = x2 + y2 was given the same values for x and y as above, it returns an array.
– user10476896
Nov 9 at 9:28
does that simpler function produce a 1d or 2d array?
– hpaulj
Nov 9 at 15:11
1d, then I used the meshgrid function to get a 2d array.
– user10476896
Nov 9 at 17:42
add a comment |
sum_list
starts as a list.
sum_term
looks like it would produce an array the same size asx
andy
. Then you append this tosum_list
usingnp.append
(why notsum_list.append
?). Sosum_list
ends up a 1d array (read thenp.append
docs). Then younp.sum
that reducing it to one number (read its docs). It isn't clear where the 2d is supposed to come from? Fromx
,y
,n
or some outer product?
– hpaulj
Nov 9 at 5:04
From x and y, the summation is just a part of the function. Basically, I want to get a single number as an outcome but when I input an array, I'd like for it to return an array. For example, if the function z = x2 + y2 was given the same values for x and y as above, it returns an array.
– user10476896
Nov 9 at 9:28
does that simpler function produce a 1d or 2d array?
– hpaulj
Nov 9 at 15:11
1d, then I used the meshgrid function to get a 2d array.
– user10476896
Nov 9 at 17:42
sum_list
starts as a list
. sum_term
looks like it would produce an array the same size as x
and y
. Then you append this to sum_list
using np.append
(why not sum_list.append
?). So sum_list
ends up a 1d array (read the np.append
docs). Then you np.sum
that reducing it to one number (read its docs). It isn't clear where the 2d is supposed to come from? From x
, y
, n
or some outer product?– hpaulj
Nov 9 at 5:04
sum_list
starts as a list
. sum_term
looks like it would produce an array the same size as x
and y
. Then you append this to sum_list
using np.append
(why not sum_list.append
?). So sum_list
ends up a 1d array (read the np.append
docs). Then you np.sum
that reducing it to one number (read its docs). It isn't clear where the 2d is supposed to come from? From x
, y
, n
or some outer product?– hpaulj
Nov 9 at 5:04
From x and y, the summation is just a part of the function. Basically, I want to get a single number as an outcome but when I input an array, I'd like for it to return an array. For example, if the function z = x2 + y2 was given the same values for x and y as above, it returns an array.
– user10476896
Nov 9 at 9:28
From x and y, the summation is just a part of the function. Basically, I want to get a single number as an outcome but when I input an array, I'd like for it to return an array. For example, if the function z = x2 + y2 was given the same values for x and y as above, it returns an array.
– user10476896
Nov 9 at 9:28
does that simpler function produce a 1d or 2d array?
– hpaulj
Nov 9 at 15:11
does that simpler function produce a 1d or 2d array?
– hpaulj
Nov 9 at 15:11
1d, then I used the meshgrid function to get a 2d array.
– user10476896
Nov 9 at 17:42
1d, then I used the meshgrid function to get a 2d array.
– user10476896
Nov 9 at 17:42
add a comment |
2 Answers
2
active
oldest
votes
up vote
0
down vote
Try this:
def V_func(x,y):
a = 5
b = 4
Vo = 4
n = np.arange(1,100,2)
# sum_list =
sum_list = np.zeros(50)
for indx in range(len(n)):
sum_term = (1/n[indx])*(np.cosh(n[indx]*np.pi*x/a))/(np.cosh(n[indx]*np.pi*b/a))*np.sin(n[indx]*np.pi*y/a)
# sum_list = np.append(sum_list,sum_term)
sum_list += sum_term
# summation = np.sum(sum_list)
# V = 4*Vo/np.pi * summation
V = 4*Vo/np.pi * sum_list
return V
When I do that, I end up with an array of 2500 instead of 50. I'm having trouble incorporating the summation of terms for n=1,3,5... in the function.
– user10476896
Nov 9 at 2:54
Ah! I see, edited. Is that better?
– mjhm
Nov 9 at 3:07
Wow, I see now! Thanks so much, I guess np.sum was messing up the output.
– user10476896
Nov 9 at 20:05
add a comment |
up vote
0
down vote
Define a pair of arrays:
In [6]: x = np.arange(3); y = np.arange(10,13)
In [7]: x,y
Out[7]: (array([0, 1, 2]), array([10, 11, 12]))
Try a simple function of the 2
In [8]: x + y
Out[8]: array([10, 12, 14])
Since they have the same size, they can be summed (or otherwise combined) elementwise. The result has the same shape as the 2 inputs.
Now try 'broadcasting'. x[:,None]
has shape (3,1)
In [9]: x[:,None] + y
Out[9]:
array([[10, 11, 12],
[11, 12, 13],
[12, 13, 14]])
The result is (3,3), the first 3 from the reshaped x
, the second from y
.
I can generate the pair of arrays with meshgrid
:
In [10]: I,J = np.meshgrid(x,y,sparse=True, indexing='ij')
In [11]: I
Out[11]:
array([[0],
[1],
[2]])
In [12]: J
Out[12]: array([[10, 11, 12]])
In [13]: I + J
Out[13]:
array([[10, 11, 12],
[11, 12, 13],
[12, 13, 14]])
Note the added parameters in meshgrid
. So that's how we go about generating 2d values from a pair of 1d arrays.
Now look at what sum
does. As you use it in the function:
In [14]: np.sum(I + J)
Out[14]: 108
the result is a scalar. See the docs. If I specify an axis
I get an array.
In [15]: np.sum(I + J, axis=0)
Out[15]: array([33, 36, 39])
If you gave V_func
the right x
and y
, sum_list
could be a 3d array. That axis-less sum
reduces it to a scalar.
In code like this you need to keep track of array shapes. Include test prints if needed; don't just assume anything; test it. Pay attention to how dimensions grow and shrink as they pass through various operations.
Thanks so much, I figured it out.
– user10476896
Nov 9 at 20:05
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
Try this:
def V_func(x,y):
a = 5
b = 4
Vo = 4
n = np.arange(1,100,2)
# sum_list =
sum_list = np.zeros(50)
for indx in range(len(n)):
sum_term = (1/n[indx])*(np.cosh(n[indx]*np.pi*x/a))/(np.cosh(n[indx]*np.pi*b/a))*np.sin(n[indx]*np.pi*y/a)
# sum_list = np.append(sum_list,sum_term)
sum_list += sum_term
# summation = np.sum(sum_list)
# V = 4*Vo/np.pi * summation
V = 4*Vo/np.pi * sum_list
return V
When I do that, I end up with an array of 2500 instead of 50. I'm having trouble incorporating the summation of terms for n=1,3,5... in the function.
– user10476896
Nov 9 at 2:54
Ah! I see, edited. Is that better?
– mjhm
Nov 9 at 3:07
Wow, I see now! Thanks so much, I guess np.sum was messing up the output.
– user10476896
Nov 9 at 20:05
add a comment |
up vote
0
down vote
Try this:
def V_func(x,y):
a = 5
b = 4
Vo = 4
n = np.arange(1,100,2)
# sum_list =
sum_list = np.zeros(50)
for indx in range(len(n)):
sum_term = (1/n[indx])*(np.cosh(n[indx]*np.pi*x/a))/(np.cosh(n[indx]*np.pi*b/a))*np.sin(n[indx]*np.pi*y/a)
# sum_list = np.append(sum_list,sum_term)
sum_list += sum_term
# summation = np.sum(sum_list)
# V = 4*Vo/np.pi * summation
V = 4*Vo/np.pi * sum_list
return V
When I do that, I end up with an array of 2500 instead of 50. I'm having trouble incorporating the summation of terms for n=1,3,5... in the function.
– user10476896
Nov 9 at 2:54
Ah! I see, edited. Is that better?
– mjhm
Nov 9 at 3:07
Wow, I see now! Thanks so much, I guess np.sum was messing up the output.
– user10476896
Nov 9 at 20:05
add a comment |
up vote
0
down vote
up vote
0
down vote
Try this:
def V_func(x,y):
a = 5
b = 4
Vo = 4
n = np.arange(1,100,2)
# sum_list =
sum_list = np.zeros(50)
for indx in range(len(n)):
sum_term = (1/n[indx])*(np.cosh(n[indx]*np.pi*x/a))/(np.cosh(n[indx]*np.pi*b/a))*np.sin(n[indx]*np.pi*y/a)
# sum_list = np.append(sum_list,sum_term)
sum_list += sum_term
# summation = np.sum(sum_list)
# V = 4*Vo/np.pi * summation
V = 4*Vo/np.pi * sum_list
return V
Try this:
def V_func(x,y):
a = 5
b = 4
Vo = 4
n = np.arange(1,100,2)
# sum_list =
sum_list = np.zeros(50)
for indx in range(len(n)):
sum_term = (1/n[indx])*(np.cosh(n[indx]*np.pi*x/a))/(np.cosh(n[indx]*np.pi*b/a))*np.sin(n[indx]*np.pi*y/a)
# sum_list = np.append(sum_list,sum_term)
sum_list += sum_term
# summation = np.sum(sum_list)
# V = 4*Vo/np.pi * summation
V = 4*Vo/np.pi * sum_list
return V
edited Nov 9 at 3:06
answered Nov 9 at 2:43
mjhm
13.6k93555
13.6k93555
When I do that, I end up with an array of 2500 instead of 50. I'm having trouble incorporating the summation of terms for n=1,3,5... in the function.
– user10476896
Nov 9 at 2:54
Ah! I see, edited. Is that better?
– mjhm
Nov 9 at 3:07
Wow, I see now! Thanks so much, I guess np.sum was messing up the output.
– user10476896
Nov 9 at 20:05
add a comment |
When I do that, I end up with an array of 2500 instead of 50. I'm having trouble incorporating the summation of terms for n=1,3,5... in the function.
– user10476896
Nov 9 at 2:54
Ah! I see, edited. Is that better?
– mjhm
Nov 9 at 3:07
Wow, I see now! Thanks so much, I guess np.sum was messing up the output.
– user10476896
Nov 9 at 20:05
When I do that, I end up with an array of 2500 instead of 50. I'm having trouble incorporating the summation of terms for n=1,3,5... in the function.
– user10476896
Nov 9 at 2:54
When I do that, I end up with an array of 2500 instead of 50. I'm having trouble incorporating the summation of terms for n=1,3,5... in the function.
– user10476896
Nov 9 at 2:54
Ah! I see, edited. Is that better?
– mjhm
Nov 9 at 3:07
Ah! I see, edited. Is that better?
– mjhm
Nov 9 at 3:07
Wow, I see now! Thanks so much, I guess np.sum was messing up the output.
– user10476896
Nov 9 at 20:05
Wow, I see now! Thanks so much, I guess np.sum was messing up the output.
– user10476896
Nov 9 at 20:05
add a comment |
up vote
0
down vote
Define a pair of arrays:
In [6]: x = np.arange(3); y = np.arange(10,13)
In [7]: x,y
Out[7]: (array([0, 1, 2]), array([10, 11, 12]))
Try a simple function of the 2
In [8]: x + y
Out[8]: array([10, 12, 14])
Since they have the same size, they can be summed (or otherwise combined) elementwise. The result has the same shape as the 2 inputs.
Now try 'broadcasting'. x[:,None]
has shape (3,1)
In [9]: x[:,None] + y
Out[9]:
array([[10, 11, 12],
[11, 12, 13],
[12, 13, 14]])
The result is (3,3), the first 3 from the reshaped x
, the second from y
.
I can generate the pair of arrays with meshgrid
:
In [10]: I,J = np.meshgrid(x,y,sparse=True, indexing='ij')
In [11]: I
Out[11]:
array([[0],
[1],
[2]])
In [12]: J
Out[12]: array([[10, 11, 12]])
In [13]: I + J
Out[13]:
array([[10, 11, 12],
[11, 12, 13],
[12, 13, 14]])
Note the added parameters in meshgrid
. So that's how we go about generating 2d values from a pair of 1d arrays.
Now look at what sum
does. As you use it in the function:
In [14]: np.sum(I + J)
Out[14]: 108
the result is a scalar. See the docs. If I specify an axis
I get an array.
In [15]: np.sum(I + J, axis=0)
Out[15]: array([33, 36, 39])
If you gave V_func
the right x
and y
, sum_list
could be a 3d array. That axis-less sum
reduces it to a scalar.
In code like this you need to keep track of array shapes. Include test prints if needed; don't just assume anything; test it. Pay attention to how dimensions grow and shrink as they pass through various operations.
Thanks so much, I figured it out.
– user10476896
Nov 9 at 20:05
add a comment |
up vote
0
down vote
Define a pair of arrays:
In [6]: x = np.arange(3); y = np.arange(10,13)
In [7]: x,y
Out[7]: (array([0, 1, 2]), array([10, 11, 12]))
Try a simple function of the 2
In [8]: x + y
Out[8]: array([10, 12, 14])
Since they have the same size, they can be summed (or otherwise combined) elementwise. The result has the same shape as the 2 inputs.
Now try 'broadcasting'. x[:,None]
has shape (3,1)
In [9]: x[:,None] + y
Out[9]:
array([[10, 11, 12],
[11, 12, 13],
[12, 13, 14]])
The result is (3,3), the first 3 from the reshaped x
, the second from y
.
I can generate the pair of arrays with meshgrid
:
In [10]: I,J = np.meshgrid(x,y,sparse=True, indexing='ij')
In [11]: I
Out[11]:
array([[0],
[1],
[2]])
In [12]: J
Out[12]: array([[10, 11, 12]])
In [13]: I + J
Out[13]:
array([[10, 11, 12],
[11, 12, 13],
[12, 13, 14]])
Note the added parameters in meshgrid
. So that's how we go about generating 2d values from a pair of 1d arrays.
Now look at what sum
does. As you use it in the function:
In [14]: np.sum(I + J)
Out[14]: 108
the result is a scalar. See the docs. If I specify an axis
I get an array.
In [15]: np.sum(I + J, axis=0)
Out[15]: array([33, 36, 39])
If you gave V_func
the right x
and y
, sum_list
could be a 3d array. That axis-less sum
reduces it to a scalar.
In code like this you need to keep track of array shapes. Include test prints if needed; don't just assume anything; test it. Pay attention to how dimensions grow and shrink as they pass through various operations.
Thanks so much, I figured it out.
– user10476896
Nov 9 at 20:05
add a comment |
up vote
0
down vote
up vote
0
down vote
Define a pair of arrays:
In [6]: x = np.arange(3); y = np.arange(10,13)
In [7]: x,y
Out[7]: (array([0, 1, 2]), array([10, 11, 12]))
Try a simple function of the 2
In [8]: x + y
Out[8]: array([10, 12, 14])
Since they have the same size, they can be summed (or otherwise combined) elementwise. The result has the same shape as the 2 inputs.
Now try 'broadcasting'. x[:,None]
has shape (3,1)
In [9]: x[:,None] + y
Out[9]:
array([[10, 11, 12],
[11, 12, 13],
[12, 13, 14]])
The result is (3,3), the first 3 from the reshaped x
, the second from y
.
I can generate the pair of arrays with meshgrid
:
In [10]: I,J = np.meshgrid(x,y,sparse=True, indexing='ij')
In [11]: I
Out[11]:
array([[0],
[1],
[2]])
In [12]: J
Out[12]: array([[10, 11, 12]])
In [13]: I + J
Out[13]:
array([[10, 11, 12],
[11, 12, 13],
[12, 13, 14]])
Note the added parameters in meshgrid
. So that's how we go about generating 2d values from a pair of 1d arrays.
Now look at what sum
does. As you use it in the function:
In [14]: np.sum(I + J)
Out[14]: 108
the result is a scalar. See the docs. If I specify an axis
I get an array.
In [15]: np.sum(I + J, axis=0)
Out[15]: array([33, 36, 39])
If you gave V_func
the right x
and y
, sum_list
could be a 3d array. That axis-less sum
reduces it to a scalar.
In code like this you need to keep track of array shapes. Include test prints if needed; don't just assume anything; test it. Pay attention to how dimensions grow and shrink as they pass through various operations.
Define a pair of arrays:
In [6]: x = np.arange(3); y = np.arange(10,13)
In [7]: x,y
Out[7]: (array([0, 1, 2]), array([10, 11, 12]))
Try a simple function of the 2
In [8]: x + y
Out[8]: array([10, 12, 14])
Since they have the same size, they can be summed (or otherwise combined) elementwise. The result has the same shape as the 2 inputs.
Now try 'broadcasting'. x[:,None]
has shape (3,1)
In [9]: x[:,None] + y
Out[9]:
array([[10, 11, 12],
[11, 12, 13],
[12, 13, 14]])
The result is (3,3), the first 3 from the reshaped x
, the second from y
.
I can generate the pair of arrays with meshgrid
:
In [10]: I,J = np.meshgrid(x,y,sparse=True, indexing='ij')
In [11]: I
Out[11]:
array([[0],
[1],
[2]])
In [12]: J
Out[12]: array([[10, 11, 12]])
In [13]: I + J
Out[13]:
array([[10, 11, 12],
[11, 12, 13],
[12, 13, 14]])
Note the added parameters in meshgrid
. So that's how we go about generating 2d values from a pair of 1d arrays.
Now look at what sum
does. As you use it in the function:
In [14]: np.sum(I + J)
Out[14]: 108
the result is a scalar. See the docs. If I specify an axis
I get an array.
In [15]: np.sum(I + J, axis=0)
Out[15]: array([33, 36, 39])
If you gave V_func
the right x
and y
, sum_list
could be a 3d array. That axis-less sum
reduces it to a scalar.
In code like this you need to keep track of array shapes. Include test prints if needed; don't just assume anything; test it. Pay attention to how dimensions grow and shrink as they pass through various operations.
answered Nov 9 at 18:00
hpaulj
107k673137
107k673137
Thanks so much, I figured it out.
– user10476896
Nov 9 at 20:05
add a comment |
Thanks so much, I figured it out.
– user10476896
Nov 9 at 20:05
Thanks so much, I figured it out.
– user10476896
Nov 9 at 20:05
Thanks so much, I figured it out.
– user10476896
Nov 9 at 20:05
add a comment |
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53218937%2ftwo-dimensional-function-not-returning-array-of-values%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
lC IuFgEy8rFiOoe0B1TAQviSSCN2o663SzrO,R4JVLaS8j6kgu9 3F4cHLuG si,T MZrIgtk,9hd
sum_list
starts as a list.
sum_term
looks like it would produce an array the same size asx
andy
. Then you append this tosum_list
usingnp.append
(why notsum_list.append
?). Sosum_list
ends up a 1d array (read thenp.append
docs). Then younp.sum
that reducing it to one number (read its docs). It isn't clear where the 2d is supposed to come from? Fromx
,y
,n
or some outer product?– hpaulj
Nov 9 at 5:04
From x and y, the summation is just a part of the function. Basically, I want to get a single number as an outcome but when I input an array, I'd like for it to return an array. For example, if the function z = x2 + y2 was given the same values for x and y as above, it returns an array.
– user10476896
Nov 9 at 9:28
does that simpler function produce a 1d or 2d array?
– hpaulj
Nov 9 at 15:11
1d, then I used the meshgrid function to get a 2d array.
– user10476896
Nov 9 at 17:42