How to get the returned reference to a vector?
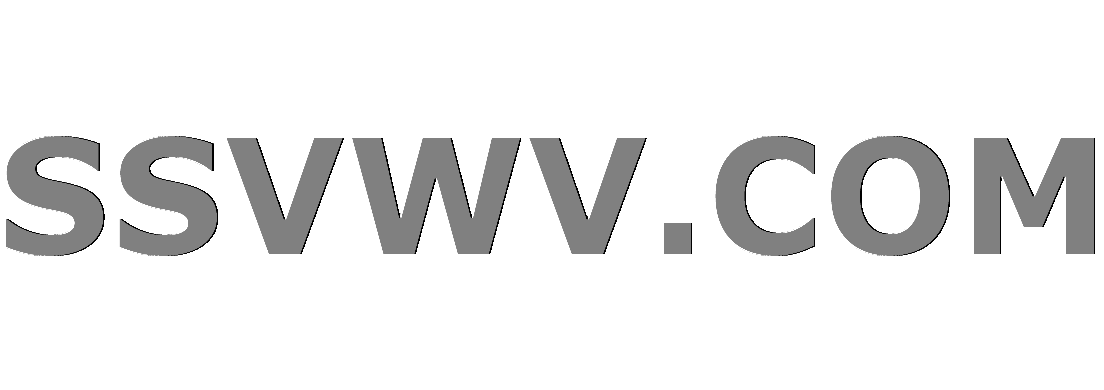
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty height:90px;width:728px;box-sizing:border-box;
I found two ways to get the reference returned by the function.
vector<int> vec1 = 4,5,6;
vector<int>& rtn_vec(void)
return vec1;
int main()
vector<int> &vec2 = rtn_vec(); //way 1
vector<int> vec3 = rtn_vec(); //way2
vec2[0] = 3;
return 0;
I understand way 1 means passing the reference to vec1
to &vec2
, so vec2[0] = 3;
changes vec1
to 3,5,6
.
But about way 2, I have 2 questions:
Why can I pass a reference (
vector<int>&
) to an instance (vector<int>
), how does it work?Does way 2 involve deep copy? Because I run this code and
vector<int> vec3 = rtn_vec();
seems just copyvec1
tovec3
.
c++ reference return-value
add a comment |
I found two ways to get the reference returned by the function.
vector<int> vec1 = 4,5,6;
vector<int>& rtn_vec(void)
return vec1;
int main()
vector<int> &vec2 = rtn_vec(); //way 1
vector<int> vec3 = rtn_vec(); //way2
vec2[0] = 3;
return 0;
I understand way 1 means passing the reference to vec1
to &vec2
, so vec2[0] = 3;
changes vec1
to 3,5,6
.
But about way 2, I have 2 questions:
Why can I pass a reference (
vector<int>&
) to an instance (vector<int>
), how does it work?Does way 2 involve deep copy? Because I run this code and
vector<int> vec3 = rtn_vec();
seems just copyvec1
tovec3
.
c++ reference return-value
1
Since the function returns a reference tovec1
, way 1 is equivalent tovector<int>& vec2 = vec1;
, way 2 is equivalent tovector<int> vec3 = vec1;
.
– molbdnilo
Nov 15 '18 at 15:23
add a comment |
I found two ways to get the reference returned by the function.
vector<int> vec1 = 4,5,6;
vector<int>& rtn_vec(void)
return vec1;
int main()
vector<int> &vec2 = rtn_vec(); //way 1
vector<int> vec3 = rtn_vec(); //way2
vec2[0] = 3;
return 0;
I understand way 1 means passing the reference to vec1
to &vec2
, so vec2[0] = 3;
changes vec1
to 3,5,6
.
But about way 2, I have 2 questions:
Why can I pass a reference (
vector<int>&
) to an instance (vector<int>
), how does it work?Does way 2 involve deep copy? Because I run this code and
vector<int> vec3 = rtn_vec();
seems just copyvec1
tovec3
.
c++ reference return-value
I found two ways to get the reference returned by the function.
vector<int> vec1 = 4,5,6;
vector<int>& rtn_vec(void)
return vec1;
int main()
vector<int> &vec2 = rtn_vec(); //way 1
vector<int> vec3 = rtn_vec(); //way2
vec2[0] = 3;
return 0;
I understand way 1 means passing the reference to vec1
to &vec2
, so vec2[0] = 3;
changes vec1
to 3,5,6
.
But about way 2, I have 2 questions:
Why can I pass a reference (
vector<int>&
) to an instance (vector<int>
), how does it work?Does way 2 involve deep copy? Because I run this code and
vector<int> vec3 = rtn_vec();
seems just copyvec1
tovec3
.
c++ reference return-value
c++ reference return-value
edited Nov 15 '18 at 15:16


Toby Speight
17.6k134469
17.6k134469
asked Nov 15 '18 at 15:12


Wei-Shou YangWei-Shou Yang
61
61
1
Since the function returns a reference tovec1
, way 1 is equivalent tovector<int>& vec2 = vec1;
, way 2 is equivalent tovector<int> vec3 = vec1;
.
– molbdnilo
Nov 15 '18 at 15:23
add a comment |
1
Since the function returns a reference tovec1
, way 1 is equivalent tovector<int>& vec2 = vec1;
, way 2 is equivalent tovector<int> vec3 = vec1;
.
– molbdnilo
Nov 15 '18 at 15:23
1
1
Since the function returns a reference to
vec1
, way 1 is equivalent to vector<int>& vec2 = vec1;
, way 2 is equivalent to vector<int> vec3 = vec1;
.– molbdnilo
Nov 15 '18 at 15:23
Since the function returns a reference to
vec1
, way 1 is equivalent to vector<int>& vec2 = vec1;
, way 2 is equivalent to vector<int> vec3 = vec1;
.– molbdnilo
Nov 15 '18 at 15:23
add a comment |
2 Answers
2
active
oldest
votes
vector<int> vec3 = rtn_vec(); //way2
This allocates a new vector and invokes a copy constructor, so yes, this is "deep" copy.
Actually, this is in no way different from simply writing
vector<int> &vec2 = vec1;
vector<int> vec3 = vec1;
Or to make things even clearer
vector<int> &return_value = vec1;
vector<int> &vec2 = return_value;
vector<int> vec3 = return_value;
(Though be careful with term "deep". If it was vector<int*>
, then only the pointers would be copied, not the int
s themselves.)
Thanks for explanation, but I still don't know why can I dovector<int> &return_value = vec1; vector<int> vec3 = return_value;
Becausereturn_value
is a reference, butvec3
is not a reference. I thought reference is similar to pointer, but I can't doint a; int *p = &a; int b = p;
this cause build error, so why can I do this while using reference ?
– Wei-Shou Yang
Nov 17 '18 at 2:30
@Wei-ShouYang, in your example instead ofint b = p;
you should doint b = *p
. References are somewhat like pointers (and technically are pointers), just you don't need to use&
for address and*
for accessing the value.
– Petr
Nov 17 '18 at 6:26
add a comment |
When you copy-construct vec3
, a shallow copy is made (C++ doesn't really do "deep" copy). All the elements in the vector are copied by value, just as with any other copy of a std::vector
.
I don't see how you could copyvec
and more "deeply" than what happens withvector<int> vec3 = rtn_vec();
. It's not a vector of pointers.
– François Andrieux
Nov 15 '18 at 15:31
No, but if it was a vector of pointers, then the pointed-to values wouldn't be copied. Hence, it's a shallow copy.
– Toby Speight
Nov 15 '18 at 15:33
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53322459%2fhow-to-get-the-returned-reference-to-a-vector%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
vector<int> vec3 = rtn_vec(); //way2
This allocates a new vector and invokes a copy constructor, so yes, this is "deep" copy.
Actually, this is in no way different from simply writing
vector<int> &vec2 = vec1;
vector<int> vec3 = vec1;
Or to make things even clearer
vector<int> &return_value = vec1;
vector<int> &vec2 = return_value;
vector<int> vec3 = return_value;
(Though be careful with term "deep". If it was vector<int*>
, then only the pointers would be copied, not the int
s themselves.)
Thanks for explanation, but I still don't know why can I dovector<int> &return_value = vec1; vector<int> vec3 = return_value;
Becausereturn_value
is a reference, butvec3
is not a reference. I thought reference is similar to pointer, but I can't doint a; int *p = &a; int b = p;
this cause build error, so why can I do this while using reference ?
– Wei-Shou Yang
Nov 17 '18 at 2:30
@Wei-ShouYang, in your example instead ofint b = p;
you should doint b = *p
. References are somewhat like pointers (and technically are pointers), just you don't need to use&
for address and*
for accessing the value.
– Petr
Nov 17 '18 at 6:26
add a comment |
vector<int> vec3 = rtn_vec(); //way2
This allocates a new vector and invokes a copy constructor, so yes, this is "deep" copy.
Actually, this is in no way different from simply writing
vector<int> &vec2 = vec1;
vector<int> vec3 = vec1;
Or to make things even clearer
vector<int> &return_value = vec1;
vector<int> &vec2 = return_value;
vector<int> vec3 = return_value;
(Though be careful with term "deep". If it was vector<int*>
, then only the pointers would be copied, not the int
s themselves.)
Thanks for explanation, but I still don't know why can I dovector<int> &return_value = vec1; vector<int> vec3 = return_value;
Becausereturn_value
is a reference, butvec3
is not a reference. I thought reference is similar to pointer, but I can't doint a; int *p = &a; int b = p;
this cause build error, so why can I do this while using reference ?
– Wei-Shou Yang
Nov 17 '18 at 2:30
@Wei-ShouYang, in your example instead ofint b = p;
you should doint b = *p
. References are somewhat like pointers (and technically are pointers), just you don't need to use&
for address and*
for accessing the value.
– Petr
Nov 17 '18 at 6:26
add a comment |
vector<int> vec3 = rtn_vec(); //way2
This allocates a new vector and invokes a copy constructor, so yes, this is "deep" copy.
Actually, this is in no way different from simply writing
vector<int> &vec2 = vec1;
vector<int> vec3 = vec1;
Or to make things even clearer
vector<int> &return_value = vec1;
vector<int> &vec2 = return_value;
vector<int> vec3 = return_value;
(Though be careful with term "deep". If it was vector<int*>
, then only the pointers would be copied, not the int
s themselves.)
vector<int> vec3 = rtn_vec(); //way2
This allocates a new vector and invokes a copy constructor, so yes, this is "deep" copy.
Actually, this is in no way different from simply writing
vector<int> &vec2 = vec1;
vector<int> vec3 = vec1;
Or to make things even clearer
vector<int> &return_value = vec1;
vector<int> &vec2 = return_value;
vector<int> vec3 = return_value;
(Though be careful with term "deep". If it was vector<int*>
, then only the pointers would be copied, not the int
s themselves.)
edited Nov 16 '18 at 6:32
answered Nov 15 '18 at 15:14
PetrPetr
8,28711843
8,28711843
Thanks for explanation, but I still don't know why can I dovector<int> &return_value = vec1; vector<int> vec3 = return_value;
Becausereturn_value
is a reference, butvec3
is not a reference. I thought reference is similar to pointer, but I can't doint a; int *p = &a; int b = p;
this cause build error, so why can I do this while using reference ?
– Wei-Shou Yang
Nov 17 '18 at 2:30
@Wei-ShouYang, in your example instead ofint b = p;
you should doint b = *p
. References are somewhat like pointers (and technically are pointers), just you don't need to use&
for address and*
for accessing the value.
– Petr
Nov 17 '18 at 6:26
add a comment |
Thanks for explanation, but I still don't know why can I dovector<int> &return_value = vec1; vector<int> vec3 = return_value;
Becausereturn_value
is a reference, butvec3
is not a reference. I thought reference is similar to pointer, but I can't doint a; int *p = &a; int b = p;
this cause build error, so why can I do this while using reference ?
– Wei-Shou Yang
Nov 17 '18 at 2:30
@Wei-ShouYang, in your example instead ofint b = p;
you should doint b = *p
. References are somewhat like pointers (and technically are pointers), just you don't need to use&
for address and*
for accessing the value.
– Petr
Nov 17 '18 at 6:26
Thanks for explanation, but I still don't know why can I do
vector<int> &return_value = vec1; vector<int> vec3 = return_value;
Because return_value
is a reference, but vec3
is not a reference. I thought reference is similar to pointer, but I can't do int a; int *p = &a; int b = p;
this cause build error, so why can I do this while using reference ?– Wei-Shou Yang
Nov 17 '18 at 2:30
Thanks for explanation, but I still don't know why can I do
vector<int> &return_value = vec1; vector<int> vec3 = return_value;
Because return_value
is a reference, but vec3
is not a reference. I thought reference is similar to pointer, but I can't do int a; int *p = &a; int b = p;
this cause build error, so why can I do this while using reference ?– Wei-Shou Yang
Nov 17 '18 at 2:30
@Wei-ShouYang, in your example instead of
int b = p;
you should do int b = *p
. References are somewhat like pointers (and technically are pointers), just you don't need to use &
for address and *
for accessing the value.– Petr
Nov 17 '18 at 6:26
@Wei-ShouYang, in your example instead of
int b = p;
you should do int b = *p
. References are somewhat like pointers (and technically are pointers), just you don't need to use &
for address and *
for accessing the value.– Petr
Nov 17 '18 at 6:26
add a comment |
When you copy-construct vec3
, a shallow copy is made (C++ doesn't really do "deep" copy). All the elements in the vector are copied by value, just as with any other copy of a std::vector
.
I don't see how you could copyvec
and more "deeply" than what happens withvector<int> vec3 = rtn_vec();
. It's not a vector of pointers.
– François Andrieux
Nov 15 '18 at 15:31
No, but if it was a vector of pointers, then the pointed-to values wouldn't be copied. Hence, it's a shallow copy.
– Toby Speight
Nov 15 '18 at 15:33
add a comment |
When you copy-construct vec3
, a shallow copy is made (C++ doesn't really do "deep" copy). All the elements in the vector are copied by value, just as with any other copy of a std::vector
.
I don't see how you could copyvec
and more "deeply" than what happens withvector<int> vec3 = rtn_vec();
. It's not a vector of pointers.
– François Andrieux
Nov 15 '18 at 15:31
No, but if it was a vector of pointers, then the pointed-to values wouldn't be copied. Hence, it's a shallow copy.
– Toby Speight
Nov 15 '18 at 15:33
add a comment |
When you copy-construct vec3
, a shallow copy is made (C++ doesn't really do "deep" copy). All the elements in the vector are copied by value, just as with any other copy of a std::vector
.
When you copy-construct vec3
, a shallow copy is made (C++ doesn't really do "deep" copy). All the elements in the vector are copied by value, just as with any other copy of a std::vector
.
edited Nov 15 '18 at 15:34
answered Nov 15 '18 at 15:17


Toby SpeightToby Speight
17.6k134469
17.6k134469
I don't see how you could copyvec
and more "deeply" than what happens withvector<int> vec3 = rtn_vec();
. It's not a vector of pointers.
– François Andrieux
Nov 15 '18 at 15:31
No, but if it was a vector of pointers, then the pointed-to values wouldn't be copied. Hence, it's a shallow copy.
– Toby Speight
Nov 15 '18 at 15:33
add a comment |
I don't see how you could copyvec
and more "deeply" than what happens withvector<int> vec3 = rtn_vec();
. It's not a vector of pointers.
– François Andrieux
Nov 15 '18 at 15:31
No, but if it was a vector of pointers, then the pointed-to values wouldn't be copied. Hence, it's a shallow copy.
– Toby Speight
Nov 15 '18 at 15:33
I don't see how you could copy
vec
and more "deeply" than what happens with vector<int> vec3 = rtn_vec();
. It's not a vector of pointers.– François Andrieux
Nov 15 '18 at 15:31
I don't see how you could copy
vec
and more "deeply" than what happens with vector<int> vec3 = rtn_vec();
. It's not a vector of pointers.– François Andrieux
Nov 15 '18 at 15:31
No, but if it was a vector of pointers, then the pointed-to values wouldn't be copied. Hence, it's a shallow copy.
– Toby Speight
Nov 15 '18 at 15:33
No, but if it was a vector of pointers, then the pointed-to values wouldn't be copied. Hence, it's a shallow copy.
– Toby Speight
Nov 15 '18 at 15:33
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53322459%2fhow-to-get-the-returned-reference-to-a-vector%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ua,S6uL9Mr19rOWP
1
Since the function returns a reference to
vec1
, way 1 is equivalent tovector<int>& vec2 = vec1;
, way 2 is equivalent tovector<int> vec3 = vec1;
.– molbdnilo
Nov 15 '18 at 15:23