Move objecj within canvas canvas boundary limit with zoom Applied
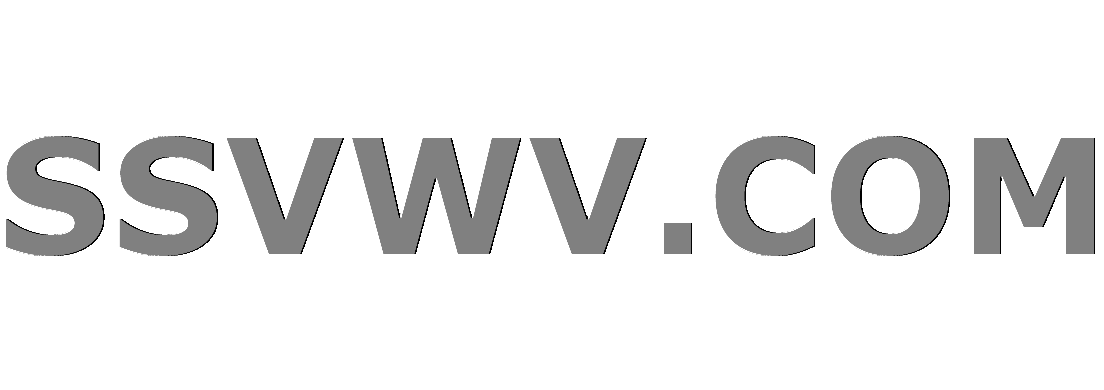
Multi tool use
I'm trying to limit the movement of an object only inside the canvas. This I have achieved with the following code
if(obj.getBoundingRect().top < 0 || obj.getBoundingRect().left< 0)
obj.top = Math.max(obj.top, obj.top-obj.getBoundingRect().top);
obj.left = Math.max(obj.left, obj.left-obj.getBoundingRect().left));
// bot-right corner
if(obj.getBoundingRect().top+obj.getBoundingRect().height > obj.canvas.height || obj.getBoundingRect().left+obj.getBoundingRect().width > obj.canvas.width)
obj.top = Math.min(obj.top, obj.canvas.height-obj.getBoundingRect().height+obj.top-obj.getBoundingRect().top);
obj.left = Math.min(obj.left, obj.canvas.width-obj.getBoundingRect().width+obj.left-obj.getBoundingRect().left);
This works well when I have no zoom applied to the canvas.
I need to be able to make it work also when I apply a zoom + or a zoom -
I had thought about trying to solve it like this
if(obj.getBoundingRect().top*canvas.getZoom() < 0 || obj.getBoundingRect().left*canvas.getZoom() < 0)
obj.top = Math.max(obj.top, obj.top-obj.getBoundingRect().top)*canvas.getZoom();
obj.left = Math.max(obj.left, obj.left-obj.getBoundingRect().left)*canvas.getZoom();
// bot-right corner
if(obj.getBoundingRect().top*canvas.getZoom()+obj.getBoundingRect().height > obj.canvas.height || obj.getBoundingRect().left*canvas.getZoom()+obj.getBoundingRect().width > obj.canvas.width)
obj.top = Math.min(obj.top, obj.canvas.height-obj.getBoundingRect().height+obj.top-obj.getBoundingRect().top)*canvas.getZoom();
obj.left = Math.min(obj.left, obj.canvas.width-obj.getBoundingRect().width+obj.left-obj.getBoundingRect().left)*canvas.getZoom();
But it does not work. Can you help me?
Edit: Add the fiddle
https://jsfiddle.net/samael205/m19cuk0j/1/
Edit: Sloved, that ONLY works with Fabric 2.0.0+
if(obj.getBoundingRect(true).top < 0 || obj.getBoundingRect(true).left < 0)
obj.top = Math.max(obj.top, obj.top-obj.getBoundingRect(true).top);
obj.left = Math.max(obj.left, obj.left-obj.getBoundingRect(true).left);
// bot-right corner
if(obj.getBoundingRect(true).top+obj.getBoundingRect(true).height > obj.canvas.height/canvas.getZoom() || obj.getBoundingRect(true).left+obj.getBoundingRect(true).width > obj.canvas.width/canvas.getZoom())
obj.top = Math.min(obj.top, obj.canvas.height/canvas.getZoom()-obj.getBoundingRect(true).height+obj.top-obj.getBoundingRect(true).top);
obj.left = Math.min(obj.left, obj.canvas.width/canvas.getZoom()-obj.getBoundingRect(true).width+obj.left-obj.getBoundingRect(true).left);
javascript html5 canvas fabricjs
add a comment |
I'm trying to limit the movement of an object only inside the canvas. This I have achieved with the following code
if(obj.getBoundingRect().top < 0 || obj.getBoundingRect().left< 0)
obj.top = Math.max(obj.top, obj.top-obj.getBoundingRect().top);
obj.left = Math.max(obj.left, obj.left-obj.getBoundingRect().left));
// bot-right corner
if(obj.getBoundingRect().top+obj.getBoundingRect().height > obj.canvas.height || obj.getBoundingRect().left+obj.getBoundingRect().width > obj.canvas.width)
obj.top = Math.min(obj.top, obj.canvas.height-obj.getBoundingRect().height+obj.top-obj.getBoundingRect().top);
obj.left = Math.min(obj.left, obj.canvas.width-obj.getBoundingRect().width+obj.left-obj.getBoundingRect().left);
This works well when I have no zoom applied to the canvas.
I need to be able to make it work also when I apply a zoom + or a zoom -
I had thought about trying to solve it like this
if(obj.getBoundingRect().top*canvas.getZoom() < 0 || obj.getBoundingRect().left*canvas.getZoom() < 0)
obj.top = Math.max(obj.top, obj.top-obj.getBoundingRect().top)*canvas.getZoom();
obj.left = Math.max(obj.left, obj.left-obj.getBoundingRect().left)*canvas.getZoom();
// bot-right corner
if(obj.getBoundingRect().top*canvas.getZoom()+obj.getBoundingRect().height > obj.canvas.height || obj.getBoundingRect().left*canvas.getZoom()+obj.getBoundingRect().width > obj.canvas.width)
obj.top = Math.min(obj.top, obj.canvas.height-obj.getBoundingRect().height+obj.top-obj.getBoundingRect().top)*canvas.getZoom();
obj.left = Math.min(obj.left, obj.canvas.width-obj.getBoundingRect().width+obj.left-obj.getBoundingRect().left)*canvas.getZoom();
But it does not work. Can you help me?
Edit: Add the fiddle
https://jsfiddle.net/samael205/m19cuk0j/1/
Edit: Sloved, that ONLY works with Fabric 2.0.0+
if(obj.getBoundingRect(true).top < 0 || obj.getBoundingRect(true).left < 0)
obj.top = Math.max(obj.top, obj.top-obj.getBoundingRect(true).top);
obj.left = Math.max(obj.left, obj.left-obj.getBoundingRect(true).left);
// bot-right corner
if(obj.getBoundingRect(true).top+obj.getBoundingRect(true).height > obj.canvas.height/canvas.getZoom() || obj.getBoundingRect(true).left+obj.getBoundingRect(true).width > obj.canvas.width/canvas.getZoom())
obj.top = Math.min(obj.top, obj.canvas.height/canvas.getZoom()-obj.getBoundingRect(true).height+obj.top-obj.getBoundingRect(true).top);
obj.left = Math.min(obj.left, obj.canvas.width/canvas.getZoom()-obj.getBoundingRect(true).width+obj.left-obj.getBoundingRect(true).left);
javascript html5 canvas fabricjs
add a comment |
I'm trying to limit the movement of an object only inside the canvas. This I have achieved with the following code
if(obj.getBoundingRect().top < 0 || obj.getBoundingRect().left< 0)
obj.top = Math.max(obj.top, obj.top-obj.getBoundingRect().top);
obj.left = Math.max(obj.left, obj.left-obj.getBoundingRect().left));
// bot-right corner
if(obj.getBoundingRect().top+obj.getBoundingRect().height > obj.canvas.height || obj.getBoundingRect().left+obj.getBoundingRect().width > obj.canvas.width)
obj.top = Math.min(obj.top, obj.canvas.height-obj.getBoundingRect().height+obj.top-obj.getBoundingRect().top);
obj.left = Math.min(obj.left, obj.canvas.width-obj.getBoundingRect().width+obj.left-obj.getBoundingRect().left);
This works well when I have no zoom applied to the canvas.
I need to be able to make it work also when I apply a zoom + or a zoom -
I had thought about trying to solve it like this
if(obj.getBoundingRect().top*canvas.getZoom() < 0 || obj.getBoundingRect().left*canvas.getZoom() < 0)
obj.top = Math.max(obj.top, obj.top-obj.getBoundingRect().top)*canvas.getZoom();
obj.left = Math.max(obj.left, obj.left-obj.getBoundingRect().left)*canvas.getZoom();
// bot-right corner
if(obj.getBoundingRect().top*canvas.getZoom()+obj.getBoundingRect().height > obj.canvas.height || obj.getBoundingRect().left*canvas.getZoom()+obj.getBoundingRect().width > obj.canvas.width)
obj.top = Math.min(obj.top, obj.canvas.height-obj.getBoundingRect().height+obj.top-obj.getBoundingRect().top)*canvas.getZoom();
obj.left = Math.min(obj.left, obj.canvas.width-obj.getBoundingRect().width+obj.left-obj.getBoundingRect().left)*canvas.getZoom();
But it does not work. Can you help me?
Edit: Add the fiddle
https://jsfiddle.net/samael205/m19cuk0j/1/
Edit: Sloved, that ONLY works with Fabric 2.0.0+
if(obj.getBoundingRect(true).top < 0 || obj.getBoundingRect(true).left < 0)
obj.top = Math.max(obj.top, obj.top-obj.getBoundingRect(true).top);
obj.left = Math.max(obj.left, obj.left-obj.getBoundingRect(true).left);
// bot-right corner
if(obj.getBoundingRect(true).top+obj.getBoundingRect(true).height > obj.canvas.height/canvas.getZoom() || obj.getBoundingRect(true).left+obj.getBoundingRect(true).width > obj.canvas.width/canvas.getZoom())
obj.top = Math.min(obj.top, obj.canvas.height/canvas.getZoom()-obj.getBoundingRect(true).height+obj.top-obj.getBoundingRect(true).top);
obj.left = Math.min(obj.left, obj.canvas.width/canvas.getZoom()-obj.getBoundingRect(true).width+obj.left-obj.getBoundingRect(true).left);
javascript html5 canvas fabricjs
I'm trying to limit the movement of an object only inside the canvas. This I have achieved with the following code
if(obj.getBoundingRect().top < 0 || obj.getBoundingRect().left< 0)
obj.top = Math.max(obj.top, obj.top-obj.getBoundingRect().top);
obj.left = Math.max(obj.left, obj.left-obj.getBoundingRect().left));
// bot-right corner
if(obj.getBoundingRect().top+obj.getBoundingRect().height > obj.canvas.height || obj.getBoundingRect().left+obj.getBoundingRect().width > obj.canvas.width)
obj.top = Math.min(obj.top, obj.canvas.height-obj.getBoundingRect().height+obj.top-obj.getBoundingRect().top);
obj.left = Math.min(obj.left, obj.canvas.width-obj.getBoundingRect().width+obj.left-obj.getBoundingRect().left);
This works well when I have no zoom applied to the canvas.
I need to be able to make it work also when I apply a zoom + or a zoom -
I had thought about trying to solve it like this
if(obj.getBoundingRect().top*canvas.getZoom() < 0 || obj.getBoundingRect().left*canvas.getZoom() < 0)
obj.top = Math.max(obj.top, obj.top-obj.getBoundingRect().top)*canvas.getZoom();
obj.left = Math.max(obj.left, obj.left-obj.getBoundingRect().left)*canvas.getZoom();
// bot-right corner
if(obj.getBoundingRect().top*canvas.getZoom()+obj.getBoundingRect().height > obj.canvas.height || obj.getBoundingRect().left*canvas.getZoom()+obj.getBoundingRect().width > obj.canvas.width)
obj.top = Math.min(obj.top, obj.canvas.height-obj.getBoundingRect().height+obj.top-obj.getBoundingRect().top)*canvas.getZoom();
obj.left = Math.min(obj.left, obj.canvas.width-obj.getBoundingRect().width+obj.left-obj.getBoundingRect().left)*canvas.getZoom();
But it does not work. Can you help me?
Edit: Add the fiddle
https://jsfiddle.net/samael205/m19cuk0j/1/
Edit: Sloved, that ONLY works with Fabric 2.0.0+
if(obj.getBoundingRect(true).top < 0 || obj.getBoundingRect(true).left < 0)
obj.top = Math.max(obj.top, obj.top-obj.getBoundingRect(true).top);
obj.left = Math.max(obj.left, obj.left-obj.getBoundingRect(true).left);
// bot-right corner
if(obj.getBoundingRect(true).top+obj.getBoundingRect(true).height > obj.canvas.height/canvas.getZoom() || obj.getBoundingRect(true).left+obj.getBoundingRect(true).width > obj.canvas.width/canvas.getZoom())
obj.top = Math.min(obj.top, obj.canvas.height/canvas.getZoom()-obj.getBoundingRect(true).height+obj.top-obj.getBoundingRect(true).top);
obj.left = Math.min(obj.left, obj.canvas.width/canvas.getZoom()-obj.getBoundingRect(true).width+obj.left-obj.getBoundingRect(true).left);
javascript html5 canvas fabricjs
javascript html5 canvas fabricjs
edited Dec 14 '18 at 7:04
Cœur
18.1k9108148
18.1k9108148
asked Jan 15 '18 at 17:02


Pedro JosePedro Jose
10511
10511
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Thanks, everything works fine, I rewrote a little, can it be useful to someone
canvas.on("object:moving", function(e)
var obj = e.target;
obj.setCoords();
var bound = obj.getBoundingRect(true);
var width = obj.canvas.width;
var height = obj.canvas.height;
obj.left = Math.min(Math.max(0, bound.left), width - bound.width);
obj.top = Math.min(Math.max(0, bound.top), height - bound.height);
)
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f48267587%2fmove-objecj-within-canvas-canvas-boundary-limit-with-zoom-applied%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks, everything works fine, I rewrote a little, can it be useful to someone
canvas.on("object:moving", function(e)
var obj = e.target;
obj.setCoords();
var bound = obj.getBoundingRect(true);
var width = obj.canvas.width;
var height = obj.canvas.height;
obj.left = Math.min(Math.max(0, bound.left), width - bound.width);
obj.top = Math.min(Math.max(0, bound.top), height - bound.height);
)
add a comment |
Thanks, everything works fine, I rewrote a little, can it be useful to someone
canvas.on("object:moving", function(e)
var obj = e.target;
obj.setCoords();
var bound = obj.getBoundingRect(true);
var width = obj.canvas.width;
var height = obj.canvas.height;
obj.left = Math.min(Math.max(0, bound.left), width - bound.width);
obj.top = Math.min(Math.max(0, bound.top), height - bound.height);
)
add a comment |
Thanks, everything works fine, I rewrote a little, can it be useful to someone
canvas.on("object:moving", function(e)
var obj = e.target;
obj.setCoords();
var bound = obj.getBoundingRect(true);
var width = obj.canvas.width;
var height = obj.canvas.height;
obj.left = Math.min(Math.max(0, bound.left), width - bound.width);
obj.top = Math.min(Math.max(0, bound.top), height - bound.height);
)
Thanks, everything works fine, I rewrote a little, can it be useful to someone
canvas.on("object:moving", function(e)
var obj = e.target;
obj.setCoords();
var bound = obj.getBoundingRect(true);
var width = obj.canvas.width;
var height = obj.canvas.height;
obj.left = Math.min(Math.max(0, bound.left), width - bound.width);
obj.top = Math.min(Math.max(0, bound.top), height - bound.height);
)
answered Nov 13 '18 at 12:57


Artem SinelnikovArtem Sinelnikov
186
186
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f48267587%2fmove-objecj-within-canvas-canvas-boundary-limit-with-zoom-applied%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
EoI1Exf3E4,I2INiq5eLz4y 0fukP7IYlONby7NYPcOP