Spring Data : issue with OneToMany, child not saved properly
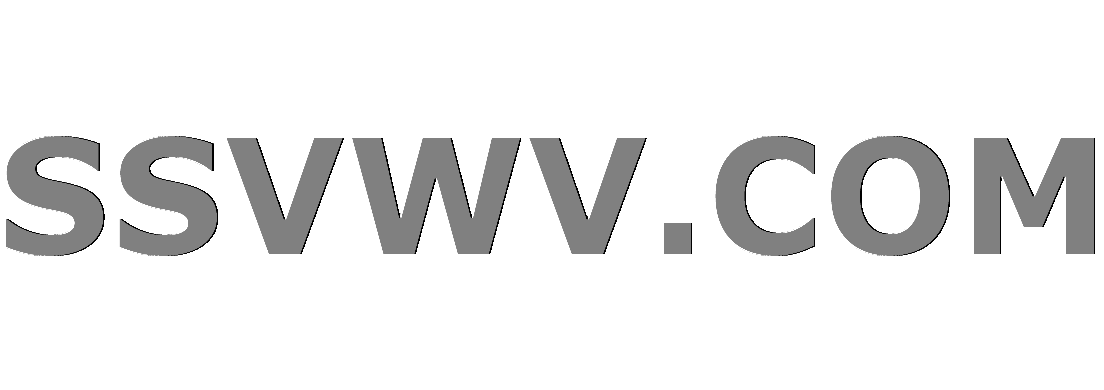
Multi tool use
up vote
1
down vote
favorite
I am having trouble dealing with @OneToMany
relationship.
Here is my code :
@Entity
@Table(name = "type_mouvement")
@Cache(usage = CacheConcurrencyStrategy.NONSTRICT_READ_WRITE)
public class TypeMouvement implements Serializable
private static final long serialVersionUID = 1L;
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
@OneToMany(mappedBy="typeMouvement", fetch = FetchType.EAGER,cascade = CascadeType.PERSIST)
private List<CompteTypeMouvement> comptes;
...
@Entity
@Table(name = "type_mouvement_comptes")
@Cache(usage = CacheConcurrencyStrategy.NONSTRICT_READ_WRITE)
public class CompteTypeMouvement implements Serializable
private static final long serialVersionUID = 1L;
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
private String numCompte;
@ManyToOne
private TypeMouvement typeMouvement;
...
How I use these entities :
TypeMouvement typeMouvementFromDB = typeMouvementRepository.findOne(new Long(1));
CompteTypeMouvement compte = new CompteTypeMouvement();
compte.setNumCompte("123");
compte.setTypeMouvement(typeMouvementFromDB);
typeMouvementFromDB.getComptes().add(compte);
typeMouvementRepository.save(typeMouvementFromDB);
The result I get :
I thought I would get :
Why are the properties of CompteTypeMouvement
not filled when I save TypeMouvement
?
java hibernate jpa orm spring-data
add a comment |
up vote
1
down vote
favorite
I am having trouble dealing with @OneToMany
relationship.
Here is my code :
@Entity
@Table(name = "type_mouvement")
@Cache(usage = CacheConcurrencyStrategy.NONSTRICT_READ_WRITE)
public class TypeMouvement implements Serializable
private static final long serialVersionUID = 1L;
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
@OneToMany(mappedBy="typeMouvement", fetch = FetchType.EAGER,cascade = CascadeType.PERSIST)
private List<CompteTypeMouvement> comptes;
...
@Entity
@Table(name = "type_mouvement_comptes")
@Cache(usage = CacheConcurrencyStrategy.NONSTRICT_READ_WRITE)
public class CompteTypeMouvement implements Serializable
private static final long serialVersionUID = 1L;
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
private String numCompte;
@ManyToOne
private TypeMouvement typeMouvement;
...
How I use these entities :
TypeMouvement typeMouvementFromDB = typeMouvementRepository.findOne(new Long(1));
CompteTypeMouvement compte = new CompteTypeMouvement();
compte.setNumCompte("123");
compte.setTypeMouvement(typeMouvementFromDB);
typeMouvementFromDB.getComptes().add(compte);
typeMouvementRepository.save(typeMouvementFromDB);
The result I get :
I thought I would get :
Why are the properties of CompteTypeMouvement
not filled when I save TypeMouvement
?
java hibernate jpa orm spring-data
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I am having trouble dealing with @OneToMany
relationship.
Here is my code :
@Entity
@Table(name = "type_mouvement")
@Cache(usage = CacheConcurrencyStrategy.NONSTRICT_READ_WRITE)
public class TypeMouvement implements Serializable
private static final long serialVersionUID = 1L;
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
@OneToMany(mappedBy="typeMouvement", fetch = FetchType.EAGER,cascade = CascadeType.PERSIST)
private List<CompteTypeMouvement> comptes;
...
@Entity
@Table(name = "type_mouvement_comptes")
@Cache(usage = CacheConcurrencyStrategy.NONSTRICT_READ_WRITE)
public class CompteTypeMouvement implements Serializable
private static final long serialVersionUID = 1L;
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
private String numCompte;
@ManyToOne
private TypeMouvement typeMouvement;
...
How I use these entities :
TypeMouvement typeMouvementFromDB = typeMouvementRepository.findOne(new Long(1));
CompteTypeMouvement compte = new CompteTypeMouvement();
compte.setNumCompte("123");
compte.setTypeMouvement(typeMouvementFromDB);
typeMouvementFromDB.getComptes().add(compte);
typeMouvementRepository.save(typeMouvementFromDB);
The result I get :
I thought I would get :
Why are the properties of CompteTypeMouvement
not filled when I save TypeMouvement
?
java hibernate jpa orm spring-data
I am having trouble dealing with @OneToMany
relationship.
Here is my code :
@Entity
@Table(name = "type_mouvement")
@Cache(usage = CacheConcurrencyStrategy.NONSTRICT_READ_WRITE)
public class TypeMouvement implements Serializable
private static final long serialVersionUID = 1L;
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
@OneToMany(mappedBy="typeMouvement", fetch = FetchType.EAGER,cascade = CascadeType.PERSIST)
private List<CompteTypeMouvement> comptes;
...
@Entity
@Table(name = "type_mouvement_comptes")
@Cache(usage = CacheConcurrencyStrategy.NONSTRICT_READ_WRITE)
public class CompteTypeMouvement implements Serializable
private static final long serialVersionUID = 1L;
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
private String numCompte;
@ManyToOne
private TypeMouvement typeMouvement;
...
How I use these entities :
TypeMouvement typeMouvementFromDB = typeMouvementRepository.findOne(new Long(1));
CompteTypeMouvement compte = new CompteTypeMouvement();
compte.setNumCompte("123");
compte.setTypeMouvement(typeMouvementFromDB);
typeMouvementFromDB.getComptes().add(compte);
typeMouvementRepository.save(typeMouvementFromDB);
The result I get :
I thought I would get :
Why are the properties of CompteTypeMouvement
not filled when I save TypeMouvement
?
java hibernate jpa orm spring-data
java hibernate jpa orm spring-data
edited 21 hours ago


Maciej Kowalski
11.1k72037
11.1k72037
asked 21 hours ago
thomas
9110
9110
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
2
down vote
accepted
You are trying to invoke save passing an already existing entity:
TypeMouvement typeMouvementFromDB = typeMouvementRepository.findOne(new Long(1));
typeMouvementRepository.save(typeMouvementFromDB);
The relationship has a persist cascade type only though:
@OneToMany(mappedBy="typeMouvement", fetch = FetchType.EAGER,cascade = CascadeType.PERSIST)
private List<CompteTypeMouvement> comptes;
The save impl is a follows (spring-data-jpa-1.11.3):
public <S extends T> S save(S entity)
if (entityInformation.isNew(entity))
em.persist(entity);
return entity;
else
return em.merge(entity);
Which means that a merge
instead of a persist
will be invoked.
This if you add merge
to cascade it should work:
@OneToMany(mappedBy="typeMouvement", fetch = FetchType.EAGER,
cascade = CascadeType.PERSIST, CascadeType.MERGE)
private List<CompteTypeMouvement> comptes;
If he is saving a TypeMouvement then won't he need to add the cascade on the@ManyToOne
?
– Alan Hay
20 hours ago
If he was saving CompteTypeMouvement then yes.. and in that case he would only need PERSIST cascade. But that is not the situation
– Maciej Kowalski
20 hours ago
Sorry, yes, of course mis-read the code.
– Alan Hay
19 hours ago
Thanks! Now I understand
– thomas
17 hours ago
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
You are trying to invoke save passing an already existing entity:
TypeMouvement typeMouvementFromDB = typeMouvementRepository.findOne(new Long(1));
typeMouvementRepository.save(typeMouvementFromDB);
The relationship has a persist cascade type only though:
@OneToMany(mappedBy="typeMouvement", fetch = FetchType.EAGER,cascade = CascadeType.PERSIST)
private List<CompteTypeMouvement> comptes;
The save impl is a follows (spring-data-jpa-1.11.3):
public <S extends T> S save(S entity)
if (entityInformation.isNew(entity))
em.persist(entity);
return entity;
else
return em.merge(entity);
Which means that a merge
instead of a persist
will be invoked.
This if you add merge
to cascade it should work:
@OneToMany(mappedBy="typeMouvement", fetch = FetchType.EAGER,
cascade = CascadeType.PERSIST, CascadeType.MERGE)
private List<CompteTypeMouvement> comptes;
If he is saving a TypeMouvement then won't he need to add the cascade on the@ManyToOne
?
– Alan Hay
20 hours ago
If he was saving CompteTypeMouvement then yes.. and in that case he would only need PERSIST cascade. But that is not the situation
– Maciej Kowalski
20 hours ago
Sorry, yes, of course mis-read the code.
– Alan Hay
19 hours ago
Thanks! Now I understand
– thomas
17 hours ago
add a comment |
up vote
2
down vote
accepted
You are trying to invoke save passing an already existing entity:
TypeMouvement typeMouvementFromDB = typeMouvementRepository.findOne(new Long(1));
typeMouvementRepository.save(typeMouvementFromDB);
The relationship has a persist cascade type only though:
@OneToMany(mappedBy="typeMouvement", fetch = FetchType.EAGER,cascade = CascadeType.PERSIST)
private List<CompteTypeMouvement> comptes;
The save impl is a follows (spring-data-jpa-1.11.3):
public <S extends T> S save(S entity)
if (entityInformation.isNew(entity))
em.persist(entity);
return entity;
else
return em.merge(entity);
Which means that a merge
instead of a persist
will be invoked.
This if you add merge
to cascade it should work:
@OneToMany(mappedBy="typeMouvement", fetch = FetchType.EAGER,
cascade = CascadeType.PERSIST, CascadeType.MERGE)
private List<CompteTypeMouvement> comptes;
If he is saving a TypeMouvement then won't he need to add the cascade on the@ManyToOne
?
– Alan Hay
20 hours ago
If he was saving CompteTypeMouvement then yes.. and in that case he would only need PERSIST cascade. But that is not the situation
– Maciej Kowalski
20 hours ago
Sorry, yes, of course mis-read the code.
– Alan Hay
19 hours ago
Thanks! Now I understand
– thomas
17 hours ago
add a comment |
up vote
2
down vote
accepted
up vote
2
down vote
accepted
You are trying to invoke save passing an already existing entity:
TypeMouvement typeMouvementFromDB = typeMouvementRepository.findOne(new Long(1));
typeMouvementRepository.save(typeMouvementFromDB);
The relationship has a persist cascade type only though:
@OneToMany(mappedBy="typeMouvement", fetch = FetchType.EAGER,cascade = CascadeType.PERSIST)
private List<CompteTypeMouvement> comptes;
The save impl is a follows (spring-data-jpa-1.11.3):
public <S extends T> S save(S entity)
if (entityInformation.isNew(entity))
em.persist(entity);
return entity;
else
return em.merge(entity);
Which means that a merge
instead of a persist
will be invoked.
This if you add merge
to cascade it should work:
@OneToMany(mappedBy="typeMouvement", fetch = FetchType.EAGER,
cascade = CascadeType.PERSIST, CascadeType.MERGE)
private List<CompteTypeMouvement> comptes;
You are trying to invoke save passing an already existing entity:
TypeMouvement typeMouvementFromDB = typeMouvementRepository.findOne(new Long(1));
typeMouvementRepository.save(typeMouvementFromDB);
The relationship has a persist cascade type only though:
@OneToMany(mappedBy="typeMouvement", fetch = FetchType.EAGER,cascade = CascadeType.PERSIST)
private List<CompteTypeMouvement> comptes;
The save impl is a follows (spring-data-jpa-1.11.3):
public <S extends T> S save(S entity)
if (entityInformation.isNew(entity))
em.persist(entity);
return entity;
else
return em.merge(entity);
Which means that a merge
instead of a persist
will be invoked.
This if you add merge
to cascade it should work:
@OneToMany(mappedBy="typeMouvement", fetch = FetchType.EAGER,
cascade = CascadeType.PERSIST, CascadeType.MERGE)
private List<CompteTypeMouvement> comptes;
answered 21 hours ago


Maciej Kowalski
11.1k72037
11.1k72037
If he is saving a TypeMouvement then won't he need to add the cascade on the@ManyToOne
?
– Alan Hay
20 hours ago
If he was saving CompteTypeMouvement then yes.. and in that case he would only need PERSIST cascade. But that is not the situation
– Maciej Kowalski
20 hours ago
Sorry, yes, of course mis-read the code.
– Alan Hay
19 hours ago
Thanks! Now I understand
– thomas
17 hours ago
add a comment |
If he is saving a TypeMouvement then won't he need to add the cascade on the@ManyToOne
?
– Alan Hay
20 hours ago
If he was saving CompteTypeMouvement then yes.. and in that case he would only need PERSIST cascade. But that is not the situation
– Maciej Kowalski
20 hours ago
Sorry, yes, of course mis-read the code.
– Alan Hay
19 hours ago
Thanks! Now I understand
– thomas
17 hours ago
If he is saving a TypeMouvement then won't he need to add the cascade on the
@ManyToOne
?– Alan Hay
20 hours ago
If he is saving a TypeMouvement then won't he need to add the cascade on the
@ManyToOne
?– Alan Hay
20 hours ago
If he was saving CompteTypeMouvement then yes.. and in that case he would only need PERSIST cascade. But that is not the situation
– Maciej Kowalski
20 hours ago
If he was saving CompteTypeMouvement then yes.. and in that case he would only need PERSIST cascade. But that is not the situation
– Maciej Kowalski
20 hours ago
Sorry, yes, of course mis-read the code.
– Alan Hay
19 hours ago
Sorry, yes, of course mis-read the code.
– Alan Hay
19 hours ago
Thanks! Now I understand
– thomas
17 hours ago
Thanks! Now I understand
– thomas
17 hours ago
add a comment |
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53224098%2fspring-data-issue-with-onetomany-child-not-saved-properly%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
G,3ouv4dqZ1lM3v K93,mD1HdWHVb