Angular Material Date Picker not letting me fill in date
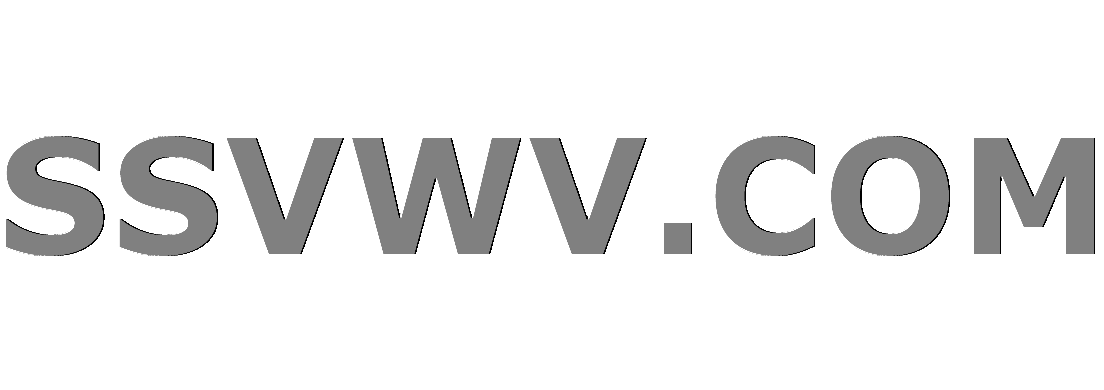
Multi tool use
Im using the Angular Material Datepicker.
Ive followed the tutorial here -> https://theinfogrid.com/tech/developers/angular/custom-date-formats-angular-material-date-picker/ because the Date picker was not allowing me to input a date.
THE PROBLEM
When the user starts typing in a date, the datepicker automatically enters in a date in 2018. It is then a hard experience fixing that date
SOLUTIONS IVE TRIED
Ive tried using all the types of Date pickers on the angular material guide. Ive tried the solution listed above. Im not sure why this is happening
THE CODE
<!--app.html file-->
<app-date-picker name="date-picker" placeholder="mm/DD/yyyy" [(ngModel)]="birthDate" format="MM/DD/YYYY"></app-date-picker>
//app.ts file
birthDate = moment();
<!--utility/date-picker/date-picker.html-->
<mat-form-field>
<mat-datepicker-toggle matPrefix [for]="picker"></mat-datepicker-toggle>
<input matInput name="date-picker" [matDatepicker]="picker" [value]="dateValue" (dateInput)="addEvent('input', $event)" [placeholder]="placeholder">
<mat-datepicker #picker></mat-datepicker>
</mat-form-field>
// utility/date-picker/date-picker.ts
import Component, forwardRef, Input from '@angular/core';
import ControlValueAccessor, NG_VALUE_ACCESSOR from '@angular/forms';
import MatDatepickerInputEvent from '@angular/material/datepicker';
import * as moment from 'moment';
@Component(
selector: 'app-date-picker',
templateUrl: './date-picker.component.html',
// styleUrls: ['./date-picker.component.css'],
providers: [
provide: NG_VALUE_ACCESSOR,
useExisting: forwardRef(() => DatePickerComponent),
multi: true
]
)
export class DatePickerComponent implements ControlValueAccessor
/**
* Set the value of the date set by user, notice the underscore infront of the datevalue
*/
@Input() _dateValue: string = null;
/**
* Placeholder value for the material control input
*/
@Input() public placeholder: string = null;
/**
* The date format to use with default format but allowing you to pass a custom date format
*/
@Input() private format = 'YYYY/MM/DD HH:mm:ss';
@Input() private isTouch: boolean = false;
get dateValue()
return moment(this._dateValue, this.format);
set dateValue(val)
this._dateValue = moment(val).format(this.format);
this.propagateChange(this._dateValue);
addEvent(type: string, event: MatDatepickerInputEvent<Date>)
this.dateValue = moment(event.value, this.format);
writeValue(value: any)
if (value !== undefined)
this.dateValue = moment(value, this.format);
propagateChange = (_: any) => ;
registerOnChange(fn)
this.propagateChange = fn;
registerOnTouched()
The module files follow the imports in the guide.
Is this happening to anyone else?
html

add a comment |
Im using the Angular Material Datepicker.
Ive followed the tutorial here -> https://theinfogrid.com/tech/developers/angular/custom-date-formats-angular-material-date-picker/ because the Date picker was not allowing me to input a date.
THE PROBLEM
When the user starts typing in a date, the datepicker automatically enters in a date in 2018. It is then a hard experience fixing that date
SOLUTIONS IVE TRIED
Ive tried using all the types of Date pickers on the angular material guide. Ive tried the solution listed above. Im not sure why this is happening
THE CODE
<!--app.html file-->
<app-date-picker name="date-picker" placeholder="mm/DD/yyyy" [(ngModel)]="birthDate" format="MM/DD/YYYY"></app-date-picker>
//app.ts file
birthDate = moment();
<!--utility/date-picker/date-picker.html-->
<mat-form-field>
<mat-datepicker-toggle matPrefix [for]="picker"></mat-datepicker-toggle>
<input matInput name="date-picker" [matDatepicker]="picker" [value]="dateValue" (dateInput)="addEvent('input', $event)" [placeholder]="placeholder">
<mat-datepicker #picker></mat-datepicker>
</mat-form-field>
// utility/date-picker/date-picker.ts
import Component, forwardRef, Input from '@angular/core';
import ControlValueAccessor, NG_VALUE_ACCESSOR from '@angular/forms';
import MatDatepickerInputEvent from '@angular/material/datepicker';
import * as moment from 'moment';
@Component(
selector: 'app-date-picker',
templateUrl: './date-picker.component.html',
// styleUrls: ['./date-picker.component.css'],
providers: [
provide: NG_VALUE_ACCESSOR,
useExisting: forwardRef(() => DatePickerComponent),
multi: true
]
)
export class DatePickerComponent implements ControlValueAccessor
/**
* Set the value of the date set by user, notice the underscore infront of the datevalue
*/
@Input() _dateValue: string = null;
/**
* Placeholder value for the material control input
*/
@Input() public placeholder: string = null;
/**
* The date format to use with default format but allowing you to pass a custom date format
*/
@Input() private format = 'YYYY/MM/DD HH:mm:ss';
@Input() private isTouch: boolean = false;
get dateValue()
return moment(this._dateValue, this.format);
set dateValue(val)
this._dateValue = moment(val).format(this.format);
this.propagateChange(this._dateValue);
addEvent(type: string, event: MatDatepickerInputEvent<Date>)
this.dateValue = moment(event.value, this.format);
writeValue(value: any)
if (value !== undefined)
this.dateValue = moment(value, this.format);
propagateChange = (_: any) => ;
registerOnChange(fn)
this.propagateChange = fn;
registerOnTouched()
The module files follow the imports in the guide.
Is this happening to anyone else?
html

Please create stackblitz demo.
– Sunil Singh
Nov 15 '18 at 7:23
add a comment |
Im using the Angular Material Datepicker.
Ive followed the tutorial here -> https://theinfogrid.com/tech/developers/angular/custom-date-formats-angular-material-date-picker/ because the Date picker was not allowing me to input a date.
THE PROBLEM
When the user starts typing in a date, the datepicker automatically enters in a date in 2018. It is then a hard experience fixing that date
SOLUTIONS IVE TRIED
Ive tried using all the types of Date pickers on the angular material guide. Ive tried the solution listed above. Im not sure why this is happening
THE CODE
<!--app.html file-->
<app-date-picker name="date-picker" placeholder="mm/DD/yyyy" [(ngModel)]="birthDate" format="MM/DD/YYYY"></app-date-picker>
//app.ts file
birthDate = moment();
<!--utility/date-picker/date-picker.html-->
<mat-form-field>
<mat-datepicker-toggle matPrefix [for]="picker"></mat-datepicker-toggle>
<input matInput name="date-picker" [matDatepicker]="picker" [value]="dateValue" (dateInput)="addEvent('input', $event)" [placeholder]="placeholder">
<mat-datepicker #picker></mat-datepicker>
</mat-form-field>
// utility/date-picker/date-picker.ts
import Component, forwardRef, Input from '@angular/core';
import ControlValueAccessor, NG_VALUE_ACCESSOR from '@angular/forms';
import MatDatepickerInputEvent from '@angular/material/datepicker';
import * as moment from 'moment';
@Component(
selector: 'app-date-picker',
templateUrl: './date-picker.component.html',
// styleUrls: ['./date-picker.component.css'],
providers: [
provide: NG_VALUE_ACCESSOR,
useExisting: forwardRef(() => DatePickerComponent),
multi: true
]
)
export class DatePickerComponent implements ControlValueAccessor
/**
* Set the value of the date set by user, notice the underscore infront of the datevalue
*/
@Input() _dateValue: string = null;
/**
* Placeholder value for the material control input
*/
@Input() public placeholder: string = null;
/**
* The date format to use with default format but allowing you to pass a custom date format
*/
@Input() private format = 'YYYY/MM/DD HH:mm:ss';
@Input() private isTouch: boolean = false;
get dateValue()
return moment(this._dateValue, this.format);
set dateValue(val)
this._dateValue = moment(val).format(this.format);
this.propagateChange(this._dateValue);
addEvent(type: string, event: MatDatepickerInputEvent<Date>)
this.dateValue = moment(event.value, this.format);
writeValue(value: any)
if (value !== undefined)
this.dateValue = moment(value, this.format);
propagateChange = (_: any) => ;
registerOnChange(fn)
this.propagateChange = fn;
registerOnTouched()
The module files follow the imports in the guide.
Is this happening to anyone else?
html

Im using the Angular Material Datepicker.
Ive followed the tutorial here -> https://theinfogrid.com/tech/developers/angular/custom-date-formats-angular-material-date-picker/ because the Date picker was not allowing me to input a date.
THE PROBLEM
When the user starts typing in a date, the datepicker automatically enters in a date in 2018. It is then a hard experience fixing that date
SOLUTIONS IVE TRIED
Ive tried using all the types of Date pickers on the angular material guide. Ive tried the solution listed above. Im not sure why this is happening
THE CODE
<!--app.html file-->
<app-date-picker name="date-picker" placeholder="mm/DD/yyyy" [(ngModel)]="birthDate" format="MM/DD/YYYY"></app-date-picker>
//app.ts file
birthDate = moment();
<!--utility/date-picker/date-picker.html-->
<mat-form-field>
<mat-datepicker-toggle matPrefix [for]="picker"></mat-datepicker-toggle>
<input matInput name="date-picker" [matDatepicker]="picker" [value]="dateValue" (dateInput)="addEvent('input', $event)" [placeholder]="placeholder">
<mat-datepicker #picker></mat-datepicker>
</mat-form-field>
// utility/date-picker/date-picker.ts
import Component, forwardRef, Input from '@angular/core';
import ControlValueAccessor, NG_VALUE_ACCESSOR from '@angular/forms';
import MatDatepickerInputEvent from '@angular/material/datepicker';
import * as moment from 'moment';
@Component(
selector: 'app-date-picker',
templateUrl: './date-picker.component.html',
// styleUrls: ['./date-picker.component.css'],
providers: [
provide: NG_VALUE_ACCESSOR,
useExisting: forwardRef(() => DatePickerComponent),
multi: true
]
)
export class DatePickerComponent implements ControlValueAccessor
/**
* Set the value of the date set by user, notice the underscore infront of the datevalue
*/
@Input() _dateValue: string = null;
/**
* Placeholder value for the material control input
*/
@Input() public placeholder: string = null;
/**
* The date format to use with default format but allowing you to pass a custom date format
*/
@Input() private format = 'YYYY/MM/DD HH:mm:ss';
@Input() private isTouch: boolean = false;
get dateValue()
return moment(this._dateValue, this.format);
set dateValue(val)
this._dateValue = moment(val).format(this.format);
this.propagateChange(this._dateValue);
addEvent(type: string, event: MatDatepickerInputEvent<Date>)
this.dateValue = moment(event.value, this.format);
writeValue(value: any)
if (value !== undefined)
this.dateValue = moment(value, this.format);
propagateChange = (_: any) => ;
registerOnChange(fn)
this.propagateChange = fn;
registerOnTouched()
The module files follow the imports in the guide.
Is this happening to anyone else?
html

html

asked Nov 15 '18 at 2:39


Jonathan CorrinJonathan Corrin
912221
912221
Please create stackblitz demo.
– Sunil Singh
Nov 15 '18 at 7:23
add a comment |
Please create stackblitz demo.
– Sunil Singh
Nov 15 '18 at 7:23
Please create stackblitz demo.
– Sunil Singh
Nov 15 '18 at 7:23
Please create stackblitz demo.
– Sunil Singh
Nov 15 '18 at 7:23
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53311639%2fangular-material-date-picker-not-letting-me-fill-in-date%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53311639%2fangular-material-date-picker-not-letting-me-fill-in-date%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
rZo2,l6o 9tLFwUvX,ML17imUFERPyiufyPe HPDYrkWrj8cpS30YhSeHrPzwhxng2qU,rT24Ig,PMK9GgA LZv0H9Rr13Cjlebr
Please create stackblitz demo.
– Sunil Singh
Nov 15 '18 at 7:23