Get Objects Not Transacted for Last 3 Months
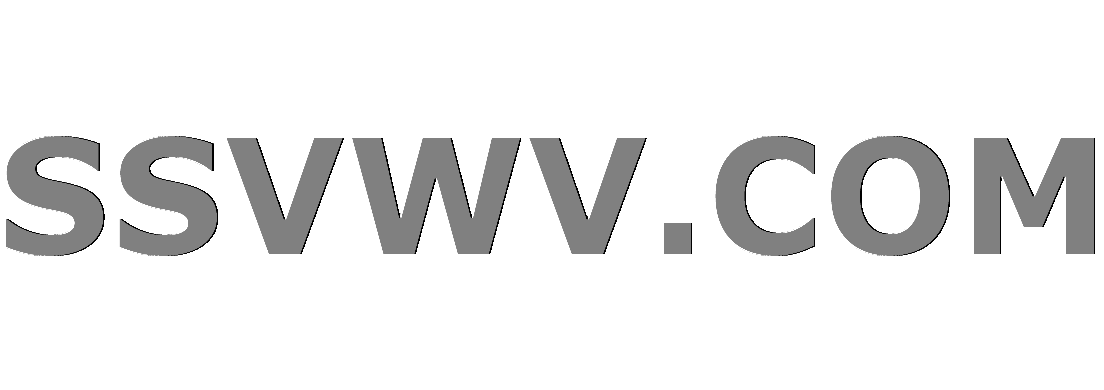
Multi tool use
I'm fairly new to Django. I have two objects in a django project: Transaction and Item. Item can have many Transaction objects. Transaction has date_time and item fields. How can I write a django query to fetch Items that haven't had any transactions in the past 20 days?
class Item(models.Model):
name = models.CharField(max_length=80)
class Transaction(models.Model):
date_time = models.DateTimeField(default=timezone.now)
item = models.ForeignKey(Item)
Thanks in advance.
python django
add a comment |
I'm fairly new to Django. I have two objects in a django project: Transaction and Item. Item can have many Transaction objects. Transaction has date_time and item fields. How can I write a django query to fetch Items that haven't had any transactions in the past 20 days?
class Item(models.Model):
name = models.CharField(max_length=80)
class Transaction(models.Model):
date_time = models.DateTimeField(default=timezone.now)
item = models.ForeignKey(Item)
Thanks in advance.
python django
Show your model and view codes.
– MD. Khairul Basar
Nov 13 '18 at 9:20
Hi Basar, I have shown the models
– Teddy
Nov 13 '18 at 10:09
add a comment |
I'm fairly new to Django. I have two objects in a django project: Transaction and Item. Item can have many Transaction objects. Transaction has date_time and item fields. How can I write a django query to fetch Items that haven't had any transactions in the past 20 days?
class Item(models.Model):
name = models.CharField(max_length=80)
class Transaction(models.Model):
date_time = models.DateTimeField(default=timezone.now)
item = models.ForeignKey(Item)
Thanks in advance.
python django
I'm fairly new to Django. I have two objects in a django project: Transaction and Item. Item can have many Transaction objects. Transaction has date_time and item fields. How can I write a django query to fetch Items that haven't had any transactions in the past 20 days?
class Item(models.Model):
name = models.CharField(max_length=80)
class Transaction(models.Model):
date_time = models.DateTimeField(default=timezone.now)
item = models.ForeignKey(Item)
Thanks in advance.
python django
python django
edited Nov 13 '18 at 10:08
Teddy
asked Nov 13 '18 at 8:51
TeddyTeddy
83
83
Show your model and view codes.
– MD. Khairul Basar
Nov 13 '18 at 9:20
Hi Basar, I have shown the models
– Teddy
Nov 13 '18 at 10:09
add a comment |
Show your model and view codes.
– MD. Khairul Basar
Nov 13 '18 at 9:20
Hi Basar, I have shown the models
– Teddy
Nov 13 '18 at 10:09
Show your model and view codes.
– MD. Khairul Basar
Nov 13 '18 at 9:20
Show your model and view codes.
– MD. Khairul Basar
Nov 13 '18 at 9:20
Hi Basar, I have shown the models
– Teddy
Nov 13 '18 at 10:09
Hi Basar, I have shown the models
– Teddy
Nov 13 '18 at 10:09
add a comment |
1 Answer
1
active
oldest
votes
Assuming your models are:
class Item(models.Model):
name = models.CharField(max_length=100)
class Transaction(models.Model):
item = models.ForeignKey(Item, on_delete=models.CASCADE)
created_at = models.DateTimeField()
You can do a query like:
from datetime import datetime, timedelta
Item.objects.distinct().exclude(transaction__created_at__gte=datetime.now() - timedelta(days=20))
This query will find items with transactions in past 20 days, then show rest of the items (which dont have any transaction in past 20 days).
Try this on Django Shell. To open shell: python manage.py shell
What version of Django are you using?import django
django.VERSION
should give you version.
– jerrymouse
Nov 13 '18 at 10:49
@Teddy can you please copy and paste the exact command that gave you this error?
– jerrymouse
Nov 13 '18 at 10:52
There's no field namedtransaction
inItem
model.
– MD. Khairul Basar
Nov 13 '18 at 10:55
1
@MD.KhairulBasar From docs.djangoproject.com/en/2.1/topics/db/queries/…It works backwards, too. To refer to a “reverse” relationship, just use the lowercase name of the model.
– jerrymouse
Nov 13 '18 at 11:02
@jerrymouse if there has been a transaction both before and after 20 days, the Item will still show up but all I need is only the Items that haven't had any transaction in the last 20 days
– Teddy
Nov 13 '18 at 11:42
|
show 2 more comments
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53277088%2fget-objects-not-transacted-for-last-3-months%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Assuming your models are:
class Item(models.Model):
name = models.CharField(max_length=100)
class Transaction(models.Model):
item = models.ForeignKey(Item, on_delete=models.CASCADE)
created_at = models.DateTimeField()
You can do a query like:
from datetime import datetime, timedelta
Item.objects.distinct().exclude(transaction__created_at__gte=datetime.now() - timedelta(days=20))
This query will find items with transactions in past 20 days, then show rest of the items (which dont have any transaction in past 20 days).
Try this on Django Shell. To open shell: python manage.py shell
What version of Django are you using?import django
django.VERSION
should give you version.
– jerrymouse
Nov 13 '18 at 10:49
@Teddy can you please copy and paste the exact command that gave you this error?
– jerrymouse
Nov 13 '18 at 10:52
There's no field namedtransaction
inItem
model.
– MD. Khairul Basar
Nov 13 '18 at 10:55
1
@MD.KhairulBasar From docs.djangoproject.com/en/2.1/topics/db/queries/…It works backwards, too. To refer to a “reverse” relationship, just use the lowercase name of the model.
– jerrymouse
Nov 13 '18 at 11:02
@jerrymouse if there has been a transaction both before and after 20 days, the Item will still show up but all I need is only the Items that haven't had any transaction in the last 20 days
– Teddy
Nov 13 '18 at 11:42
|
show 2 more comments
Assuming your models are:
class Item(models.Model):
name = models.CharField(max_length=100)
class Transaction(models.Model):
item = models.ForeignKey(Item, on_delete=models.CASCADE)
created_at = models.DateTimeField()
You can do a query like:
from datetime import datetime, timedelta
Item.objects.distinct().exclude(transaction__created_at__gte=datetime.now() - timedelta(days=20))
This query will find items with transactions in past 20 days, then show rest of the items (which dont have any transaction in past 20 days).
Try this on Django Shell. To open shell: python manage.py shell
What version of Django are you using?import django
django.VERSION
should give you version.
– jerrymouse
Nov 13 '18 at 10:49
@Teddy can you please copy and paste the exact command that gave you this error?
– jerrymouse
Nov 13 '18 at 10:52
There's no field namedtransaction
inItem
model.
– MD. Khairul Basar
Nov 13 '18 at 10:55
1
@MD.KhairulBasar From docs.djangoproject.com/en/2.1/topics/db/queries/…It works backwards, too. To refer to a “reverse” relationship, just use the lowercase name of the model.
– jerrymouse
Nov 13 '18 at 11:02
@jerrymouse if there has been a transaction both before and after 20 days, the Item will still show up but all I need is only the Items that haven't had any transaction in the last 20 days
– Teddy
Nov 13 '18 at 11:42
|
show 2 more comments
Assuming your models are:
class Item(models.Model):
name = models.CharField(max_length=100)
class Transaction(models.Model):
item = models.ForeignKey(Item, on_delete=models.CASCADE)
created_at = models.DateTimeField()
You can do a query like:
from datetime import datetime, timedelta
Item.objects.distinct().exclude(transaction__created_at__gte=datetime.now() - timedelta(days=20))
This query will find items with transactions in past 20 days, then show rest of the items (which dont have any transaction in past 20 days).
Try this on Django Shell. To open shell: python manage.py shell
Assuming your models are:
class Item(models.Model):
name = models.CharField(max_length=100)
class Transaction(models.Model):
item = models.ForeignKey(Item, on_delete=models.CASCADE)
created_at = models.DateTimeField()
You can do a query like:
from datetime import datetime, timedelta
Item.objects.distinct().exclude(transaction__created_at__gte=datetime.now() - timedelta(days=20))
This query will find items with transactions in past 20 days, then show rest of the items (which dont have any transaction in past 20 days).
Try this on Django Shell. To open shell: python manage.py shell
edited Nov 13 '18 at 18:02
answered Nov 13 '18 at 9:27
jerrymousejerrymouse
8,590104967
8,590104967
What version of Django are you using?import django
django.VERSION
should give you version.
– jerrymouse
Nov 13 '18 at 10:49
@Teddy can you please copy and paste the exact command that gave you this error?
– jerrymouse
Nov 13 '18 at 10:52
There's no field namedtransaction
inItem
model.
– MD. Khairul Basar
Nov 13 '18 at 10:55
1
@MD.KhairulBasar From docs.djangoproject.com/en/2.1/topics/db/queries/…It works backwards, too. To refer to a “reverse” relationship, just use the lowercase name of the model.
– jerrymouse
Nov 13 '18 at 11:02
@jerrymouse if there has been a transaction both before and after 20 days, the Item will still show up but all I need is only the Items that haven't had any transaction in the last 20 days
– Teddy
Nov 13 '18 at 11:42
|
show 2 more comments
What version of Django are you using?import django
django.VERSION
should give you version.
– jerrymouse
Nov 13 '18 at 10:49
@Teddy can you please copy and paste the exact command that gave you this error?
– jerrymouse
Nov 13 '18 at 10:52
There's no field namedtransaction
inItem
model.
– MD. Khairul Basar
Nov 13 '18 at 10:55
1
@MD.KhairulBasar From docs.djangoproject.com/en/2.1/topics/db/queries/…It works backwards, too. To refer to a “reverse” relationship, just use the lowercase name of the model.
– jerrymouse
Nov 13 '18 at 11:02
@jerrymouse if there has been a transaction both before and after 20 days, the Item will still show up but all I need is only the Items that haven't had any transaction in the last 20 days
– Teddy
Nov 13 '18 at 11:42
What version of Django are you using?
import django
django.VERSION
should give you version.– jerrymouse
Nov 13 '18 at 10:49
What version of Django are you using?
import django
django.VERSION
should give you version.– jerrymouse
Nov 13 '18 at 10:49
@Teddy can you please copy and paste the exact command that gave you this error?
– jerrymouse
Nov 13 '18 at 10:52
@Teddy can you please copy and paste the exact command that gave you this error?
– jerrymouse
Nov 13 '18 at 10:52
There's no field named
transaction
in Item
model.– MD. Khairul Basar
Nov 13 '18 at 10:55
There's no field named
transaction
in Item
model.– MD. Khairul Basar
Nov 13 '18 at 10:55
1
1
@MD.KhairulBasar From docs.djangoproject.com/en/2.1/topics/db/queries/…
It works backwards, too. To refer to a “reverse” relationship, just use the lowercase name of the model.
– jerrymouse
Nov 13 '18 at 11:02
@MD.KhairulBasar From docs.djangoproject.com/en/2.1/topics/db/queries/…
It works backwards, too. To refer to a “reverse” relationship, just use the lowercase name of the model.
– jerrymouse
Nov 13 '18 at 11:02
@jerrymouse if there has been a transaction both before and after 20 days, the Item will still show up but all I need is only the Items that haven't had any transaction in the last 20 days
– Teddy
Nov 13 '18 at 11:42
@jerrymouse if there has been a transaction both before and after 20 days, the Item will still show up but all I need is only the Items that haven't had any transaction in the last 20 days
– Teddy
Nov 13 '18 at 11:42
|
show 2 more comments
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53277088%2fget-objects-not-transacted-for-last-3-months%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
BD3kRwkmA R53OstDZMOfATAojgs
Show your model and view codes.
– MD. Khairul Basar
Nov 13 '18 at 9:20
Hi Basar, I have shown the models
– Teddy
Nov 13 '18 at 10:09