RPi.GPIO interrupt not calling function long enough
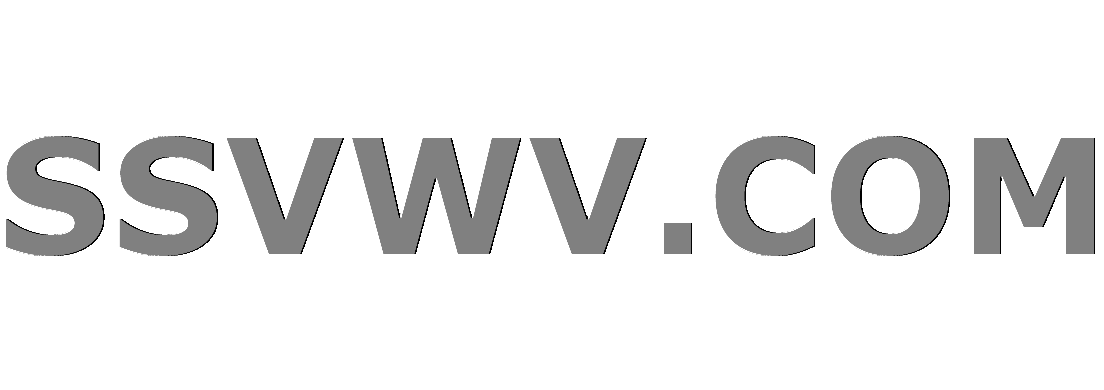
Multi tool use
up vote
1
down vote
favorite
So I'm trying to design an LCD screen to have a Menu and a bunch of different functions and its supposed to take 4 buttons. A select, a menu, and an up and down. Right now I'm just trying to work on the Menu button. I want it to always display the clock unless the menu button is pressed. But when I press the button it doesn't stay on screen for the 5 seconds it's supposed to. I have no idea why it wont either. I've looked up the RPi interrupt and followed it, but it still won't stay up. The "hi" appears for not even half a second. I'm also using the raspberry pi spy's LCD library which I can link if needed be. The only thing I did was modify some timing things to match those of my displays and added the abilty to add text to any of the four rows at once seeings that I have a 20x4 display. If someone could help me, it would be greatly appreciated.
Here's the code:
#!usr/bin/python3
import RPi.GPIO as GPIO
import time
import sys
sys.path.append('/home/pi/Downloads')
import lcd
GPIO.setwarnings(False)
GPIO.setmode(GPIO.BOARD)
#GPIO.setup(33, GPIO.IN, pull_up_down=GPIO.PUD_UP)
GPIO.setup(11, GPIO.IN, pull_up_down=GPIO.PUD_UP)
#GPIO.setup(13, GPIO.IN, pull_up_down=GPIO.PUD_UP)
#GPIO.setup(15, GPIO.IN, pull_up_down=GPIO.PUD_UP)
lcd.lcd_init()
def start():
for x in range(0,8):
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[}>----TestOS----<]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[<>---TestOS-----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-<>--TestOS-----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[--<>-TestOS-----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[---<>TestOS-----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[----<TestOS>----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-----TestOS<>---]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-----TestOS-<>--]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-----TestOS--<>-]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-----TestOS---<>"), 1)
lcd.lcd_byte(lcd.LCD_LINE_3, lcd.LCD_CMD)
lcd.lcd_string(time.strftime(">----TestOS----<]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[<>---TestOS-----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-<>--TestOS-----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[--<>-TestOS-----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[---<>TestOS-----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[----<TestOS>----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-----TestOS<>---]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-----TestOS-<>--]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-----TestOS--<>-]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-----TestOS---<>%I:%M %p >----TestOS----<]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[<>---TestOS-----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-<>--TestOS-----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[--<>-TestOS-----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[---<>TestOS-----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[----<TestOS>----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-----TestOS<>---]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-----TestOS-<>--]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-----TestOS--<>-]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-----TestOS---<>]", 1)
time.sleep(0.1)
lcd.lcd_init()
for x in range(0,120):
lcd.lcd_byte(0xFF,True)
time.sleep(5)
lcd.lcd_init()
def Menu():
lcd.lcd_init()
lcd.lcd_byte(lcd.LCD_LINE_1, lcd.LCD_CMD)
lcd.lcd_string("hi", 1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("hi", 1)
time.sleep(5)
def clock():
lcd.lcd_byte(lcd.LCD_LINE_1, lcd.LCD_CMD)
lcd.lcd_string("+------------------+", 1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string(time.strftime(">----TestOS----<]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[<>---TestOS-----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-<>--TestOS-----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[--<>-TestOS-----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[---<>TestOS-----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[----<TestOS>----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-----TestOS<>---]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-----TestOS-<>--]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-----TestOS--<>-]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-----TestOS---<>improve this question
edited Nov 10 at 17:35


PGCodeRider
2,1611725
2,1611725
asked Nov 8 at 0:21


Charlie C
132
132
add a comment >----TestOS----<]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[<>---TestOS-----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-<>--TestOS-----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[--<>-TestOS-----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[---<>TestOS-----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[----<TestOS>----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-----TestOS<>---]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-----TestOS-<>--]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-----TestOS--<>-]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-----TestOS---<>
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
Your method clock() which is running in infinite loop, always override text on display with time.
You must stop clock() method when Menu() function runs.
I added global variable menu pressed:
#!usr/bin/python3
import RPi.GPIO as GPIO
import time
import sys
sys.path.append('/home/pi/Downloads')
import lcd
GPIO.setwarnings(False)
GPIO.setmode(GPIO.BOARD)
#GPIO.setup(33, GPIO.IN, pull_up_down=GPIO.PUD_UP)
GPIO.setup(11, GPIO.IN, pull_up_down=GPIO.PUD_UP)
#GPIO.setup(13, GPIO.IN, pull_up_down=GPIO.PUD_UP)
#GPIO.setup(15, GPIO.IN, pull_up_down=GPIO.PUD_UP)
menupressed = False
lcd.lcd_init()
def start():
for x in range(0,8):
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[>----TestOS----<]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[<>---TestOS-----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-<>--TestOS-----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[--<>-TestOS-----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[---<>TestOS-----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[----<TestOS>----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-----TestOS<>---]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-----TestOS-<>--]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-----TestOS--<>-]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-----TestOS---<>
up vote
1
down vote
Your method clock() which is running in infinite loop, always override text on display with time.
You must stop clock() method when Menu() function runs.
I added global variable menu pressed:
#!usr/bin/python3
import RPi.GPIO as GPIO
import time
import sys
sys.path.append('/home/pi/Downloads')
import lcd
GPIO.setwarnings(False)
GPIO.setmode(GPIO.BOARD)
#GPIO.setup(33, GPIO.IN, pull_up_down=GPIO.PUD_UP)
GPIO.setup(11, GPIO.IN, pull_up_down=GPIO.PUD_UP)
#GPIO.setup(13, GPIO.IN, pull_up_down=GPIO.PUD_UP)
#GPIO.setup(15, GPIO.IN, pull_up_down=GPIO.PUD_UP)
menupressed = False
lcd.lcd_init()
def start():
for x in range(0,8):
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[>----TestOS----<]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[<>---TestOS-----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-<>--TestOS-----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[--<>-TestOS-----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[---<>TestOS-----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[----<TestOS>----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-----TestOS<>---]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-----TestOS-<>--]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-----TestOS--<>-]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-----TestOS---<>improve this answer
So, now it does stay on the screen for the 5 seconds its supposed to but, now it calls it twice before going back to the clock. And I tried to figure it out but I dont have any clue why
– Charlie C
Nov 9 at 19:31
Does it run twice everytime? Did you try to increase bounce time? I have no other idea.
– Koxo
Nov 11 at 0:29
1
What type of LCD display are you using?
– Koxo
Nov 11 at 1:26
I tried the bouncetime and nothing changed. The site for the display is adafru.it/198
– Charlie C
Nov 12 at 0:05
I should have one at home. I will try this code when I will have more time.
– Koxo
Nov 15 at 12:13
add a comment >----TestOS----<]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[<>---TestOS-----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-<>--TestOS-----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[--<>-TestOS-----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[---<>TestOS-----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[----<TestOS>----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-----TestOS<>---]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-----TestOS-<>--]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-----TestOS--<>-]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-----TestOS---<>%a, %b %d, %Y >----TestOS----<]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[<>---TestOS-----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-<>--TestOS-----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[--<>-TestOS-----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[---<>TestOS-----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[----<TestOS>----]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-----TestOS<>---]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-----TestOS-<>--]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-----TestOS--<>-]", 1)
time.sleep(0.1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("[-----TestOS---<>{]", 1)
time.sleep(0.1)
lcd.lcd_init()
for x in range(0,120):
lcd.lcd_byte(0xFF,True)
time.sleep(5)
lcd.lcd_init()
def Menu():
global menupressed
menupressed = True
lcd.lcd_init()
lcd.lcd_byte(lcd.LCD_LINE_1, lcd.LCD_CMD)
lcd.lcd_string("hi", 1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string("hi", 1)
time.sleep(5)
menupressed = False
def clock():
if(menupressed):
return #when menu button is pressed, return - dont show nothing on display
lcd.lcd_byte(lcd.LCD_LINE_1, lcd.LCD_CMD)
lcd.lcd_string("+------------------+", 1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string(time.strftime("|%I:%M %p |"), 1)
lcd.lcd_byte(lcd.LCD_LINE_3, lcd.LCD_CMD)
lcd.lcd_string(time.strftime("|%a, %b %d, %Y |"), 1)
lcd.lcd_byte(lcd.LCD_LINE_4, lcd.LCD_CMD)
lcd.lcd_string("+------------------+", 1)
time.sleep(1)
lcd.lcd_byte(lcd.LCD_LINE_1, lcd.LCD_CMD)
lcd.lcd_string("+------------------+", 1)
lcd.lcd_byte(lcd.LCD_LINE_2, lcd.LCD_CMD)
lcd.lcd_string(time.strftime("|%I %M %p |"), 1)
lcd.lcd_byte(lcd.LCD_LINE_3, lcd.LCD_CMD)
lcd.lcd_string(time.strftime("|%a, %b %d, %Y |"), 1)
lcd.lcd_byte(lcd.LCD_LINE_4, lcd.LCD_CMD)
lcd.lcd_string("+------------------+", 1)
time.sleep(1)
GPIO.add_event_detect(11, GPIO.FALLING, callback=Menu, bouncetime=100)
start()
try:
while True:
clock()
finally:
lcd.lcd_init()
lcd.GPIO.cleanup()
GPIO.cleanup()
Hope it was helpful
answered Nov 9 at 8:08


Koxo
694
694
So, now it does stay on the screen for the 5 seconds its supposed to but, now it calls it twice before going back to the clock. And I tried to figure it out but I dont have any clue why
– Charlie C
Nov 9 at 19:31
Does it run twice everytime? Did you try to increase bounce time? I have no other idea.
– Koxo
Nov 11 at 0:29
1
What type of LCD display are you using?
– Koxo
Nov 11 at 1:26
I tried the bouncetime and nothing changed. The site for the display is adafru.it/198
– Charlie C
Nov 12 at 0:05
I should have one at home. I will try this code when I will have more time.
– Koxo
Nov 15 at 12:13
add a comment |
So, now it does stay on the screen for the 5 seconds its supposed to but, now it calls it twice before going back to the clock. And I tried to figure it out but I dont have any clue why
– Charlie C
Nov 9 at 19:31
Does it run twice everytime? Did you try to increase bounce time? I have no other idea.
– Koxo
Nov 11 at 0:29
1
What type of LCD display are you using?
– Koxo
Nov 11 at 1:26
I tried the bouncetime and nothing changed. The site for the display is adafru.it/198
– Charlie C
Nov 12 at 0:05
I should have one at home. I will try this code when I will have more time.
– Koxo
Nov 15 at 12:13
So, now it does stay on the screen for the 5 seconds its supposed to but, now it calls it twice before going back to the clock. And I tried to figure it out but I dont have any clue why
– Charlie C
Nov 9 at 19:31
So, now it does stay on the screen for the 5 seconds its supposed to but, now it calls it twice before going back to the clock. And I tried to figure it out but I dont have any clue why
– Charlie C
Nov 9 at 19:31
Does it run twice everytime? Did you try to increase bounce time? I have no other idea.
– Koxo
Nov 11 at 0:29
Does it run twice everytime? Did you try to increase bounce time? I have no other idea.
– Koxo
Nov 11 at 0:29
1
1
What type of LCD display are you using?
– Koxo
Nov 11 at 1:26
What type of LCD display are you using?
– Koxo
Nov 11 at 1:26
I tried the bouncetime and nothing changed. The site for the display is adafru.it/198
– Charlie C
Nov 12 at 0:05
I tried the bouncetime and nothing changed. The site for the display is adafru.it/198
– Charlie C
Nov 12 at 0:05
I should have one at home. I will try this code when I will have more time.
– Koxo
Nov 15 at 12:13
I should have one at home. I will try this code when I will have more time.
– Koxo
Nov 15 at 12:13
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53199871%2frpi-gpio-interrupt-not-calling-function-long-enough%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown