Clear all widgets in a layout in pyqt
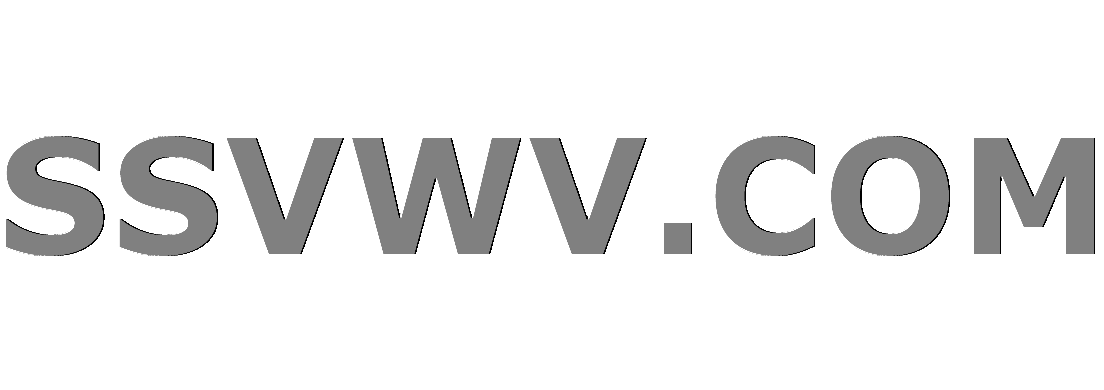
Multi tool use
Is there a way to clear (delete) all the widgets in a layout?
self.plot_layout = QtGui.QGridLayout()
self.plot_layout.setGeometry(QtCore.QRect(200,200,200,200))
self.root_layout.addLayout(self.plot_layout)
self.plot_layout.addWidget(MyWidget())
Now I want to replace the widget in plot_layout
with a new widget. Is there an easy way to clear all the widgets in plot_layout
? I don't see any method such.
python qt pyqt pyqt4
add a comment |
Is there a way to clear (delete) all the widgets in a layout?
self.plot_layout = QtGui.QGridLayout()
self.plot_layout.setGeometry(QtCore.QRect(200,200,200,200))
self.root_layout.addLayout(self.plot_layout)
self.plot_layout.addWidget(MyWidget())
Now I want to replace the widget in plot_layout
with a new widget. Is there an easy way to clear all the widgets in plot_layout
? I don't see any method such.
python qt pyqt pyqt4
add a comment |
Is there a way to clear (delete) all the widgets in a layout?
self.plot_layout = QtGui.QGridLayout()
self.plot_layout.setGeometry(QtCore.QRect(200,200,200,200))
self.root_layout.addLayout(self.plot_layout)
self.plot_layout.addWidget(MyWidget())
Now I want to replace the widget in plot_layout
with a new widget. Is there an easy way to clear all the widgets in plot_layout
? I don't see any method such.
python qt pyqt pyqt4
Is there a way to clear (delete) all the widgets in a layout?
self.plot_layout = QtGui.QGridLayout()
self.plot_layout.setGeometry(QtCore.QRect(200,200,200,200))
self.root_layout.addLayout(self.plot_layout)
self.plot_layout.addWidget(MyWidget())
Now I want to replace the widget in plot_layout
with a new widget. Is there an easy way to clear all the widgets in plot_layout
? I don't see any method such.
python qt pyqt pyqt4
python qt pyqt pyqt4
edited Jul 11 '17 at 2:41


eyllanesc
78.7k103256
78.7k103256
asked Dec 24 '10 at 21:07


FalmarriFalmarri
32.7k34122178
32.7k34122178
add a comment |
add a comment |
11 Answers
11
active
oldest
votes
After a lot of research (and this one took quite time, so I add it here for future reference), this is the way I found to really clear and delete the widgets in a layout:
for i in reversed(range(layout.count())):
layout.itemAt(i).widget().setParent(None)
What the documentation says about the QWidget is that:
The new widget is deleted when its parent is deleted.
Important note: You need to loop backwards because removing things from the beginning shifts items and changes the order of items in the layout.
To test and confirm that the layout is empty:
for i in range(layout.count()): print i
There seems to be another way to do it. Instead of using the setParent function, use the deleteLater() function like this:
for i in reversed(range(layout.count())):
layout.itemAt(i).widget().deleteLater()
The documentation says that QObject.deleteLater (self)
Schedules this object for deletion.
However, if you run the test code specified above, it prints some values. This indicates that the layout still has items, as opposed to the code with setParent.
for i in reversed(range(layout.count()-1)):
is correct to prevent an out of bounds error
– Max
Jul 12 '16 at 20:18
3
You should usetakeAt()
instead ofitemAt()
.takeAt()
will also remove spacers/stretches (that do not have a parent) from QBoxLayout:widget = layout.takeAt(i).widget(); if widget is not None: widget.setParent(None)
– Stefan Scherfke
Aug 31 '16 at 11:44
Are you sure about thesetParent(None)
approche? I dont think this will delete the Widget. "The new widget is deleted when its parent is deleted." sound to me like a recursive call ofdeleteLater()
when it is called for the parent.
– Skandix
Oct 24 '18 at 14:15
I wrote a simple test that shows that setParent(None) doesn't delete the Widget. Even if this command answers the question in the way of clearing the layout, it is should not be recommended. This will lead to a memory leak!
– Skandix
Oct 24 '18 at 14:27
add a comment |
This may be a bit too late but just wanted to add this for future reference:
def clearLayout(layout):
while layout.count():
child = layout.takeAt(0)
if child.widget():
child.widget().deleteLater()
Adapted from Qt docs http://doc.qt.io/qt-5/qlayout.html#takeAt. Remember that when you are removing children from the layout in a while or for loop, you are effectively modifying the index # of each child item in the layout. That's why you'll run into problems using a for i in range()
loop.
I assume you mean child.widget().deleteLater()... Thanks a ton for this - appears to be a fairly elegant solution. If deleteLater() gives me any trouble I may try close() or setParent(None) as others suggest.
– flutefreak7
May 12 '16 at 21:31
add a comment |
The answer from PALEN works well if you do not need to put new widgets to your layout.
for i in reversed(range(layout.count())):
layout.itemAt(i).widget().setParent(None)
But you will get a "Segmentation fault (core dumped)" at some point if you empty and fill the layout many times or with many widgets. It seems that the layout keeps a list of widget and that this list is limited in size.
If you remove the widgets that way:
for i in reversed(range(layout.count())):
widgetToRemove = layout.itemAt(i).widget()
# remove it from the layout list
layout.removeWidget(widgetToRemove)
# remove it from the gui
widgetToRemove.setParent(None)
You won't get that problem.
1
Thanks a lot, it was driving me crazy the fact I was getting "Process finished with exit code 139" after clearing and adding more items few times.
– Ramon Blanquer
Jun 7 '16 at 15:54
setParent(None)
doesn't delete the widgets. See my comments and answers on palens answer. This is also the reason for theSegmentation fault
. UsedeleteLater()
instead.
– Skandix
Oct 24 '18 at 14:57
python widget.setAttribute(QtCore.Qt.WA_DeleteOnClose) widget.close() gridLayout.removeWidget(widget)
It works for me perfectly
– HOuadhour
Jan 24 at 19:12
add a comment |
You can use the close()
method of widget
:
for i in range(layout.count()): layout.itemAt(i).widget().close()
add a comment |
That's how I clear a layout :
def clearLayout(layout):
if layout != None:
while layout.count():
child = layout.takeAt(0)
if child.widget() is not None:
child.widget().deleteLater()
elif child.layout() is not None:
clearLayout(child.layout())
add a comment |
My solution to this problem is to override the setLayout method of QWidget. The following code updates the layout to the new layout which may or may not contain items that are already displayed. You can simply create a new layout object, add whatever you want to it, then call setLayout. Of course, you can also just call clearLayout to remove everything.
def setLayout(self, layout):
self.clearLayout()
QWidget.setLayout(self, layout)
def clearLayout(self):
if self.layout() is not None:
old_layout = self.layout()
for i in reversed(range(old_layout.count())):
old_layout.itemAt(i).widget().setParent(None)
import sip
sip.delete(old_layout)
add a comment |
From the docs:
To remove a widget from a layout, call
removeWidget()
. CallingQWidget.hide()
on a widget also effectively removes the widget from the layout untilQWidget.show()
is called.
removeWidget
is inherited from QLayout
, that's why it's not listed among the QGridLayout
methods.
But removeWidget() takes a widget as an argumentremoveWidget (self, QWidget w)
. I don't have the reference to the widget.
– Falmarri
Dec 24 '10 at 22:23
1
@Falmarri:grid.itemAt(idx).widget()
to go by index.
– user395760
Dec 24 '10 at 23:26
add a comment |
I use:
while layout.count() > 0:
layout.itemAt(0).setParent(None)
add a comment |
A couple of solutions, if you are swapping between known views using a stacked widget and just flipping the shown index might be a lot easier than adding and removing single widgets from a layout.
If you want to replace all the children of a widget then the QObject
functions findChildren
should get you there e.g. I don't know how the template functions are wrapped in pyqt though. But you could also search for the widgets by name if you know them.
Well this is my first Qt app (other than just messing with it) so I'm sure I'm doing a lot of things really inefficiently. For notplot_layout
will only have 1 widget at a time (I made it a gridlayout for future expansion). The widget it has is a custom widget that extends matplotlib'sFigureCanvasQTAgg
. I have a lot of plots, and what I want to do is when the user clicks on a plot in a treeview (this is working), I want to show that plot.
– Falmarri
Dec 25 '10 at 19:16
Maybe it might be a better idea to move the plotting code from theFigureCanvasQTAgg
subclasses to a separate place, just updating a givenFigure
on request. Then you can simply redraw the plot instead of messing with widgets and layouts. See eli.thegreenplace.net/files/prog_code/qt_mpl_bars.py.txt for example code.
– Ferdinand Beyer
May 9 '11 at 15:26
add a comment |
this my first time actually answering a stack overflow question but I saw that all the answers here are slightly wrong. (yes, I know that the question was to delete all widgets) The problem with most of them is that they don't account for nested layouts, so I made a recursive function, that given a layout it will recursively delete everything inside it, and all the layouts inside of it. here it is:
def clearLayout(layout):
print("-- -- input layout: "+str(layout))
for i in reversed(range(layout.count())):
layoutItem = layout.itemAt(i)
if layoutItem.widget() is not None:
widgetToRemove = layoutItem.widget()
print("found widget: " + str(widgetToRemove))
widgetToRemove.setParent(None)
layout.removeWidget(widgetToRemove)
elif layoutItem.spacerItem() is not None:
print("found spacer: " + str(layoutItem.spacerItem()))
else:
layoutToRemove = layout.itemAt(i)
print("-- found Layout: "+str(layoutToRemove))
clearLayout(layoutToRemove)
I might not have accounted for all UI types, not sure. Hope this helps!
add a comment |
for i in reversed (range(layout.count())):
layout.itemAt(i).widget().close()
layout.takeAt(i)
or
for i in range(layout.count()):
layout.itemAt(0).widget().close()
layout.takeAt(0)
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f4528347%2fclear-all-widgets-in-a-layout-in-pyqt%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
11 Answers
11
active
oldest
votes
11 Answers
11
active
oldest
votes
active
oldest
votes
active
oldest
votes
After a lot of research (and this one took quite time, so I add it here for future reference), this is the way I found to really clear and delete the widgets in a layout:
for i in reversed(range(layout.count())):
layout.itemAt(i).widget().setParent(None)
What the documentation says about the QWidget is that:
The new widget is deleted when its parent is deleted.
Important note: You need to loop backwards because removing things from the beginning shifts items and changes the order of items in the layout.
To test and confirm that the layout is empty:
for i in range(layout.count()): print i
There seems to be another way to do it. Instead of using the setParent function, use the deleteLater() function like this:
for i in reversed(range(layout.count())):
layout.itemAt(i).widget().deleteLater()
The documentation says that QObject.deleteLater (self)
Schedules this object for deletion.
However, if you run the test code specified above, it prints some values. This indicates that the layout still has items, as opposed to the code with setParent.
for i in reversed(range(layout.count()-1)):
is correct to prevent an out of bounds error
– Max
Jul 12 '16 at 20:18
3
You should usetakeAt()
instead ofitemAt()
.takeAt()
will also remove spacers/stretches (that do not have a parent) from QBoxLayout:widget = layout.takeAt(i).widget(); if widget is not None: widget.setParent(None)
– Stefan Scherfke
Aug 31 '16 at 11:44
Are you sure about thesetParent(None)
approche? I dont think this will delete the Widget. "The new widget is deleted when its parent is deleted." sound to me like a recursive call ofdeleteLater()
when it is called for the parent.
– Skandix
Oct 24 '18 at 14:15
I wrote a simple test that shows that setParent(None) doesn't delete the Widget. Even if this command answers the question in the way of clearing the layout, it is should not be recommended. This will lead to a memory leak!
– Skandix
Oct 24 '18 at 14:27
add a comment |
After a lot of research (and this one took quite time, so I add it here for future reference), this is the way I found to really clear and delete the widgets in a layout:
for i in reversed(range(layout.count())):
layout.itemAt(i).widget().setParent(None)
What the documentation says about the QWidget is that:
The new widget is deleted when its parent is deleted.
Important note: You need to loop backwards because removing things from the beginning shifts items and changes the order of items in the layout.
To test and confirm that the layout is empty:
for i in range(layout.count()): print i
There seems to be another way to do it. Instead of using the setParent function, use the deleteLater() function like this:
for i in reversed(range(layout.count())):
layout.itemAt(i).widget().deleteLater()
The documentation says that QObject.deleteLater (self)
Schedules this object for deletion.
However, if you run the test code specified above, it prints some values. This indicates that the layout still has items, as opposed to the code with setParent.
for i in reversed(range(layout.count()-1)):
is correct to prevent an out of bounds error
– Max
Jul 12 '16 at 20:18
3
You should usetakeAt()
instead ofitemAt()
.takeAt()
will also remove spacers/stretches (that do not have a parent) from QBoxLayout:widget = layout.takeAt(i).widget(); if widget is not None: widget.setParent(None)
– Stefan Scherfke
Aug 31 '16 at 11:44
Are you sure about thesetParent(None)
approche? I dont think this will delete the Widget. "The new widget is deleted when its parent is deleted." sound to me like a recursive call ofdeleteLater()
when it is called for the parent.
– Skandix
Oct 24 '18 at 14:15
I wrote a simple test that shows that setParent(None) doesn't delete the Widget. Even if this command answers the question in the way of clearing the layout, it is should not be recommended. This will lead to a memory leak!
– Skandix
Oct 24 '18 at 14:27
add a comment |
After a lot of research (and this one took quite time, so I add it here for future reference), this is the way I found to really clear and delete the widgets in a layout:
for i in reversed(range(layout.count())):
layout.itemAt(i).widget().setParent(None)
What the documentation says about the QWidget is that:
The new widget is deleted when its parent is deleted.
Important note: You need to loop backwards because removing things from the beginning shifts items and changes the order of items in the layout.
To test and confirm that the layout is empty:
for i in range(layout.count()): print i
There seems to be another way to do it. Instead of using the setParent function, use the deleteLater() function like this:
for i in reversed(range(layout.count())):
layout.itemAt(i).widget().deleteLater()
The documentation says that QObject.deleteLater (self)
Schedules this object for deletion.
However, if you run the test code specified above, it prints some values. This indicates that the layout still has items, as opposed to the code with setParent.
After a lot of research (and this one took quite time, so I add it here for future reference), this is the way I found to really clear and delete the widgets in a layout:
for i in reversed(range(layout.count())):
layout.itemAt(i).widget().setParent(None)
What the documentation says about the QWidget is that:
The new widget is deleted when its parent is deleted.
Important note: You need to loop backwards because removing things from the beginning shifts items and changes the order of items in the layout.
To test and confirm that the layout is empty:
for i in range(layout.count()): print i
There seems to be another way to do it. Instead of using the setParent function, use the deleteLater() function like this:
for i in reversed(range(layout.count())):
layout.itemAt(i).widget().deleteLater()
The documentation says that QObject.deleteLater (self)
Schedules this object for deletion.
However, if you run the test code specified above, it prints some values. This indicates that the layout still has items, as opposed to the code with setParent.
edited Jul 11 '17 at 2:42


eyllanesc
78.7k103256
78.7k103256
answered Oct 27 '12 at 19:50


PALENPALEN
1,64511623
1,64511623
for i in reversed(range(layout.count()-1)):
is correct to prevent an out of bounds error
– Max
Jul 12 '16 at 20:18
3
You should usetakeAt()
instead ofitemAt()
.takeAt()
will also remove spacers/stretches (that do not have a parent) from QBoxLayout:widget = layout.takeAt(i).widget(); if widget is not None: widget.setParent(None)
– Stefan Scherfke
Aug 31 '16 at 11:44
Are you sure about thesetParent(None)
approche? I dont think this will delete the Widget. "The new widget is deleted when its parent is deleted." sound to me like a recursive call ofdeleteLater()
when it is called for the parent.
– Skandix
Oct 24 '18 at 14:15
I wrote a simple test that shows that setParent(None) doesn't delete the Widget. Even if this command answers the question in the way of clearing the layout, it is should not be recommended. This will lead to a memory leak!
– Skandix
Oct 24 '18 at 14:27
add a comment |
for i in reversed(range(layout.count()-1)):
is correct to prevent an out of bounds error
– Max
Jul 12 '16 at 20:18
3
You should usetakeAt()
instead ofitemAt()
.takeAt()
will also remove spacers/stretches (that do not have a parent) from QBoxLayout:widget = layout.takeAt(i).widget(); if widget is not None: widget.setParent(None)
– Stefan Scherfke
Aug 31 '16 at 11:44
Are you sure about thesetParent(None)
approche? I dont think this will delete the Widget. "The new widget is deleted when its parent is deleted." sound to me like a recursive call ofdeleteLater()
when it is called for the parent.
– Skandix
Oct 24 '18 at 14:15
I wrote a simple test that shows that setParent(None) doesn't delete the Widget. Even if this command answers the question in the way of clearing the layout, it is should not be recommended. This will lead to a memory leak!
– Skandix
Oct 24 '18 at 14:27
for i in reversed(range(layout.count()-1)):
is correct to prevent an out of bounds error– Max
Jul 12 '16 at 20:18
for i in reversed(range(layout.count()-1)):
is correct to prevent an out of bounds error– Max
Jul 12 '16 at 20:18
3
3
You should use
takeAt()
instead of itemAt()
. takeAt()
will also remove spacers/stretches (that do not have a parent) from QBoxLayout: widget = layout.takeAt(i).widget(); if widget is not None: widget.setParent(None)
– Stefan Scherfke
Aug 31 '16 at 11:44
You should use
takeAt()
instead of itemAt()
. takeAt()
will also remove spacers/stretches (that do not have a parent) from QBoxLayout: widget = layout.takeAt(i).widget(); if widget is not None: widget.setParent(None)
– Stefan Scherfke
Aug 31 '16 at 11:44
Are you sure about the
setParent(None)
approche? I dont think this will delete the Widget. "The new widget is deleted when its parent is deleted." sound to me like a recursive call of deleteLater()
when it is called for the parent.– Skandix
Oct 24 '18 at 14:15
Are you sure about the
setParent(None)
approche? I dont think this will delete the Widget. "The new widget is deleted when its parent is deleted." sound to me like a recursive call of deleteLater()
when it is called for the parent.– Skandix
Oct 24 '18 at 14:15
I wrote a simple test that shows that setParent(None) doesn't delete the Widget. Even if this command answers the question in the way of clearing the layout, it is should not be recommended. This will lead to a memory leak!
– Skandix
Oct 24 '18 at 14:27
I wrote a simple test that shows that setParent(None) doesn't delete the Widget. Even if this command answers the question in the way of clearing the layout, it is should not be recommended. This will lead to a memory leak!
– Skandix
Oct 24 '18 at 14:27
add a comment |
This may be a bit too late but just wanted to add this for future reference:
def clearLayout(layout):
while layout.count():
child = layout.takeAt(0)
if child.widget():
child.widget().deleteLater()
Adapted from Qt docs http://doc.qt.io/qt-5/qlayout.html#takeAt. Remember that when you are removing children from the layout in a while or for loop, you are effectively modifying the index # of each child item in the layout. That's why you'll run into problems using a for i in range()
loop.
I assume you mean child.widget().deleteLater()... Thanks a ton for this - appears to be a fairly elegant solution. If deleteLater() gives me any trouble I may try close() or setParent(None) as others suggest.
– flutefreak7
May 12 '16 at 21:31
add a comment |
This may be a bit too late but just wanted to add this for future reference:
def clearLayout(layout):
while layout.count():
child = layout.takeAt(0)
if child.widget():
child.widget().deleteLater()
Adapted from Qt docs http://doc.qt.io/qt-5/qlayout.html#takeAt. Remember that when you are removing children from the layout in a while or for loop, you are effectively modifying the index # of each child item in the layout. That's why you'll run into problems using a for i in range()
loop.
I assume you mean child.widget().deleteLater()... Thanks a ton for this - appears to be a fairly elegant solution. If deleteLater() gives me any trouble I may try close() or setParent(None) as others suggest.
– flutefreak7
May 12 '16 at 21:31
add a comment |
This may be a bit too late but just wanted to add this for future reference:
def clearLayout(layout):
while layout.count():
child = layout.takeAt(0)
if child.widget():
child.widget().deleteLater()
Adapted from Qt docs http://doc.qt.io/qt-5/qlayout.html#takeAt. Remember that when you are removing children from the layout in a while or for loop, you are effectively modifying the index # of each child item in the layout. That's why you'll run into problems using a for i in range()
loop.
This may be a bit too late but just wanted to add this for future reference:
def clearLayout(layout):
while layout.count():
child = layout.takeAt(0)
if child.widget():
child.widget().deleteLater()
Adapted from Qt docs http://doc.qt.io/qt-5/qlayout.html#takeAt. Remember that when you are removing children from the layout in a while or for loop, you are effectively modifying the index # of each child item in the layout. That's why you'll run into problems using a for i in range()
loop.
edited Oct 11 '17 at 12:21


djvg
1,0921728
1,0921728
answered Apr 8 '12 at 23:56
Nadeem DoubaNadeem Douba
35326
35326
I assume you mean child.widget().deleteLater()... Thanks a ton for this - appears to be a fairly elegant solution. If deleteLater() gives me any trouble I may try close() or setParent(None) as others suggest.
– flutefreak7
May 12 '16 at 21:31
add a comment |
I assume you mean child.widget().deleteLater()... Thanks a ton for this - appears to be a fairly elegant solution. If deleteLater() gives me any trouble I may try close() or setParent(None) as others suggest.
– flutefreak7
May 12 '16 at 21:31
I assume you mean child.widget().deleteLater()... Thanks a ton for this - appears to be a fairly elegant solution. If deleteLater() gives me any trouble I may try close() or setParent(None) as others suggest.
– flutefreak7
May 12 '16 at 21:31
I assume you mean child.widget().deleteLater()... Thanks a ton for this - appears to be a fairly elegant solution. If deleteLater() gives me any trouble I may try close() or setParent(None) as others suggest.
– flutefreak7
May 12 '16 at 21:31
add a comment |
The answer from PALEN works well if you do not need to put new widgets to your layout.
for i in reversed(range(layout.count())):
layout.itemAt(i).widget().setParent(None)
But you will get a "Segmentation fault (core dumped)" at some point if you empty and fill the layout many times or with many widgets. It seems that the layout keeps a list of widget and that this list is limited in size.
If you remove the widgets that way:
for i in reversed(range(layout.count())):
widgetToRemove = layout.itemAt(i).widget()
# remove it from the layout list
layout.removeWidget(widgetToRemove)
# remove it from the gui
widgetToRemove.setParent(None)
You won't get that problem.
1
Thanks a lot, it was driving me crazy the fact I was getting "Process finished with exit code 139" after clearing and adding more items few times.
– Ramon Blanquer
Jun 7 '16 at 15:54
setParent(None)
doesn't delete the widgets. See my comments and answers on palens answer. This is also the reason for theSegmentation fault
. UsedeleteLater()
instead.
– Skandix
Oct 24 '18 at 14:57
python widget.setAttribute(QtCore.Qt.WA_DeleteOnClose) widget.close() gridLayout.removeWidget(widget)
It works for me perfectly
– HOuadhour
Jan 24 at 19:12
add a comment |
The answer from PALEN works well if you do not need to put new widgets to your layout.
for i in reversed(range(layout.count())):
layout.itemAt(i).widget().setParent(None)
But you will get a "Segmentation fault (core dumped)" at some point if you empty and fill the layout many times or with many widgets. It seems that the layout keeps a list of widget and that this list is limited in size.
If you remove the widgets that way:
for i in reversed(range(layout.count())):
widgetToRemove = layout.itemAt(i).widget()
# remove it from the layout list
layout.removeWidget(widgetToRemove)
# remove it from the gui
widgetToRemove.setParent(None)
You won't get that problem.
1
Thanks a lot, it was driving me crazy the fact I was getting "Process finished with exit code 139" after clearing and adding more items few times.
– Ramon Blanquer
Jun 7 '16 at 15:54
setParent(None)
doesn't delete the widgets. See my comments and answers on palens answer. This is also the reason for theSegmentation fault
. UsedeleteLater()
instead.
– Skandix
Oct 24 '18 at 14:57
python widget.setAttribute(QtCore.Qt.WA_DeleteOnClose) widget.close() gridLayout.removeWidget(widget)
It works for me perfectly
– HOuadhour
Jan 24 at 19:12
add a comment |
The answer from PALEN works well if you do not need to put new widgets to your layout.
for i in reversed(range(layout.count())):
layout.itemAt(i).widget().setParent(None)
But you will get a "Segmentation fault (core dumped)" at some point if you empty and fill the layout many times or with many widgets. It seems that the layout keeps a list of widget and that this list is limited in size.
If you remove the widgets that way:
for i in reversed(range(layout.count())):
widgetToRemove = layout.itemAt(i).widget()
# remove it from the layout list
layout.removeWidget(widgetToRemove)
# remove it from the gui
widgetToRemove.setParent(None)
You won't get that problem.
The answer from PALEN works well if you do not need to put new widgets to your layout.
for i in reversed(range(layout.count())):
layout.itemAt(i).widget().setParent(None)
But you will get a "Segmentation fault (core dumped)" at some point if you empty and fill the layout many times or with many widgets. It seems that the layout keeps a list of widget and that this list is limited in size.
If you remove the widgets that way:
for i in reversed(range(layout.count())):
widgetToRemove = layout.itemAt(i).widget()
# remove it from the layout list
layout.removeWidget(widgetToRemove)
# remove it from the gui
widgetToRemove.setParent(None)
You won't get that problem.
edited Oct 24 '18 at 18:23
answered Aug 15 '14 at 16:18


Blaa_ThorBlaa_Thor
16717
16717
1
Thanks a lot, it was driving me crazy the fact I was getting "Process finished with exit code 139" after clearing and adding more items few times.
– Ramon Blanquer
Jun 7 '16 at 15:54
setParent(None)
doesn't delete the widgets. See my comments and answers on palens answer. This is also the reason for theSegmentation fault
. UsedeleteLater()
instead.
– Skandix
Oct 24 '18 at 14:57
python widget.setAttribute(QtCore.Qt.WA_DeleteOnClose) widget.close() gridLayout.removeWidget(widget)
It works for me perfectly
– HOuadhour
Jan 24 at 19:12
add a comment |
1
Thanks a lot, it was driving me crazy the fact I was getting "Process finished with exit code 139" after clearing and adding more items few times.
– Ramon Blanquer
Jun 7 '16 at 15:54
setParent(None)
doesn't delete the widgets. See my comments and answers on palens answer. This is also the reason for theSegmentation fault
. UsedeleteLater()
instead.
– Skandix
Oct 24 '18 at 14:57
python widget.setAttribute(QtCore.Qt.WA_DeleteOnClose) widget.close() gridLayout.removeWidget(widget)
It works for me perfectly
– HOuadhour
Jan 24 at 19:12
1
1
Thanks a lot, it was driving me crazy the fact I was getting "Process finished with exit code 139" after clearing and adding more items few times.
– Ramon Blanquer
Jun 7 '16 at 15:54
Thanks a lot, it was driving me crazy the fact I was getting "Process finished with exit code 139" after clearing and adding more items few times.
– Ramon Blanquer
Jun 7 '16 at 15:54
setParent(None)
doesn't delete the widgets. See my comments and answers on palens answer. This is also the reason for the Segmentation fault
. Use deleteLater()
instead.– Skandix
Oct 24 '18 at 14:57
setParent(None)
doesn't delete the widgets. See my comments and answers on palens answer. This is also the reason for the Segmentation fault
. Use deleteLater()
instead.– Skandix
Oct 24 '18 at 14:57
python widget.setAttribute(QtCore.Qt.WA_DeleteOnClose) widget.close() gridLayout.removeWidget(widget)
It works for me perfectly– HOuadhour
Jan 24 at 19:12
python widget.setAttribute(QtCore.Qt.WA_DeleteOnClose) widget.close() gridLayout.removeWidget(widget)
It works for me perfectly– HOuadhour
Jan 24 at 19:12
add a comment |
You can use the close()
method of widget
:
for i in range(layout.count()): layout.itemAt(i).widget().close()
add a comment |
You can use the close()
method of widget
:
for i in range(layout.count()): layout.itemAt(i).widget().close()
add a comment |
You can use the close()
method of widget
:
for i in range(layout.count()): layout.itemAt(i).widget().close()
You can use the close()
method of widget
:
for i in range(layout.count()): layout.itemAt(i).widget().close()
edited Nov 28 '11 at 16:28
Justin
65.4k40186326
65.4k40186326
answered Nov 28 '11 at 16:21
Volodymyr PavlenkoVolodymyr Pavlenko
428513
428513
add a comment |
add a comment |
That's how I clear a layout :
def clearLayout(layout):
if layout != None:
while layout.count():
child = layout.takeAt(0)
if child.widget() is not None:
child.widget().deleteLater()
elif child.layout() is not None:
clearLayout(child.layout())
add a comment |
That's how I clear a layout :
def clearLayout(layout):
if layout != None:
while layout.count():
child = layout.takeAt(0)
if child.widget() is not None:
child.widget().deleteLater()
elif child.layout() is not None:
clearLayout(child.layout())
add a comment |
That's how I clear a layout :
def clearLayout(layout):
if layout != None:
while layout.count():
child = layout.takeAt(0)
if child.widget() is not None:
child.widget().deleteLater()
elif child.layout() is not None:
clearLayout(child.layout())
That's how I clear a layout :
def clearLayout(layout):
if layout != None:
while layout.count():
child = layout.takeAt(0)
if child.widget() is not None:
child.widget().deleteLater()
elif child.layout() is not None:
clearLayout(child.layout())
answered Apr 15 '14 at 14:42
user3369214user3369214
181419
181419
add a comment |
add a comment |
My solution to this problem is to override the setLayout method of QWidget. The following code updates the layout to the new layout which may or may not contain items that are already displayed. You can simply create a new layout object, add whatever you want to it, then call setLayout. Of course, you can also just call clearLayout to remove everything.
def setLayout(self, layout):
self.clearLayout()
QWidget.setLayout(self, layout)
def clearLayout(self):
if self.layout() is not None:
old_layout = self.layout()
for i in reversed(range(old_layout.count())):
old_layout.itemAt(i).widget().setParent(None)
import sip
sip.delete(old_layout)
add a comment |
My solution to this problem is to override the setLayout method of QWidget. The following code updates the layout to the new layout which may or may not contain items that are already displayed. You can simply create a new layout object, add whatever you want to it, then call setLayout. Of course, you can also just call clearLayout to remove everything.
def setLayout(self, layout):
self.clearLayout()
QWidget.setLayout(self, layout)
def clearLayout(self):
if self.layout() is not None:
old_layout = self.layout()
for i in reversed(range(old_layout.count())):
old_layout.itemAt(i).widget().setParent(None)
import sip
sip.delete(old_layout)
add a comment |
My solution to this problem is to override the setLayout method of QWidget. The following code updates the layout to the new layout which may or may not contain items that are already displayed. You can simply create a new layout object, add whatever you want to it, then call setLayout. Of course, you can also just call clearLayout to remove everything.
def setLayout(self, layout):
self.clearLayout()
QWidget.setLayout(self, layout)
def clearLayout(self):
if self.layout() is not None:
old_layout = self.layout()
for i in reversed(range(old_layout.count())):
old_layout.itemAt(i).widget().setParent(None)
import sip
sip.delete(old_layout)
My solution to this problem is to override the setLayout method of QWidget. The following code updates the layout to the new layout which may or may not contain items that are already displayed. You can simply create a new layout object, add whatever you want to it, then call setLayout. Of course, you can also just call clearLayout to remove everything.
def setLayout(self, layout):
self.clearLayout()
QWidget.setLayout(self, layout)
def clearLayout(self):
if self.layout() is not None:
old_layout = self.layout()
for i in reversed(range(old_layout.count())):
old_layout.itemAt(i).widget().setParent(None)
import sip
sip.delete(old_layout)
answered Apr 19 '11 at 0:38
joshuajoshua
2,2311116
2,2311116
add a comment |
add a comment |
From the docs:
To remove a widget from a layout, call
removeWidget()
. CallingQWidget.hide()
on a widget also effectively removes the widget from the layout untilQWidget.show()
is called.
removeWidget
is inherited from QLayout
, that's why it's not listed among the QGridLayout
methods.
But removeWidget() takes a widget as an argumentremoveWidget (self, QWidget w)
. I don't have the reference to the widget.
– Falmarri
Dec 24 '10 at 22:23
1
@Falmarri:grid.itemAt(idx).widget()
to go by index.
– user395760
Dec 24 '10 at 23:26
add a comment |
From the docs:
To remove a widget from a layout, call
removeWidget()
. CallingQWidget.hide()
on a widget also effectively removes the widget from the layout untilQWidget.show()
is called.
removeWidget
is inherited from QLayout
, that's why it's not listed among the QGridLayout
methods.
But removeWidget() takes a widget as an argumentremoveWidget (self, QWidget w)
. I don't have the reference to the widget.
– Falmarri
Dec 24 '10 at 22:23
1
@Falmarri:grid.itemAt(idx).widget()
to go by index.
– user395760
Dec 24 '10 at 23:26
add a comment |
From the docs:
To remove a widget from a layout, call
removeWidget()
. CallingQWidget.hide()
on a widget also effectively removes the widget from the layout untilQWidget.show()
is called.
removeWidget
is inherited from QLayout
, that's why it's not listed among the QGridLayout
methods.
From the docs:
To remove a widget from a layout, call
removeWidget()
. CallingQWidget.hide()
on a widget also effectively removes the widget from the layout untilQWidget.show()
is called.
removeWidget
is inherited from QLayout
, that's why it's not listed among the QGridLayout
methods.
answered Dec 24 '10 at 21:31
user395760
But removeWidget() takes a widget as an argumentremoveWidget (self, QWidget w)
. I don't have the reference to the widget.
– Falmarri
Dec 24 '10 at 22:23
1
@Falmarri:grid.itemAt(idx).widget()
to go by index.
– user395760
Dec 24 '10 at 23:26
add a comment |
But removeWidget() takes a widget as an argumentremoveWidget (self, QWidget w)
. I don't have the reference to the widget.
– Falmarri
Dec 24 '10 at 22:23
1
@Falmarri:grid.itemAt(idx).widget()
to go by index.
– user395760
Dec 24 '10 at 23:26
But removeWidget() takes a widget as an argument
removeWidget (self, QWidget w)
. I don't have the reference to the widget.– Falmarri
Dec 24 '10 at 22:23
But removeWidget() takes a widget as an argument
removeWidget (self, QWidget w)
. I don't have the reference to the widget.– Falmarri
Dec 24 '10 at 22:23
1
1
@Falmarri:
grid.itemAt(idx).widget()
to go by index.– user395760
Dec 24 '10 at 23:26
@Falmarri:
grid.itemAt(idx).widget()
to go by index.– user395760
Dec 24 '10 at 23:26
add a comment |
I use:
while layout.count() > 0:
layout.itemAt(0).setParent(None)
add a comment |
I use:
while layout.count() > 0:
layout.itemAt(0).setParent(None)
add a comment |
I use:
while layout.count() > 0:
layout.itemAt(0).setParent(None)
I use:
while layout.count() > 0:
layout.itemAt(0).setParent(None)
answered Oct 8 '14 at 15:57
SoloPilotSoloPilot
1,0351215
1,0351215
add a comment |
add a comment |
A couple of solutions, if you are swapping between known views using a stacked widget and just flipping the shown index might be a lot easier than adding and removing single widgets from a layout.
If you want to replace all the children of a widget then the QObject
functions findChildren
should get you there e.g. I don't know how the template functions are wrapped in pyqt though. But you could also search for the widgets by name if you know them.
Well this is my first Qt app (other than just messing with it) so I'm sure I'm doing a lot of things really inefficiently. For notplot_layout
will only have 1 widget at a time (I made it a gridlayout for future expansion). The widget it has is a custom widget that extends matplotlib'sFigureCanvasQTAgg
. I have a lot of plots, and what I want to do is when the user clicks on a plot in a treeview (this is working), I want to show that plot.
– Falmarri
Dec 25 '10 at 19:16
Maybe it might be a better idea to move the plotting code from theFigureCanvasQTAgg
subclasses to a separate place, just updating a givenFigure
on request. Then you can simply redraw the plot instead of messing with widgets and layouts. See eli.thegreenplace.net/files/prog_code/qt_mpl_bars.py.txt for example code.
– Ferdinand Beyer
May 9 '11 at 15:26
add a comment |
A couple of solutions, if you are swapping between known views using a stacked widget and just flipping the shown index might be a lot easier than adding and removing single widgets from a layout.
If you want to replace all the children of a widget then the QObject
functions findChildren
should get you there e.g. I don't know how the template functions are wrapped in pyqt though. But you could also search for the widgets by name if you know them.
Well this is my first Qt app (other than just messing with it) so I'm sure I'm doing a lot of things really inefficiently. For notplot_layout
will only have 1 widget at a time (I made it a gridlayout for future expansion). The widget it has is a custom widget that extends matplotlib'sFigureCanvasQTAgg
. I have a lot of plots, and what I want to do is when the user clicks on a plot in a treeview (this is working), I want to show that plot.
– Falmarri
Dec 25 '10 at 19:16
Maybe it might be a better idea to move the plotting code from theFigureCanvasQTAgg
subclasses to a separate place, just updating a givenFigure
on request. Then you can simply redraw the plot instead of messing with widgets and layouts. See eli.thegreenplace.net/files/prog_code/qt_mpl_bars.py.txt for example code.
– Ferdinand Beyer
May 9 '11 at 15:26
add a comment |
A couple of solutions, if you are swapping between known views using a stacked widget and just flipping the shown index might be a lot easier than adding and removing single widgets from a layout.
If you want to replace all the children of a widget then the QObject
functions findChildren
should get you there e.g. I don't know how the template functions are wrapped in pyqt though. But you could also search for the widgets by name if you know them.
A couple of solutions, if you are swapping between known views using a stacked widget and just flipping the shown index might be a lot easier than adding and removing single widgets from a layout.
If you want to replace all the children of a widget then the QObject
functions findChildren
should get you there e.g. I don't know how the template functions are wrapped in pyqt though. But you could also search for the widgets by name if you know them.
answered Dec 25 '10 at 16:42
Harald ScheirichHarald Scheirich
8,8912348
8,8912348
Well this is my first Qt app (other than just messing with it) so I'm sure I'm doing a lot of things really inefficiently. For notplot_layout
will only have 1 widget at a time (I made it a gridlayout for future expansion). The widget it has is a custom widget that extends matplotlib'sFigureCanvasQTAgg
. I have a lot of plots, and what I want to do is when the user clicks on a plot in a treeview (this is working), I want to show that plot.
– Falmarri
Dec 25 '10 at 19:16
Maybe it might be a better idea to move the plotting code from theFigureCanvasQTAgg
subclasses to a separate place, just updating a givenFigure
on request. Then you can simply redraw the plot instead of messing with widgets and layouts. See eli.thegreenplace.net/files/prog_code/qt_mpl_bars.py.txt for example code.
– Ferdinand Beyer
May 9 '11 at 15:26
add a comment |
Well this is my first Qt app (other than just messing with it) so I'm sure I'm doing a lot of things really inefficiently. For notplot_layout
will only have 1 widget at a time (I made it a gridlayout for future expansion). The widget it has is a custom widget that extends matplotlib'sFigureCanvasQTAgg
. I have a lot of plots, and what I want to do is when the user clicks on a plot in a treeview (this is working), I want to show that plot.
– Falmarri
Dec 25 '10 at 19:16
Maybe it might be a better idea to move the plotting code from theFigureCanvasQTAgg
subclasses to a separate place, just updating a givenFigure
on request. Then you can simply redraw the plot instead of messing with widgets and layouts. See eli.thegreenplace.net/files/prog_code/qt_mpl_bars.py.txt for example code.
– Ferdinand Beyer
May 9 '11 at 15:26
Well this is my first Qt app (other than just messing with it) so I'm sure I'm doing a lot of things really inefficiently. For not
plot_layout
will only have 1 widget at a time (I made it a gridlayout for future expansion). The widget it has is a custom widget that extends matplotlib's FigureCanvasQTAgg
. I have a lot of plots, and what I want to do is when the user clicks on a plot in a treeview (this is working), I want to show that plot.– Falmarri
Dec 25 '10 at 19:16
Well this is my first Qt app (other than just messing with it) so I'm sure I'm doing a lot of things really inefficiently. For not
plot_layout
will only have 1 widget at a time (I made it a gridlayout for future expansion). The widget it has is a custom widget that extends matplotlib's FigureCanvasQTAgg
. I have a lot of plots, and what I want to do is when the user clicks on a plot in a treeview (this is working), I want to show that plot.– Falmarri
Dec 25 '10 at 19:16
Maybe it might be a better idea to move the plotting code from the
FigureCanvasQTAgg
subclasses to a separate place, just updating a given Figure
on request. Then you can simply redraw the plot instead of messing with widgets and layouts. See eli.thegreenplace.net/files/prog_code/qt_mpl_bars.py.txt for example code.– Ferdinand Beyer
May 9 '11 at 15:26
Maybe it might be a better idea to move the plotting code from the
FigureCanvasQTAgg
subclasses to a separate place, just updating a given Figure
on request. Then you can simply redraw the plot instead of messing with widgets and layouts. See eli.thegreenplace.net/files/prog_code/qt_mpl_bars.py.txt for example code.– Ferdinand Beyer
May 9 '11 at 15:26
add a comment |
this my first time actually answering a stack overflow question but I saw that all the answers here are slightly wrong. (yes, I know that the question was to delete all widgets) The problem with most of them is that they don't account for nested layouts, so I made a recursive function, that given a layout it will recursively delete everything inside it, and all the layouts inside of it. here it is:
def clearLayout(layout):
print("-- -- input layout: "+str(layout))
for i in reversed(range(layout.count())):
layoutItem = layout.itemAt(i)
if layoutItem.widget() is not None:
widgetToRemove = layoutItem.widget()
print("found widget: " + str(widgetToRemove))
widgetToRemove.setParent(None)
layout.removeWidget(widgetToRemove)
elif layoutItem.spacerItem() is not None:
print("found spacer: " + str(layoutItem.spacerItem()))
else:
layoutToRemove = layout.itemAt(i)
print("-- found Layout: "+str(layoutToRemove))
clearLayout(layoutToRemove)
I might not have accounted for all UI types, not sure. Hope this helps!
add a comment |
this my first time actually answering a stack overflow question but I saw that all the answers here are slightly wrong. (yes, I know that the question was to delete all widgets) The problem with most of them is that they don't account for nested layouts, so I made a recursive function, that given a layout it will recursively delete everything inside it, and all the layouts inside of it. here it is:
def clearLayout(layout):
print("-- -- input layout: "+str(layout))
for i in reversed(range(layout.count())):
layoutItem = layout.itemAt(i)
if layoutItem.widget() is not None:
widgetToRemove = layoutItem.widget()
print("found widget: " + str(widgetToRemove))
widgetToRemove.setParent(None)
layout.removeWidget(widgetToRemove)
elif layoutItem.spacerItem() is not None:
print("found spacer: " + str(layoutItem.spacerItem()))
else:
layoutToRemove = layout.itemAt(i)
print("-- found Layout: "+str(layoutToRemove))
clearLayout(layoutToRemove)
I might not have accounted for all UI types, not sure. Hope this helps!
add a comment |
this my first time actually answering a stack overflow question but I saw that all the answers here are slightly wrong. (yes, I know that the question was to delete all widgets) The problem with most of them is that they don't account for nested layouts, so I made a recursive function, that given a layout it will recursively delete everything inside it, and all the layouts inside of it. here it is:
def clearLayout(layout):
print("-- -- input layout: "+str(layout))
for i in reversed(range(layout.count())):
layoutItem = layout.itemAt(i)
if layoutItem.widget() is not None:
widgetToRemove = layoutItem.widget()
print("found widget: " + str(widgetToRemove))
widgetToRemove.setParent(None)
layout.removeWidget(widgetToRemove)
elif layoutItem.spacerItem() is not None:
print("found spacer: " + str(layoutItem.spacerItem()))
else:
layoutToRemove = layout.itemAt(i)
print("-- found Layout: "+str(layoutToRemove))
clearLayout(layoutToRemove)
I might not have accounted for all UI types, not sure. Hope this helps!
this my first time actually answering a stack overflow question but I saw that all the answers here are slightly wrong. (yes, I know that the question was to delete all widgets) The problem with most of them is that they don't account for nested layouts, so I made a recursive function, that given a layout it will recursively delete everything inside it, and all the layouts inside of it. here it is:
def clearLayout(layout):
print("-- -- input layout: "+str(layout))
for i in reversed(range(layout.count())):
layoutItem = layout.itemAt(i)
if layoutItem.widget() is not None:
widgetToRemove = layoutItem.widget()
print("found widget: " + str(widgetToRemove))
widgetToRemove.setParent(None)
layout.removeWidget(widgetToRemove)
elif layoutItem.spacerItem() is not None:
print("found spacer: " + str(layoutItem.spacerItem()))
else:
layoutToRemove = layout.itemAt(i)
print("-- found Layout: "+str(layoutToRemove))
clearLayout(layoutToRemove)
I might not have accounted for all UI types, not sure. Hope this helps!
answered Aug 5 '18 at 3:09


Arthur FakhreddineArthur Fakhreddine
6114
6114
add a comment |
add a comment |
for i in reversed (range(layout.count())):
layout.itemAt(i).widget().close()
layout.takeAt(i)
or
for i in range(layout.count()):
layout.itemAt(0).widget().close()
layout.takeAt(0)
add a comment |
for i in reversed (range(layout.count())):
layout.itemAt(i).widget().close()
layout.takeAt(i)
or
for i in range(layout.count()):
layout.itemAt(0).widget().close()
layout.takeAt(0)
add a comment |
for i in reversed (range(layout.count())):
layout.itemAt(i).widget().close()
layout.takeAt(i)
or
for i in range(layout.count()):
layout.itemAt(0).widget().close()
layout.takeAt(0)
for i in reversed (range(layout.count())):
layout.itemAt(i).widget().close()
layout.takeAt(i)
or
for i in range(layout.count()):
layout.itemAt(0).widget().close()
layout.takeAt(0)
answered Dec 15 '12 at 23:15


borovskyborovsky
499514
499514
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f4528347%2fclear-all-widgets-in-a-layout-in-pyqt%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
lujJ NPlz,HAuP8JJ8ysOr WV2 oK cXOPUQPC,FZUODEtt 1HWgfIeDHGOIBiElvf