“Train crossing mutex problem” - Getting NULL on last 2 lines
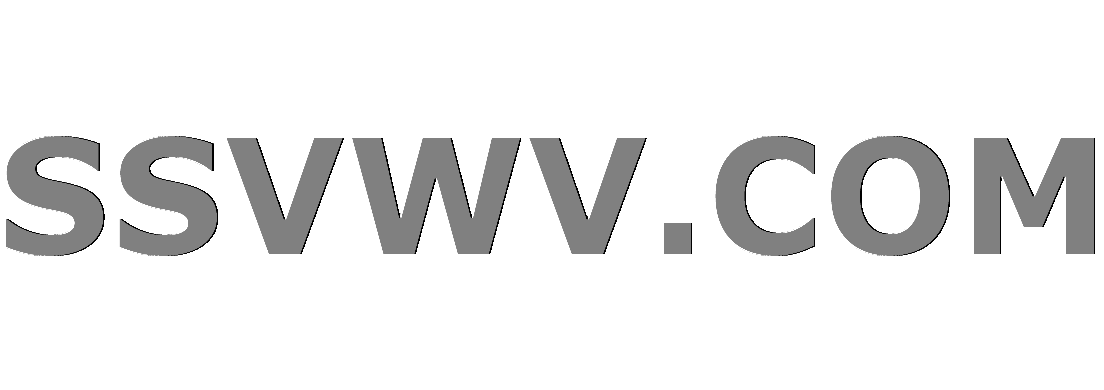
Multi tool use
Hey I'm having trouble solving a bug that causes some unwanted NULL's to be displayed in my final output. When you run the program enter a string such as 'nnseeeewwwew' into the command line before running.
Here is the code
#include <stdio.h>
#include <unistd.h>
#include <stdlib.h>
#include <pthread.h>
#include <semaphore.h>
#include <string.h>
/* mutex */
pthread_mutex_t thisMutex;
/* Condition Variables */
sem_t nQueue, eQueue, sQueue, wQueue;
int nFirst, sFirst, eFirst, wFirst;
int done = 0;
/* Thread Prototypes */
void *bats(void *);
void *checking(void *);
/* Prototypes */
void arrive(int num, char *dir);
void cross();
void leave(int num, char *dir);
void check();
/* Global pointer to argv */
char *directions = NULL;
int main(int argc, char *argv)
void *checking(void *arg)
while( done == 0 )
sFirst == 1
exit(0);
void *bats(void *arg)
int index = *(int *)arg;
char *dir;
switch (directions[index])
case 'n':
dir = "North";
break;
case 's':
dir = "South";
break;
case 'e':
dir = "East";
break;
case'w':
dir = "West";
break;
arrive(index, dir);
leave(index, dir);
_exit(0);
/* Functions */
void arrive(int num, char *dir)
printf("BAT %d from %s arrives at crossingn", num, dir);
if(strcmp(dir,"North")== 0)
sem_wait(&nQueue);
nFirst = 1;
while(eFirst == 1) /* do nothing */
cross();
nFirst = 0;
sem_post(&nQueue);
else if(strcmp(dir,"West")== 0)
sem_wait(&wQueue);
wFirst = 1;
while(nFirst == 1) /* do nothing */
cross();
wFirst = 0;
sem_post(&wQueue);
else if(strcmp(dir,"South")== 0)
sem_wait(&sQueue);
sFirst = 1;
while(wFirst == 1) /* do nothing */
cross();
sFirst = 0;
sem_post(&sQueue);
else if(strcmp(dir,"East")== 0)
sem_wait(&eQueue);
eFirst = 1;
while(sFirst == 1) /* do nothing */
cross();
eFirst= 0;
sem_post(&eQueue);
void cross()
pthread_mutex_lock(&thisMutex);
sleep(1);
pthread_mutex_unlock(&thisMutex);;
void leave(int num, char *dir)
printf("BAT %d from %s leaving crossingn", num, dir);
void check()
if( nFirst == 1 && sFirst == 1 &&
eFirst == 1 && wFirst == 1 )
eFirst = 0;
sleep(1);
eFirst = 1;
The goal of this assignment is to
BATs arriving from the same direction line up behind the first BAT already at the crossing;
BATs arriving from the right always have the right of way (unless the waiting BAT receives a signal to go);
Deadlock has to be prevented
Starvation has to be prevented
Once you run the code, you'll see the last two lines have 'NULL' for the direction the BAT is arriving from. Need help to solve that issue
Thanks!
c mutex
add a comment |
Hey I'm having trouble solving a bug that causes some unwanted NULL's to be displayed in my final output. When you run the program enter a string such as 'nnseeeewwwew' into the command line before running.
Here is the code
#include <stdio.h>
#include <unistd.h>
#include <stdlib.h>
#include <pthread.h>
#include <semaphore.h>
#include <string.h>
/* mutex */
pthread_mutex_t thisMutex;
/* Condition Variables */
sem_t nQueue, eQueue, sQueue, wQueue;
int nFirst, sFirst, eFirst, wFirst;
int done = 0;
/* Thread Prototypes */
void *bats(void *);
void *checking(void *);
/* Prototypes */
void arrive(int num, char *dir);
void cross();
void leave(int num, char *dir);
void check();
/* Global pointer to argv */
char *directions = NULL;
int main(int argc, char *argv)
void *checking(void *arg)
while( done == 0 )
sFirst == 1
exit(0);
void *bats(void *arg)
int index = *(int *)arg;
char *dir;
switch (directions[index])
case 'n':
dir = "North";
break;
case 's':
dir = "South";
break;
case 'e':
dir = "East";
break;
case'w':
dir = "West";
break;
arrive(index, dir);
leave(index, dir);
_exit(0);
/* Functions */
void arrive(int num, char *dir)
printf("BAT %d from %s arrives at crossingn", num, dir);
if(strcmp(dir,"North")== 0)
sem_wait(&nQueue);
nFirst = 1;
while(eFirst == 1) /* do nothing */
cross();
nFirst = 0;
sem_post(&nQueue);
else if(strcmp(dir,"West")== 0)
sem_wait(&wQueue);
wFirst = 1;
while(nFirst == 1) /* do nothing */
cross();
wFirst = 0;
sem_post(&wQueue);
else if(strcmp(dir,"South")== 0)
sem_wait(&sQueue);
sFirst = 1;
while(wFirst == 1) /* do nothing */
cross();
sFirst = 0;
sem_post(&sQueue);
else if(strcmp(dir,"East")== 0)
sem_wait(&eQueue);
eFirst = 1;
while(sFirst == 1) /* do nothing */
cross();
eFirst= 0;
sem_post(&eQueue);
void cross()
pthread_mutex_lock(&thisMutex);
sleep(1);
pthread_mutex_unlock(&thisMutex);;
void leave(int num, char *dir)
printf("BAT %d from %s leaving crossingn", num, dir);
void check()
if( nFirst == 1 && sFirst == 1 &&
eFirst == 1 && wFirst == 1 )
eFirst = 0;
sleep(1);
eFirst = 1;
The goal of this assignment is to
BATs arriving from the same direction line up behind the first BAT already at the crossing;
BATs arriving from the right always have the right of way (unless the waiting BAT receives a signal to go);
Deadlock has to be prevented
Starvation has to be prevented
Once you run the code, you'll see the last two lines have 'NULL' for the direction the BAT is arriving from. Need help to solve that issue
Thanks!
c mutex
dir
is achar
pointer. So you cannot directly use an equality sign likeif( dir == "North")
What you need isif ((strcmp(dir,"North")== 0)
– Rishikesh Raje
Nov 15 '18 at 5:01
I replaced the if statements, still getting NULL error
– BobbyWidThaTool
Nov 15 '18 at 5:13
add a comment |
Hey I'm having trouble solving a bug that causes some unwanted NULL's to be displayed in my final output. When you run the program enter a string such as 'nnseeeewwwew' into the command line before running.
Here is the code
#include <stdio.h>
#include <unistd.h>
#include <stdlib.h>
#include <pthread.h>
#include <semaphore.h>
#include <string.h>
/* mutex */
pthread_mutex_t thisMutex;
/* Condition Variables */
sem_t nQueue, eQueue, sQueue, wQueue;
int nFirst, sFirst, eFirst, wFirst;
int done = 0;
/* Thread Prototypes */
void *bats(void *);
void *checking(void *);
/* Prototypes */
void arrive(int num, char *dir);
void cross();
void leave(int num, char *dir);
void check();
/* Global pointer to argv */
char *directions = NULL;
int main(int argc, char *argv)
void *checking(void *arg)
while( done == 0 )
sFirst == 1
exit(0);
void *bats(void *arg)
int index = *(int *)arg;
char *dir;
switch (directions[index])
case 'n':
dir = "North";
break;
case 's':
dir = "South";
break;
case 'e':
dir = "East";
break;
case'w':
dir = "West";
break;
arrive(index, dir);
leave(index, dir);
_exit(0);
/* Functions */
void arrive(int num, char *dir)
printf("BAT %d from %s arrives at crossingn", num, dir);
if(strcmp(dir,"North")== 0)
sem_wait(&nQueue);
nFirst = 1;
while(eFirst == 1) /* do nothing */
cross();
nFirst = 0;
sem_post(&nQueue);
else if(strcmp(dir,"West")== 0)
sem_wait(&wQueue);
wFirst = 1;
while(nFirst == 1) /* do nothing */
cross();
wFirst = 0;
sem_post(&wQueue);
else if(strcmp(dir,"South")== 0)
sem_wait(&sQueue);
sFirst = 1;
while(wFirst == 1) /* do nothing */
cross();
sFirst = 0;
sem_post(&sQueue);
else if(strcmp(dir,"East")== 0)
sem_wait(&eQueue);
eFirst = 1;
while(sFirst == 1) /* do nothing */
cross();
eFirst= 0;
sem_post(&eQueue);
void cross()
pthread_mutex_lock(&thisMutex);
sleep(1);
pthread_mutex_unlock(&thisMutex);;
void leave(int num, char *dir)
printf("BAT %d from %s leaving crossingn", num, dir);
void check()
if( nFirst == 1 && sFirst == 1 &&
eFirst == 1 && wFirst == 1 )
eFirst = 0;
sleep(1);
eFirst = 1;
The goal of this assignment is to
BATs arriving from the same direction line up behind the first BAT already at the crossing;
BATs arriving from the right always have the right of way (unless the waiting BAT receives a signal to go);
Deadlock has to be prevented
Starvation has to be prevented
Once you run the code, you'll see the last two lines have 'NULL' for the direction the BAT is arriving from. Need help to solve that issue
Thanks!
c mutex
Hey I'm having trouble solving a bug that causes some unwanted NULL's to be displayed in my final output. When you run the program enter a string such as 'nnseeeewwwew' into the command line before running.
Here is the code
#include <stdio.h>
#include <unistd.h>
#include <stdlib.h>
#include <pthread.h>
#include <semaphore.h>
#include <string.h>
/* mutex */
pthread_mutex_t thisMutex;
/* Condition Variables */
sem_t nQueue, eQueue, sQueue, wQueue;
int nFirst, sFirst, eFirst, wFirst;
int done = 0;
/* Thread Prototypes */
void *bats(void *);
void *checking(void *);
/* Prototypes */
void arrive(int num, char *dir);
void cross();
void leave(int num, char *dir);
void check();
/* Global pointer to argv */
char *directions = NULL;
int main(int argc, char *argv)
void *checking(void *arg)
while( done == 0 )
sFirst == 1
exit(0);
void *bats(void *arg)
int index = *(int *)arg;
char *dir;
switch (directions[index])
case 'n':
dir = "North";
break;
case 's':
dir = "South";
break;
case 'e':
dir = "East";
break;
case'w':
dir = "West";
break;
arrive(index, dir);
leave(index, dir);
_exit(0);
/* Functions */
void arrive(int num, char *dir)
printf("BAT %d from %s arrives at crossingn", num, dir);
if(strcmp(dir,"North")== 0)
sem_wait(&nQueue);
nFirst = 1;
while(eFirst == 1) /* do nothing */
cross();
nFirst = 0;
sem_post(&nQueue);
else if(strcmp(dir,"West")== 0)
sem_wait(&wQueue);
wFirst = 1;
while(nFirst == 1) /* do nothing */
cross();
wFirst = 0;
sem_post(&wQueue);
else if(strcmp(dir,"South")== 0)
sem_wait(&sQueue);
sFirst = 1;
while(wFirst == 1) /* do nothing */
cross();
sFirst = 0;
sem_post(&sQueue);
else if(strcmp(dir,"East")== 0)
sem_wait(&eQueue);
eFirst = 1;
while(sFirst == 1) /* do nothing */
cross();
eFirst= 0;
sem_post(&eQueue);
void cross()
pthread_mutex_lock(&thisMutex);
sleep(1);
pthread_mutex_unlock(&thisMutex);;
void leave(int num, char *dir)
printf("BAT %d from %s leaving crossingn", num, dir);
void check()
if( nFirst == 1 && sFirst == 1 &&
eFirst == 1 && wFirst == 1 )
eFirst = 0;
sleep(1);
eFirst = 1;
The goal of this assignment is to
BATs arriving from the same direction line up behind the first BAT already at the crossing;
BATs arriving from the right always have the right of way (unless the waiting BAT receives a signal to go);
Deadlock has to be prevented
Starvation has to be prevented
Once you run the code, you'll see the last two lines have 'NULL' for the direction the BAT is arriving from. Need help to solve that issue
Thanks!
c mutex
c mutex
edited Nov 15 '18 at 5:34
BobbyWidThaTool
asked Nov 15 '18 at 2:46


BobbyWidThaToolBobbyWidThaTool
427
427
dir
is achar
pointer. So you cannot directly use an equality sign likeif( dir == "North")
What you need isif ((strcmp(dir,"North")== 0)
– Rishikesh Raje
Nov 15 '18 at 5:01
I replaced the if statements, still getting NULL error
– BobbyWidThaTool
Nov 15 '18 at 5:13
add a comment |
dir
is achar
pointer. So you cannot directly use an equality sign likeif( dir == "North")
What you need isif ((strcmp(dir,"North")== 0)
– Rishikesh Raje
Nov 15 '18 at 5:01
I replaced the if statements, still getting NULL error
– BobbyWidThaTool
Nov 15 '18 at 5:13
dir
is a char
pointer. So you cannot directly use an equality sign like if( dir == "North")
What you need is if ((strcmp(dir,"North")== 0)
– Rishikesh Raje
Nov 15 '18 at 5:01
dir
is a char
pointer. So you cannot directly use an equality sign like if( dir == "North")
What you need is if ((strcmp(dir,"North")== 0)
– Rishikesh Raje
Nov 15 '18 at 5:01
I replaced the if statements, still getting NULL error
– BobbyWidThaTool
Nov 15 '18 at 5:13
I replaced the if statements, still getting NULL error
– BobbyWidThaTool
Nov 15 '18 at 5:13
add a comment |
2 Answers
2
active
oldest
votes
Your program has undefined behaviour becasue you are passing pointer to local variable into your thread function. In bats
function when dereferencing pointer you may get garbage value:
for(int j = 0; j < i; j++)
pthread_create(&tid[j], NULL, bats, (void*) &j);
j
is local inside for loop.
You could allocate memory to hold value for int
variable:
for(int j = 0; j < i; j++)
int* var = malloc(sizeof(int));
*var = j;
pthread_create(&tid[j], NULL, bats, var);
remember to free this memory in bats
function:
void *bats(void *arg)
int index = *(int *)arg;
//...
leave(index, dir);
free (arg); // <--
pthread_exit(NULL); // instead of _exit(0);
Thank you! Solved the null issue. I'm seeing the last line of the program saying "BAT 0 from North leaving crossing" Any reason why 0 is waiting until the very end to leave?
– BobbyWidThaTool
Nov 15 '18 at 6:28
@BobbyWidThaTool_exit(0)
close your program while joining at first thread, you should callpthread_exit(NULL)
instead of _exit(0).
– rafix07
Nov 15 '18 at 6:50
you're a legend. Thanks! Running perfect now. Man I wish I was good at C
– BobbyWidThaTool
Nov 15 '18 at 7:01
add a comment |
dir
is a char pointer. So you cannot directly use an equality sign likeif( dir == "North")
What you need isif ((strcmp(dir,"North")== 0)
in the loop below,
while( argv[1][i] == 'n' || argv[1][i] == 'e' ||
argv[1][i] == 's' || argv[1][i] == 'w' )
i++;
if(argv[1][i] == 'n')n++;
if(argv[1][i] == 's')s++;
if(argv[1][i] == 'e')e++;
if(argv[1][i] == 'w')w++;
You are incrementing i
first inside the loop. So, for the last element in the array argv[1][i]
you are reading junk values. The increment of i
should be the last statement in the loop. i.e. after if(argv[1][i] == 'w')w++;
Made these changes, now I still get NULL on the last 'arrive' statement and segmentation fault after that
– BobbyWidThaTool
Nov 15 '18 at 5:21
It is working for me..
– Rishikesh Raje
Nov 15 '18 at 5:25
ok I pasted my new code in the original post. See if I still have a mistake in there please, thanks!
– BobbyWidThaTool
Nov 15 '18 at 5:34
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53311694%2ftrain-crossing-mutex-problem-getting-null-on-last-2-lines%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Your program has undefined behaviour becasue you are passing pointer to local variable into your thread function. In bats
function when dereferencing pointer you may get garbage value:
for(int j = 0; j < i; j++)
pthread_create(&tid[j], NULL, bats, (void*) &j);
j
is local inside for loop.
You could allocate memory to hold value for int
variable:
for(int j = 0; j < i; j++)
int* var = malloc(sizeof(int));
*var = j;
pthread_create(&tid[j], NULL, bats, var);
remember to free this memory in bats
function:
void *bats(void *arg)
int index = *(int *)arg;
//...
leave(index, dir);
free (arg); // <--
pthread_exit(NULL); // instead of _exit(0);
Thank you! Solved the null issue. I'm seeing the last line of the program saying "BAT 0 from North leaving crossing" Any reason why 0 is waiting until the very end to leave?
– BobbyWidThaTool
Nov 15 '18 at 6:28
@BobbyWidThaTool_exit(0)
close your program while joining at first thread, you should callpthread_exit(NULL)
instead of _exit(0).
– rafix07
Nov 15 '18 at 6:50
you're a legend. Thanks! Running perfect now. Man I wish I was good at C
– BobbyWidThaTool
Nov 15 '18 at 7:01
add a comment |
Your program has undefined behaviour becasue you are passing pointer to local variable into your thread function. In bats
function when dereferencing pointer you may get garbage value:
for(int j = 0; j < i; j++)
pthread_create(&tid[j], NULL, bats, (void*) &j);
j
is local inside for loop.
You could allocate memory to hold value for int
variable:
for(int j = 0; j < i; j++)
int* var = malloc(sizeof(int));
*var = j;
pthread_create(&tid[j], NULL, bats, var);
remember to free this memory in bats
function:
void *bats(void *arg)
int index = *(int *)arg;
//...
leave(index, dir);
free (arg); // <--
pthread_exit(NULL); // instead of _exit(0);
Thank you! Solved the null issue. I'm seeing the last line of the program saying "BAT 0 from North leaving crossing" Any reason why 0 is waiting until the very end to leave?
– BobbyWidThaTool
Nov 15 '18 at 6:28
@BobbyWidThaTool_exit(0)
close your program while joining at first thread, you should callpthread_exit(NULL)
instead of _exit(0).
– rafix07
Nov 15 '18 at 6:50
you're a legend. Thanks! Running perfect now. Man I wish I was good at C
– BobbyWidThaTool
Nov 15 '18 at 7:01
add a comment |
Your program has undefined behaviour becasue you are passing pointer to local variable into your thread function. In bats
function when dereferencing pointer you may get garbage value:
for(int j = 0; j < i; j++)
pthread_create(&tid[j], NULL, bats, (void*) &j);
j
is local inside for loop.
You could allocate memory to hold value for int
variable:
for(int j = 0; j < i; j++)
int* var = malloc(sizeof(int));
*var = j;
pthread_create(&tid[j], NULL, bats, var);
remember to free this memory in bats
function:
void *bats(void *arg)
int index = *(int *)arg;
//...
leave(index, dir);
free (arg); // <--
pthread_exit(NULL); // instead of _exit(0);
Your program has undefined behaviour becasue you are passing pointer to local variable into your thread function. In bats
function when dereferencing pointer you may get garbage value:
for(int j = 0; j < i; j++)
pthread_create(&tid[j], NULL, bats, (void*) &j);
j
is local inside for loop.
You could allocate memory to hold value for int
variable:
for(int j = 0; j < i; j++)
int* var = malloc(sizeof(int));
*var = j;
pthread_create(&tid[j], NULL, bats, var);
remember to free this memory in bats
function:
void *bats(void *arg)
int index = *(int *)arg;
//...
leave(index, dir);
free (arg); // <--
pthread_exit(NULL); // instead of _exit(0);
edited Nov 15 '18 at 6:52
answered Nov 15 '18 at 6:06
rafix07rafix07
8,4281916
8,4281916
Thank you! Solved the null issue. I'm seeing the last line of the program saying "BAT 0 from North leaving crossing" Any reason why 0 is waiting until the very end to leave?
– BobbyWidThaTool
Nov 15 '18 at 6:28
@BobbyWidThaTool_exit(0)
close your program while joining at first thread, you should callpthread_exit(NULL)
instead of _exit(0).
– rafix07
Nov 15 '18 at 6:50
you're a legend. Thanks! Running perfect now. Man I wish I was good at C
– BobbyWidThaTool
Nov 15 '18 at 7:01
add a comment |
Thank you! Solved the null issue. I'm seeing the last line of the program saying "BAT 0 from North leaving crossing" Any reason why 0 is waiting until the very end to leave?
– BobbyWidThaTool
Nov 15 '18 at 6:28
@BobbyWidThaTool_exit(0)
close your program while joining at first thread, you should callpthread_exit(NULL)
instead of _exit(0).
– rafix07
Nov 15 '18 at 6:50
you're a legend. Thanks! Running perfect now. Man I wish I was good at C
– BobbyWidThaTool
Nov 15 '18 at 7:01
Thank you! Solved the null issue. I'm seeing the last line of the program saying "BAT 0 from North leaving crossing" Any reason why 0 is waiting until the very end to leave?
– BobbyWidThaTool
Nov 15 '18 at 6:28
Thank you! Solved the null issue. I'm seeing the last line of the program saying "BAT 0 from North leaving crossing" Any reason why 0 is waiting until the very end to leave?
– BobbyWidThaTool
Nov 15 '18 at 6:28
@BobbyWidThaTool
_exit(0)
close your program while joining at first thread, you should call pthread_exit(NULL)
instead of _exit(0).– rafix07
Nov 15 '18 at 6:50
@BobbyWidThaTool
_exit(0)
close your program while joining at first thread, you should call pthread_exit(NULL)
instead of _exit(0).– rafix07
Nov 15 '18 at 6:50
you're a legend. Thanks! Running perfect now. Man I wish I was good at C
– BobbyWidThaTool
Nov 15 '18 at 7:01
you're a legend. Thanks! Running perfect now. Man I wish I was good at C
– BobbyWidThaTool
Nov 15 '18 at 7:01
add a comment |
dir
is a char pointer. So you cannot directly use an equality sign likeif( dir == "North")
What you need isif ((strcmp(dir,"North")== 0)
in the loop below,
while( argv[1][i] == 'n' || argv[1][i] == 'e' ||
argv[1][i] == 's' || argv[1][i] == 'w' )
i++;
if(argv[1][i] == 'n')n++;
if(argv[1][i] == 's')s++;
if(argv[1][i] == 'e')e++;
if(argv[1][i] == 'w')w++;
You are incrementing i
first inside the loop. So, for the last element in the array argv[1][i]
you are reading junk values. The increment of i
should be the last statement in the loop. i.e. after if(argv[1][i] == 'w')w++;
Made these changes, now I still get NULL on the last 'arrive' statement and segmentation fault after that
– BobbyWidThaTool
Nov 15 '18 at 5:21
It is working for me..
– Rishikesh Raje
Nov 15 '18 at 5:25
ok I pasted my new code in the original post. See if I still have a mistake in there please, thanks!
– BobbyWidThaTool
Nov 15 '18 at 5:34
add a comment |
dir
is a char pointer. So you cannot directly use an equality sign likeif( dir == "North")
What you need isif ((strcmp(dir,"North")== 0)
in the loop below,
while( argv[1][i] == 'n' || argv[1][i] == 'e' ||
argv[1][i] == 's' || argv[1][i] == 'w' )
i++;
if(argv[1][i] == 'n')n++;
if(argv[1][i] == 's')s++;
if(argv[1][i] == 'e')e++;
if(argv[1][i] == 'w')w++;
You are incrementing i
first inside the loop. So, for the last element in the array argv[1][i]
you are reading junk values. The increment of i
should be the last statement in the loop. i.e. after if(argv[1][i] == 'w')w++;
Made these changes, now I still get NULL on the last 'arrive' statement and segmentation fault after that
– BobbyWidThaTool
Nov 15 '18 at 5:21
It is working for me..
– Rishikesh Raje
Nov 15 '18 at 5:25
ok I pasted my new code in the original post. See if I still have a mistake in there please, thanks!
– BobbyWidThaTool
Nov 15 '18 at 5:34
add a comment |
dir
is a char pointer. So you cannot directly use an equality sign likeif( dir == "North")
What you need isif ((strcmp(dir,"North")== 0)
in the loop below,
while( argv[1][i] == 'n' || argv[1][i] == 'e' ||
argv[1][i] == 's' || argv[1][i] == 'w' )
i++;
if(argv[1][i] == 'n')n++;
if(argv[1][i] == 's')s++;
if(argv[1][i] == 'e')e++;
if(argv[1][i] == 'w')w++;
You are incrementing i
first inside the loop. So, for the last element in the array argv[1][i]
you are reading junk values. The increment of i
should be the last statement in the loop. i.e. after if(argv[1][i] == 'w')w++;
dir
is a char pointer. So you cannot directly use an equality sign likeif( dir == "North")
What you need isif ((strcmp(dir,"North")== 0)
in the loop below,
while( argv[1][i] == 'n' || argv[1][i] == 'e' ||
argv[1][i] == 's' || argv[1][i] == 'w' )
i++;
if(argv[1][i] == 'n')n++;
if(argv[1][i] == 's')s++;
if(argv[1][i] == 'e')e++;
if(argv[1][i] == 'w')w++;
You are incrementing i
first inside the loop. So, for the last element in the array argv[1][i]
you are reading junk values. The increment of i
should be the last statement in the loop. i.e. after if(argv[1][i] == 'w')w++;
answered Nov 15 '18 at 5:13
Rishikesh RajeRishikesh Raje
5,6071828
5,6071828
Made these changes, now I still get NULL on the last 'arrive' statement and segmentation fault after that
– BobbyWidThaTool
Nov 15 '18 at 5:21
It is working for me..
– Rishikesh Raje
Nov 15 '18 at 5:25
ok I pasted my new code in the original post. See if I still have a mistake in there please, thanks!
– BobbyWidThaTool
Nov 15 '18 at 5:34
add a comment |
Made these changes, now I still get NULL on the last 'arrive' statement and segmentation fault after that
– BobbyWidThaTool
Nov 15 '18 at 5:21
It is working for me..
– Rishikesh Raje
Nov 15 '18 at 5:25
ok I pasted my new code in the original post. See if I still have a mistake in there please, thanks!
– BobbyWidThaTool
Nov 15 '18 at 5:34
Made these changes, now I still get NULL on the last 'arrive' statement and segmentation fault after that
– BobbyWidThaTool
Nov 15 '18 at 5:21
Made these changes, now I still get NULL on the last 'arrive' statement and segmentation fault after that
– BobbyWidThaTool
Nov 15 '18 at 5:21
It is working for me..
– Rishikesh Raje
Nov 15 '18 at 5:25
It is working for me..
– Rishikesh Raje
Nov 15 '18 at 5:25
ok I pasted my new code in the original post. See if I still have a mistake in there please, thanks!
– BobbyWidThaTool
Nov 15 '18 at 5:34
ok I pasted my new code in the original post. See if I still have a mistake in there please, thanks!
– BobbyWidThaTool
Nov 15 '18 at 5:34
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53311694%2ftrain-crossing-mutex-problem-getting-null-on-last-2-lines%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
4coBJ3C6yT XLOGtZjBKMVl4Gj
dir
is achar
pointer. So you cannot directly use an equality sign likeif( dir == "North")
What you need isif ((strcmp(dir,"North")== 0)
– Rishikesh Raje
Nov 15 '18 at 5:01
I replaced the if statements, still getting NULL error
– BobbyWidThaTool
Nov 15 '18 at 5:13